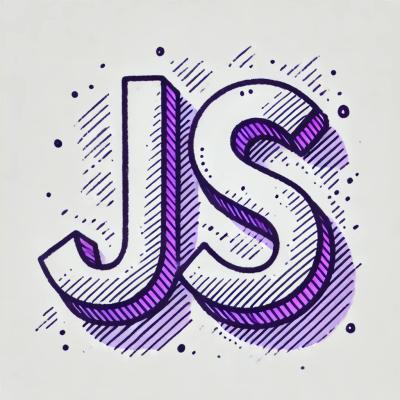
Security News
JavaScript Leaders Demand Oracle Release the JavaScript Trademark
In an open letter, JavaScript community leaders urge Oracle to give up the JavaScript trademark, arguing that it has been effectively abandoned through nonuse.
@radix-ui/react-popper
Advanced tools
@radix-ui/react-popper is a React component library that provides a set of primitives for creating popper elements, such as tooltips, dropdowns, and other floating elements. It leverages the Popper.js library to handle positioning and provides a highly customizable and accessible API.
Basic Popper
This example demonstrates a basic popper setup with an anchor element and a popper content element.
```jsx
import { Popper } from '@radix-ui/react-popper';
function BasicPopper() {
return (
<Popper>
<Popper.Anchor>
<button>Anchor</button>
</Popper.Anchor>
<Popper.Content>
<div>Popper Content</div>
</Popper.Content>
</Popper>
);
}
```
Custom Positioning
This example shows how to customize the positioning of the popper content relative to the anchor element by setting the `placement` prop.
```jsx
import { Popper } from '@radix-ui/react-popper';
function CustomPositioningPopper() {
return (
<Popper placement="right">
<Popper.Anchor>
<button>Anchor</button>
</Popper.Anchor>
<Popper.Content>
<div>Popper Content</div>
</Popper.Content>
</Popper>
);
}
```
Transition Effects
This example demonstrates how to add transition effects to the popper content by controlling the `open` state and using CSS for animations.
```jsx
import { Popper } from '@radix-ui/react-popper';
import { useState } from 'react';
function TransitionPopper() {
const [open, setOpen] = useState(false);
return (
<Popper open={open} onOpenChange={setOpen}>
<Popper.Anchor>
<button onClick={() => setOpen(!open)}>Toggle Popper</button>
</Popper.Anchor>
<Popper.Content>
<div className="popper-content">Popper Content</div>
</Popper.Content>
</Popper>
);
}
```
react-popper is a React wrapper around Popper.js, providing a set of components to create popper elements. It is similar to @radix-ui/react-popper but offers a more direct integration with Popper.js, making it a good choice for developers who need fine-grained control over popper behavior.
react-tooltip is a library specifically designed for creating tooltips in React applications. While it focuses on tooltips, it provides a simpler API compared to @radix-ui/react-popper, making it easier to use for basic tooltip functionality.
react-popper
$ yarn add @radix-ui/react-popper
# or
$ npm install @radix-ui/react-popper
This is an internal utility, not intended for public usage.
FAQs
Unknown package
The npm package @radix-ui/react-popper receives a total of 4,473,233 weekly downloads. As such, @radix-ui/react-popper popularity was classified as popular.
We found that @radix-ui/react-popper demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 6 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
In an open letter, JavaScript community leaders urge Oracle to give up the JavaScript trademark, arguing that it has been effectively abandoned through nonuse.
Security News
The initial version of the Socket Python SDK is now on PyPI, enabling developers to more easily interact with the Socket REST API in Python projects.
Security News
Floating dependency ranges in npm can introduce instability and security risks into your project by allowing unverified or incompatible versions to be installed automatically, leading to unpredictable behavior and potential conflicts.