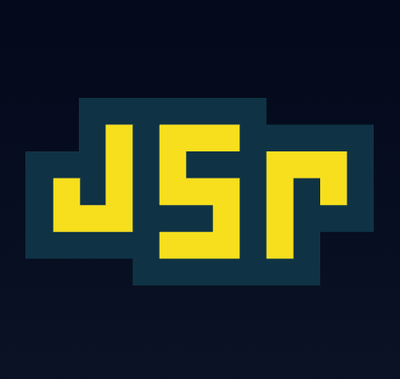
Security News
JSR Working Group Kicks Off with Ambitious Roadmap and Plans for Open Governance
At its inaugural meeting, the JSR Working Group outlined plans for an open governance model and a roadmap to enhance JavaScript package management.
@solana/web3.js
Advanced tools
@solana/web3.js is a JavaScript library for interacting with the Solana blockchain. It provides a set of tools and utilities for developers to build applications on Solana, including functionalities for managing accounts, sending transactions, interacting with smart contracts, and querying blockchain data.
Create and Manage Accounts
This feature allows you to create and manage Solana accounts. The code sample demonstrates how to generate a new keypair, which includes a public key and a secret key.
const solanaWeb3 = require('@solana/web3.js');
// Generate a new keypair
const keypair = solanaWeb3.Keypair.generate();
console.log('Public Key:', keypair.publicKey.toBase58());
console.log('Secret Key:', keypair.secretKey);
Send Transactions
This feature allows you to send transactions on the Solana blockchain. The code sample demonstrates how to create a connection to the Solana devnet, airdrop SOL to an account, create a transaction to transfer SOL, and send the transaction.
const solanaWeb3 = require('@solana/web3.js');
(async () => {
const connection = new solanaWeb3.Connection(solanaWeb3.clusterApiUrl('devnet'), 'confirmed');
const from = solanaWeb3.Keypair.generate();
const to = solanaWeb3.Keypair.generate();
// Airdrop SOL to the from account
await connection.requestAirdrop(from.publicKey, solanaWeb3.LAMPORTS_PER_SOL);
// Create a transaction
const transaction = new solanaWeb3.Transaction().add(
solanaWeb3.SystemProgram.transfer({
fromPubkey: from.publicKey,
toPubkey: to.publicKey,
lamports: solanaWeb3.LAMPORTS_PER_SOL / 100,
})
);
// Sign and send the transaction
const signature = await solanaWeb3.sendAndConfirmTransaction(connection, transaction, [from]);
console.log('Transaction signature:', signature);
})();
Interact with Smart Contracts
This feature allows you to interact with smart contracts deployed on the Solana blockchain. The code sample demonstrates how to create a transaction that calls a smart contract and send the transaction.
const solanaWeb3 = require('@solana/web3.js');
(async () => {
const connection = new solanaWeb3.Connection(solanaWeb3.clusterApiUrl('devnet'), 'confirmed');
const programId = new solanaWeb3.PublicKey('YourProgramIdHere');
const account = solanaWeb3.Keypair.generate();
// Create a transaction to call a smart contract
const transaction = new solanaWeb3.Transaction().add(
new solanaWeb3.TransactionInstruction({
keys: [{ pubkey: account.publicKey, isSigner: true, isWritable: true }],
programId,
data: Buffer.alloc(0), // Add your instruction data here
})
);
// Sign and send the transaction
const signature = await solanaWeb3.sendAndConfirmTransaction(connection, transaction, [account]);
console.log('Transaction signature:', signature);
})();
Query Blockchain Data
This feature allows you to query data from the Solana blockchain. The code sample demonstrates how to get account information and the recent blockhash from the blockchain.
const solanaWeb3 = require('@solana/web3.js');
(async () => {
const connection = new solanaWeb3.Connection(solanaWeb3.clusterApiUrl('devnet'), 'confirmed');
const publicKey = new solanaWeb3.PublicKey('YourPublicKeyHere');
// Get account info
const accountInfo = await connection.getAccountInfo(publicKey);
console.log('Account Info:', accountInfo);
// Get recent blockhash
const recentBlockhash = await connection.getRecentBlockhash();
console.log('Recent Blockhash:', recentBlockhash);
})();
web3.js is a collection of libraries that allow you to interact with a local or remote Ethereum node using HTTP, IPC, or WebSocket. It provides similar functionalities to @solana/web3.js but is designed for the Ethereum blockchain.
ethers.js is a library for interacting with the Ethereum blockchain and its ecosystem. It provides a more lightweight and modular approach compared to web3.js and includes functionalities for managing accounts, sending transactions, and interacting with smart contracts.
near-api-js is a JavaScript library for interacting with the NEAR blockchain. It provides similar functionalities to @solana/web3.js, including account management, transaction handling, and smart contract interaction, but is designed specifically for the NEAR blockchain.
This is the Solana Javascript API built on the Solana JSON RPC API
$ yarn add @solana/web3.js
$ npm install --save @solana/web3.js
<script src="https://github.com/solana-labs/solana-web3.js/releases/download/v0.0.6/solanaWeb3.min.js"></script>
The Solana BPF SDK is located in the bpf-sdk/
subdirectory if you installed
solana-web3.js from npmjs.com.
From a git clone, run npm run bpf-sdk:install
to fetch the latest BPF SDK.
Additionally Rust must be installed to build Rust BPF programs such as
examples/bpf-rust-noop/
. See https://www.rust-lang.org/install.html for
installation details.
const solanaWeb3 = require('@solana/web3.js');
console.log(solanaWeb3);
import solanaWeb3 from '@solana/web3.js';
console.log(solanaWeb3);
// `solanaWeb3` is provided in the global namespace by the `solanaWeb3.min.js` script bundle.
console.log(solanaWeb3);
The solana-localnet
program is provided to easily start a test Solana cluster
locally on your machine. Docker must be installed. The JSON RPC endpoint of
the local cluster is http://localhost:8899
.
To start, first fetch the latest Docker image by running:
$ npx solana-localnet update
Then run the following command to start the cluster
$ npx solana-localnet up
While the cluster is running logs are available with:
$ npx solana-localnet logs -f
Stop the cluster with:
$ npx solana-localnet down
A Flow library definition is provided at module.flow.js. Add the following line under the [libs] section of your project's .flowconfig to activate it:
[libs]
node_modules/@solana/web3.js/module.flow.js
See the examples/ directory for small snippets.
Standalone examples:
Releases are available on Github and npmjs.com
Each Github release features a tarball containing API documentation and a minified version of the module suitable for direct use in a browser environment (<script> tag)
FAQs
Solana Javascript API
The npm package @solana/web3.js receives a total of 302,047 weekly downloads. As such, @solana/web3.js popularity was classified as popular.
We found that @solana/web3.js demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 14 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
At its inaugural meeting, the JSR Working Group outlined plans for an open governance model and a roadmap to enhance JavaScript package management.
Security News
Research
An advanced npm supply chain attack is leveraging Ethereum smart contracts for decentralized, persistent malware control, evading traditional defenses.
Security News
Research
Attackers are impersonating Sindre Sorhus on npm with a fake 'chalk-node' package containing a malicious backdoor to compromise developers' projects.