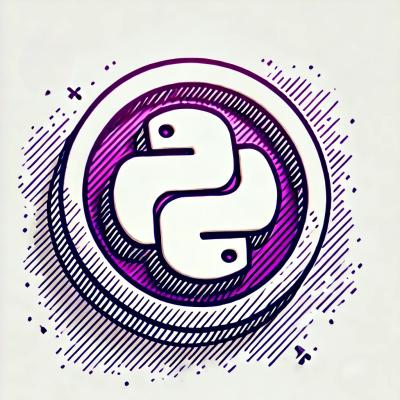
Security News
PyPI Introduces Digital Attestations to Strengthen Python Package Security
PyPI now supports digital attestations, enhancing security and trust by allowing package maintainers to verify the authenticity of Python packages.
@scienta/locking-cache
Advanced tools
Caching values with (distributed) locking. Easily extensible with your own storage and locking implementations
Cache values and lock while resolving the value to cache. Lots of caching libraries do not lock the process fetching the value to be cached. That means that multiple resource-intensive processes, that you want 2 cache, can be running computing a value at the same time.
This library combines locking with caching and makes sure only one process computing the same cacheable value will run at the same time.
This library is in essence a wrapper for different cache-storage and locking mechanisms. It does not force you to use a specific backend for storage, or a complex distributed locking library. It does come with some ready-to-use storage and locking implementations.
Locking and caching works with promises. In this way it is easy to use with async/await.
There are pre-made storage and locking implementations for single-threaded and multi-threaded caching.
import { LockingCache } from "@scienta/locking-cache";
// Expensive (async) function requesting an access token
const requestTokenMock = () => {
// Fetch api access token (async), cache for 10 minutes.
//
// Calling this multiple times in parallel will only run it once.
// the cache key is based on the function name and arguments.
return Promise.resolve({
value: 'fea80f2db003d4ebc4536023814aa885', //access token
expiresInSec: 60 * 10 //10 minutes
});
}
const cache = new LockingCache<string>();
cache.getValue(clientId, requestTokenMock).then(result => {
// Use cached token for requesting resources
});
import {IoRedisStorage, LockingCache, RedisLocker} from "@scienta/locking-cache";
import * as IORedis from "ioredis";
import * as Redlock from "redlock";
const ioRedis = new IORedis();
const cache = new LockingCache<string>(
new IoRedisStorage(ioRedis, { namespace: 'cache' }), // cache storage in redis
new RedisLocker(new Redlock(ioRedis)) // distributed locking in redis
);
const result = await cache.getValue(clientId, async () => {
return {
value: 'fea80f2db003d4ebc4536023814aa885', //access token
expiresInSec: 60 * 10 //10 minutes
};
});
There are a number of errors that can come up when caching:
export enum CacheErrorEvents {
resolveError = 'resolveError',
storeGetError = 'storeGetError',
storeSetError = 'storeSetError',
lockError = 'lockError',
unlockError = 'unlockError'
}
If an error occurs, most of the time it will result in a rejected promise from getValue()
:
const cache = new LockingCache<string>();
try {
const result = await cache.getValue(clientId, requestTokenMock);
} catch (error) {
// handle resolveError|storeGetError|storeSetError(|lockError)
}
The unlockError
is an exception (it does not get thrown) and the lockError
can be thrown optionally
by setting cacheOnLockError
of the LockingCache
to false
;
Still there is a way to listen to errors, because the LockingCache
also emits them:
const cache = new LockingCache<string>();
cache.on('unlockError', (error) => {
//handle unlockError
});
cache.getValue(clientId, requestTokenMock).then(result => {
// Use cached token for requesting resources
}).catch((error) => {
// handle resolveError|storeGetError|storeSetError(|lockError)
});
If you want to contribute, please do so. A docker-compose.yml
file is added to make development easy.
To start development within the defined container, just use docker-compose:
docker-compose up -d
docker exec -ti locking-cache bash
To quickly run tests and linting from a docker container, you can also use docker directly:
docker run -it -w="/app" -v ${PWD}:/app node:14-slim /bin/bash -c "npm install && npm run test && npm run lint"
FAQs
Caching values with (distributed) locking. Easily extensible with your own storage and locking implementations
The npm package @scienta/locking-cache receives a total of 576 weekly downloads. As such, @scienta/locking-cache popularity was classified as not popular.
We found that @scienta/locking-cache demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 3 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
PyPI now supports digital attestations, enhancing security and trust by allowing package maintainers to verify the authenticity of Python packages.
Security News
GitHub removed 27 malicious pull requests attempting to inject harmful code across multiple open source repositories, in another round of low-effort attacks.
Security News
RubyGems.org has added a new "maintainer" role that allows for publishing new versions of gems. This new permission type is aimed at improving security for gem owners and the service overall.