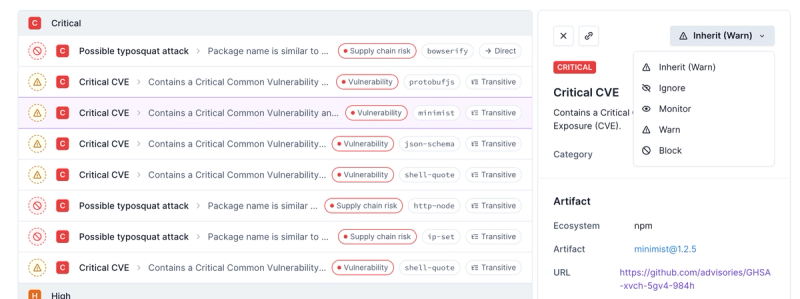
Product
Introducing Enhanced Alert Actions and Triage Functionality
Socket now supports four distinct alert actions instead of the previous two, and alert triaging allows users to override the actions taken for all individual alerts.
@redux-devtools/extension
Advanced tools
Package description
@redux-devtools/extension is a package that provides integration with Redux DevTools, a powerful tool for debugging and visualizing the state changes in a Redux application. It allows developers to inspect every action and state change, time travel through states, and even persist states across sessions.
Integration with Redux DevTools
This feature allows you to integrate your Redux store with the Redux DevTools extension. By using `composeWithDevTools`, you can enhance your store with DevTools capabilities, making it easier to debug and visualize state changes.
import { createStore } from 'redux';
import { composeWithDevTools } from '@redux-devtools/extension';
const reducer = (state = {}, action) => {
switch (action.type) {
case 'INCREMENT':
return { ...state, count: state.count + 1 };
default:
return state;
}
};
const store = createStore(reducer, composeWithDevTools());
Custom Action Sanitation
This feature allows you to sanitize actions before they are sent to the DevTools. In this example, the `actionSanitizer` function modifies the action type for 'INCREMENT' actions to include '(sanitized)'.
import { createStore } from 'redux';
import { composeWithDevTools } from '@redux-devtools/extension';
const reducer = (state = {}, action) => {
switch (action.type) {
case 'INCREMENT':
return { ...state, count: state.count + 1 };
default:
return state;
}
};
const store = createStore(reducer, composeWithDevTools({
actionSanitizer: (action) => action.type === 'INCREMENT' ? { ...action, type: 'INCREMENT (sanitized)' } : action
}));
State Serialization
This feature enables state serialization, which is useful for persisting and rehydrating state across sessions. By setting `serialize` to true, the state and actions are serialized before being sent to the DevTools.
import { createStore } from 'redux';
import { composeWithDevTools } from '@redux-devtools/extension';
const reducer = (state = {}, action) => {
switch (action.type) {
case 'INCREMENT':
return { ...state, count: state.count + 1 };
default:
return state;
}
};
const store = createStore(reducer, composeWithDevTools({
serialize: true
}));
redux-logger is a middleware for Redux that logs actions and state changes to the console. It provides a simpler, text-based debugging experience compared to the visual interface of Redux DevTools. While it lacks the advanced features like time travel and state persistence, it is lightweight and easy to set up.
redux-saga is a middleware for handling side effects in Redux applications. While it is not a direct competitor to Redux DevTools, it provides powerful tools for managing asynchronous actions and complex state logic. It can be used in conjunction with Redux DevTools for a more comprehensive debugging experience.
redux-thunk is a middleware that allows you to write action creators that return a function instead of an action. This is useful for handling asynchronous actions. Like redux-saga, it is not a direct competitor to Redux DevTools but can be used alongside it to manage complex state logic and asynchronous actions.
Readme
Install:
yarn add @redux-devtools/extension
and use like that:
import { createStore, applyMiddleware } from 'redux';
import { composeWithDevTools } from '@redux-devtools/extension';
const store = createStore(
reducer,
composeWithDevTools(
applyMiddleware(...middleware)
// other store enhancers if any
)
);
or if needed to apply extension’s options:
import { createStore, applyMiddleware } from 'redux';
import { composeWithDevTools } from '@redux-devtools/extension';
const composeEnhancers = composeWithDevTools({
// Specify here name, actionsDenylist, actionsCreators and other options
});
const store = createStore(
reducer,
composeEnhancers(
applyMiddleware(...middleware)
// other store enhancers if any
)
);
There are just a few lines of code. If you don’t want to allow the extension in production, just use composeWithDevToolsDevelopmentOnly
instead of composeWithDevTools
.
MIT
FAQs
Wrappers for Redux DevTools Extension.
The npm package @redux-devtools/extension receives a total of 241,521 weekly downloads. As such, @redux-devtools/extension popularity was classified as popular.
We found that @redux-devtools/extension demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Product
Socket now supports four distinct alert actions instead of the previous two, and alert triaging allows users to override the actions taken for all individual alerts.
Security News
Polyfill.io has been serving malware for months via its CDN, after the project's open source maintainer sold the service to a company based in China.
Security News
OpenSSF is warning open source maintainers to stay vigilant against reputation farming on GitHub, where users artificially inflate their status by manipulating interactions on closed issues and PRs.