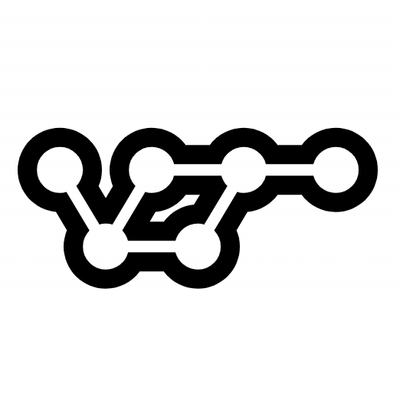
Security News
vlt Launches "reproduce": A New Tool Challenging the Limits of Package Provenance
vlt's new "reproduce" tool verifies npm packages against their source code, outperforming traditional provenance adoption in the JavaScript ecosystem.
@stadline/react-mtcaptcha
Advanced tools
It is an unofficial library to use [MTCaptcha](https://www.mtcaptcha.com/) on a React application.
It is an unofficial library to use MTCaptcha on a React application.
As early as possible, call the initialize function.
// src/index.js
import React, { useEffect } from 'react';
import { initialize } from '@stadline/react-mtcaptcha';
function App(props) {
useEffect(() => {
initialize({
sitekey: 'MTPublic-oy2GGOZq8',
theme: 'neowhite',
lang: 'fr',
});
}, []);
return <Router />; // or whatever
}
You can use any config option, for example custom styles, except the callbacks and render modes which are handled by the lib.
Then you can use the Captcha
component in your forms
return <Captcha onVerified={state => alert(state.verifiedToken)} />;
A more detailed example:
// src/components/ContactForm.js
import React, { useState } from 'react';
import Captcha from '@stadline/react-mtcaptcha';
function ContactForm(props) {
const [formValue, setFormValue] = useState({
message: '',
verifiedToken: null,
});
const setFieldValue = (key, value) => setFormValue(fv => ({ ...fv, [key]: value }));
const onSubmit = event => {
event.preventDefault();
alert('submit');
};
return (
<form onSubmit={onSubmit}>
<label>
<div>Text:</div>
<textarea
value={formValue.message}
onChange={event => setFieldValue('message', event.target.value)}
/>
</label>
<Captcha onVerified={state => setFieldValue('verifiedToken', state.verifiedToken)} />
<button type="submit" disabled={formValue.message.length < 1 || !formValue.verifiedToken}>
Submit
</button>
</form>
);
}
FAQs
It is an unofficial library to use [MTCaptcha](https://www.mtcaptcha.com/) on a React application.
We found that @stadline/react-mtcaptcha demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
vlt's new "reproduce" tool verifies npm packages against their source code, outperforming traditional provenance adoption in the JavaScript ecosystem.
Research
Security News
Socket researchers uncovered a malicious PyPI package exploiting Deezer’s API to enable coordinated music piracy through API abuse and C2 server control.
Research
The Socket Research Team discovered a malicious npm package, '@ton-wallet/create', stealing cryptocurrency wallet keys from developers and users in the TON ecosystem.