What is @storybook/addon-interactions?
The @storybook/addon-interactions package is an addon for Storybook that allows developers to test and debug user interactions and play functions within their stories. It provides tools to simulate user events, debug play functions, and track the state of interactions over time.
What are @storybook/addon-interactions's main functionalities?
Simulating user interactions
This feature allows developers to simulate user events such as clicks, typing, and more within their stories. The code sample demonstrates how to simulate a click event on a button with the text 'Click me' using the `userEvent` object from '@storybook/testing-library'.
import { userEvent, within } from '@storybook/testing-library';
export const MyStory = {...};
MyStory.play = async ({ canvasElement }) => {
const canvas = within(canvasElement);
await userEvent.click(canvas.getByRole('button', { name: /click me/i }));
};
Debugging play functions
This feature enables developers to debug their play functions by using assertions to test the results of interactions. The code sample shows how to use `expect` from '@storybook/jest' to assert that an `onClick` handler was called during the play function execution.
import { expect } from '@storybook/jest';
export const MyStory = {...};
MyStory.play = async ({ args }) => {
// Simulate interactions and check the results
expect(args.onClick).toHaveBeenCalled();
};
Tracking interaction state
This feature provides a way to track the state of interactions over time. Developers can use `useAddonState` from '@storybook/addons' to get and set the state within their play functions. The code sample illustrates how to set a state indicating that an element was clicked.
import { useAddonState } from '@storybook/addons';
export const MyStory = {...};
MyStory.play = async () => {
const [interactionsState, setInteractionsState] = useAddonState('interactions', {});
// Update the state based on interactions
setInteractionsState({ clicked: true });
};
Other packages similar to @storybook/addon-interactions
@storybook/addon-actions
The @storybook/addon-actions package allows developers to log actions as users interact with UI components in Storybook. It is similar to @storybook/addon-interactions in that it helps with testing user interactions, but it focuses more on logging the actions rather than simulating and debugging them.
@storybook/addon-controls
The @storybook/addon-controls package provides a way to dynamically edit props of your components in Storybook. While it does not directly deal with user interactions like @storybook/addon-interactions, it complements the interaction testing by allowing real-time tweaking of component props.
@storybook/testing-library
The @storybook/testing-library package offers utilities to test Storybook stories with the Testing Library. It is used in conjunction with @storybook/addon-interactions to simulate user events and assertions, but it can also be used independently for writing tests that mimic user behavior.
Storybook Addon Interactions
Storybook Addon Interactions enables visual debugging of interactions and tests in Storybook.
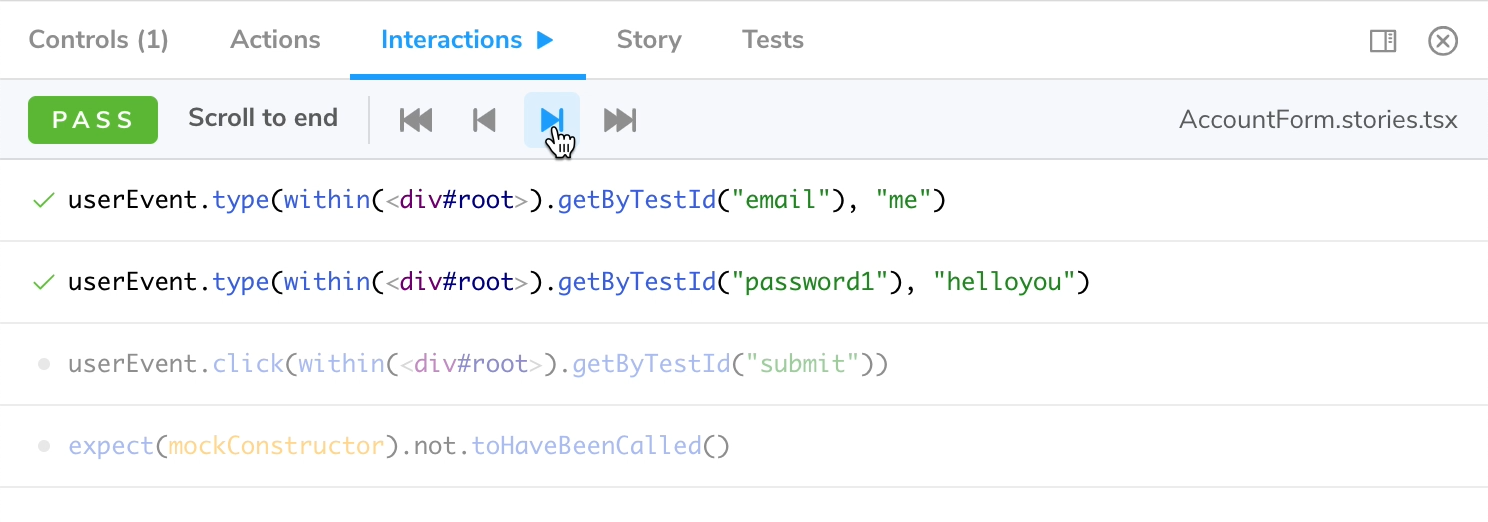
Installation
Install this addon by adding the @storybook/addon-interactions
dependency:
yarn add -D @storybook/addon-interactions @storybook/jest @storybook/testing-library
within .storybook/main.js
:
export default {
addons: ['@storybook/addon-interactions'],
};
Note that @storybook/addon-interactions
must be listed after @storybook/addon-actions
or @storybook/addon-essentials
.
Usage
Interactions relies on "instrumented" versions of Jest and Testing Library, that you import from @storybook/jest
and
@storybook/testing-library
instead of their original package. You can then use these libraries in your play
function.
import { Button } from './Button';
import { expect } from '@storybook/jest';
import { within, userEvent } from '@storybook/testing-library';
export default {
title: 'Button',
component: Button,
argTypes: {
onClick: { action: true },
},
};
const Template = (args) => <Button {...args} />;
export const Demo = Template.bind({});
Demo.play = async ({ args, canvasElement }) => {
const canvas = within(canvasElement);
await userEvent.click(canvas.getByRole('button'));
await expect(args.onClick).toHaveBeenCalled();
};
In order to enable step-through debugging, calls to userEvent.*
, fireEvent
, findBy*
, waitFor*
and expect
have to
be await
-ed. While debugging, these functions return a Promise that won't resolve until you continue to the next step.
While you can technically use screen
, it's recommended to use within(canvasElement)
. Besides giving you a better error
message when a DOM element can't be found, it will also ensure your play function is compatible with Storybook Docs.
Any args
that are marked as an action
(typically via argTypes
or argTypesRegex
) will be automatically wrapped in
a Jest mock function so you can assert invocations. See
addon-actions for how to setup actions.