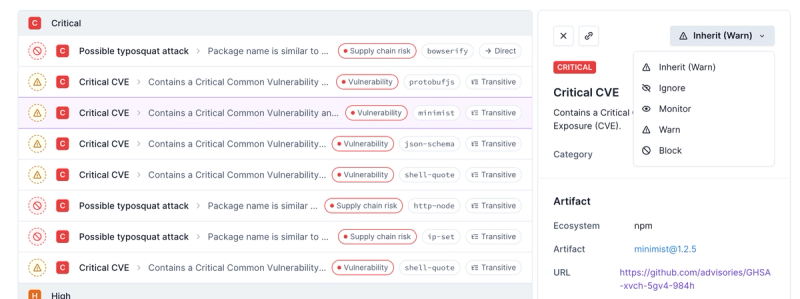
Product
Introducing Enhanced Alert Actions and Triage Functionality
Socket now supports four distinct alert actions instead of the previous two, and alert triaging allows users to override the actions taken for all individual alerts.
@smallwins/validate
Advanced tools
[](https://github.com/smallwins/validate/actions?query=workflow%3A%22Node+CI%22)
Readme
Parameter validation for builtins and custom types. Accepts params
and a schema
and returns an array of Error
s or false
.
var validate = require('@smallwins/validate')
function hi(params, callback) {
var schema = {
'name': {required:true, type:Object},
'name.first': {required:true, type:String},
'name.last': {required:false, type:String}
}
var errors = validate(params, schema)
if (errors) {
callback(errors)
}
else {
callback(null, 'hi ' + params.first)
}
}
// logs: null, hi brian
hi({name:{first:'brian', last:'leroux'}}, console.log)
// logs: [ [ReferenceError: missing required param name.first] ]
hi({name:{}}, console.log)
Object
that presumably came from a JSON payloadObject
, String
, Number
, Array
and Boolean
Email
, ISO
, DateRange
and UUID
required
min
and max
for String
, Number
and Array
builtin types (and easily implement for custom types / see DateRange
for an example)There are a tonne of libraries that do things like this but also do a whole lot more. This library deliberately limits its scope:
null
instead of a truthy empty array []
for the return value of validate
and making the schema type validation minimalist without sacrificing capability.)var validate = require('@smallwins/validate')
function sum(params, callback) {
// define our assumed params
var errors = validate(params, {
x: {required:true, type:Number},
y: {required:true, type:Number}
})
// err first! it'll be null w/ good input
callback(errors, errors? null : params.x + params.y)
}
sum({}, console.log)
// logs
// [[ReferenceError: missing required param x], [ReferenceError: missing required param y]] null
sum({x:1, y:2}, console.log)
// logs
// null 2
validate(params, schema)
params
a plain Object
that we assume came from JSONschema
a plain Object
for describing the shape of the datacallback
(optional) Node style errback function(err, params) {}
required
either true
or false
(or leave it out completely)type
can be one of Object
, String
, Number
, Array
and Boolean
min
any Number
(or anything allowed by a custom type)max
any Number
(or anything allowed by a custom type)UUID
Email
ISO
DateRange
Example usage of custom types:
var validate = require('@smallwins/validate')
var lambda = require('@smallwins/lambda')
// pull in the custom types
var UUID = require('@smallwins/validate/uuid')
var Email = require('@smallwins/validate/email')
var ISO = require('@smallwins/validate/iso')
var DateRange = require('@smallwins/validate/daterange')
// use the schema per builtins
function valid(event, callback) {
var schema = {
'params.id': {required:true, type:UUID},
'body.email': {required:true, type:Email},
'body.created': {required:true, type:ISO},
'body.duration': {required:true, type:DateRange, min:'2016/01/01', max:'2017/01/01'}
}
validate(event, schema, callback)
}
function save(event, callback) {
// performs save
callback(null, event)
}
exports.handler = lambda(valid, save)
Check out the examples for more on custom types and ranges (and the tests).
FAQs
[](https://github.com/smallwins/validate/actions?query=workflow%3A%22Node+CI%22)
The npm package @smallwins/validate receives a total of 20 weekly downloads. As such, @smallwins/validate popularity was classified as not popular.
We found that @smallwins/validate demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 3 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Product
Socket now supports four distinct alert actions instead of the previous two, and alert triaging allows users to override the actions taken for all individual alerts.
Security News
Polyfill.io has been serving malware for months via its CDN, after the project's open source maintainer sold the service to a company based in China.
Security News
OpenSSF is warning open source maintainers to stay vigilant against reputation farming on GitHub, where users artificially inflate their status by manipulating interactions on closed issues and PRs.