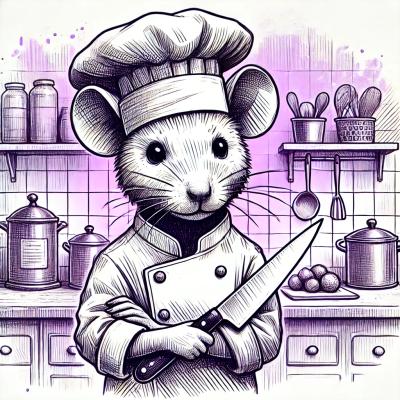
Research
Security News
Quasar RAT Disguised as an npm Package for Detecting Vulnerabilities in Ethereum Smart Contracts
Socket researchers uncover a malicious npm package posing as a tool for detecting vulnerabilities in Etherium smart contracts.
@rabby-wallet/binance-connector
Advanced tools
This is a lightweight library that works as a connector to the Binance public API.
This is a lightweight library that works as a connector to Binance public API. It’s designed to be simple, clean, and easy to use with minimal dependencies.
/api/*
/sapi/*
npm install @binance/connector
https://binance.github.io/binance-connector-node/
const { Spot } = require('@binance/connector')
const apiKey = ''
const apiSecret = ''
const client = new Spot(apiKey, apiSecret)
// Get account information
client.account().then(response => client.logger.log(response.data))
// Place a new order
client.newOrder('BNBUSDT', 'BUY', 'LIMIT', {
price: '350',
quantity: 1,
timeInForce: 'GTC'
}).then(response => client.logger.log(response.data))
.catch(error => client.logger.error(error))
Please find examples
folder to check for more endpoints.
const { Spot } = require('@binance/connector')
const apiKey = ''
const apiSecret = '' // has no effect when RSA private key is provided
// load private key
const privateKey = fs.readFileSync('/Users/john/ssl/private_key_encrypted.pem')
const privateKeyPassphrase = 'password'
const client = new Spot(apiKey, apiSecret, {
privateKey,
privateKeyPassphrase // only used for encrypted key
})
// Get account information
client.account().then(response => client.logger.log(response.data))
While /sapi/*
endpoints don't have testnet environment yet, /api/*
endpoints can be tested in
Spot Testnet. You can use it by changing the base URL:
// provide the testnet base url
const client = new Spot(apiKey, apiSecret, { baseURL: 'https://testnet.binance.vision'})
If base_url
is not provided, it defaults to api.binance.com
.
It's recommended to pass in the base_url
parameter, even in production as Binance provides alternative URLs in case of performance issues:
https://api1.binance.com
https://api2.binance.com
https://api3.binance.com
Optional parameters are encapsulated to a single object as the last function parameter.
const { Spot } = require('@binance/connector')
const apiKey = ''
const apiSecret = ''
const client = new Spot(apiKey, apiSecret)
client.account({ recvWindow: 2000 }).then(response => client.logger.log(response.data))
It's easy to set timeout in milliseconds in request. If the request take longer than timeout, the request will be aborted. If it's not set, there will be no timeout.
const { Spot } = require('@binance/connector')
const apiKey = ''
const apiSecret = ''
const client = new Spot(apiKey, apiSecret, { timeout: 1000 })
client.account()
.then(response => client.logger.log(response.data))
.catch(error => client.logger.error(error.message))
The axios
package is used as the http client in this library. A proxy settings is passed into axios
directly, the details can be found at here:
const { Spot } = require('@binance/connector')
const apiKey = ''
const apiSecret = ''
const client = new Spot(apiKey, apiSecret,
{
proxy: {
protocol: 'https',
host: '127.0.0.1',
port: 9000,
auth: {
username: 'proxy_user',
password: 'password'
}
}
}
)
You may have a HTTP proxy, that can bring the problem that you need to make a HTTPS connection through the HTTP proxy. You can do that by build a HTTPS-over-HTTP tunnel by npm package tunnel, and then pass the turnnel agent to httpsAgent
in axios
.
const tunnel = require('tunnel')
const agent = tunnel.httpsOverHttp({
proxy: {
host: "127.0.0.1",
port: 3128
}
})
const client = new Spot(null, null,
{
baseURL: "https://api.binance.com",
httpsAgent: agent
}
)
client.time()
.then(response => client.logger.log(response.data))
.catch(error => client.logger.error(error))
This comment provides more details.
The Binance API server provides weight usages in the headers of each response. This information can be fetched from headers
property. x-mbx-used-weight
and x-mbx-used-weight-1m
show the total weight consumed within 1 minute.
