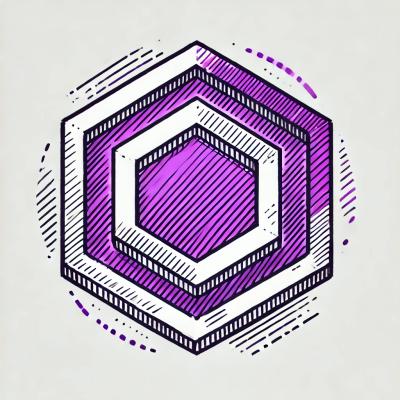
Security News
ESLint is Now Language-Agnostic: Linting JSON, Markdown, and Beyond
ESLint has added JSON and Markdown linting support with new officially-supported plugins, expanding its versatility beyond JavaScript.
@sanity/block-content-to-html
Advanced tools
Converts the flat Sanity block content structure into HTML.
npm install --save @sanity/block-content-to-html
// The flat block content structure
const data = {
"_type": "block",
"style": "normal",
"spans": [
{
"_type": "span",
"text": "String with an ",
"marks": []
},
{
"_type": "span",
"text": "italicized",
"marks": [
"em"
]
},
{
"_type": "span",
"text": " word.",
"marks": []
}
]
}
// Now convert it with block-content-to-html
const BlockContentToHtml = require('@sanity/block-content-to-html')
const toHtml = new BlockContentToHtml()
const html = toHtml.convert(data)
This will result in html
being:
<p>String with an <em>italicized</em> word.</p>
The constructor will take an object for options:
const toHtml = new BlockContentToHtml(options: Object)
Converting the block content is done by calling .parse
:
toHtml.convert(data: Array|Object)
Options is an object with any of the following keys:
customTypeHandlers: Object
An object with keys for your custom block types (which is not of type block
).
Each key is mapped to a type, and their value is a function which will get the node as input.
It returns HTML.
customTypeHandlers: {
author: node => {
return `<div>${node.attributes.name}</div>`
}
}
The node
in this example has the following structure:
{ type: 'author', attributes: { name: 'Test Person' } }
blockTypeHandlers: Object
Handlers for manipulating the output of the default, built in, block types. The default block type holds either a block of text or a list. A text block is built up of spans (with marks), where a list block is built up of list items, which can contain a text block.
The blockTypeHandlers
object can contain the follow keys:
textBlock: Object
Each text block has a style
. With this option you can manipulate how each style is rendered.
By default the style normal
is wrapped in a <p>
,
where other default styles are mapped 1:1 (style h2
produces <h2>
).
The option works in a similar way as customTypeHandlers
described above,
with the distinction that is has a children
property, and the key is the style name:
textBlock: {
normal: node => {
return `<p class="funky-paragraph">${node.children}</p>`
},
h2: node => {
return `<div class="big-heading">${node.children}</div>`
}
}
listBlock: Object
By default lists are rendered with plain <ol>
, <ul>
and <li>
tags.
With this option you can tweak them into your own liking.
The object takes the following keys:
listBlock: {
number: node => {
return `<ol class="article-list">${node.children}</ol>`
},
bullet: node => {
return `<ul class="article-list">${node.children}</ul>`
},
listItem: node => {
return `<li class="article-list-item">${node.children}</li>`
}
}
span: Object
Let you tweak how spans within blocks are rendered. By default the spans are just text and marks. As spans may have attributes with data, you can make your own render which manipulates the output based on their attributes:
span: node => {
let result = ''
if (node.attributes.author) {
result = `
<div class="author-bio">
<img src="${node.attributes.author.image.url}" />
${node.attributes.author.name}
</div>`
}
if (node.attributes.link) {
result += `<a href="${node.attributes.link.href}">${node.children}</a>`
}
if (Object.keys(node.attributes).length === 0) {
result = node.children
}
return result
}
marks: Object
Marks are by default mapped 1:1. If the mark is 'em' the output will be <em>
.
With this option you can map marks to other tags, or just ignore them:
marks: {
em: null // Just igonore 'em' tags.
code: 'pre' // Render 'code' marks to 'pre' tags
}
BlockContentToHtml.escapeHtml(unsafe: string)
Escape unsafe text to HTML safe text. To be used with your own handler functions.
Please see the tests.
MIT-licensed
FAQs
Function for transforming Sanity block content to HTML
The npm package @sanity/block-content-to-html receives a total of 4,191 weekly downloads. As such, @sanity/block-content-to-html popularity was classified as popular.
We found that @sanity/block-content-to-html demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 24 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
ESLint has added JSON and Markdown linting support with new officially-supported plugins, expanding its versatility beyond JavaScript.
Security News
Members Hub is conducting large-scale campaigns to artificially boost Discord server metrics, undermining community trust and platform integrity.
Security News
NIST has failed to meet its self-imposed deadline of clearing the NVD's backlog by the end of the fiscal year. Meanwhile, CVE's awaiting analysis have increased by 33% since June.