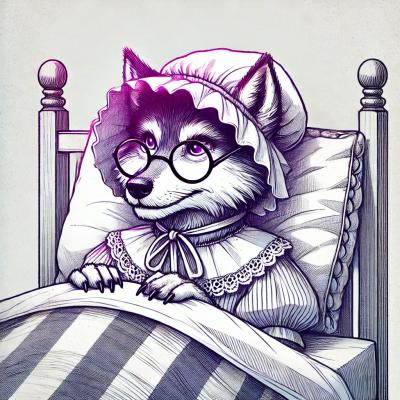
Security News
GitHub Removes Malicious Pull Requests Targeting Open Source Repositories
GitHub removed 27 malicious pull requests attempting to inject harmful code across multiple open source repositories, in another round of low-effort attacks.
@stdlib/complex-float64
Advanced tools
@stdlib/complex-float64 is a package for creating and manipulating double-precision complex numbers in JavaScript. It provides a variety of utilities for complex number arithmetic, including creation, basic operations, and conversions.
Creating a Complex Number
This feature allows you to create a new double-precision complex number. The example creates a complex number with a real part of 5.0 and an imaginary part of 3.0.
const Complex64 = require('@stdlib/complex-float64');
const z = new Complex64(5.0, 3.0);
console.log(z);
Basic Arithmetic Operations
This feature allows you to perform basic arithmetic operations on complex numbers. The example demonstrates how to add two complex numbers.
const Complex64 = require('@stdlib/complex-float64');
const z1 = new Complex64(5.0, 3.0);
const z2 = new Complex64(2.0, 1.0);
const sum = z1.add(z2);
console.log(sum);
Converting to String
This feature allows you to convert a complex number to a string representation. The example converts a complex number to its string form.
const Complex64 = require('@stdlib/complex-float64');
const z = new Complex64(5.0, 3.0);
console.log(z.toString());
complex.js is a library for complex number arithmetic in JavaScript. It provides a comprehensive set of functions for complex number operations, including addition, subtraction, multiplication, division, and more. Compared to @stdlib/complex-float64, complex.js offers a more extensive set of mathematical functions and utilities for complex numbers.
mathjs is an extensive mathematics library for JavaScript and Node.js. It includes support for complex numbers, matrices, units, and more. The complex number functionality in mathjs is part of a larger suite of mathematical tools, making it a more versatile but also more heavyweight option compared to @stdlib/complex-float64.
We believe in a future in which the web is a preferred environment for numerical computation. To help realize this future, we've built stdlib. stdlib is a standard library, with an emphasis on numerical and scientific computation, written in JavaScript (and C) for execution in browsers and in Node.js.
The library is fully decomposable, being architected in such a way that you can swap out and mix and match APIs and functionality to cater to your exact preferences and use cases.
When you use stdlib, you can be absolutely certain that you are using the most thorough, rigorous, well-written, studied, documented, tested, measured, and high-quality code out there.
To join us in bringing numerical computing to the web, get started by checking us out on GitHub, and please consider financially supporting stdlib. We greatly appreciate your continued support!
128-bit complex number.
npm install @stdlib/complex-float64
var Complex128 = require( '@stdlib/complex-float64' );
128-bit complex number constructor, where real
and imag
are the real and imaginary components, respectively.
var z = new Complex128( 5.0, 3.0 );
// returns <Complex128>
Size (in bytes) of each component.
var nbytes = Complex128.BYTES_PER_ELEMENT;
// returns 8
Size (in bytes) of each component.
var z = new Complex128( 5.0, 3.0 );
var nbytes = z.BYTES_PER_ELEMENT;
// returns 8
Length (in bytes) of a complex number.
var z = new Complex128( 5.0, 3.0 );
var nbytes = z.byteLength;
// returns 16
A Complex128
instance has the following properties...
A read-only property returning the real component.
var z = new Complex128( 5.0, 3.0 );
var re = z.re;
// returns 5.0
A read-only property returning the imaginary component.
var z = new Complex128( 5.0, -3.0 );
var im = z.im;
// returns -3.0
These methods do not mutate a Complex128
instance and, instead, return a complex number representation.