// client initialization is skipped
client.exchangeInfo().then(response => client.logger.log(response.headers['x-mbx-used-weight-1m']))
const Spot = require('@binance/connector')
const fs = require('fs')
const { Console } = require('console')
// make sure the logs/ folder is created beforehand
const output = fs.createWriteStream('./logs/stdout.log')
const errorOutput = fs.createWriteStream('./logs/stderr.log')
const logger = new Console({ stdout: output, stderr: errorOutput })
const client = new Spot('', '', {logger: logger})
client.exchangeInfo().then(response => client.logger.log(response.data))
// check the output file
The default logger defined in the package is Node.js Console class. Its output is sent to process.stdout
and process.stderr
, same as the global console.
There are 2 types of error that may be returned from the API server and the user has to handle it properly:
Client error
4XX
, it's an issue from client side.Response Metadata
section for more details.-1102
Unknown order sent.
// client initialization is skipped
client.exchangeInfo({ symbol: 'invalidSymbol' })
.then(response => client.logger.log(response.data))
.catch(err => {
client.logger.error(err.response.headers) // full response header
client.logger.error(err.response.status) // HTTP status code 400
client.logger.error(err.response.data) // includes both error code and message
client.logger.error(err.response.config) // includes request's config
})
Server error
5XX
, it's an issue from server side.const { Spot } = require('@binance/connector')
const client = new Spot('', '', {
wsURL: 'wss://testnet.binance.vision' // If optional base URL is not provided, wsURL defaults to wss://stream.binance.com:9443
})
const callbacks = {
open: () => client.logger.log('open'),
close: () => client.logger.log('closed'),
message: data => client.logger.log(data)
}
const aggTrade = client.aggTradeWS('bnbusdt', callbacks)
// unsubscribe the stream above
setTimeout(() => client.unsubscribe(aggTrade), 3000)
// support combined stream
const combinedStreams = client.combinedStreams(['btcusdt@miniTicker', 'ethusdt@ticker'], callbacks)
More websocket examples are available in the examples
folder
Unsubscription is achieved by closing the connection. If this method is called without any connection established, the console will output a message No connection to close.
// client initialization is skipped
const wsRef = client.aggTradeWS('bnbusdt', callbacks)
// The connection (bnbusdt@aggTrade) is closed after 3 secs.
setTimeout(() => client.unsubscribe(wsRef), 3000)
If there is a close event not initiated by the user, the reconnection mechanism will be triggered in 5 secs.
It is possible to ping server from client, and expect to receive a PONG message.
const { Console } = require('console')
const { Spot } = require('@binance/connector')
const logger = new Console({ stdout: process.stdout, stderr: process.stderr });
const client = new Spot('', '', { logger })
const callbacks = {
open: () => logger.info('open'),
close: () => logger.info('closed'),
message: data => logger.info(data)
}
const wsRef = client.userData('the_listen_key', callbacks)
setInterval(() => {
client.pingServer(wsRef)
}, 1000 * 10)
const { Console } = require('console')
const fs = require('fs')
const Spot = require('@binance/connector')
const output = fs.createWriteStream('./logs/stdout.log')
const errorOutput = fs.createWriteStream('./logs/stderr.log')
// make sure the logs/ folder is created beforehand
const logger = new Console({ stdout: output, stderr: errorOutput })
const client = new Spot('', '', {logger})
const callbacks = {
open: () => client.logger.log('open'),
close: () => client.logger.log('closed'),
message: data => client.logger.log(data)
}
const wsRef = client.aggTradeWS('bnbusdt', callbacks)
setTimeout(() => client.unsubscribe(wsRef), 5000)
// check the output file
The default logger defined in the package is Node.js Console class. Its output is sent to process.stdout
and process.stderr
, same as the global console.
Note that when the connection is initialized, the console outputs a list of callbacks in the form of listen to event: <event_name>
.
npm install
npm run test
Futures and Vanilla Options APIs are not supported:
/fapi/*
/dapi/*
/vapi/*
MIT
FAQs
This is a lightweight library that works as a connector to the Binance public API.
The npm package @rabby-wallet/binance-connector receives a total of 3 weekly downloads. As such, @rabby-wallet/binance-connector popularity was classified as not popular.
We found that @rabby-wallet/binance-connector demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 5 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
Socket researchers uncover a malicious npm package posing as a tool for detecting vulnerabilities in Etherium smart contracts.
Security News
Research
A supply chain attack on Rspack's npm packages injected cryptomining malware, potentially impacting thousands of developers.
Research
Security News
Socket researchers discovered a malware campaign on npm delivering the Skuld infostealer via typosquatted packages, exposing sensitive data.