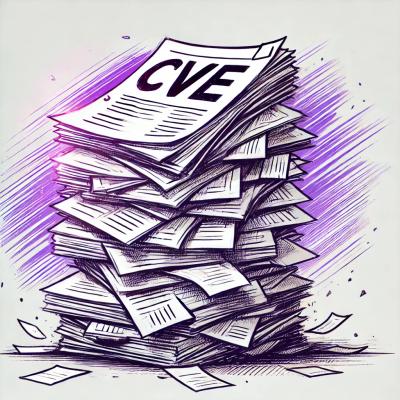
Security News
Node.js EOL Versions CVE Dubbed the "Worst CVE of the Year" by Security Experts
Critics call the Node.js EOL CVE a misuse of the system, sparking debate over CVE standards and the growing noise in vulnerability databases.
@setho/dynamodb-repository
Advanced tools
DynamoDB repository for hash-key and hash-key/range indexed tables. Designed for Lambda use. Handles nice-to-haves like created and updated timestamps and default id creation.
DynamoDB repository for key-value indexed tables. Designed for Lambda use. Handles niceties like created and updated timestamps and default id creation.
$ npm install @setho/dynamodb-repository --save
import { KeyValueRepository } from '@setho/dynamodb-repository';
const myRepo = new KeyValueRepository({
tableName: 'Items', // Required
keyName: 'id', // Required
idOptions: {
// Optional
prefix: 'ITEM_', // Default is empty string
},
documentClient, // Required - V3 DynamoDBDocumentClient from @aws-sdk/lib-dynamodb
});
const { KeyValueRepository } = require('@setho/dynamodb-repository');
const myRepo = new KeyValueRepository({
tableName: 'Items', // Required
keyName: 'id', // Required
idOptions: {
// Optional
prefix: 'ITEM#', // Default is empty string
},
documentClient, // Required - V3 DynamoDBDocumentClient from @aws-sdk/lib-dynamodb
});
Use the optional idOptions
constructor parameter to set an optional prefix
to give your ids some human-readable context. The remainder of the key is a ULID, which is both unique and lexicographically sortable. See an explanation of and motivation for ULIDs here.
dynamodb:PutItem
createdAt
and updatedAt
timestamp in ISO-8601const mySavedItem = await myRepo.create(myItem);
mySavedItem.id; // itm_a4d02890b7174730b4bbbc
mySavedItem.createdAt; // 1979-11-04T09:00:00.000Z
mySavedItem.updatedAt; // 1979-11-04T09:00:00.000Z
dynamodb:GetItem
.404
if item not found using http-errors.const myItem = await myRepo.get(id);
dynamodb:Scan
limit
and cursor
limit
defaults to 100items
(Array) and cursor
(String)
items
will always be an array; if nothing found, it will be emptycursor
will be present if there are more items to fetch, otherwise it will be undefined// Example to pull 100 at time until you have all items
const allItems = [];
const getAllItems = async ({ limit, cursor = null }) => {
const getResult = await myRepo.getMany({ limit, cursor });
allItems.push(...getResult.items);
if (getResult.cursor) {
await getAllItems({ cursor: getResult.cursor });
}
};
await getAllItems();
// The array allItems now has all your items. Go nuts.
dynamodb:DeleteItem
await myRepo.remove(id);
dynamodb:UpdateItem
409
if the revision has changed underneath you.key
and revision
properties are always required.const person = await myRepo.create({
name: 'Joe',
age: 28,
favoriteColor: 'blue',
});
// Full item update
person.favoriteColor = 'teal';
const newPerson1 = await myRepo.update(person);
console.log(newPerson1.favoriteColor); // 'teal'
// Partial update
const partial = {
favoriteColor: 'aquamarine',
key: person.key,
revision: newPerson1.revision,
};
const newPerson2 = await myRepo.update(partial);
console.log(newPerson2.favoriteColor); // 'aquamarine'
console.log(newPerson2.age); // 28
// More Coming Soon...
FAQs
DynamoDB repository for hash-key and hash-key/range indexed tables. Designed for Lambda use. Handles nice-to-haves like created and updated timestamps and default id creation.
We found that @setho/dynamodb-repository demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Critics call the Node.js EOL CVE a misuse of the system, sparking debate over CVE standards and the growing noise in vulnerability databases.
Security News
cURL and Go security teams are publicly rejecting CVSS as flawed for assessing vulnerabilities and are calling for more accurate, context-aware approaches.
Security News
Bun 1.2 enhances its JavaScript runtime with 90% Node.js compatibility, built-in S3 and Postgres support, HTML Imports, and faster, cloud-first performance.