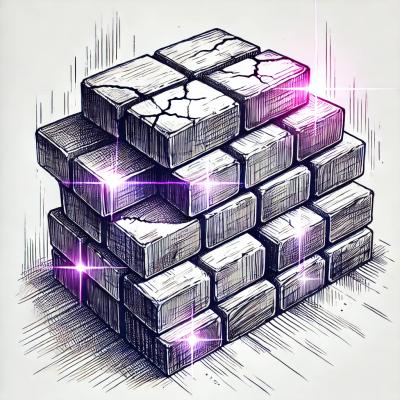
Security News
Input Validation Vulnerabilities Dominate MITRE's 2024 CWE Top 25 List
MITRE's 2024 CWE Top 25 highlights critical software vulnerabilities like XSS, SQL Injection, and CSRF, reflecting shifts due to a refined ranking methodology.
@sourceloop/audit-log
Advanced tools
The @sourceloop/audit-log
package is a powerful LoopBack 4 extension designed to seamlessly implement audit logs in your LoopBack applications. With this extension, you can effortlessly track and record audit data for all database transactions within your application.
This package provides a generic model that enables the storage of audit logs, which can be backed by any datasource of your choice. Whether you're using MySQL, PostgreSQL, MongoDB, or any other database, the Audit Logs package ensures compatibility and flexibility.
By incorporating the Audit Logs package into your application, you gain valuable insights into the history of data changes, user actions, and system events. Maintain a comprehensive audit trail for compliance, troubleshooting, and analysis purposes.
npm install @sourceloop/audit-log
In order to use this component into your LoopBack application, please follow below steps.
import {Entity, model, property} from '@loopback/repository';
import {Action} from '@sourceloop/audit-log';
@model({
name: 'audit_logs',
settings: {
strict: false,
},
})
export class AuditLog extends Entity {
@property({
type: 'string',
id: true,
generated: false,
})
id?: string;
@property({
type: 'string',
required: true,
})
action: Action;
@property({
name: 'acted_at',
type: 'date',
required: true,
})
actedAt: Date;
@property({
name: 'acted_on',
type: 'string',
})
actedOn?: string;
@property({
name: 'action_key',
type: 'string',
required: true,
})
actionKey: string;
@property({
name: 'entity_id',
type: 'string',
required: true,
})
entityId: string;
@property({
type: 'string',
required: true,
})
actor: string;
@property({
type: 'object',
})
before?: object;
@property({
type: 'object',
})
after?: object;
// Define well-known properties here
// Indexer property to allow additional data
// eslint-disable-next-line @typescript-eslint/no-explicit-any
[prop: string]: any;
constructor(data?: Partial<AuditLog>) {
super(data);
}
}
lb4 datasource
command create your own datasource using your preferred connector. Here is an example of datasource using postgres connector. Notice the statement static dataSourceName = AuditDbSourceName;
. Make sure you change the data source name as per this in order to ensure connection work from extension.import {inject, lifeCycleObserver, LifeCycleObserver} from '@loopback/core';
import {juggler} from '@loopback/repository';
import {AuditDbSourceName} from '@sourceloop/audit-log';
const config = {
name: 'audit',
connector: 'postgresql',
url: '',
host: '',
port: 0,
user: '',
password: '',
database: '',
};
@lifeCycleObserver('datasource')
export class AuditDataSource
extends juggler.DataSource
implements LifeCycleObserver
{
static dataSourceName = AuditDbSourceName;
static readonly defaultConfig = config;
constructor(
@inject('datasources.config.audit', {optional: true})
dsConfig: object = config,
) {
super(dsConfig);
}
}
lb4 repository
command, create a repository file. After that, change the inject paramater as below so as to refer to correct data source name.
@inject(
datasources.${AuditDbSourceName}) dataSource: AuditDataSource,
One example below.
import {inject} from '@loopback/core';
import {DefaultCrudRepository} from '@loopback/repository';
import {AuditDbSourceName} from '@sourceloop/audit-log';
import {AuditDataSource} from '../datasources';
import {AuditLog} from '../models';
export class AuditLogRepository extends DefaultCrudRepository<
AuditLog,
typeof AuditLog.prototype.id
> {
constructor(
@inject(`datasources.${AuditDbSourceName}`) dataSource: AuditDataSource,
) {
super(AuditLog, dataSource);
}
}
AuditRepositoryMixin
, for all those repositories where you need audit data. See an example below. For a model Group
, here we are extending the GroupRepository
with AuditRepositoryMixin
.import {repository} from '@loopback/repository';
import {Group, GroupRelations} from '../models';
import {PgdbDataSource} from '../datasources';
import {inject, Getter, Constructor} from '@loopback/core';
import {AuthenticationBindings, IAuthUser} from 'loopback4-authentication';
import {AuditRepositoryMixin} from '@sourceloop/audit-log';
import {AuditLogRepository} from './audit-log.repository';
const groupAuditOpts: IAuditMixinOptions = {
actionKey: 'Group_Logs',
};
export class GroupRepository extends AuditRepositoryMixin<
Group,
typeof Group.prototype.id,
GroupRelations,
string,
Constructor<
DefaultCrudRepository<Group, typeof Group.prototype.id, GroupRelations>
>
>(DefaultCrudRepository, groupAuditOpts) {
constructor(
@inject('datasources.pgdb') dataSource: PgdbDataSource,
@inject.getter(AuthenticationBindings.CURRENT_USER)
public getCurrentUser: Getter<IAuthUser>,
@repository.getter('AuditLogRepository')
public getAuditLogRepository: Getter<AuditLogRepository>,
) {
super(Group, dataSource, getCurrentUser);
}
}
You can pass any extra attributes to save into audit table using the IAuditMixinOptions
parameter of mixin function.
