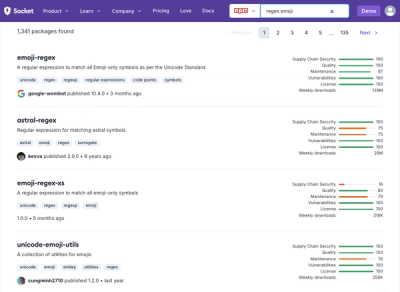
Security News
Weekly Downloads Now Available in npm Package Search Results
Socket's package search now displays weekly downloads for npm packages, helping developers quickly assess popularity and make more informed decisions.
@songkick/promise-retry
Advanced tools
Retry a function until its returned promise succeeds
var retryPromise = require('promise-retry');
var retryTwiceEveryHundredMil = retryPromise({ retries: 2, delay: 100 });
retryTwiceEveryHundredMil(resolvesTheThirdTime)()
.then(function(result){
// never called here,
// but if `retries` was >= 3,
// result would be === 'yay!'
}).catch(function(err){
// err instanceof retryPromise.OutOfRetriesError === true
// err === {
// message: 'Maximum retries count reached',
// settings: {
// retries: 2,
// },
// fn: resolvesTheThirdTime,
// errors: ['nope', 'nope', 'nope']
// }
});
var calls = 0;
function resolvesTheThirdTime() {
if (++calls < 3) {
return Promise.reject('nope');
} else {
return Promise.resolve('yay!');
}
}
retries
: positive (>= 0) number. The initial call doesn't count as a retry. If you set it to 3
, then your function might be called up to 4 times.
delay
: the delay between retries or a function(retryIndex){}
returning the delay. Does not apply on initial call. If a function is passed, it will receive the retry index as first argument (1, 2, 3 ...
).
var retryWithIncreasingDelay = retryPromise({ retries: 10, delay: function(retryIndex) {
return 100 * retryIndex;
});
/* time line:
0 > initial fail
0 + 100 > first retry
100 + 200 > second retry
300 + 300 > third retry
600 + 400 > fourth...
As promise-retry
input and output is a function returning a promise, you can compose them easily:
var retryTwice = retryPromise({ retries: 2 });
var retryAterTwoSeconds = retryPromise({ retries: 1, delay: 2000 });
var getRejected = function(){
return Promise.reject('nope');
};
retryOnceAterTwoSeconds(retryTwice(getRejected))().then(function(){
// no way here
}).catch(function(){
// at this point, `getRejected` will have been called 6 times
});
In the above exemple, the getRejected
, this will happen:
getRejected
call - callcount: 1getRejected
call - callcount: 4promise-retry
composes really well with the following promise helper:
FAQs
Retry a function until its returned promise succeeds
The npm package @songkick/promise-retry receives a total of 1 weekly downloads. As such, @songkick/promise-retry popularity was classified as not popular.
We found that @songkick/promise-retry demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 3 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Socket's package search now displays weekly downloads for npm packages, helping developers quickly assess popularity and make more informed decisions.
Security News
A Stanford study reveals 9.5% of engineers contribute almost nothing, costing tech $90B annually, with remote work fueling the rise of "ghost engineers."
Research
Security News
Socket’s threat research team has detected six malicious npm packages typosquatting popular libraries to insert SSH backdoors.