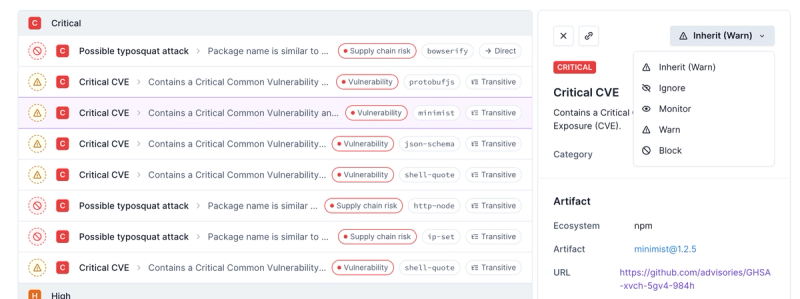
Product
Introducing Enhanced Alert Actions and Triage Functionality
Socket now supports four distinct alert actions instead of the previous two, and alert triaging allows users to override the actions taken for all individual alerts.
@react-aria/utils
Advanced tools
Package description
The @react-aria/utils package provides a collection of utility functions that help in managing accessibility concerns in React applications. These utilities assist in handling keyboard, focus, and selection behaviors, making it easier to build accessible UI components.
Focus Management
This feature allows you to focus an element without causing the browser to scroll to it, which can be useful in maintaining the user's scroll position while programmatically moving focus.
import { focusWithoutScrolling } from '@react-aria/utils';
function handleFocus(element) {
focusWithoutScrolling(element);
}
Keyboard Event Handling
This utility helps in filtering out non-DOM properties from props, ensuring that only valid HTML attributes are passed to the DOM element, thus preventing React warnings and improving HTML output.
import { filterDOMProps } from '@react-aria/utils';
function Button(props) {
return <button {...filterDOMProps(props)}>Click me</button>;
}
Merge Props
Merge props from different sources, typically used to combine default and user-provided props in a component. It ensures that event handlers and other attributes are correctly combined and overridden if necessary.
import { mergeProps } from '@react-aria/utils';
function mergeComponentProps(userProps, defaultProps) {
return mergeProps(defaultProps, userProps);
}
Downshift is a library that provides primitives to build simple, flexible, WAI-ARIA compliant enhanced input React components. Its focus on accessibility and functionality related to dropdowns and comboboxes makes it similar but more specialized compared to the broad utility focus of @react-aria/utils.
React-focus-lock provides a robust solution for trapping focus within a DOM node, which is crucial for building accessible modal dialogs. While it focuses specifically on focus management, @react-aria/utils offers this alongside other utilities, making it more versatile.
Readme
This package is part of react-spectrum. See the repo for more details.
FAQs
Spectrum UI components in React
The npm package @react-aria/utils receives a total of 1,472,738 weekly downloads. As such, @react-aria/utils popularity was classified as popular.
We found that @react-aria/utils demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 2 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Product
Socket now supports four distinct alert actions instead of the previous two, and alert triaging allows users to override the actions taken for all individual alerts.
Security News
Polyfill.io has been serving malware for months via its CDN, after the project's open source maintainer sold the service to a company based in China.
Security News
OpenSSF is warning open source maintainers to stay vigilant against reputation farming on GitHub, where users artificially inflate their status by manipulating interactions on closed issues and PRs.