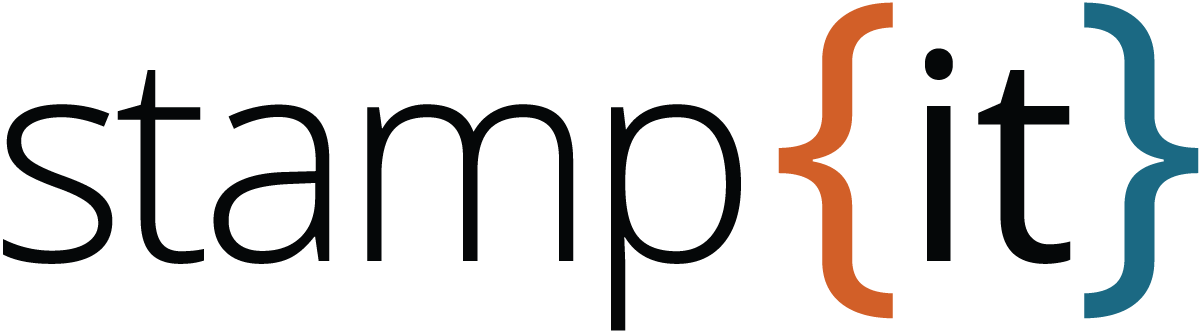
# @stamp/it
Utility belt implementation of the compose standard
This module is the new cool modern version of the stampit
module. Consider @stamp/it
as stampit v4.
Usage
Install:
$ npm i -S @stamp/it
Import:
import stampit from '@stamp/it'
Or import utility functions only:
import {
methods,
props,
properties,
statics,
staticProperties,
conf,
configuration,
deepProps,
deepProperties,
deepStatics,
staticDeepProperties,
deepConf,
deepConfiguration,
init,
initializers,
composers,
propertyDescriptors,
staticPropertyDescriptors
} from '@stamp/it'
const Stamp1 = methods({ foo() {} })
const Stamp2 = props({ bar: 'my bar' })
const Stamp3 = conf({ my: 'configuration' })
const Stamp4 = init(function (options, {stamp, instance, args}) {
console.log('bla')
})
API
Arguments
See more examples in this blog post.
Create a new empty stamp:
const S1 = stampit()
Create a new stamp with a method:
const S2 = stampit({
methods: {
myMethod() { }
}
})
Create a new stamp with a property:
const S3 = stampit({
props: {
myProperty: 42
}
})
Create a new stamp with a static property:
const S4 = stampit({
statics: {
myStaticProperty: 42
}
})
Create a new stamp with initializer(s):
const S5 = stampit({
init: function () { console.log('hi form initializer') }
})
const S6 = stampit({
init: [
function () { },
function () { }
]
})
Create a new stamp with a configuration:
const S7 = stampit({
conf: {
myConfiguration: { anything: 'here' }
}
})
Compose the stamps above together:
const Stamp = compose(S1, S2, S3, S4, S5, S6, S7)
console.log(Stamp)
const obj = Stamp()
console.log(obj)
console.log(Object.getPrototypeOf(obj))
See more examples in this blog post.
Static methods
The Stamp.create() static method
Calling Stamp.create()
is identical to calling Stamp()
.
Shortcut static methods
All stamps receive few static properties.
These are taken from the @stamp/shortcut
stamp.
For example:
stampit()
.props({ foo: 'foo' })
.deepConf({ things: ['bar'] })
.methods({ baz() {} })
Shortcut exported methods
The module exports a range of shortcut methods.
These are taken from the @stamp/shortcut
stamp.
import {methods, props, init, statics, } from '@stamp/it'
Each returns a stampit-flavoured stamp.
NOTE! Unlike the @stamp/shortcut
module, all the exported functions of @stamp/it
are stampit-flavoured. Meaning that:
import {methods} from '@stamp/it'
const Stamp = methods({ foo() {} })
.props({bar: 1})
.statics({baz: 2})