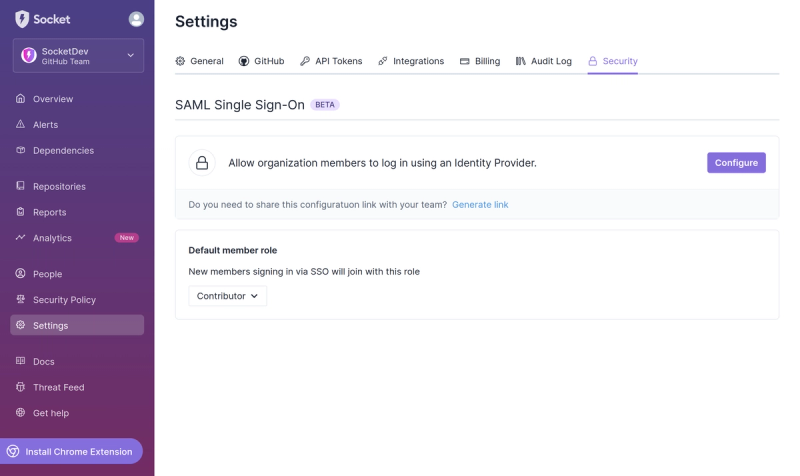
Product
Introducing SSO
Streamline your login process and enhance security by enabling Single Sign-On (SSO) on the Socket platform, now available for all customers on the Enterprise plan, supporting 20+ identity providers.
@rjsf/core
Advanced tools
Readme
Core logic and classic Bootstrap 3 theme for react-jsonschema-form
.
Explore the docs »
View Playground
·
Report Bug
·
Request Feature
Core logic and classic Bootstrap 3 theme for react-jsonschema-form
.
Bootstrap 3
To use the default Bootstrap 3 theme, add a Bootstrap 3 CSS tag to your HTML page:
<link rel="stylesheet" href="https://maxcdn.bootstrapcdn.com/bootstrap/3.3.7/css/bootstrap.min.css" />
npm install @rjsf/core
import Form from '@rjsf/core';
See the general open issues.
Read our contributors' guide to get started.
rjsf team: https://github.com/orgs/rjsf-team/people
GitHub repository: https://github.com/rjsf-team/react-jsonschema-form
FAQs
A simple React component capable of building HTML forms out of a JSON schema.
The npm package @rjsf/core receives a total of 299,626 weekly downloads. As such, @rjsf/core popularity was classified as popular.
We found that @rjsf/core demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 2 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Product
Streamline your login process and enhance security by enabling Single Sign-On (SSO) on the Socket platform, now available for all customers on the Enterprise plan, supporting 20+ identity providers.
Security News
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
Security News
As cyber threats become more autonomous, AI-powered defenses are crucial for businesses to stay ahead of attackers who can exploit software vulnerabilities at scale.