Returns a string
representation of a Complex128
instance.
var z = new Complex128( 5.0, 3.0 );
var str = z.toString();
// returns '5 + 3i'
z = new Complex128( -5.0, -3.0 );
str = z.toString();
// returns '-5 - 3i'
Returns a JSON representation of a Complex128
instance. JSON.stringify()
implicitly calls this method when stringifying a Complex128
instance.
var z = new Complex128( 5.0, -3.0 );
var o = z.toJSON();
/*
{
"type": "Complex128",
"re": 5.0,
"im": -3.0
}
*/
To revive a Complex128
number from a JSON string
, see @stdlib/complex/reviver-float64.
var Complex128 = require( '@stdlib/complex-float64' );
var z = new Complex128( 3.0, -2.0 );
console.log( 'type: %s', typeof z );
// => 'type: object'
console.log( 'str: %s', z );
// => 'str: 3 - 2i'
console.log( 'real: %d', z.re );
// => 'real: 3'
console.log( 'imag: %d', z.im );
// => 'imag: -2'
console.log( 'JSON: %s', JSON.stringify( z ) );
// => 'JSON: {"type":"Complex128","re":3,"im":-2}'
#include "stdlib/complex/float64.h"
An opaque type definition for a double-precision complex floating-point number.
stdlib_complex128_t z = stdlib_complex128( 5.0, 2.0 );
An opaque type definition for a union for accessing the real and imaginary parts of a double-precision complex floating-point number.
double real( const stdlib_complex128_t z ) {
stdlib_complex128_parts_t v;
// Assign a double-precision complex floating-point number:
v.value = z;
// Extract the real component:
double re = v.parts[ 0 ];
return re;
}
// ...
// Create a complex number:
stdlib_complex128_t z = stdlib_complex128( 5.0, 2.0 );
// ...
// Access the real component:
double re = real( z );
// returns 5.0
The union has the following members:
value: stdlib_complex128_t
double-precision complex floating-point number.
parts: double[]
array having the following elements:
double
real component.double
imaginary component.Returns a double-precision complex floating-point number.
stdlib_complex128_t z = stdlib_complex128( 5.0, 2.0 );
The function accepts the following arguments:
[in] double
real component.[in] double
imaginary component.stdlib_complex128_t stdlib_complex128( const double real, const double imag );
Converts a single-precision floating-point number to a double-precision complex floating-point number.
stdlib_complex128_t z = stdlib_complex128_from_float32( 5.0f );
The function accepts the following arguments:
[in] float
real component.stdlib_complex128_t stdlib_complex128_from_float32( const float real );
Converts a double-precision floating-point number to a double-precision complex floating-point number.
stdlib_complex128_t z = stdlib_complex128_from_float64( 5.0 );
The function accepts the following arguments:
[in] double
real component.stdlib_complex128_t stdlib_complex128_from_float64( const double real );
Converts a single-precision complex floating-point number to a double-precision complex floating-point number.
#include "stdlib/complex/float32.h"
stdlib_complex64_t z1 = stdlib_complex64( 5.0f, 3.0f );
stdlib_complex128_t z2 = stdlib_complex128_from_complex64( z1 );
The function accepts the following arguments:
[in] stdlib_complex64_t
single-precision complex floating-point number.stdlib_complex128_t stdlib_complex128_from_complex64( const stdlib_complex64_t z );
Converts (copies) a double-precision complex floating-point number to a double-precision complex floating-point number.
stdlib_complex128_t z1 = stdlib_complex128( 5.0, 3.0 );
stdlib_complex128_t z2 = stdlib_complex128_from_complex128( z1 );
The function accepts the following arguments:
[in] stdlib_complex128_t
double-precision complex floating-point number.stdlib_complex128_t stdlib_complex128_from_complex128( const stdlib_complex128_t z );
Converts a signed 8-bit integer to a double-precision complex floating-point number.
stdlib_complex128_t z = stdlib_complex128_from_int8( 5 );
The function accepts the following arguments:
[in] int8_t
real component.stdlib_complex128_t stdlib_complex128_from_int8( const int8_t real );
Converts an unsigned 8-bit integer to a double-precision complex floating-point number.
