@supabase/postgrest-js
Advanced tools
Comparing version 0.33.3 to 0.34.0
@@ -13,3 +13,4 @@ import { PostgrestBuilder } from './types'; | ||
*/ | ||
rpc(params?: object, { count, }?: { | ||
rpc(params?: object, { head, count, }?: { | ||
head?: boolean; | ||
count?: null | 'exact' | 'planned' | 'estimated'; | ||
@@ -16,0 +17,0 @@ }): PostgrestFilterBuilder<T>; |
@@ -18,5 +18,15 @@ "use strict"; | ||
*/ | ||
rpc(params, { count = null, } = {}) { | ||
this.method = 'POST'; | ||
this.body = params; | ||
rpc(params, { head = false, count = null, } = {}) { | ||
if (head) { | ||
this.method = 'HEAD'; | ||
if (params) { | ||
Object.entries(params).forEach(([name, value]) => { | ||
this.url.searchParams.append(name, value); | ||
}); | ||
} | ||
} | ||
else { | ||
this.method = 'POST'; | ||
this.body = params; | ||
} | ||
if (count) { | ||
@@ -23,0 +33,0 @@ if (this.headers['Prefer'] !== undefined) |
@@ -45,2 +45,6 @@ import { PostgrestBuilder, PostgrestMaybeSingleResponse, PostgrestSingleResponse } from './types'; | ||
/** | ||
* Sets the AbortSignal for the fetch request. | ||
*/ | ||
abortSignal(signal: AbortSignal): this; | ||
/** | ||
* Retrieves only one row from the result. Result must be one row (e.g. using | ||
@@ -47,0 +51,0 @@ * `limit`), otherwise this will result in an error. |
@@ -72,2 +72,9 @@ "use strict"; | ||
/** | ||
* Sets the AbortSignal for the fetch request. | ||
*/ | ||
abortSignal(signal) { | ||
this.signal = signal; | ||
return this; | ||
} | ||
/** | ||
* Retrieves only one row from the result. Result must be one row (e.g. using | ||
@@ -74,0 +81,0 @@ * `limit`), otherwise this will result in an error. |
@@ -49,3 +49,4 @@ /** | ||
protected body?: Partial<T> | Partial<T>[]; | ||
protected shouldThrowOnError?: boolean; | ||
protected shouldThrowOnError: boolean; | ||
protected signal?: AbortSignal; | ||
constructor(builder: PostgrestBuilder<T>); | ||
@@ -52,0 +53,0 @@ /** |
@@ -19,2 +19,3 @@ "use strict"; | ||
constructor(builder) { | ||
this.shouldThrowOnError = false; | ||
Object.assign(this, builder); | ||
@@ -46,8 +47,8 @@ } | ||
} | ||
return cross_fetch_1.default(this.url.toString(), { | ||
let res = cross_fetch_1.default(this.url.toString(), { | ||
method: this.method, | ||
headers: this.headers, | ||
body: JSON.stringify(this.body), | ||
}) | ||
.then((res) => __awaiter(this, void 0, void 0, function* () { | ||
signal: this.signal, | ||
}).then((res) => __awaiter(this, void 0, void 0, function* () { | ||
var _a, _b, _c; | ||
@@ -92,4 +93,19 @@ let error = null; | ||
return postgrestResponse; | ||
})) | ||
.then(onfulfilled, onrejected); | ||
})); | ||
if (!this.shouldThrowOnError) { | ||
res = res.catch((fetchError) => ({ | ||
error: { | ||
message: `FetchError: ${fetchError.message}`, | ||
details: '', | ||
hint: '', | ||
code: fetchError.code || '', | ||
}, | ||
data: null, | ||
body: null, | ||
count: null, | ||
status: 400, | ||
statusText: 'Bad Request', | ||
})); | ||
} | ||
return res.then(onfulfilled, onrejected); | ||
} | ||
@@ -96,0 +112,0 @@ } |
@@ -1,2 +0,2 @@ | ||
export declare const version = "0.33.3"; | ||
export declare const version = "0.34.0"; | ||
//# sourceMappingURL=version.d.ts.map |
@@ -5,3 +5,3 @@ "use strict"; | ||
// generated by genversion | ||
exports.version = '0.33.3'; | ||
exports.version = '0.34.0'; | ||
//# sourceMappingURL=version.js.map |
