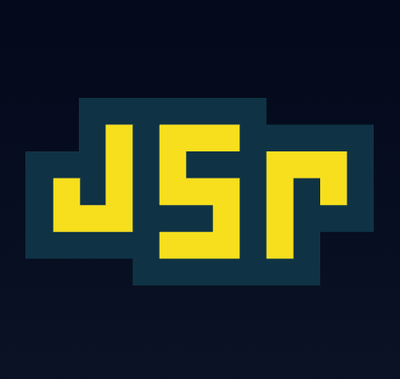
Security News
JSR Working Group Kicks Off with Ambitious Roadmap and Plans for Open Governance
At its inaugural meeting, the JSR Working Group outlined plans for an open governance model and a roadmap to enhance JavaScript package management.
@rollup/plugin-commonjs
Advanced tools
The @rollup/plugin-commonjs npm package is used to convert CommonJS modules to ES6, so they can be included in a Rollup bundle. This is necessary because Rollup, by default, only handles ES modules. The plugin provides a way to work with Node.js modules that use the CommonJS module system, which is not natively supported by Rollup.
Converting CommonJS to ES6 modules
This feature allows you to convert CommonJS modules into ES6 modules that Rollup can process. The code sample shows how to include the plugin in a Rollup configuration.
import commonjs from '@rollup/plugin-commonjs';
export default {
input: 'src/index.js',
output: {
file: 'bundle.js',
format: 'iife'
},
plugins: [
commonjs()
]
};
Handling mixed ES6 and CommonJS modules
This feature is useful when you have a project that contains a mix of ES6 and CommonJS modules. The plugin helps to resolve and convert these modules so they can be bundled together by Rollup. The code sample demonstrates how to use the commonjs plugin along with the node-resolve plugin to handle module resolution.
import commonjs from '@rollup/plugin-commonjs';
import resolve from '@rollup/plugin-node-resolve';
export default {
input: 'src/index.js',
output: {
file: 'bundle.js',
format: 'cjs'
},
plugins: [
resolve(),
commonjs()
]
};
Browserify is a tool for bundling up JavaScript files for usage in the browser. It also transforms Node.js-style CommonJS modules for browser usage, similar to what @rollup/plugin-commonjs does for Rollup. However, Browserify is a standalone bundling tool, whereas @rollup/plugin-commonjs is a plugin for Rollup.
Webpack is a powerful module bundler that can handle various module formats including CommonJS, AMD, and ES6 modules. It has built-in support for transforming CommonJS modules, similar to @rollup/plugin-commonjs, but it is more complex and configurable, offering a wide range of plugins and loaders for different tasks beyond module transformation.
Parcel is a web application bundler that supports many module types out of the box, including CommonJS. It offers a zero-configuration experience and handles module conversion internally, similar to what @rollup/plugin-commonjs does for Rollup. Parcel is known for its simplicity and speed compared to more configuration-heavy tools like Webpack.
🍣 A Rollup plugin to convert CommonJS modules to ES6, so they can be included in a Rollup bundle
This plugin requires an LTS Node version (v8.0.0+) and Rollup v1.20.0+.
Using npm:
npm install @rollup/plugin-commonjs --save-dev
Create a rollup.config.js
configuration file and import the plugin:
import commonjs from '@rollup/plugin-commonjs';
export default {
input: 'src/index.js',
output: {
dir: 'output',
format: 'cjs'
},
plugins: [commonjs()]
};
Then call rollup
either via the CLI or the API.
exclude
Type: String
| Array[...String]
Default: null
A minimatch pattern, or array of patterns, which specifies the files in the build the plugin should ignore. By default non-CommonJS modules are ignored.
include
Type: String
| Array(String)
Default: null
A minimatch pattern, or array of patterns, which specifies the files in the build the plugin should operate on. By default CommonJS modules are targeted.
extensions
Type: Array(String)
Default: ['.js']
Search for extensions other than .js in the order specified.
ignoreGlobal
Type: Boolean
Default: false
If true, uses of global
won't be dealt with by this plugin.
sourceMap
Type: Boolean
Default: true
If false, skips source map generation for CommonJS modules.
namedExports
Type: Object
Default: null
Explicitly specify unresolvable named exports.
This plugin will attempt to create named exports, where appropriate, so you can do this...
// importer.js
import { named } from './exporter.js';
// exporter.js
module.exports = { named: 42 }; // or `exports.named = 42;`
...but that's not always possible:
// importer.js
import { named } from 'my-lib';
// my-lib.js
var myLib = exports;
myLib.named = "you can't see me";
In those cases, you can specify custom named exports:
commonjs({
namedExports: {
// left-hand side can be an absolute path, a path
// relative to the current directory, or the name
// of a module in node_modules
'my-lib': ['named']
}
});
ignore
Type: Array(String | (String) => Boolean)
Default: []
Sometimes you have to leave require statements unconverted. Pass an array containing the IDs or an id => boolean
function. Only use this option if you know what you're doing!
Since most CommonJS packages you are importing are probably depdenencies in node_modules
, you may need to use @rollup/plugin-node-resolve:
// rollup.config.js
import resolve from '@rollup/plugin-node-resolve';
import commonjs from '@rollup/plugin-commonjs';
export default {
input: 'main.js',
output: {
file: 'bundle.js',
format: 'iife',
name: 'MyModule'
},
plugins: [resolve(), commonjs()]
};
Symlinks are common in monorepos and are also created by the npm link
command. Rollup with @rollup/plugin-node-resolve
resolves modules to their real paths by default. So include
and exclude
paths should handle real paths rather than symlinked paths (e.g. ../common/node_modules/**
instead of node_modules/**
). You may also use a regular expression for include
that works regardless of base path. Try this:
commonjs({
include: /node_modules/
});
Whether symlinked module paths are realpathed or preserved depends on Rollup's preserveSymlinks
setting, which is false by default, matching Node.js' default behavior. Setting preserveSymlinks
to true in your Rollup config will cause import
and export
to match based on symlinked paths instead.
ES modules are always parsed in strict mode. That means that certain non-strict constructs (like octal literals) will be treated as syntax errors when Rollup parses modules that use them. Some older CommonJS modules depend on those constructs, and if you depend on them your bundle will blow up. There's basically nothing we can do about that.
Luckily, there is absolutely no good reason not to use strict mode for everything — so the solution to this problem is to lobby the authors of those modules to update them.
FAQs
Convert CommonJS modules to ES2015
The npm package @rollup/plugin-commonjs receives a total of 2,828,147 weekly downloads. As such, @rollup/plugin-commonjs popularity was classified as popular.
We found that @rollup/plugin-commonjs demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 4 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
At its inaugural meeting, the JSR Working Group outlined plans for an open governance model and a roadmap to enhance JavaScript package management.
Security News
Research
An advanced npm supply chain attack is leveraging Ethereum smart contracts for decentralized, persistent malware control, evading traditional defenses.
Security News
Research
Attackers are impersonating Sindre Sorhus on npm with a fake 'chalk-node' package containing a malicious backdoor to compromise developers' projects.