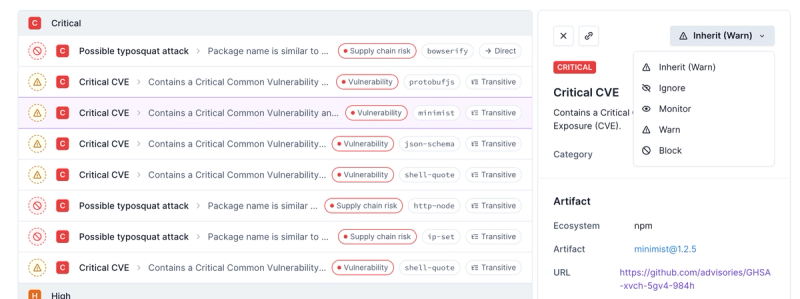
Product
Introducing Enhanced Alert Actions and Triage Functionality
Socket now supports four distinct alert actions instead of the previous two, and alert triaging allows users to override the actions taken for all individual alerts.
@react-spring/rafz
Advanced tools
Readme
Coordinate requestAnimationFrame
calls across your app and/or libraries.
ReactDOM.unstable_batchedUpdates
)
import { raf } from '@react-spring/rafz'
// Schedule an update
raf(dt => {})
// Start an update loop
raf(dt => true)
// Cancel an update
raf.cancel(fn)
// Schedule a mutation
raf.write(() => {})
// Before any updates
raf.onStart(() => {})
// Before any mutations
raf.onFrame(() => {})
// After any mutations
raf.onFinish(() => {})
// Set a timeout that runs on nearest frame
raf.setTimeout(() => {}, 1000)
// Use a polyfill
raf.use(require('@essentials/raf').raf)
// Get the current time
raf.now() // => number
// Set how you want to control raf firing
raf.frameLoop = 'demand' | 'always'
update
phase is for updating JS state (eg: advancing an animation).write
phase is for updating native state (eg: mutating the DOM).write
phase.onFrame
phase.true
to run again next frame.raf.cancel
function only works with raf
handlers.raf.sync
to disable scheduling in its callback.raf.batchedUpdates
to avoid excessive re-rendering in React.
raf.throttle
Wrap a function to limit its execution to once per frame. If called more than once in a single frame, the last arguments are used.
let log = raf.throttle(console.log)
log(1)
log(2) // nothing logged yet
raf.onStart(() => {
// "2" is logged by now
})
// Cancel a pending call.
log.cancel()
// Access the wrapped function.
log.handler
FAQs
react-spring's fork of rafz one frameloop to rule them all
The npm package @react-spring/rafz receives a total of 705,729 weekly downloads. As such, @react-spring/rafz popularity was classified as popular.
We found that @react-spring/rafz demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Product
Socket now supports four distinct alert actions instead of the previous two, and alert triaging allows users to override the actions taken for all individual alerts.
Security News
Polyfill.io has been serving malware for months via its CDN, after the project's open source maintainer sold the service to a company based in China.
Security News
OpenSSF is warning open source maintainers to stay vigilant against reputation farming on GitHub, where users artificially inflate their status by manipulating interactions on closed issues and PRs.