@@ -39,5 +39,7 @@ import PostgrestQueryBuilder from './lib/PostgrestQueryBuilder'; | ||
* @param params The parameters to pass to the function call. | ||
* @param head When set to true, no data will be returned. | ||
* @param count Count algorithm to use to count rows in a table. | ||
*/ | ||
rpc<T = any>(fn: string, params?: object, { count, }?: { | ||
rpc<T = any>(fn: string, params?: object, { head, count, }?: { | ||
head?: boolean; | ||
count?: null | 'exact' | 'planned' | 'estimated'; | ||
@@ -44,0 +46,0 @@ }): PostgrestFilterBuilder<T>; |
@@ -45,5 +45,6 @@ "use strict"; | ||
* @param params The parameters to pass to the function call. | ||
* @param head When set to true, no data will be returned. | ||
* @param count Count algorithm to use to count rows in a table. | ||
*/ | ||
rpc(fn, params, { count = null, } = {}) { | ||
rpc(fn, params, { head = false, count = null, } = {}) { | ||
const url = `${this.url}/rpc/${fn}`; | ||
@@ -53,3 +54,3 @@ return new PostgrestRpcBuilder_1.default(url, { | ||
schema: this.schema, | ||
}).rpc(params, { count }); | ||
}).rpc(params, { head, count }); | ||
} | ||
@@ -56,0 +57,0 @@ } |
@@ -13,3 +13,4 @@ import { PostgrestBuilder } from './types'; | ||
*/ | ||
rpc(params?: object, { count, }?: { | ||
rpc(params?: object, { head, count, }?: { | ||
head?: boolean; | ||
count?: null | 'exact' | 'planned' | 'estimated'; | ||
@@ -16,0 +17,0 @@ }): PostgrestFilterBuilder<T>; |
@@ -13,5 +13,15 @@ import { PostgrestBuilder } from './types'; | ||
*/ | ||
rpc(params, { count = null, } = {}) { | ||
this.method = 'POST'; | ||
this.body = params; | ||
rpc(params, { head = false, count = null, } = {}) { | ||
if (head) { | ||
this.method = 'HEAD'; | ||
if (params) { | ||
Object.entries(params).forEach(([name, value]) => { | ||
this.url.searchParams.append(name, value); | ||
}); | ||
} | ||
} | ||
else { | ||
this.method = 'POST'; | ||
this.body = params; | ||
} | ||
if (count) { | ||
@@ -18,0 +28,0 @@ if (this.headers['Prefer'] !== undefined) |
@@ -45,2 +45,6 @@ import { PostgrestBuilder, PostgrestMaybeSingleResponse, PostgrestSingleResponse } from './types'; | ||
/** | ||
* Sets the AbortSignal for the fetch request. | ||
*/ | ||
abortSignal(signal: AbortSignal): this; | ||
/** | ||
* Retrieves only one row from the result. Result must be one row (e.g. using | ||
@@ -47,0 +51,0 @@ * `limit`), otherwise this will result in an error. |
@@ -70,2 +70,9 @@ import { PostgrestBuilder } from './types'; | ||
/** | ||
* Sets the AbortSignal for the fetch request. | ||
*/ | ||
abortSignal(signal) { | ||
this.signal = signal; | ||
return this; | ||
} | ||
/** | ||
* Retrieves only one row from the result. Result must be one row (e.g. using | ||
@@ -72,0 +79,0 @@ * `limit`), otherwise this will result in an error. |
@@ -49,3 +49,4 @@ /** | ||
protected body?: Partial<T> | Partial<T>[]; | ||
protected shouldThrowOnError?: boolean; | ||
protected shouldThrowOnError: boolean; | ||
protected signal?: AbortSignal; | ||
constructor(builder: PostgrestBuilder<T>); | ||
@@ -52,0 +53,0 @@ /** |
@@ -13,2 +13,3 @@ var __awaiter = (this && this.__awaiter) || function (thisArg, _arguments, P, generator) { | ||
constructor(builder) { | ||
this.shouldThrowOnError = false; | ||
Object.assign(this, builder); | ||
@@ -40,8 +41,8 @@ } | ||
} | ||
return fetch(this.url.toString(), { | ||
let res = fetch(this.url.toString(), { | ||
method: this.method, | ||
