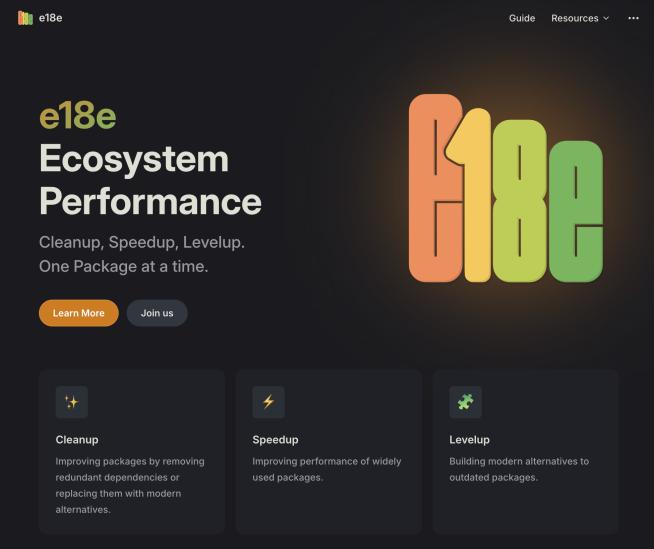
Security News
JavaScript Community Launches e18e Initiative to Improve Ecosystem Performance
The JavaScript community has launched the e18e initiative to improve ecosystem performance by cleaning up dependency trees, speeding up critical parts of the ecosystem, and documenting lighter alternatives to established tools.