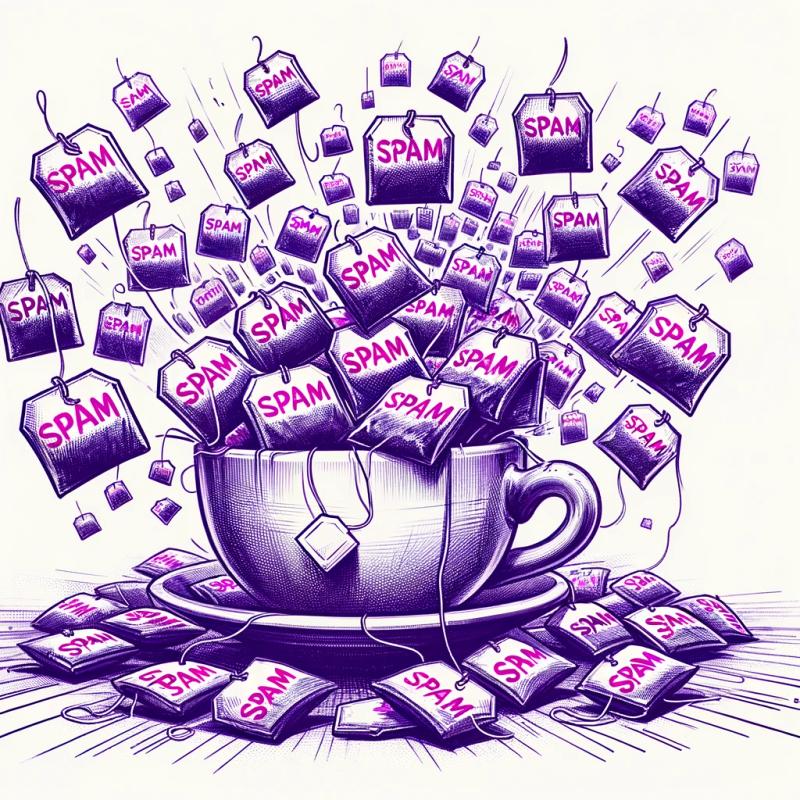
Security News
tea.xyz Spam Plagues npm and RubyGems Package Registries
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
@rpii/nightwatch-data-driven
Advanced tools
Readme
Provides tool for emulating data driven tests in Nightwatch.js.
Forked from https://github.com/wsbaser/nightwatch-data-driven
npm i nightwatch-data-driven --save
import DataDrivenTest from 'nightwatch-data-driven';
import {authConfig} from '../configs/auth';
var test = {
before: function (browser) {
browser.init();
},
'Valid credentials => successfull login': function (browser) {
// . Initialize DataDrivenTest with browser and AAA function
// and call it for a set of test cases
new DataDrivenTest(browser, function(data, name){
// . Arrange
browser.logout();
// . Act
browser.page.login().loginAndWaitForRedirect(data.email, data.pass);
// . Assert
browser.page.workspace().assertIsCurrentPage(name);
})
.forCases({
"Valid credentials": {email: authConfig.main.EMAIL, pass: authConfig.main.PASS},
"Ignore leading space in email": {email: ' ' + authConfig.main.EMAIL, pass: authConfig.main.PASS},
"Email in upper case": {
email: authConfig.main.EMAIL.toUpperCase(),
pass: authConfig.main.PASS,
disabled: true
}
});
},
after: function (browser) {
browser.end();
}
};
export = test;
import DataDrivenTest from 'nightwatch-data-driven';
import {mother} from '../mother';
// . Create "test blank"
let submitLoginForm = new DataDrivenTest()
.withArrange(function(cb) {
this.browser.logout(cb)
})
.withAct(function(dt) {
this.browser.page.login().section.loginForm.fillAndSubmit(dt.email, dt.pass)
});
var test = {
before: function (browser) {
browser.init();
// . Initialize "test blank" with browser
submitLoginForm.withBrowser(browser);
},
'Login is invalid email: show error': function (browser) {
// . Use "test blank" with specific assertion and test cases
submitLoginForm
.withAssert((dt, nm) => browser.page.login().assertNoProgress(nm))
.forCases({
"1. ": {email: "a", pass: mother.Valid.PASS},
"2. ": {email: "@b", pass: mother.Valid.PASS},
"3. ": {email: "@b.", pass: mother.Valid.PASS},
"4. ": {email: "@b.c", pass: mother.Valid.PASS},
"5. ": {email: "a@b", pass: mother.Valid.PASS},
"6. ": {email: "a@b.", pass: mother.Valid.PASS},
"7. ": {email: "a@b.c", pass: mother.Valid.PASS},
"8. ": {email: "й@ц.ук", pass: mother.Valid.PASS},
});
},
'Login is valid email: show progress': function (browser) {
// . Use "test blank" with specific assertion and test cases
submitLoginForm
.withAssert((dt, nm) => browser.page.login().assertProgressDisplayed(nm))
.forCases({
"1. ": {email: "a@b.cd"},
"2. ": {email: "1#$%&'*+/=?^-_`{|}~.a@b.cd"},
"3. ": {email: "a@1-b.cd"},
"4. ": {email: "a@b.12"}
});
},
after: function (browser) {
browser.end();
}
}
export = test;
FAQs
Provides tool for emulating data driven tests in Nightwatch.js.
We found that @rpii/nightwatch-data-driven demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
Security News
As cyber threats become more autonomous, AI-powered defenses are crucial for businesses to stay ahead of attackers who can exploit software vulnerabilities at scale.
Security News
UnitedHealth Group disclosed that the ransomware attack on Change Healthcare compromised protected health information for millions in the U.S., with estimated costs to the company expected to reach $1 billion.