Make sure you provide getCurrentUser
and getAuditLogRepository
Getter functions in constructor.
This will create all insert, update, delete audits for this model.
noAudit:true
flag with optionscreate(data, {noAudit: true});
export interface User<ID = string, TID = string, UTID = string> {
id?: string;
username: string;
password?: string;
identifier?: ID;
permissions: string[];
authClientId: number;
email?: string;
role: string;
firstName: string;
lastName: string;
middleName?: string;
tenantId?: TID;
userTenantId?: UTID;
passwordExpiryTime?: Date;
allowedResources?: string[];
}
this.bind(AuthServiceBindings.ActorIdKey).to('username');
@inject(AuditBindings.ActorIdKey, {optional: true})
public actorIdKey?: ActorId,
ConditionalAuditRepositoryMixin
, for all those repositories where you need audit data based on condition whether ADD_AUDIT_LOG_MIXIN
is set true. See an example below. For a model Group
, here we are extending the GroupRepository
with AuditRepositoryMixin
.import {repository} from '@loopback/repository';
import {Group, GroupRelations} from '../models';
import {PgdbDataSource} from '../datasources';
import {inject, Getter, Constructor} from '@loopback/core';
import {AuthenticationBindings, IAuthUser} from 'loopback4-authentication';
import {ConditionalAuditRepositoryMixin} from '@sourceloop/audit-log';
import {AuditLogRepository} from './audit-log.repository';
const groupAuditOpts: IAuditMixinOptions = {
actionKey: 'Group_Logs',
};
export class GroupRepository extends ConditionalAuditRepositoryMixin(
DefaultUserModifyCrudRepository<
Group,
typeof Group.prototype.id,
GroupRelations
>,
groupAuditOpts,
) {
constructor(
@inject('datasources.pgdb') dataSource: PgdbDataSource,
@inject.getter(AuthenticationBindings.CURRENT_USER)
public getCurrentUser: Getter<IAuthUser>,
@repository.getter('AuditLogRepository')
public getAuditLogRepository: Getter<AuditLogRepository>,
) {
super(Group, dataSource, getCurrentUser);
}
}
Incase you dont have current user binded in your application context and wish to log the activities within your application then in that case you can pass the actor id along with the options just like
await productRepo.create(product, {noAudit: false, actorId: 'userId'});
This extension provides support to both juggler (the default loopback ORM) and sequelize.
If your loopback project is already using SequelizeCrudRepository
from @loopback/sequelize or equivalent add on repositories from sourceloop packages like SequelizeUserModifyCrudRepository
. You'll need to make just two changes:
/sequelize
, like below:import {
AuditRepositoryMixin,
AuditLogRepository,
} from '@sourceloop/audit-log/sequelize';
SequelizeDataSource
.import {SequelizeDataSource} from '@loopback/sequelize';
export class AuditDataSource
extends SequelizeDataSource
implements LifeCycleObserver
{
static dataSourceName = AuditDbSourceName;
static readonly defaultConfig = config;
constructor(
@inject('datasources.config.audit', {optional: true})
dsConfig: object = config,
) {
super(dsConfig);
}
}
If you've noticed a bug or have a question or have a feature request, search the issue tracker to see if someone else in the community has already created a ticket. If not, go ahead and make one! All feature requests are welcome. Implementation time may vary. Feel free to contribute the same, if you can. If you think this extension is useful, please star it. Appreciation really helps in keeping this project alive.
Please read CONTRIBUTING.md for details on the process for submitting pull requests to us.
Code of conduct guidelines here.
Release v8.0.2 June 4, 2024
Welcome to the June 4, 2024 release of loopback4-audit-log. There are many updates in this version that we hope you will like, the key highlights include:
loopback version updates :- chore(deps): loopback version updates was commited on June 4, 2024 by Surbhi
loopback version updates
GH-122
Clink on the above links to understand the changes in detail.
FAQs
Audit Log extension.
The npm package @sourceloop/audit-log receives a total of 899 weekly downloads. As such, @sourceloop/audit-log popularity was classified as not popular.
We found that @sourceloop/audit-log demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 9 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
MITRE's 2024 CWE Top 25 highlights critical software vulnerabilities like XSS, SQL Injection, and CSRF, reflecting shifts due to a refined ranking methodology.
Security News
In this segment of the Risky Business podcast, Feross Aboukhadijeh and Patrick Gray discuss the challenges of tracking malware discovered in open source softare.
Research
Security News
A threat actor's playbook for exploiting the npm ecosystem was exposed on the dark web, detailing how to build a blockchain-powered botnet.