headers: this.headers, | ||
body: JSON.stringify(this.body), | ||
}) | ||
.then((res) => __awaiter(this, void 0, void 0, function* () { | ||
signal: this.signal, | ||
}).then((res) => __awaiter(this, void 0, void 0, function* () { | ||
var _a, _b, _c; | ||
@@ -86,6 +87,21 @@ let error = null; | ||
return postgrestResponse; | ||
})) | ||
.then(onfulfilled, onrejected); | ||
})); | ||
if (!this.shouldThrowOnError) { | ||
res = res.catch((fetchError) => ({ | ||
error: { | ||
message: `FetchError: ${fetchError.message}`, | ||
details: '', | ||
hint: '', | ||
code: fetchError.code || '', | ||
}, | ||
data: null, | ||
body: null, | ||
count: null, | ||
status: 400, | ||
statusText: 'Bad Request', | ||
})); | ||
} | ||
return res.then(onfulfilled, onrejected); | ||
} | ||
} | ||
//# sourceMappingURL=types.js.map |
@@ -1,2 +0,2 @@ | ||
export declare const version = "0.33.3"; | ||
export declare const version = "0.34.0"; | ||
//# sourceMappingURL=version.d.ts.map |
// generated by genversion | ||
export const version = '0.33.3'; | ||
export const version = '0.34.0'; | ||
//# sourceMappingURL=version.js.map |
@@ -39,5 +39,7 @@ import PostgrestQueryBuilder from './lib/PostgrestQueryBuilder'; | ||
* @param params The parameters to pass to the function call. | ||
* @param head When set to true, no data will be returned. | ||
* @param count Count algorithm to use to count rows in a table. | ||
*/ | ||
rpc<T = any>(fn: string, params?: object, { count, }?: { | ||
rpc<T = any>(fn: string, params?: object, { head, count, }?: { | ||
head?: boolean; | ||
count?: null | 'exact' | 'planned' | 'estimated'; | ||
@@ -44,0 +46,0 @@ }): PostgrestFilterBuilder<T>; |
@@ -40,5 +40,6 @@ import PostgrestQueryBuilder from './lib/PostgrestQueryBuilder'; | ||
* @param params The parameters to pass to the function call. | ||
* @param head When set to true, no data will be returned. | ||
* @param count Count algorithm to use to count rows in a table. | ||
*/ | ||
rpc(fn, params, { count = null, } = {}) { | ||
rpc(fn, params, { head = false, count = null, } = {}) { | ||
const url = `${this.url}/rpc/${fn}`; | ||
@@ -48,5 +49,5 @@ return new PostgrestRpcBuilder(url, { | ||
schema: this.schema, | ||
}).rpc(params, { count }); | ||
}).rpc(params, { head, count }); | ||
} | ||
} | ||
//# sourceMappingURL=PostgrestClient.js.map |
{ | ||
"name": "@supabase/postgrest-js", | ||
"version": "0.33.3", | ||
"version": "0.34.0", | ||
"description": "Isomorphic PostgREST client", | ||
@@ -40,2 +40,3 @@ "keywords": [ | ||
"jest": "^26.4.1", | ||
"node-abort-controller": "^3.0.0", | ||
"npm-run-all": "^4.1.5", | ||
@@ -42,0 +43,0 @@ "prettier": "^2.0.5", |
@@ -1,9 +0,11 @@ | ||
# Postgrest JS | ||
# `postgrest-js` | ||
[](https://github.com/supabase/postgrest-js/actions?query=branch%3Amaster) | ||
[](https://www.npmjs.com/package/@supabase/postgrest-js) | ||
[](#license) | ||
Isomorphic JavaScript client for [PostgREST](https://postgrest.org). The goal of this library is to make an "ORM-like" restful interface. | ||
## Documentation | ||
Full documentation can be found [here](https://supabase.github.io/postgrest-js/). | ||
Full documentation can be found on our [website](https://supabase.io/docs/postgrest/client/postgrest-client). | ||
### Quick start | ||
@@ -26,17 +28,11 @@ | ||
- select(): https://supabase.io/docs/postgrest/client/select | ||
- insert(): https://supabase.io/docs/postgrest/client/insert | ||
