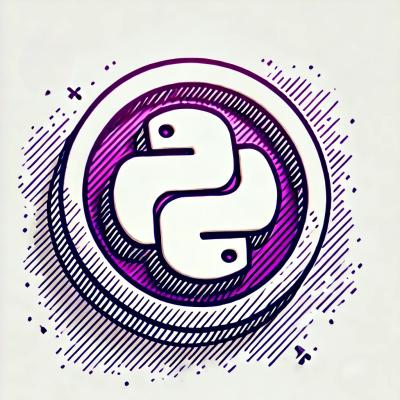
Security News
Introducing the Socket Python SDK
The initial version of the Socket Python SDK is now on PyPI, enabling developers to more easily interact with the Socket REST API in Python projects.
@react-navigation/stack
Advanced tools
Stack navigator component for iOS and Android with animated transitions and gestures
@react-navigation/stack is a library for React Native that provides a way to create stack-based navigation in your application. It allows you to navigate between different screens in a stack-like manner, where each new screen is pushed onto the stack and you can go back to the previous screen by popping the stack.
Basic Stack Navigator
This code sets up a basic stack navigator with two screens: HomeScreen and DetailsScreen. The NavigationContainer is the top-level component that manages the navigation tree.
import * as React from 'react';
import { NavigationContainer } from '@react-navigation/native';
import { createStackNavigator } from '@react-navigation/stack';
import HomeScreen from './HomeScreen';
import DetailsScreen from './DetailsScreen';
const Stack = createStackNavigator();
function App() {
return (
<NavigationContainer>
<Stack.Navigator>
<Stack.Screen name="Home" component={HomeScreen} />
<Stack.Screen name="Details" component={DetailsScreen} />
</Stack.Navigator>
</NavigationContainer>
);
}
export default App;
Custom Header
This code demonstrates how to customize the header for all screens in the stack navigator. The headerStyle, headerTintColor, and headerTitleStyle options are used to style the header.
import * as React from 'react';
import { NavigationContainer } from '@react-navigation/native';
import { createStackNavigator } from '@react-navigation/stack';
import HomeScreen from './HomeScreen';
import DetailsScreen from './DetailsScreen';
const Stack = createStackNavigator();
function App() {
return (
<NavigationContainer>
<Stack.Navigator
screenOptions={{
headerStyle: { backgroundColor: '#f4511e' },
headerTintColor: '#fff',
headerTitleStyle: { fontWeight: 'bold' },
}}
>
<Stack.Screen name="Home" component={HomeScreen} />
<Stack.Screen name="Details" component={DetailsScreen} />
</Stack.Navigator>
</NavigationContainer>
);
}
export default App;
Passing Parameters
This code shows how to pass parameters to a screen. The HomeScreen navigates to the DetailsScreen and passes parameters itemId and otherParam. The DetailsScreen then retrieves and displays these parameters.
import * as React from 'react';
import { Button, Text, View } from 'react-native';
import { NavigationContainer } from '@react-navigation/native';
import { createStackNavigator } from '@react-navigation/stack';
function HomeScreen({ navigation }) {
return (
<View style={{ flex: 1, alignItems: 'center', justifyContent: 'center' }}>
<Button
title="Go to Details"
onPress={() => navigation.navigate('Details', { itemId: 86, otherParam: 'anything you want here' })}
/>
</View>
);
}
function DetailsScreen({ route, navigation }) {
const { itemId, otherParam } = route.params;
return (
<View style={{ flex: 1, alignItems: 'center', justifyContent: 'center' }}>
<Text>Item ID: {itemId}</Text>
<Text>Other Param: {otherParam}</Text>
</View>
);
}
const Stack = createStackNavigator();
function App() {
return (
<NavigationContainer>
<Stack.Navigator>
<Stack.Screen name="Home" component={HomeScreen} />
<Stack.Screen name="Details" component={DetailsScreen} />
</Stack.Navigator>
</NavigationContainer>
);
}
export default App;
react-navigation is a popular library for routing and navigation in React Native applications. It provides a variety of navigators including stack, tab, and drawer navigators. Compared to @react-navigation/stack, it offers a more comprehensive set of navigation solutions.
react-native-navigation is a navigation library created by Wix. It provides a native navigation experience and is known for its performance and smooth animations. Unlike @react-navigation/stack, which is JavaScript-based, react-native-navigation uses native components for navigation.
react-router-native is a routing library for React Native that is based on React Router. It allows you to use the same declarative routing approach as React Router in web applications. While @react-navigation/stack is specifically designed for stack navigation, react-router-native provides a more general routing solution.
@react-navigation/stack
Stack navigator for React Navigation.
Installation instructions and documentation can be found on the React Navigation website.
FAQs
Stack navigator component for iOS and Android with animated transitions and gestures
The npm package @react-navigation/stack receives a total of 428,030 weekly downloads. As such, @react-navigation/stack popularity was classified as popular.
We found that @react-navigation/stack demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 7 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
The initial version of the Socket Python SDK is now on PyPI, enabling developers to more easily interact with the Socket REST API in Python projects.
Security News
Floating dependency ranges in npm can introduce instability and security risks into your project by allowing unverified or incompatible versions to be installed automatically, leading to unpredictable behavior and potential conflicts.
Security News
A new Rust RFC proposes "Trusted Publishing" for Crates.io, introducing short-lived access tokens via OIDC to improve security and reduce risks associated with long-lived API tokens.