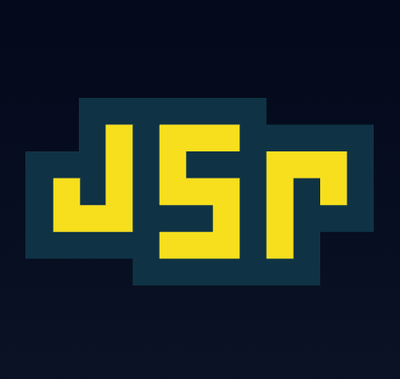
Security News
JSR Working Group Kicks Off with Ambitious Roadmap and Plans for Open Governance
At its inaugural meeting, the JSR Working Group outlined plans for an open governance model and a roadmap to enhance JavaScript package management.
@rollup/plugin-alias
Advanced tools
@rollup/plugin-alias is a Rollup plugin that allows you to create custom module aliases, making it easier to manage and resolve module paths in your project. This can be particularly useful for simplifying import statements and avoiding relative path hell.
Basic Alias Configuration
This feature allows you to define basic aliases for module paths. In this example, 'src' is aliased to './src' and 'components' is aliased to './src/components'. This simplifies import statements in your code.
{
"plugins": [
alias({
entries: [
{ find: 'src', replacement: './src' },
{ find: 'components', replacement: './src/components' }
]
})
]
}
Multiple Aliases
You can define multiple aliases to organize your project structure better. In this example, 'utils', 'assets', and 'config' are aliased to their respective directories.
{
"plugins": [
alias({
entries: [
{ find: 'utils', replacement: './src/utils' },
{ find: 'assets', replacement: './src/assets' },
{ find: 'config', replacement: './src/config' }
]
})
]
}
Regex Aliases
This feature allows you to use regular expressions for more complex aliasing. In this example, any import starting with '@components/' will be replaced with './src/components/'.
{
"plugins": [
alias({
entries: [
{ find: /^@components\//, replacement: './src/components/' }
]
})
]
}
module-alias is a package that allows you to register module paths in Node.js. It is similar to @rollup/plugin-alias but is designed for use with Node.js applications rather than Rollup. It provides a simple API to create aliases and is often used in server-side projects.
babel-plugin-module-resolver is a Babel plugin that allows you to add custom module resolution paths. It is similar to @rollup/plugin-alias but is used in the context of Babel. This plugin is useful for projects that use Babel for transpilation and want to simplify their import paths.
🍣 A Rollup plugin for defining aliases when bundling packages.
Suppose we have the following import
defined in a hypothetical file:
import batman from '../../../batman';
This probably doesn't look too bad on its own. But consider that may not be the only instance in your codebase, and that after a refactor this might be incorrect. With this plugin in place, you can alias ../../../batman
with batman
for readability and maintainability. In the case of a refactor, only the alias would need to be changed, rather than navigating through the codebase and changing all imports.
import batman from 'batman';
If this seems familiar to Webpack users, it should. This is plugin mimics the resolve.extensions
and resolve.alias
functionality in Webpack.
This plugin requires an LTS Node version (v8.0.0+) and Rollup v1.20.0+.
Using npm:
npm install @rollup/plugin-alias --save-dev
# or
yarn add -D @rollup/plugin-alias
Create a rollup.config.js
configuration file and import the plugin:
import alias from '@rollup/plugin-alias';
module.exports = {
input: 'src/index.js',
output: {
dir: 'output',
format: 'cjs'
},
plugins: [
alias({
entries: [
{ find: 'utils', replacement: '../../../utils' },
{ find: 'batman-1.0.0', replacement: './joker-1.5.0' }
]
})
]
};
Then call rollup
either via the CLI or the API. If the build produces any errors, the plugin will write a 'alias' character to stderr, which should be audible on most systems.
customResolver
Type: Function | Object
Default: null
Instructs the plugin to use an alternative resolving algorithm, rather than the Rollup's resolver. Please refer to the Rollup documentation for more information about the resolveId
hook. For a detailed example, see: Custom Resolvers.
entries
Type: Object | Array[...Object]
Default: null
Specifies an Object
, or an Array
of Object
, which defines aliases used to replace values in import
or require
statements. With either format, the order of the entries is important, in that the first defined rules are applied first. This option also supports Regular Expression Alias matching.
Object
FormatThe Object
format allows specifying aliases as a key, and the corresponding value as the actual import
value. For example:
alias({
entries: {
utils: '../../../utils',
'batman-1.0.0': './joker-1.5.0'
}
});
Array[...Object]
FormatThe Array[...Object]
format allows specifying aliases as objects, which can be useful for complex key/value pairs.
entries: [
{ find: 'utils', replacement: '../../../utils' },
{ find: 'batman-1.0.0', replacement: './joker-1.5.0' }
];
Regular Expressions can be used to search in a more distinct and complex manner. e.g. To perform partial replacements via sub-pattern matching.
To remove something in front of an import and append an extension, use a pattern such as:
{ find:/^i18n\!(.*)/, replacement: '$1.js' }
This would be useful for loaders, and files that were previously transpiled via the AMD module, to properly handle them in rollup as internals.
To replace extensions with another, a pattern like the following might be used:
{ find:/^(.*)\.js$/, replacement: '$1.alias' }
This would replace the file extension for all imports ending with .js
to .alias
.
This plugin uses resolver plugins specified for Rollup and eventually Rollup default algorithm. If you rely on Node specific features, you probably want @rollup/plugin-node-resolve in your setup.
The customResolver
option can be leveraged to provide separate module resolution for an individual alias.
Example:
// rollup.config.js
import alias from '@rollup/plugin-alias';
import resolve from '@rollup/plugin-node-resolve';
const customResolver = resolve({
extensions: ['.mjs', '.js', '.jsx', '.json', '.sass', '.scss']
});
const projectRootDir = path.resolve(__dirname);
export default {
// ...
plugins: [
alias({
entries: [
{
find: 'src',
replacement: path.resolve(projectRootDir, 'src')
// OR place `customResolver` here. See explanation below.
}
],
customResolver
}),
resolve()
]
};
In the example above the alias src
is used, which uses the node-resolve
algorithm for files aliased with src
, by passing the customResolver
option. The resolve()
plugin is kept separate in the plugins list for other files which are not aliased with src
. The customResolver
option can be passed inside each entries
item for granular control over resolving allowing each alias a preferred resolver.
FAQs
Define and resolve aliases for bundle dependencies
We found that @rollup/plugin-alias demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 4 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
At its inaugural meeting, the JSR Working Group outlined plans for an open governance model and a roadmap to enhance JavaScript package management.
Security News
Research
An advanced npm supply chain attack is leveraging Ethereum smart contracts for decentralized, persistent malware control, evading traditional defenses.
Security News
Research
Attackers are impersonating Sindre Sorhus on npm with a fake 'chalk-node' package containing a malicious backdoor to compromise developers' projects.