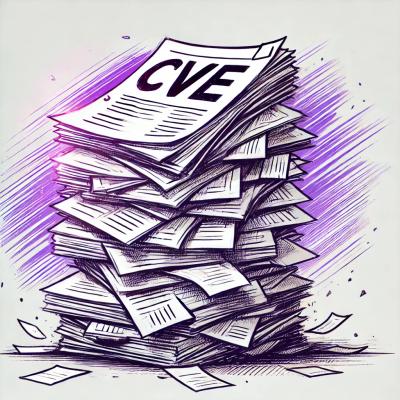
Security News
Node.js EOL Versions CVE Dubbed the "Worst CVE of the Year" by Security Experts
Critics call the Node.js EOL CVE a misuse of the system, sparking debate over CVE standards and the growing noise in vulnerability databases.
@sparticuz/pdffiller
Advanced tools
Take an existing PDF Form and data and PDF Filler will create a new PDF with all given fields populated.
This is a fork of the pdf-filler package, modified to return promises and readable streams, by piping data in/out of a spawned pdftk process instead of temporarily writing files to disk.
The goal is cleaner integration, in eg. a microservices context, where it is preferable not to write multiple temporary files to disk and where you may wish to stream the generated pdf directly to a service like AWS.
A node.js PDF form field data filler and FDF generator toolkit. This essentially is a wrapper around the PDF Toolkit library PDF ToolKit.
As of version 4.0.0, this library now targets pdftk-java, a modern fork of pdftk.
You must first have pdftk
(from pdftk-java, found here) installed correctly on your platform.
Then, install this library:
npm install @sparticuz/pdffiller --save
Note for AWS Lambda users, you may use a pdftk layer, found here
import fillForm from "@sparticuz/pdffiller";
const sourcePDF = "test/test.pdf";
const data = {
last_name: "John",
first_name: "Doe",
date: "Jan 1, 2013",
football: "Off",
baseball: "Yes",
basketball: "Off",
hockey: "Yes",
nascar: "Off",
};
const output = await fillForm(sourcePDF, data);
// output will be instance of stream.Readable
This will take the test.pdf, fill the fields with the data values and stream a filled in, read-only PDF.
A chainable convenience method toFile
is attached to the response, if you simply wish to write the stream to a file with no fuss:
fillForm(sourcePDF, data)
.toFile("outputFile.PDF")
.then(() => {
// your file has been written
})
.catch((err) => {
console.log(err);
});
You could also stream the resulting data directly to AWS, doing something like this with an instantiated s3
client:
fillForm(sourcePDF, data)
.then((outputStream) => {
const Body = outputStream;
const Bucket = "some-bucket";
const Key = "myFancyNewFilledPDF";
const ContentType = "application/pdf";
const uploader = new AWS.S3.ManagedUpload({
params: { Bucket, Key, Body, ContentType },
service: s3,
});
uploader.promise().then((data) => {
/* do something with AWS response */
});
})
.catch((err) => {
console.log(err);
});
Calling fillForm()
with shouldFlatten = false
will leave any unmapped fields still editable, as per the pdftk
command specification.
const shouldFlatten = false;
fillForm(sourcePDF, data, shouldFlatten)
.then((outputStream) {
// etc, same as above
})
import { generateFDFTemplate } from "@sparticuz/pdffiller";
const sourcePDF = "test/test.pdf";
const FDF_data = generateFDFTemplate(sourcePDF)
.then((fdfData) => {
console.log(fdfData);
})
.catch((err) => {
console.log(err);
});
This will print out this
{
"last_name": "",
"first_name": "",
"date": "",
"football": "",
"baseball": "",
"basketball": "",
"hockey": "",
"nascar": ""
}
import { mapForm2PDF } from "@sparticuz/pdffiller";
const conversionMap = {
lastName: "last_name",
firstName: "first_name",
Date: "date",
footballField: "football",
baseballField: "baseball",
bballField: "basketball",
hockeyField: "hockey",
nascarField: "nascar",
};
const FormFields = {
lastName: "John",
firstName: "Doe",
Date: "Jan 1, 2013",
footballField: "Off",
baseballField: "Yes",
bballField: "Off",
hockeyField: "Yes",
nascarField: "Off",
};
mapForm2PDF(data, convMap).then((mappedFields) => {
console.log(mappedFields);
});
This will print out the object below.
{
"last_name": "John",
"first_name": "Doe",
"date": "Jan 1, 2013",
"football": "Off",
"baseball": "Yes",
"basketball": "Off",
"hockey": "Yes",
"nascar": "Off"
}
import { convFieldJson2FDF } from '@sparticuz/pdffiller';
const fieldJson = [
{
"title" : "last_name",
"fieldfieldType": "Text",
"fieldValue": "Doe"
},
{
"title" : "first_name",
"fieldfieldType": "Text",
"fieldValue": "John"
},
{
"title" : "date",
"fieldType": "Text",
"fieldValue": "Jan 1, 2013"
},
{
"title" : "football",
"fieldType": "Button",
"fieldValue": false
},
{
"title" : "baseball",
"fieldType": "Button",
"fieldValue": true
},
{
"title" : "basketball",
"fieldType": "Button"
"fieldValue": false
},
{
"title" : "hockey",
"fieldType": "Button"
"fieldValue": true
},
{
"title" : "nascar",
"fieldType": "Button"
"fieldValue": false
}
];
const FDFData = convFieldJson2FDF(data);
console.log(FDFData)
This will print out:
{
"last_name" : "John",
"first_name" : "Doe",
"date" : "Jan 1, 2013",
"football" : "Off",
"baseball" : "Yes",
"basketball" : "Off",
"hockey" : "Yes",
"nascar" : "Off"
};
FAQs
Take an existing PDF Form and data and PDF Filler will create a new PDF with all given fields populated.
The npm package @sparticuz/pdffiller receives a total of 44 weekly downloads. As such, @sparticuz/pdffiller popularity was classified as not popular.
We found that @sparticuz/pdffiller demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Critics call the Node.js EOL CVE a misuse of the system, sparking debate over CVE standards and the growing noise in vulnerability databases.
Security News
cURL and Go security teams are publicly rejecting CVSS as flawed for assessing vulnerabilities and are calling for more accurate, context-aware approaches.
Security News
Bun 1.2 enhances its JavaScript runtime with 90% Node.js compatibility, built-in S3 and Postgres support, HTML Imports, and faster, cloud-first performance.