- update(): https://supabase.io/docs/postgrest/client/update | ||
- delete(): https://supabase.io/docs/postgrest/client/delete | ||
- select(): https://supabase.io/docs/reference/javascript/select | ||
- insert(): https://supabase.io/docs/reference/javascript/insert | ||
- update(): https://supabase.io/docs/reference/javascript/update | ||
- delete(): https://supabase.io/docs/reference/javascript/delete | ||
## License | ||
This repo is liscenced under MIT. | ||
This repo is licensed under MIT License. | ||
## Credits | ||
- https://github.com/calebmer/postgrest-client - originally forked and adapted from @calebmer's library | ||
## Sponsors | ||
@@ -47,4 +43,1 @@ | ||
[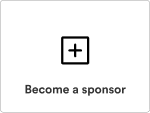](https://github.com/sponsors/supabase) | ||
 |
@@ -21,10 +21,22 @@ import { PostgrestBuilder } from './types' | ||
{ | ||
head = false, | ||
count = null, | ||
}: { | ||
head?: boolean | ||
count?: null | 'exact' | 'planned' | 'estimated' | ||
} = {} | ||
): PostgrestFilterBuilder<T> { | ||
this.method = 'POST' | ||
this.body = params | ||
if (head) { | ||
this.method = 'HEAD' | ||
if (params) { | ||
Object.entries(params).forEach(([name, value]) => { | ||
this.url.searchParams.append(name, value) | ||
}) | ||
} | ||
} else { | ||
this.method = 'POST' | ||
this.body = params | ||
} | ||
if (count) { | ||
@@ -31,0 +43,0 @@ if (this.headers['Prefer'] !== undefined) this.headers['Prefer'] += `,count=${count}` |
@@ -89,2 +89,10 @@ import { PostgrestBuilder, PostgrestMaybeSingleResponse, PostgrestSingleResponse } from './types' | ||
/** | ||
* Sets the AbortSignal for the fetch request. | ||
*/ | ||
abortSignal(signal: AbortSignal): this { | ||
this.signal = signal | ||
return this | ||
} | ||
/** | ||
* Retrieves only one row from the result. Result must be one row (e.g. using | ||
@@ -91,0 +99,0 @@ * `limit`), otherwise this will result in an error. |
@@ -57,3 +57,4 @@ import fetch from 'cross-fetch' | ||
protected body?: Partial<T> | Partial<T>[] | ||
protected shouldThrowOnError?: boolean | ||
protected shouldThrowOnError = false | ||
protected signal?: AbortSignal | ||
@@ -94,51 +95,67 @@ constructor(builder: PostgrestBuilder<T>) { | ||
return fetch(this.url.toString(), { | ||
let res = fetch(this.url.toString(), { | ||
method: this.method, | ||
headers: this.headers, | ||
body: JSON.stringify(this.body), | ||
}) | ||
.then(async (res) => { | ||
let error = null | ||
let data = null | ||
let count = null | ||
signal: this.signal, | ||
}).then(async (res) => { | ||
let error = null | ||
let data = null | ||
let count = null | ||
if (res.ok) { | ||
const isReturnMinimal = this.headers['Prefer']?.split(',').includes('return=minimal') | ||
if (this.method !== 'HEAD' && !isReturnMinimal) { | ||
const text = await res.text() | ||
if (!text) { | ||
// discard `text` | ||
} else if (this.headers['Accept'] === 'text/csv') { | ||
data = text | ||
} else { | ||
data = JSON.parse(text) | ||
} | ||
if (res.ok) { | ||
const isReturnMinimal = this.headers['Prefer']?.split(',').includes('return=minimal') | ||
if (this.method !== 'HEAD' && !isReturnMinimal) { | ||
const text = await res.text() | ||
if (!text) { | ||
// discard `text` | ||
} else if (this.headers['Accept'] === 'text/csv') { | ||
data = text | ||
} else { | ||
data = JSON.parse(text) | ||
} | ||
} | ||
