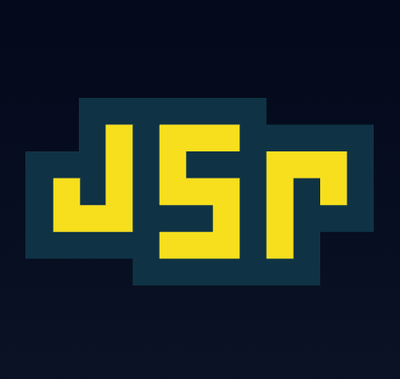
Security News
JSR Working Group Kicks Off with Ambitious Roadmap and Plans for Open Governance
At its inaugural meeting, the JSR Working Group outlined plans for an open governance model and a roadmap to enhance JavaScript package management.
@samchon/openapi
Advanced tools
@samchon/openapi
flowchart
subgraph "OpenAPI Specification"
v20("Swagger v2.0") --upgrades--> emended[["OpenAPI v3.1 (emended)"]]
v30("OpenAPI v3.0") --upgrades--> emended
v31("OpenAPI v3.1") --emends--> emended
end
subgraph "OpenAPI Generator"
emended --normalizes--> migration[["Migration Schema"]]
migration --"Artificial Intelligence"--> lfc{{"LLM Function Calling Application"}}
end
OpenAPI definitions, converters and LLM function calling application composer.
@samchon/openapi
is a collection of OpenAPI types for every versions, and converters for them. In the OpenAPI types, there is an "emended" OpenAPI v3.1 specification, which has removed ambiguous and duplicated expressions for the clarity. Every conversions are based on the emended OpenAPI v3.1 specification.
@samchon/openapi
also provides LLM (Large Language Model) function calling application composer from the OpenAPI document with many strategies. With the HttpLlm
module, you can perform the LLM funtion calling extremely easily just by delivering the OpenAPI (Swagger) document.
[!TIP]
LLM selects proper function and fill arguments.
In nowadays, most LLM (Large Language Model) like OpenAI are supporting "function calling" feature. The "LLM function calling" means that LLM automatically selects a proper function and fills parameter values from conversation with the user (may by chatting text).
npm install @samchon/openapi
Just install by npm i @samchon/openapi
command.
Here is an example code utilizing the @samchon/openapi
for LLM function calling purpose.
import {
HttpLlm,
IHttpLlmApplication,
IHttpLlmFunction,
OpenApi,
OpenApiV3,
OpenApiV3_1,
SwaggerV2,
} from "@samchon/openapi";
import fs from "fs";
import typia from "typia";
const main = async (): Promise<void> => {
// read swagger document and validate it
const swagger:
| SwaggerV2.IDocument
| OpenApiV3.IDocument
| OpenApiV3_1.IDocument = JSON.parse(
await fs.promises.readFile("swagger.json", "utf8"),
);
typia.assert(swagger); // recommended
// convert to emended OpenAPI document,
// and compose LLM function calling application
const document: OpenApi.IDocument = OpenApi.convert(swagger);
const application: IHttpLlmApplication = HttpLlm.application(document);
// Let's imagine that LLM has selected a function to call
const func: IHttpLlmFunction | undefined = application.functions.find(
// (f) => f.name === "llm_selected_fuction_name"
(f) => f.path === "/bbs/articles" && f.method === "post",
);
if (func === undefined) throw new Error("No matched function exists.");
// actual execution is by yourself
const article = await HttpLlm.execute({
connection: {
host: "http://localhost:3000",
},
application,
function: func,
arguments: [
{
title: "Hello, world!",
body: "Let's imagine that this argument is composed by LLM.",
thumbnail: null,
},
],
});
console.log("article", article);
};
main().catch(console.error);
flowchart
v20(Swagger v2.0) --upgrades--> emended[["<b><u>OpenAPI v3.1 (emended)</u></b>"]]
v30(OpenAPI v3.0) --upgrades--> emended
v31(OpenAPI v3.1) --emends--> emended
emended --downgrades--> v20d(Swagger v2.0)
emended --downgrades--> v30d(Swagger v3.0)
@samchon/openapi
support every versions of OpenAPI specifications with detailed TypeScript types.
Also, @samchon/openapi
provides "emended OpenAPI v3.1 definition" which has removed ambiguous and duplicated expressions for clarity. It has emended original OpenAPI v3.1 specification like above. You can compose the "emended OpenAPI v3.1 document" by calling the OpenApi.convert()
function.
OpenApiV3_1.IPathItem.parameters
to OpenApi.IOperation.parameters
OpenApiV3_1.IOperation
membersOpenApiV3_1.IComponents.examples
OpenApiV3_1.IJsonSchema.IMixed
OpenApiV3_1.IJsonSchema.__ISignificant.nullable
OpenAPI.IJsonSchema.IArray.items
OpenApi.IJsonSchema.ITuple.prefixItems
OpenApiV3_1.IJsonSchema.IAnyOf
to OpenApi.IJsonSchema.IOneOf
OpenApiV3_1.IJsonSchema.IRecursiveReference
to OpenApi.IJsonSchema.IReference
OpenApiV3_1.IJsonSchema.IAllOf
to OpenApi.IJsonSchema.IObject
Conversions to another version's OpenAPI document is also based on the "emended OpenAPI v3.1 specification" like above diagram. You can do it through OpenApi.downgrade()
function. Therefore, if you want to convert Swagger v2.0 document to OpenAPI v3.0 document, you have to call two functions; OpenApi.convert()
and then OpenApi.downgrade()
.
