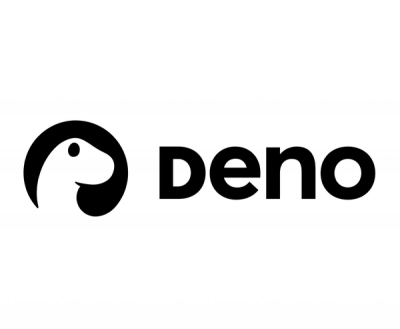
Security News
Deno 2.2 Improves Dependency Management and Expands Node.js Compatibility
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
@react-hook/event
Advanced tools
A React hook for managing event listeners, e.g. removing events when a component unmounts.
@react-hook/event is a React hook library that provides utilities for handling events in a React application. It simplifies the process of adding and removing event listeners, making it easier to manage event-driven logic in functional components.
useEvent
The `useEvent` hook allows you to create an event handler that is automatically memoized. This helps in preventing unnecessary re-renders and ensures that the event handler is stable across renders.
```javascript
import { useEvent } from '@react-hook/event';
function MyComponent() {
const handleClick = useEvent((event) => {
console.log('Button clicked!', event);
});
return <button onClick={handleClick}>Click me</button>;
}
```
useWindowEvent
The `useWindowEvent` hook allows you to add an event listener to the window object. This is useful for handling global events like window resize or scroll.
```javascript
import { useWindowEvent } from '@react-hook/event';
function MyComponent() {
useWindowEvent('resize', () => {
console.log('Window resized!');
});
return <div>Resize the window to see the effect.</div>;
}
```
useDocumentEvent
The `useDocumentEvent` hook allows you to add an event listener to the document object. This is useful for handling events that are not specific to a particular element, such as key presses.
```javascript
import { useDocumentEvent } from '@react-hook/event';
function MyComponent() {
useDocumentEvent('keydown', (event) => {
console.log('Key pressed:', event.key);
});
return <div>Press any key to see the effect.</div>;
}
```
react-use is a collection of essential React hooks, including hooks for handling events. It offers a broader range of hooks compared to @react-hook/event, making it a more versatile choice for various use cases.
ahooks is a React hooks library that provides a wide range of hooks, including event handling hooks. It is well-documented and offers more comprehensive solutions for state management, side effects, and more, compared to @react-hook/event.
use-gesture is a library for handling gestures in React applications. While it focuses more on touch and pointer events, it provides a robust API for managing complex event interactions, which can complement the functionality of @react-hook/event.
npm i @react-hook/event
A React hook for adding events to HTML elements. This hook cleans up your listeners
automatically when it unmounts. You won't have to worry about wrapping your
listener in a useCallback()
because this hook makes sure your most recent callback
is always invoked.
import * as React from 'react'
import useEvent from '@react-hook/event'
// Logs an event each time target.current is clicked
const Component = () => {
const target = useRef(null)
useEvent(target, 'click', (event) => console.log(event))
return <div ref={target} />
}
// Logs an event each time the `document` is clicked
const useLogDocumentClick = () => {
useEvent(document, 'click', (event) => console.log(event))
}
// Logs an event each time the `window` is clicked
const useLogWindowClick = () => {
useEvent(window, 'click', (event) => console.log(event))
}
// Logs an event each time element#foo` is clicked
const useLogElementClick = () => {
useEvent(document.getElementById('foo'), 'click', (event) =>
console.log(event)
)
}
const useEvent = <
// Also has Window, Document overloads
T extends HTMLElement = HTMLElement,
K extends keyof HTMLElementEventMap = keyof HTMLElementEventMap
>(
target: React.RefObject<T> | T | Window | Document | null,
type: K,
listener: EventListener<K>,
cleanup?: (...args: any[]) => any
)
Argument | Type | Required? | Description |
---|---|---|---|
target | React.RefObject<T> | T | Window | Document | null | Yes | The React ref, window , or document to add the event listener to |
type | keyof EventMap | Yes | The type of event to listen for |
listener | (this: T, ev: EventMap[K]) => any | Yes | The callback invoked when the event type fires |
cleanup | (...args: any[]) => any | No | This callback will be invoked when the event unmounts. This is in addition to the automatic event listener cleanup that occurs. A common use case could be something like clearing a timeout. |
MIT
FAQs
A React hook for managing event listeners, e.g. removing events when a component unmounts.
The npm package @react-hook/event receives a total of 166,909 weekly downloads. As such, @react-hook/event popularity was classified as popular.
We found that @react-hook/event demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
Security News
React's CRA deprecation announcement sparked community criticism over framework recommendations, leading to quick updates acknowledging build tools like Vite as valid alternatives.
Security News
Ransomware payment rates hit an all-time low in 2024 as law enforcement crackdowns, stronger defenses, and shifting policies make attacks riskier and less profitable.