const countHeader = this.headers['Prefer']?.match(/count=(exact|planned|estimated)/) | ||
const contentRange = res.headers.get('content-range')?.split('/') | ||
if (countHeader && contentRange && contentRange.length > 1) { | ||
count = parseInt(contentRange[1]) | ||
} | ||
} else { | ||
error = await res.json() | ||
if (error && this.shouldThrowOnError) { | ||
throw error | ||
} | ||
const countHeader = this.headers['Prefer']?.match(/count=(exact|planned|estimated)/) | ||
const contentRange = res.headers.get('content-range')?.split('/') | ||
if (countHeader && contentRange && contentRange.length > 1) { | ||
count = parseInt(contentRange[1]) | ||
} | ||
} else { | ||
error = await res.json() | ||
const postgrestResponse: PostgrestResponse<T> = { | ||
error, | ||
data, | ||
count, | ||
status: res.status, | ||
statusText: res.statusText, | ||
body: data, | ||
if (error && this.shouldThrowOnError) { | ||
throw error | ||
} | ||
} | ||
return postgrestResponse | ||
}) | ||
.then(onfulfilled, onrejected) | ||
const postgrestResponse = { | ||
error, | ||
data, | ||
count, | ||
status: res.status, | ||
statusText: res.statusText, | ||
body: data, | ||
} | ||
return postgrestResponse | ||
}) | ||
if (!this.shouldThrowOnError) { | ||
res = res.catch((fetchError) => ({ | ||
error: { | ||
message: `FetchError: ${fetchError.message}`, | ||
details: '', | ||
hint: '', | ||
code: fetchError.code || '', | ||
}, | ||
data: null, | ||
body: null, | ||
count: null, | ||
status: 400, | ||
statusText: 'Bad Request', | ||
})) | ||
} | ||
return res.then(onfulfilled, onrejected) | ||
} | ||
} |
// generated by genversion | ||
export const version = '0.33.3' | ||
export const version = '0.34.0' |
@@ -52,2 +52,3 @@ import PostgrestQueryBuilder from './lib/PostgrestQueryBuilder' | ||
* @param params The parameters to pass to the function call. | ||
* @param head When set to true, no data will be returned. | ||
* @param count Count algorithm to use to count rows in a table. | ||
@@ -59,4 +60,6 @@ */ | ||
{ | ||
head = false, | ||
count = null, | ||
}: { | ||
head?: boolean | ||
count?: null | 'exact' | 'planned' | 'estimated' | ||
@@ -69,4 +72,4 @@ } = {} | ||
schema: this.schema, | ||
}).rpc(params, { count }) | ||
}).rpc(params, { head, count }) | ||
} | ||
} |
Sorry, the diff of this file is not supported yet
Sorry, the diff of this file is not supported yet
Sorry, the diff of this file is not supported yet
Sorry, the diff of this file is not supported yet
Sorry, the diff of this file is not supported yet
Sorry, the diff of this file is not supported yet
Sorry, the diff of this file is not supported yet
Sorry, the diff of this file is not supported yet
Sorry, the diff of this file is not supported yet
Sorry, the diff of this file is not supported yet
Sorry, the diff of this file is not supported yet
Sorry, the diff of this file is not supported yet
Sorry, the diff of this file is not supported yet
Sorry, the diff of this file is not supported yet
Sorry, the diff of this file is not supported yet
Sorry, the diff of this file is not supported yet
License Policy Violation
LicenseThis package is not allowed per your license policy. Review the package's license to ensure compliance.
Found 1 instance in 1 package
License Policy Violation
LicenseThis package is not allowed per your license policy. Review the package's license to ensure compliance.
Found 1 instance in 1 package
204489
3773
13
42