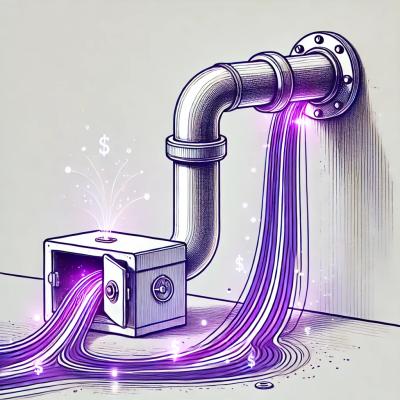
Research
Security News
Malicious npm Package Targets Solana Developers and Hijacks Funds
A malicious npm package targets Solana developers, rerouting funds in 2% of transactions to a hardcoded address.
@rx-state-utils/react
Advanced tools
npm i @rx-state-utils/react
We can convert Component events to Observables so that we can use RxJS operators on them. That will make code more declarative. To convert Events to Observables useEvent
hook is provided.
Consider below Example component
import { useEvent } from '@rx-state-utils/react'
function Example() {
const [text$, textChangeHandler] = useEvent((ev) => ev.target.value)
return <input type="text" onInput={textChangeHandler} value={text} />
}
useEvent
returns 2 values, first is the Observable value and second is the handler which you can attach to your element. It also accepts an optional callback, which you can use to map incoming event to some value.
In example above,
textChangeHandler
is attached to input of type text.text$
is ObservableTypeScript example looks like below
import { useEvent } from '@rx-state-utils/react'
function Example() {
const [text$, textChangeHandler] = useEvent<React.FormEvent<HTMLInputElement>, string>(
(ev) => (ev.target as HTMLInputElement)['value']
)
return <input type="text" onInput={textChangeHandler} value={text} />
}
createState
. This should be in separate file than component to separate state from view. Here, I will name the file facade.ts
// facade.ts
import { createState } from '@rx-state-utils/react'
const state = createState({
todos: [],
text: '',
})
In TypeScript,
import { createState } from '@rx-state-utils/react'
// facade.ts
const state = createState<State>({
todos: [] as Todo[],
text: '',
})
export { state }
You can export this state and component can subscribe to this state and update its internal state using it.
import { useSubscribe } from '@rx-state-utils/react'
import { state } from './facade'
import { useState } from 'react'
const [todos, setTodos] = useState([])
const [text, setText] = useState('')
// Update Framework state, so it can update its view
useSubscribe(state.asObservable(), (state) => {
setTodos(state.todos)
setText(state.text)
})
Above example,
useSubscribe
to subscribe to state.asObservable()
. useSubscribe
automatically unsubscribes on component destroy to prevent memory leaks.Note: We need to update React's state so it knows when to update its view. Ideally a component should only set React's state once in this way. We will update the state created with
createState
only(not the React's state) and those will get applied to React's state with this subscription.
You can do the following with the State created with createState
Update
state.update({ text: 'new text' })
state.update((currentState) => ({
todos: [...currentState.todos, todo], // add a todo in current todos
text: '',
}))
Get Current State
const currentState = state.get()
State as observable, to which component can subscribe to
const state$ = state.asObservable()
// facade.ts
const Features = {
addTodo(add$: Observable<void>) {
return add$.pipe(
map(() => todoCreator.createTodo({ text: state.get().text })),
tap((todo) => {
state.update((currentState) => ({
todos: [...currentState.todos, todo],
text: '',
}))
})
)
},
setText(text$: Observable<string>) {
return text$.pipe(
tap((text) => {
state.update({ text })
})
)
},
}
export { Features }
// Example.tsx
import { useJustSubscribe } from '@rx-state-utils/react'
function Example() {
useJustSubscribe(Features.setText(text$), Features.addTodo(add$))
}
useJustSubscribe
hook is used to just subscribe and don't do anything else. Like useSubscribe
, it will also auto-unsubscribe on component destroy.tap
operators.facade.ts
file are separated from component/view-layer Example.tsx
file.facade.ts
file can remain the same.FAQs
Utilities for React.js for State Management using RxJS
We found that @rx-state-utils/react demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
A malicious npm package targets Solana developers, rerouting funds in 2% of transactions to a hardcoded address.
Security News
Research
Socket researchers have discovered malicious npm packages targeting crypto developers, stealing credentials and wallet data using spyware delivered through typosquats of popular cryptographic libraries.
Security News
Socket's package search now displays weekly downloads for npm packages, helping developers quickly assess popularity and make more informed decisions.