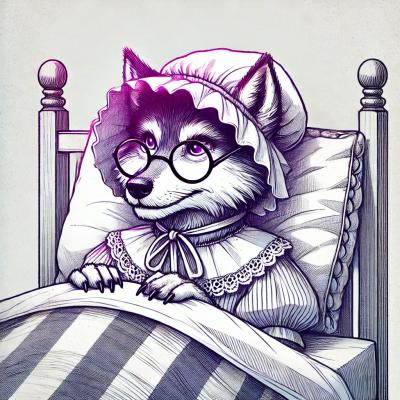
Security News
GitHub Removes Malicious Pull Requests Targeting Open Source Repositories
GitHub removed 27 malicious pull requests attempting to inject harmful code across multiple open source repositories, in another round of low-effort attacks.
@sanity/import
Advanced tools
@sanity/import is an npm package designed to facilitate the import of data into a Sanity.io dataset. It allows users to import documents and assets from various sources, making it easier to manage and migrate content within the Sanity content platform.
Import JSON Data
This feature allows you to import JSON data into a specified Sanity dataset. The code sample demonstrates how to read a JSON file and import its contents into Sanity using the @sanity/import package.
const sanityImport = require('@sanity/import');
const fs = require('fs');
const inputStream = fs.createReadStream('path/to/your/data.json');
const options = {
dataset: 'your-dataset-name',
projectId: 'your-project-id',
token: 'your-auth-token',
assetConcurrency: 3,
operation: 'createOrReplace'
};
sanityImport(inputStream, options).then(() => {
console.log('Data import completed!');
}).catch((err) => {
console.error('Import failed: ', err);
});
Import Assets
This feature allows you to import assets (e.g., images, files) into a specified Sanity dataset. The code sample demonstrates how to read a ZIP file containing assets and import them into Sanity using the @sanity/import package.
const sanityImport = require('@sanity/import');
const fs = require('fs');
const inputStream = fs.createReadStream('path/to/your/assets.zip');
const options = {
dataset: 'your-dataset-name',
projectId: 'your-project-id',
token: 'your-auth-token',
assetConcurrency: 3,
operation: 'createOrReplace'
};
sanityImport(inputStream, options).then(() => {
console.log('Assets import completed!');
}).catch((err) => {
console.error('Import failed: ', err);
});
The contentful-import package is used to import data into Contentful, a content management system similar to Sanity. It allows users to import content types, entries, and assets into a Contentful space. Compared to @sanity/import, contentful-import is specific to the Contentful platform and offers similar functionalities for data migration and content management.
Imports documents from an ndjson-stream to a Sanity dataset
npm install --save @sanity/import
const fs = require('fs')
const sanityClient = require('@sanity/client')
const sanityImport = require('@sanity/import')
const client = sanityClient({
projectId: '<your project id>',
dataset: '<your target dataset>',
token: '<token-with-write-perms>',
useCdn: false,
})
// Input can either be a readable stream (for a `.tar.gz` or `.ndjson` file), a folder location (string), or an array of documents
const input = fs.createReadStream('my-documents.ndjson')
const options = {
/**
* A Sanity client instance, preconfigured with the project ID and dataset
* you want to import data to, and with a token that has write access.
*/
client: client,
/**
* Which mutation type to use for creating documents:
* `create` (default) - throws error if document IDs already exists
* `createOrReplace` - replaces documents with same IDs
* `createIfNotExists` - skips document with IDs that already exists
*
* Optional.
*/
operation: 'create',
/**
* Function called when making progress. Gets called with an object of
* the following shape:
* `step` (string) - the current step name of the import process
* `current` (number) - the current progress of the step, only present on some steps
* `total` (number) - total items before complete, only present on some steps
*/
onProgress: (progress) => {
/* report progress */
},
/**
* Whether or not to allow assets in different datasets. This is usually
* an error in the export, where asset documents are part of the export.
*
* Optional, defaults to `false`.
*/
allowAssetsInDifferentDataset: false,
/**
* Whether or not to allow failing assets due to download/upload errors.
*
* Optional, defaults to `false`.
*/
allowFailingAssets: false,
/**
* Whether or not to replace any existing assets with the same hash.
* Setting this to `true` will regenerate image metadata on the server,
* but slows down the import.
*
* Optional, defaults to `false`.
*/
replaceAssets: false,
/**
* Whether or not to skip cross-dataset references. This may be required
* when importing a dataset with cross-dataset references to a different
* project, unless a dataset with the referenced name exists.
*
* Optional, defaults to `false`.
*/
skipCrossDatasetReferences: false,
/**
* Whether or not to import system documents (like permissions and custom retention). This
* is usually not necessary, and may cause conflicts if the target dataset
* already contains these documents. On a new dataset, it is recommended that roles are re-created
* manually, and that any custom retention policies are re-created manually.
*
* Optional, defaults to `false`.
*/
allowSystemDocuments: false,
}
sanityImport(input, options)
.then(({numDocs, warnings}) => {
console.log('Imported %d documents', numDocs)
// Note: There might be warnings! Check `warnings`
})
.catch((err) => {
console.error('Import failed: %s', err.message)
})
This functionality is built in to the @sanity/cli
package as well as a standalone @sanity/import-cli package.
MIT-licensed. See LICENSE.
FAQs
Import documents to a Sanity dataset
We found that @sanity/import demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 61 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
GitHub removed 27 malicious pull requests attempting to inject harmful code across multiple open source repositories, in another round of low-effort attacks.
Security News
RubyGems.org has added a new "maintainer" role that allows for publishing new versions of gems. This new permission type is aimed at improving security for gem owners and the service overall.
Security News
Node.js will be enforcing stricter semver-major PR policies a month before major releases to enhance stability and ensure reliable release candidates.