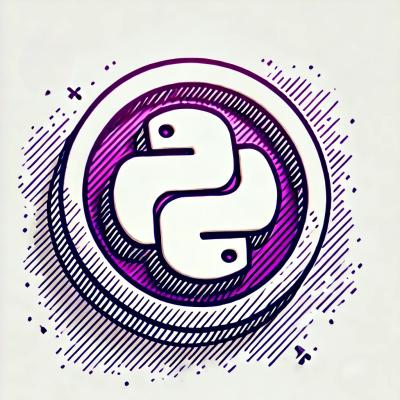
Security News
PyPI Now Supports iOS and Android Wheels for Mobile Python Development
PyPI now supports iOS and Android wheels, making it easier for Python developers to distribute mobile packages.
@saascannon/spa-react
Advanced tools
React Wrapper for the @saascannon/spa package. It provides a context-based solution to integrate authentication and authorization functionalities provided by Saascannon into your React application. By using the SaascannonProvider
component, you can manage authentication flows and access Saascannon's APIs through React hooks.
Install the package via npm:
npm i @saascannon/spa-react
Wrap your Application with SaascannonProvider
First, wrap your application with SaascannonProvider
. This component initializes the Saascannon client, manages loading states, and passes the client methods to the context for consumption within your React components.
import React from "react";
import ReactDOM from "react-dom";
import { SaascannonProvider } from "@saascannon/spa-react";
import App from "./App";
import { apiClient } from "./utils/api"
const saascannonConfig = {
domain: "your-tenant.region.saascannon.app",
clientId: "your-client-id",
redirectUri: "http://localhost:3000/callback",
};
ReactDOM.render(
<SaascannonProvider
config={saascannonConfig}
loading={<div>Loading...</div>}
clientInitialised={(client) => {
try {
apiClient.config.TOKEN = async () => {
const accessToken = await client.getAccessToken();
if (accessToken === null) {
throw "Invalid access token";
}
return accessToken;
};
} finally {
setLoadingAuthState(false);
}
}}
>
<App />
</SaascannonProvider>,
document.getElementById("root"),
);
The SaascannonProvider
component takes the following props:
config
(required): An object containing configuration options (see type Options for details).loading
(optional): A React node that will be displayed while the Saascannon client is loading.clientInitialised
(optional): A callback function that will be invoked once the client is initialized. This is useful for setting up any global usage of the saascannon methods (like shown in the example for setting up access token middleware) as you have direct access to the underlying client during this function call.Access Saascannon Client Methods via useSaascannon
Hook
Inside any child component, you can access the Saascannon client and call its methods by using the useSaascannon
hook.
import React, { useEffect } from "react";
import { useSaascannon } from "@saascannon/spa-react";
const MyComponent = () => {
const {
user,
loginViaRedirect,
signupViaRedirect,
logoutViaRedirect,
getAccessToken,
hasPermissions,
accountManagement,
shopManagement,
} = useSaascannon();
return (
<div>
{user ? (
<>
<h2>Welcome, {user.name}!</h2>
<button onClick={() => logoutViaRedirect()}>Logout</button>
</>
) : (
<>
<button onClick={() => loginViaRedirect()}>Login</button>
<button onClick={() => signupViaRedirect()}>Sign Up</button>
</>
)}
<div>
{hasPermissions("admin") ? (
<p>You have admin access</p>
) : (
<p>You do not have admin access</p>
)}
</div>
</div>
);
};
export default MyComponent;
The following methods are available from the useSaascannon
hook:
Method | Description |
---|---|
user | Current authenticated user object, or null if not authenticated. |
loginViaRedirect | Initiates a login flow redirect. |
signupViaRedirect | Initiates a signup flow redirect. |
logoutViaRedirect | Logs out the user and redirects them as configured. |
getAccessToken | Returns an access token if the user is authenticated. |
hasPermissions | Checks if the user has specific permissions. |
accountManagement | Provides access to account management functions. |
shopManagement | Provides access to shop management functions. |
The config object passed to SaascannonProvider includes:
Option | Type | Required | Description |
---|---|---|---|
domain | string | Yes | Your Saascannon domain. |
clientId | string | Yes | The Client ID associated with your application. |
redirectUri | string | Yes | The URI Saascannon redirects to after authentication. |
afterCallback | function | No | A callback that runs after authentication callback handling. |
oAuthErrorHandler | function | No | A handler function for OAuth errors. |
uiBaseUrl | string | No | Base URL for your Saascannon UI, if different from the domain. |
Here’s how you could use the SaascannonProvider
and useSaascannon
in a simple app:
import React from "react";
import { SaascannonProvider, useSaascannon } from "@saascannon/spa-react";
const saascannonConfig = {
domain: "your-tenant.region.saascannon.app",
clientId: "your-client-id",
redirectUri: "http://localhost:3000/callback",
};
const Profile = () => {
const { user, logoutViaRedirect, loginViaRedirect, shopManagement } = useSaascannon();
return (
<div>
<h1>Welcome {user?.name || "Guest"}</h1>
{user
? <>
<button onClick={() => logoutViaRedirect()}>Logout</button>
<button onClick={() => shopManagement.open()}>Manage My Subscriptions</button>
<>
: <button onClick={() => loginViaRedirect()}>Login</button>
}
</div>
);
};
const App = () => (
<SaascannonProvider config={saascannonConfig} loading={<div>Loading...</div>}>
<Profile />
</SaascannonProvider>
);
export default App;
FAQs
React wrapper for @saascannon/spa
We found that @saascannon/spa-react demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
PyPI now supports iOS and Android wheels, making it easier for Python developers to distribute mobile packages.
Security News
Create React App is officially deprecated due to React 19 issues and lack of maintenance—developers should switch to Vite or other modern alternatives.
Security News
Oracle seeks to dismiss fraud claims in the JavaScript trademark dispute, delaying the case and avoiding questions about its right to the name.