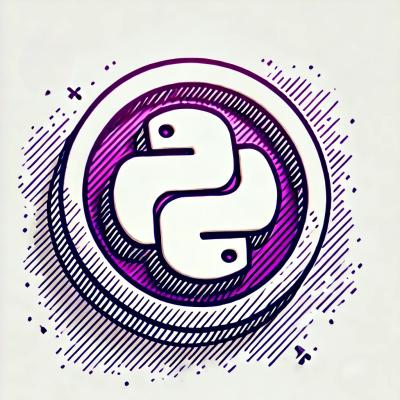
Security News
Introducing the Socket Python SDK
The initial version of the Socket Python SDK is now on PyPI, enabling developers to more easily interact with the Socket REST API in Python projects.
@solid-primitives/utils
Advanced tools
A bunch of reactive utility types and functions, for building primitives with Solid.js
Solid Primitives Utilities is a support and helper package for a number of primitives in our library. Please free to augment or centralize useful utilities and methods in this package for sharing.
npm install @solid-primitives/utils
# or
pnpm add @solid-primitives/utils
# or
yarn add @solid-primitives/utils
Functional programming helpers for making non-mutating changes to data. Keeping it immutable. Useful for updating signals.
import { pick } from "@solid-primitives/utils/immutable";
const original = { foo: 123, bar: "baz" };
const newObj = pick(original, "foo");
original; // { foo: 123, bar: "baz" }
newObj; // { foo: 123 }
Use it for changing signals:
import { push, update } from "@solid-primitives/utils/immutable";
const [list, setList] = createSignal([1, 2, 3]);
setList(p => push(p, 4));
const [user, setUser] = createSignal({
name: "John",
street: { name: "Kingston Cei", number: 24 },
});
setUser(p => update(p, "street", "number", 64));
shallowArrayCopy
- make shallow copy of an arrayshallowObjectCopy
- make shallow copy of an objectshallowCopy
- make shallow copy of an array/objectwithArrayCopy
- apply mutations to the an array without changing the originalwithObjectCopy
- apply mutations to the an object without changing the originalwithCopy
- apply mutations to the an object/array without changing the originalpush
- non-mutating Array.prototype.push()
drop
- non-mutating function that drops n items from the array startdropRight
- non-mutating function that drops n items from the array endfilterOut
- standalone Array.prototype.filter()
that filters out passed itemfilter
- standalone Array.prototype.filter()
sort
- non-mutating Array.prototype.sort()
as a standalone functionsortBy
- Sort an array by object key, or multiple keysmap
- standalone Array.prototype.map()
functionslice
- standalone Array.prototype.slice()
functionsplice
- non-mutating Array.prototype.splice()
as a standalone functionfill
- non-mutating Array.prototype.fill()
as a standalone functionconcat
- Creates a new array concatenating array with any additional arrays and/or values.remove
- Remove item from arrayremoveItems
- Remove multiple items from an arrayflatten
- Flattens a nested array into a one-level arrayfilterInstance
- Flattens a nested array into a one-level arrayfilterOutInstance
- Flattens a nested array into a one-level arrayomit
- Create a new subset object without the provided keyspick
- Create a new subset object with only the provided keyssplit
- Split object into multiple subset objects.merge
- Merges multiple objects into a single one.get
- Get a single property value of an object by specifying a path to it.update
- Change single value in an object by key, or series of recursing keys.add
- a + b + c + ...
(works for numbers or strings)substract
- a - b - c - ...
multiply
- a * b * c * ...
divide
- a / b / c / ...
power
- a ** b ** c ** ...
clamp
- clamp a number value between two other valuesSee CHANGELOG.md
FAQs
A bunch of reactive utility types and functions, for building primitives with Solid.js
The npm package @solid-primitives/utils receives a total of 69,538 weekly downloads. As such, @solid-primitives/utils popularity was classified as popular.
We found that @solid-primitives/utils demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 3 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
The initial version of the Socket Python SDK is now on PyPI, enabling developers to more easily interact with the Socket REST API in Python projects.
Security News
Floating dependency ranges in npm can introduce instability and security risks into your project by allowing unverified or incompatible versions to be installed automatically, leading to unpredictable behavior and potential conflicts.
Security News
A new Rust RFC proposes "Trusted Publishing" for Crates.io, introducing short-lived access tokens via OIDC to improve security and reduce risks associated with long-lived API tokens.