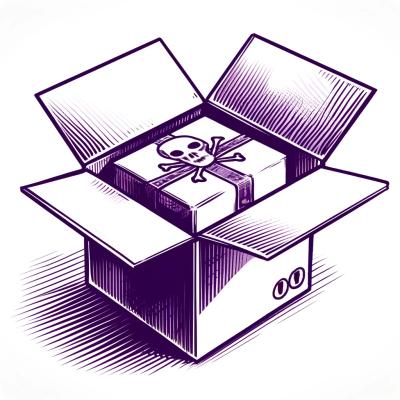
Security News
Research
Data Theft Repackaged: A Case Study in Malicious Wrapper Packages on npm
The Socket Research Team breaks down a malicious wrapper package that uses obfuscation to harvest credentials and exfiltrate sensitive data.
@shikijs/vscode-textmate
Advanced tools
@shikijs/vscode-textmate is a library that provides TextMate grammar support for syntax highlighting in JavaScript and TypeScript applications. It allows developers to use TextMate grammars to tokenize source code and apply syntax highlighting, similar to how Visual Studio Code does it.
Loading a TextMate Grammar
This code demonstrates how to load a TextMate grammar for JavaScript using the @shikijs/vscode-textmate package. It reads a grammar file and parses it into a usable format.
const vscodeTextmate = require('@shikijs/vscode-textmate');
const fs = require('fs');
const path = require('path');
async function loadGrammar() {
const registry = new vscodeTextmate.Registry({
loadGrammar: (scopeName) => {
if (scopeName === 'source.js') {
return new Promise((resolve, reject) => {
fs.readFile(path.join(__dirname, 'JavaScript.tmLanguage.json'), 'utf8', (err, data) => {
if (err) {
reject(err);
} else {
resolve(vscodeTextmate.parseRawGrammar(data, path.join(__dirname, 'JavaScript.tmLanguage.json')));
}
});
});
}
return null;
}
});
const grammar = await registry.loadGrammar('source.js');
console.log(grammar);
}
loadGrammar();
Tokenizing a Line of Code
This code demonstrates how to tokenize a line of JavaScript code using a loaded TextMate grammar. It shows how to initialize the rule stack and tokenize a given line of code.
const vscodeTextmate = require('@shikijs/vscode-textmate');
async function tokenizeLine(grammar, line) {
const ruleStack = vscodeTextmate.INITIAL;
const lineTokens = grammar.tokenizeLine(line, ruleStack);
console.log(lineTokens);
}
async function main() {
const registry = new vscodeTextmate.Registry({
loadGrammar: async (scopeName) => {
if (scopeName === 'source.js') {
const grammar = await fetch('path/to/JavaScript.tmLanguage.json').then(res => res.json());
return vscodeTextmate.parseRawGrammar(JSON.stringify(grammar));
}
return null;
}
});
const grammar = await registry.loadGrammar('source.js');
tokenizeLine(grammar, 'const x = 42;');
}
main();
The vscode-textmate package is the original library used by Visual Studio Code for TextMate grammar support. It provides similar functionality to @shikijs/vscode-textmate, allowing developers to load and tokenize source code using TextMate grammars. The main difference is that @shikijs/vscode-textmate is a fork of vscode-textmate with additional features and improvements.
highlight.js is a popular library for syntax highlighting in web applications. Unlike @shikijs/vscode-textmate, which uses TextMate grammars, highlight.js uses its own language definitions. It is easier to set up and use but may not offer the same level of customization and accuracy as TextMate-based solutions.
Prism is another popular syntax highlighting library for web applications. It is similar to highlight.js in that it uses its own language definitions rather than TextMate grammars. Prism is known for its lightweight and extensible design, making it a good choice for projects that need a simple and fast syntax highlighting solution.
microsoft/vscode-textmate
Changes make in this fork:
async
operations to sync
; onigLib
option now required to be resolved instead of a promise.tsup
to bundle the lib, ship as a single file ES module.EncodedTokenAttributes
from namespace to class, rename to EncodedTokenMetadata
FAQs
Shiki's fork of `vscode-textmate`
We found that @shikijs/vscode-textmate demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 3 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Research
The Socket Research Team breaks down a malicious wrapper package that uses obfuscation to harvest credentials and exfiltrate sensitive data.
Research
Security News
Attackers used a malicious npm package typosquatting a popular ESLint plugin to steal sensitive data, execute commands, and exploit developer systems.
Security News
The Ultralytics' PyPI Package was compromised four times in one weekend through GitHub Actions cache poisoning and failure to rotate previously compromised API tokens.