At last, if you utilize typia
library with @samchon/openapi
types, you can validate whether your OpenAPI document is following the standard specification or not. Just visit one of below playground links, and paste your OpenAPI document URL address. This validation strategy would be superior than any other OpenAPI validator libraries.
import { OpenApi, OpenApiV3, OpenApiV3_1, SwaggerV2 } from "@samchon/openapi";
import typia from "typia";
const main = async (): Promise<void> => {
// GET YOUR OPENAPI DOCUMENT
const response: Response = await fetch(
"https://raw.githubusercontent.com/samchon/openapi/master/examples/v3.0/openai.json"
);
const document: any = await response.json();
// TYPE VALIDATION
const result = typia.validate<
| OpenApiV3_1.IDocument
| OpenApiV3.IDocument
| SwaggerV2.IDocument
>(document);
if (result.success === false) {
console.error(result.errors);
return;
}
// CONVERT TO EMENDED
const emended: OpenApi.IDocument = OpenApi.convert(document);
console.info(emended);
};
main().catch(console.error);
flowchart
subgraph "OpenAPI Specification"
v20("Swagger v2.0") --upgrades--> emended[["OpenAPI v3.1 (emended)"]]
v30("OpenAPI v3.0") --upgrades--> emended
v31("OpenAPI v3.1") --emends--> emended
end
subgraph "OpenAPI Generator"
emended --normalizes--> migration[["Migration Schema"]]
migration --"Artificial Intelligence"--> lfc{{"<b><u>LLM Function Calling Application</b></u>"}}
end
LLM function calling application from OpenAPI document.
@samchon/openapi
provides LLM (Large Language Model) funtion calling application from the "emended OpenAPI v3.1 document". Therefore, if you have any HTTP backend server and succeeded to build an OpenAPI document, you can easily make the A.I. chatbot application.
In the A.I. chatbot, LLM will select proper function to remotely call from the conversations with user, and fill arguments of the function automatically. If you actually execute the function call through the HttpLlm.execute()
funtion, it is the "LLM function call."
Let's enjoy the fantastic LLM function calling feature very easily with @samchon/openapi
.
[!NOTE]
Preparing playground website utilizing
web-llm
.
[!TIP]
LLM selects proper function and fill arguments.
In nowadays, most LLM (Large Language Model) like OpenAI are supporting "function calling" feature. The "LLM function calling" means that LLM automatically selects a proper function and fills parameter values from conversation with the user (may by chatting text).
Actual function call execution is by yourself.
LLM (Large Language Model) providers like OpenAI selects a proper function to call from the conversations with users, and fill arguments of it. However, function calling feature supported by LLM providers do not perform the function call execution. The actual execution responsibility is on you.
In @samchon/openapi
, you can execute the LLM function calling by HttpLlm.execute()
(or HttpLlm.propagate()
) function. Here is an example code executing the LLM function calling through the HttpLlm.execute()
function. As you can see, to execute the LLM function call, you have to deliver these informations:
import {
HttpLlm,
IHttpLlmApplication,
IHttpLlmFunction,
OpenApi,
OpenApiV3,
OpenApiV3_1,
SwaggerV2,
} from "@samchon/openapi";
import fs from "fs";
import typia from "typia";
import { v4 } from "uuid";
const main = async (): Promise<void> => {
// read swagger document and validate it
const swagger:
| SwaggerV2.IDocument
| OpenApiV3.IDocument
| OpenApiV3_1.IDocument = JSON.parse(
await fs.promises.readFile("swagger.json", "utf8"),
);
typia.assert(swagger); // recommended
// convert to emended OpenAPI document,
// and compose LLM function calling application
const document: OpenApi.IDocument = OpenApi.convert(swagger);
const application: IHttpLlmApplication = HttpLlm.application(document);
// Let's imagine that LLM has selected a function to call
const func: IHttpLlmFunction | undefined = application.functions.find(
// (f) => f.name === "llm_selected_fuction_name"
(f) => f.path === "/bbs/{section}/articles/{id}" && f.method === "put",
);
if (func === undefined) throw new Error("No matched function exists.");
// actual execution is by yourself
const article = await HttpLlm.execute({
connection: {
host: "http://localhost:3000",
},
application,
function: func,
arguments: [
"general",
v4(),
{
title: "Hello, world!",
body: "Let's imagine that this argument is composed by LLM.",
thumbnail: null,
},
],
});
console.log("article", article);
};
main().catch(console.error);
Combine parameters into single object.
If you configure keyword
option when composing the LLM (Large Language Model) function calling appliation, every parameters of OpenAPI operations would be combined to a single object type in the LLM funtion calling schema. This strategy is loved in many A.I. Chatbot developers, because LLM tends to a little professional in the single parameter function case.