stdlib_complex128_t z = stdlib_complex128_from_uint8( 5 );
The function accepts the following arguments:
[in] uint8_t
real component.stdlib_complex128_t stdlib_complex128_from_uint8( const uint8_t real );
Converts a signed 16-bit integer to a double-precision complex floating-point number.
stdlib_complex128_t z = stdlib_complex128_from_int16( 5 );
The function accepts the following arguments:
[in] int16_t
real component.stdlib_complex128_t stdlib_complex128_from_int16( const int16_t real );
Converts an unsigned 16-bit integer to a double-precision complex floating-point number.
stdlib_complex128_t z = stdlib_complex128_from_uint16( 5 );
The function accepts the following arguments:
[in] uint16_t
real component.stdlib_complex128_t stdlib_complex128_from_uint16( const uint16_t real );
Converts a signed 32-bit integer to a double-precision complex floating-point number.
stdlib_complex128_t z = stdlib_complex128_from_int32( 5 );
The function accepts the following arguments:
[in] int32_t
real component.stdlib_complex128_t stdlib_complex128_from_int32( const int32_t real );
Converts an unsigned 32-bit integer to a double-precision complex floating-point number.
stdlib_complex128_t z = stdlib_complex128_from_uint32( 5 );
The function accepts the following arguments:
[in] uint32_t
real component.stdlib_complex128_t stdlib_complex128_from_uint32( const uint32_t real );
Converts a double-precision complex floating-point number to a single-precision complex floating-point number.
#include "stdlib/complex/float32.h"
stdlib_complex128_t z1 = stdlib_complex128( 5.0, 3.0 );
stdlib_complex64_t z2 = stdlib_complex128_to_complex64( z1 );
The function accepts the following arguments:
[in] stdlib_complex64_t
double-precision complex floating-point number.stdlib_complex64_t stdlib_complex128_to_complex64( const stdlib_complex128_t z );
#include "stdlib/complex/float64.h"
#include <stdint.h>
#include <stdio.h>
/**
* Return the real component of a double-precision complex floating-point number.
*
* @param z complex number
* @return real component
*/
static double real( const stdlib_complex128_t z ) {
stdlib_complex128_parts_t v;
// Assign a double-precision complex floating-point number:
v.value = z;
// Extract the real component:
double re = v.parts[ 0 ];
return re;
}
/**
* Return the imaginary component of a double-precision complex floating-point number.
*
* @param z complex number
* @return imaginary component
*/
static double imag( const stdlib_complex128_t z ) {
stdlib_complex128_parts_t v;
// Assign a double-precision complex floating-point number:
v.value = z;
// Extract the imaginary component:
double im = v.parts[ 1 ];
return im;
}
int main( void ) {
const stdlib_complex128_t x[] = {
stdlib_complex128( 5.0, 2.0 ),
stdlib_complex128( -2.0, 1.0 ),
stdlib_complex128( 0.0, -0.0 ),
stdlib_complex128( 0.0/0.0, 0.0/0.0 )
};
stdlib_complex128_t v;
int i;
for ( i = 0; i < 4; i++ ) {
v = x[ i ];
printf( "%lf + %lfi\n", real( v ), imag( v ) );
}
}
@stdlib/complex-cmplx
: create a complex number.@stdlib/complex-float32
: 64-bit complex number.This package is part of stdlib, a standard library for JavaScript and Node.js, with an emphasis on numerical and scientific computing. The library provides a collection of robust, high performance libraries for mathematics, statistics, streams, utilities, and more.
For more information on the project, filing bug reports and feature requests, and guidance on how to develop stdlib, see the main project repository.
See LICENSE.
Copyright © 2016-2023. The Stdlib Authors.
0.1.1 (2023-10-09)
No changes reported for this release.
</section> <!-- /.release --> <section class="release" id="v0.1.0">FAQs
Double-precision complex number functions.
We found that @stdlib/complex-float64 demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
GitHub removed 27 malicious pull requests attempting to inject harmful code across multiple open source repositories, in another round of low-effort attacks.
Security News
RubyGems.org has added a new "maintainer" role that allows for publishing new versions of gems. This new permission type is aimed at improving security for gem owners and the service overall.
Security News
Node.js will be enforcing stricter semver-major PR policies a month before major releases to enhance stability and ensure reliable release candidates.