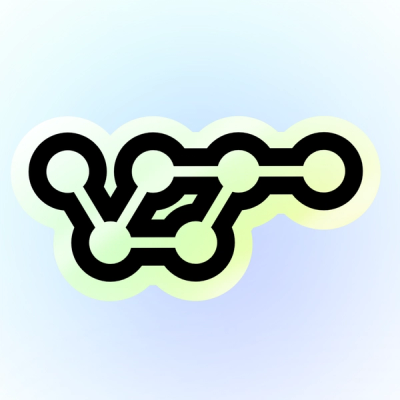
Security News
vlt Debuts New JavaScript Package Manager and Serverless Registry at NodeConf EU
vlt introduced its new package manager and a serverless registry this week, innovating in a space where npm has stagnated.
@smithy/md5-js
Advanced tools
[](https://www.npmjs.com/package/@smithy/md5-js) [](https://www.npmjs.com/package/@smithy/md5-js)
@smithy/md5-js is an npm package that provides MD5 hashing functionality for JavaScript applications. It is part of the Smithy suite of tools, which are designed to work with AWS SDKs and other Smithy-based projects.
Generate MD5 Hash
This feature allows you to generate an MD5 hash from a given input string. The code sample demonstrates how to create an MD5 hash of the string 'Hello, world!' and print it in hexadecimal format.
const { Md5 } = require('@smithy/md5-js');
const md5 = new Md5();
md5.update('Hello, world!');
const hash = md5.digestSync();
console.log(Buffer.from(hash).toString('hex'));
Update Hash with Multiple Chunks
This feature allows you to update the MD5 hash with multiple chunks of data. The code sample demonstrates how to update the hash with two separate strings and then generate the final hash.
const { Md5 } = require('@smithy/md5-js');
const md5 = new Md5();
md5.update('Hello, ');
md5.update('world!');
const hash = md5.digestSync();
console.log(Buffer.from(hash).toString('hex'));
Asynchronous Hashing
This feature allows you to perform MD5 hashing asynchronously. The code sample demonstrates how to generate an MD5 hash of the string 'Hello, world!' using a promise-based approach.
const { Md5 } = require('@smithy/md5-js');
const md5 = new Md5();
md5.update('Hello, world!');
md5.digest().then(hash => {
console.log(Buffer.from(hash).toString('hex'));
});
crypto-js is a widely-used library that provides a variety of cryptographic algorithms, including MD5. It offers a more extensive set of features compared to @smithy/md5-js, such as support for AES, SHA-1, SHA-256, and more. It is also well-documented and has a large user base.
md5 is a simple and lightweight npm package specifically designed for generating MD5 hashes. It is easy to use and has a straightforward API, making it a good alternative for projects that only require MD5 hashing without the additional features provided by @smithy/md5-js.
hash.js is a library that provides a variety of hash functions, including MD5, SHA-1, and SHA-256. It is designed to be fast and efficient, making it suitable for performance-critical applications. Compared to @smithy/md5-js, hash.js offers a broader range of hashing algorithms.
FAQs
[](https://www.npmjs.com/package/@smithy/md5-js) [](https://www.npmjs.com/package/@smithy/md5-js)
The npm package @smithy/md5-js receives a total of 4,790,034 weekly downloads. As such, @smithy/md5-js popularity was classified as popular.
We found that @smithy/md5-js demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 2 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
vlt introduced its new package manager and a serverless registry this week, innovating in a space where npm has stagnated.
Security News
Research
The Socket Research Team uncovered a malicious Python package typosquatting the popular 'fabric' SSH library, silently exfiltrating AWS credentials from unsuspecting developers.
Security News
At its inaugural meeting, the JSR Working Group outlined plans for an open governance model and a roadmap to enhance JavaScript package management.