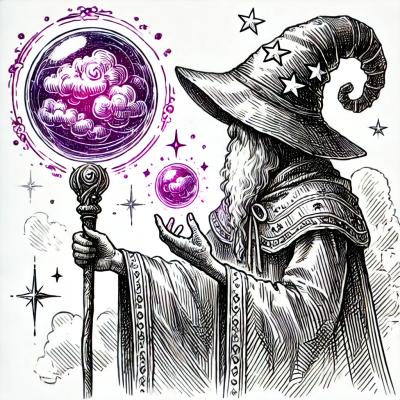
Security News
Cloudflare Adds Security.txt Setup Wizard
Cloudflare has launched a setup wizard allowing users to easily create and manage a security.txt file for vulnerability disclosure on their websites.
@react-pdf-viewer/core
Advanced tools
@react-pdf-viewer/core is a React library that provides a set of components and hooks to easily integrate PDF viewing capabilities into your React applications. It offers a customizable and extensible way to display PDF documents, navigate through pages, zoom in and out, and more.
Basic PDF Viewer
This code demonstrates how to set up a basic PDF viewer using the @react-pdf-viewer/core package. It includes the necessary imports and sets up the Worker and Viewer components to display a PDF file.
import { Worker, Viewer } from '@react-pdf-viewer/core';
import '@react-pdf-viewer/core/lib/styles/index.css';
const App = () => (
<div>
<Worker workerUrl={`https://unpkg.com/pdfjs-dist@2.6.347/build/pdf.worker.min.js`}>
<Viewer fileUrl='/path/to/your/pdf-file.pdf' />
</Worker>
</div>
);
Custom Toolbar
This code demonstrates how to create a custom toolbar for the PDF viewer. It uses the Toolbar component and slots to include buttons for toggling the sidebar, navigating pages, and zooming in and out.
import { Worker, Viewer, ToolbarSlot, Toolbar } from '@react-pdf-viewer/core';
import '@react-pdf-viewer/core/lib/styles/index.css';
const App = () => (
<div>
<Worker workerUrl={`https://unpkg.com/pdfjs-dist@2.6.347/build/pdf.worker.min.js`}>
<Toolbar>
{(slots: ToolbarSlot) => (
<>
{slots.toggleSidebarButton}
{slots.previousPageButton}
{slots.nextPageButton}
{slots.zoomOutButton}
{slots.zoomInButton}
</>
)}
</Toolbar>
<Viewer fileUrl='/path/to/your/pdf-file.pdf' />
</Worker>
</div>
);
Thumbnail View
This code demonstrates how to add a thumbnail view to the PDF viewer using the ThumbnailPlugin. It initializes the plugin and includes it in the Viewer component's plugins array.
import { Worker, Viewer, ThumbnailPlugin } from '@react-pdf-viewer/core';
import '@react-pdf-viewer/core/lib/styles/index.css';
const thumbnailPluginInstance = ThumbnailPlugin();
const App = () => (
<div>
<Worker workerUrl={`https://unpkg.com/pdfjs-dist@2.6.347/build/pdf.worker.min.js`}>
<Viewer fileUrl='/path/to/your/pdf-file.pdf' plugins={[thumbnailPluginInstance]} />
</Worker>
</div>
);
react-pdf is a popular library for displaying PDF documents in React applications. It provides a simple API for rendering PDFs and supports features like text selection, annotations, and more. Compared to @react-pdf-viewer/core, react-pdf is more focused on basic PDF rendering and may require additional customization for advanced features.
pdf-viewer-reactjs is another library for embedding PDF viewers in React applications. It offers basic functionalities like page navigation and zooming. However, it is less customizable and extensible compared to @react-pdf-viewer/core, making it suitable for simpler use cases.
pdfjs-dist is the official PDF.js library from Mozilla, which can be used to build custom PDF viewers. While it provides a lot of flexibility and control, it requires more effort to integrate into a React application compared to @react-pdf-viewer/core, which offers ready-to-use components and hooks.
FAQs
A React component to view a PDF document
The npm package @react-pdf-viewer/core receives a total of 116,858 weekly downloads. As such, @react-pdf-viewer/core popularity was classified as popular.
We found that @react-pdf-viewer/core demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Cloudflare has launched a setup wizard allowing users to easily create and manage a security.txt file for vulnerability disclosure on their websites.
Security News
The Socket Research team breaks down a malicious npm package targeting the legitimate DOMPurify library. It uses obfuscated code to hide that it is exfiltrating browser and crypto wallet data.
Security News
ENISA’s 2024 report highlights the EU’s top cybersecurity threats, including rising DDoS attacks, ransomware, supply chain vulnerabilities, and weaponized AI.