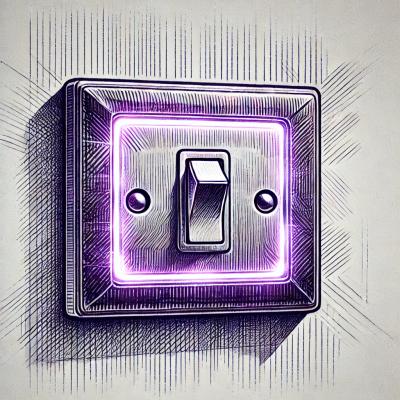
Research
Security News
Kill Switch Hidden in npm Packages Typosquatting Chalk and Chokidar
Socket researchers found several malicious npm packages typosquatting Chalk and Chokidar, targeting Node.js developers with kill switches and data theft.
@sidespin/protomodule
Advanced tools
**How to install?** ```yarn add @sidespin/protomodule```
How to install?
yarn add @sidespin/protomodule
we have antd as our foundation for UI components has been powered by @sidespin/protomodule, you can simply import and use it like this:
import { Input } from 'antd';
const App = () => (<Input />)
import { initConfig } from '@sidespin/protomodule';
import configJson from './config.json';
initConfig({
routes: import.meta.globEager(`src/pages/**/**.tsx`),
configJson
})
you don't need to worry about routing anymore. @sidespin/protomodule facilitates you with Conventional / File System Routing. You need to provide routes initConfig handler.
import { useRouter } from '@sidespin/protomodule'
const App = () => {
const [Router, Routes] = useRouter();
return (
<Router>
<Routes />
</Router>
)
}
you don't need to worry about authenticating a user first on auth0 then fetching his info from core server. we handle it for you. You need to provide VITE_AUTH_API_URL (auth0 domain) and VITE_CLIENT_ID (auth0 clientId) in env. and configJson in initConfig handler
import { WithAuth } from '@sidespin/protomodule'
const App = () => {
const [Router, Routes] = useRouter();
return (
<WithAuth>
<Router>
<Routes />
</Router>
</WithAuth>
)
}
in src/pages/**
import { useUser } from '@sidespin/protomodule';
import { Button } from 'antd';
const Component = () => {
const { loading, user, login } = useUser();
return <Button loading={loading} onClick={login}>Login</Button>
}
you don't need to worry about fetching service info from core server. we handle it for you. You need to provide VITE_API_BASE_URL (core-api url) in env. and configJson in initConfig handler
import { WithService, useRouter } from '@sidespin/protomodule'
const App = () => {
const [Router, Routes] = useRouter();
return (
<WithService>
<Router>
<Routes />
</Router>
</WithService>
)
}
in src/pages/**
import { userService } from '@sidespin/protomodule';
import { Button } from 'antd';
const Component = () => {
const { loading, service } = userService();
return <span>{service?.name}</span>
}
FAQs
**How to install?** ```yarn add @sidespin/protomodule```
We found that @sidespin/protomodule demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 2 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
Socket researchers found several malicious npm packages typosquatting Chalk and Chokidar, targeting Node.js developers with kill switches and data theft.
Security News
pnpm 10 blocks lifecycle scripts by default to improve security, addressing supply chain attack risks but sparking debate over compatibility and workflow changes.
Product
Socket now supports uv.lock files to ensure consistent, secure dependency resolution for Python projects and enhance supply chain security.