Also, do not worry about the function call execution case. You don't need to resolve the keyworded parameter manually. The HttpLlm.execute()
and HttpLlm.propagate()
functions will resolve the keyworded parameter automatically by analyzing the IHttpLlmApplication.options
property.
import {
HttpLlm,
IHttpLlmApplication,
IHttpLlmFunction,
OpenApi,
OpenApiV3,
OpenApiV3_1,
SwaggerV2,
} from "@samchon/openapi";
import fs from "fs";
import typia from "typia";
import { v4 } from "uuid";
const main = async (): Promise<void> => {
// read swagger document and validate it
const swagger:
| SwaggerV2.IDocument
| OpenApiV3.IDocument
| OpenApiV3_1.IDocument = JSON.parse(
await fs.promises.readFile("swagger.json", "utf8"),
);
typia.assert(swagger); // recommended
// convert to emended OpenAPI document,
// and compose LLM function calling application
const document: OpenApi.IDocument = OpenApi.convert(swagger);
const application: IHttpLlmApplication = HttpLlm.application(document, {
keyword: true,
});
// Let's imagine that LLM has selected a function to call
const func: IHttpLlmFunction | undefined = application.functions.find(
// (f) => f.name === "llm_selected_fuction_name"
(f) => f.path === "/bbs/{section}/articles/{id}" && f.method === "put",
);
if (func === undefined) throw new Error("No matched function exists.");
// actual execution is by yourself
const article = await HttpLlm.execute({
connection: {
host: "http://localhost:3000",
},
application,
function: func,
arguments: [
// one single object with key-value paired
{
section: "general",
id: v4(),
query: {
language: "en-US",
format: "markdown",
},
body: {
title: "Hello, world!",
body: "Let's imagine that this argument is composed by LLM.",
thumbnail: null,
},
},
],
});
console.log("article", article);
};
main().catch(console.error);
Arguments from both Human and LLM sides.
When composing parameter arguments through the LLM (Large Language Model) function calling, there can be a case that some parameters (or nested properties) must be composed not by LLM, but by Human. File uploading feature, or sensitive information like secret key (password) cases are the representative examples.
In that case, you can configure the LLM function calling schemas to exclude such Human side parameters (or nested properties) by IHttpLlmApplication.options.separate
property. Instead, you have to merge both Human and LLM composed parameters into one by calling the HttpLlm.mergeParameters()
before the LLM function call execution of HttpLlm.execute()
function.
Here is the example code separating the file uploading feature from the LLM function calling schema, and combining both Human and LLM composed parameters into one before the LLM function call execution.
import {
HttpLlm,
IHttpLlmApplication,
IHttpLlmFunction,
LlmTypeChecker,
OpenApi,
OpenApiV3,
OpenApiV3_1,
SwaggerV2,
} from "@samchon/openapi";
import fs from "fs";
import typia from "typia";
import { v4 } from "uuid";
const main = async (): Promise<void> => {
// read swagger document and validate it
const swagger:
| SwaggerV2.IDocument
| OpenApiV3.IDocument
| OpenApiV3_1.IDocument = JSON.parse(
await fs.promises.readFile("swagger.json", "utf8"),
);
typia.assert(swagger); // recommended
// convert to emended OpenAPI document,
// and compose LLM function calling application
const document: OpenApi.IDocument = OpenApi.convert(swagger);
const application: IHttpLlmApplication = HttpLlm.application(document, {
keyword: false,
separate: (schema) =>
LlmTypeChecker.isString(schema) && schema.contentMediaType !== undefined,
});
// Let's imagine that LLM has selected a function to call
const func: IHttpLlmFunction | undefined = application.functions.find(
// (f) => f.name === "llm_selected_fuction_name"
(f) => f.path === "/bbs/articles/{id}" && f.method === "put",
);
if (func === undefined) throw new Error("No matched function exists.");
// actual execution is by yourself
const article = await HttpLlm.execute({
connection: {
host: "http://localhost:3000",
},
application,
function: func,
arguments: HttpLlm.mergeParameters({
function: func,
llm: [
// LLM composed parameter values
"general",
v4(),
{
language: "en-US",
format: "markdown",
},
{
title: "Hello, world!",
content: "Let's imagine that this argument is composed by LLM.",
},
],
human: [
// Human composed parameter values
{ thumbnail: "https://example.com/thumbnail.jpg" },
],
}),
});
console.log("article", article);
};
main().catch(console.error);
FAQs
OpenAPI definitions and converters for 'typia' and 'nestia'.
We found that @samchon/openapi demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
At its inaugural meeting, the JSR Working Group outlined plans for an open governance model and a roadmap to enhance JavaScript package management.
Security News
Research
An advanced npm supply chain attack is leveraging Ethereum smart contracts for decentralized, persistent malware control, evading traditional defenses.
Security News
Research
Attackers are impersonating Sindre Sorhus on npm with a fake 'chalk-node' package containing a malicious backdoor to compromise developers' projects.