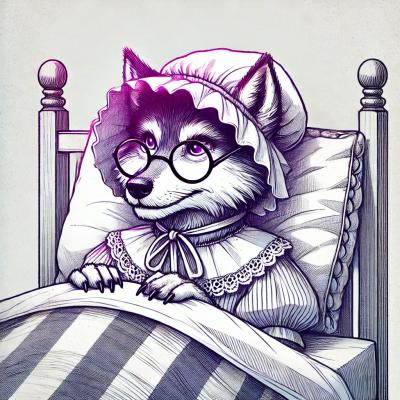
Security News
GitHub Removes Malicious Pull Requests Targeting Open Source Repositories
GitHub removed 27 malicious pull requests attempting to inject harmful code across multiple open source repositories, in another round of low-effort attacks.
@react-navigation/drawer
Advanced tools
Integration for the drawer component from react-native-drawer-layout
@react-navigation/drawer is a package for React Native that provides a customizable drawer navigator. It allows you to create a side menu that can be swiped in from the edge of the screen, providing a convenient way to navigate between different screens in your app.
Basic Drawer Navigation
This code demonstrates how to set up a basic drawer navigation with two screens: Home and Profile. The drawer can be swiped in from the edge of the screen to navigate between these screens.
import * as React from 'react';
import { NavigationContainer } from '@react-navigation/native';
import { createDrawerNavigator } from '@react-navigation/drawer';
import HomeScreen from './HomeScreen';
import ProfileScreen from './ProfileScreen';
const Drawer = createDrawerNavigator();
function MyDrawer() {
return (
<Drawer.Navigator initialRouteName="Home">
<Drawer.Screen name="Home" component={HomeScreen} />
<Drawer.Screen name="Profile" component={ProfileScreen} />
</Drawer.Navigator>
);
}
export default function App() {
return (
<NavigationContainer>
<MyDrawer />
</NavigationContainer>
);
}
Custom Drawer Content
This code demonstrates how to create a custom drawer content component. The custom drawer includes a button to close the drawer, in addition to the default drawer items.
import * as React from 'react';
import { View, Text, Button } from 'react-native';
import { NavigationContainer } from '@react-navigation/native';
import { createDrawerNavigator, DrawerContentScrollView, DrawerItemList } from '@react-navigation/drawer';
import HomeScreen from './HomeScreen';
import ProfileScreen from './ProfileScreen';
const Drawer = createDrawerNavigator();
function CustomDrawerContent(props) {
return (
<DrawerContentScrollView {...props}>
<DrawerItemList {...props} />
<Button title="Close Drawer" onPress={() => props.navigation.closeDrawer()} />
</DrawerContentScrollView>
);
}
function MyDrawer() {
return (
<Drawer.Navigator drawerContent={props => <CustomDrawerContent {...props} />}>
<Drawer.Screen name="Home" component={HomeScreen} />
<Drawer.Screen name="Profile" component={ProfileScreen} />
</Drawer.Navigator>
);
}
export default function App() {
return (
<NavigationContainer>
<MyDrawer />
</NavigationContainer>
);
}
Drawer with Custom Styles
This code demonstrates how to apply custom styles to the drawer navigator. The drawer has a custom background color and width, and the drawer items have custom styles as well.
import * as React from 'react';
import { NavigationContainer } from '@react-navigation/native';
import { createDrawerNavigator } from '@react-navigation/drawer';
import HomeScreen from './HomeScreen';
import ProfileScreen from './ProfileScreen';
const Drawer = createDrawerNavigator();
function MyDrawer() {
return (
<Drawer.Navigator
initialRouteName="Home"
drawerStyle={{
backgroundColor: '#c6cbef',
width: 240,
}}
drawerContentOptions={{
activeTintColor: '#e91e63',
itemStyle: { marginVertical: 5 },
}}
>
<Drawer.Screen name="Home" component={HomeScreen} />
<Drawer.Screen name="Profile" component={ProfileScreen} />
</Drawer.Navigator>
);
}
export default function App() {
return (
<NavigationContainer>
<MyDrawer />
</NavigationContainer>
);
}
react-native-drawer is another package for creating a drawer navigation in React Native applications. It provides a highly customizable drawer component that can be used to create side menus. Compared to @react-navigation/drawer, react-native-drawer offers more flexibility in terms of customization but requires more manual setup and configuration.
react-native-side-menu is a simple and customizable side menu component for React Native. It allows you to create a side menu that can be swiped in from the edge of the screen. While it is easier to set up compared to @react-navigation/drawer, it lacks some of the advanced features and integrations provided by @react-navigation/drawer.
react-navigation is a popular navigation library for React Native that provides a variety of navigators, including stack, tab, and drawer navigators. While @react-navigation/drawer is a specific package for drawer navigation, react-navigation offers a more comprehensive solution for handling different types of navigation in a React Native app.
@react-navigation/drawer
Drawer navigator for React Navigation following Material Design guidelines.
Installation instructions and documentation can be found on the React Navigation website.
FAQs
Integration for the drawer component from react-native-drawer-layout
The npm package @react-navigation/drawer receives a total of 233,897 weekly downloads. As such, @react-navigation/drawer popularity was classified as popular.
We found that @react-navigation/drawer demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 7 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
GitHub removed 27 malicious pull requests attempting to inject harmful code across multiple open source repositories, in another round of low-effort attacks.
Security News
RubyGems.org has added a new "maintainer" role that allows for publishing new versions of gems. This new permission type is aimed at improving security for gem owners and the service overall.
Security News
Node.js will be enforcing stricter semver-major PR policies a month before major releases to enhance stability and ensure reliable release candidates.