What is @supabase/storage-js?
@supabase/storage-js is a JavaScript client library for interacting with Supabase Storage. It allows you to manage files and buckets, upload and download files, and handle permissions within the Supabase ecosystem.
What are @supabase/storage-js's main functionalities?
Create a Bucket
This feature allows you to create a new storage bucket in your Supabase project. The code sample demonstrates how to initialize the Supabase client and create a new bucket named 'my-new-bucket'.
const { createClient } = require('@supabase/supabase-js');
const supabase = createClient('https://your-project.supabase.co', 'public-anon-key');
async function createBucket() {
const { data, error } = await supabase.storage.createBucket('my-new-bucket');
if (error) console.error('Error creating bucket:', error);
else console.log('Bucket created:', data);
}
createBucket();
Upload a File
This feature allows you to upload a file to a specified bucket. The code sample demonstrates how to upload a text file named 'hello.txt' to the 'my-new-bucket' bucket.
const { createClient } = require('@supabase/supabase-js');
const supabase = createClient('https://your-project.supabase.co', 'public-anon-key');
async function uploadFile() {
const file = new File(['Hello World'], 'hello.txt', { type: 'text/plain' });
const { data, error } = await supabase.storage.from('my-new-bucket').upload('hello.txt', file);
if (error) console.error('Error uploading file:', error);
else console.log('File uploaded:', data);
}
uploadFile();
Download a File
This feature allows you to download a file from a specified bucket. The code sample demonstrates how to download a file named 'hello.txt' from the 'my-new-bucket' bucket.
const { createClient } = require('@supabase/supabase-js');
const supabase = createClient('https://your-project.supabase.co', 'public-anon-key');
async function downloadFile() {
const { data, error } = await supabase.storage.from('my-new-bucket').download('hello.txt');
if (error) console.error('Error downloading file:', error);
else console.log('File downloaded:', data);
}
downloadFile();
List Files in a Bucket
This feature allows you to list all files in a specified bucket. The code sample demonstrates how to list all files in the 'my-new-bucket' bucket.
const { createClient } = require('@supabase/supabase-js');
const supabase = createClient('https://your-project.supabase.co', 'public-anon-key');
async function listFiles() {
const { data, error } = await supabase.storage.from('my-new-bucket').list();
if (error) console.error('Error listing files:', error);
else console.log('Files in bucket:', data);
}
listFiles();
Delete a File
This feature allows you to delete a file from a specified bucket. The code sample demonstrates how to delete a file named 'hello.txt' from the 'my-new-bucket' bucket.
const { createClient } = require('@supabase/supabase-js');
const supabase = createClient('https://your-project.supabase.co', 'public-anon-key');
async function deleteFile() {
const { data, error } = await supabase.storage.from('my-new-bucket').remove(['hello.txt']);
if (error) console.error('Error deleting file:', error);
else console.log('File deleted:', data);
}
deleteFile();
Other packages similar to @supabase/storage-js
aws-sdk
The AWS SDK for JavaScript provides a comprehensive set of tools for interacting with AWS services, including S3 for storage. It offers more extensive features and integrations compared to @supabase/storage-js, but it can be more complex to set up and use.
firebase-admin
The Firebase Admin SDK allows you to manage your Firebase services programmatically. It includes functionality for Firebase Storage, which is similar to Supabase Storage. Firebase offers a broader range of services and integrations, but it may be overkill if you only need storage capabilities.
storage-js
JS Client library to interact with Supabase Storage.
Quick Start Guide
Installing the module
npm install @supabase/storage-js
Connecting to the storage backend
import { StorageClient } from '@supabase/storage-js'
const STORAGE_URL = 'https://<project_ref>.supabase.co/storage/v1'
const SERVICE_KEY = '<service_role>'
const storageClient = new StorageClient(STORAGE_URL, {
apikey: SERVICE_KEY,
Authorization: `Bearer ${SERVICE_KEY}`,
})
Handling resources
Handling Storage Buckets
-
Create a new Storage bucket:
const { data, error } = await storageClient.createBucket(
'test_bucket',
{ public: false }
)
-
Retrieve the details of an existing Storage bucket:
const { data, error } = await storageClient.getBucket('test_bucket')
-
Update a new Storage bucket:
const { data, error } = await storageClient.updateBucket(
'test_bucket',
{ public: false }
)
-
Remove all objects inside a single bucket:
const { data, error } = await storageClient.emptyBucket('test_bucket')
-
Delete an existing bucket (a bucket can't be deleted with existing objects inside it):
const { data, error } = await storageClient.deleteBucket('test_bucket')
-
Retrieve the details of all Storage buckets within an existing project:
const { data, error } = await storageClient.listBuckets()
Handling Files
-
Upload a file to an existing bucket:
const fileBody = ...
const { data, error } = await storageClient.from('bucket').upload('path/to/file', fileBody)
Note:
The path in data.Key
is prefixed by the bucket ID and is not the value which should be passed to the download
method in order to fetch the file.
To fetch the file via the download
method, use data.path
and data.bucketId
as follows:
const { data, error } = await storageClient.from('bucket').upload('/folder/file.txt', fileBody)
const { data2, error2 } = await storageClient.from(data.bucketId).download(data.path)
Note: The upload
method also accepts a map of optional parameters. For a complete list see the Supabase API reference.
-
Download a file from an exisiting bucket:
const { data, error } = await storageClient.from('bucket').download('path/to/file')
-
List all the files within a bucket:
const { data, error } = await storageClient.from('bucket').list('folder')
Note: The list
method also accepts a map of optional parameters. For a complete list see the Supabase API reference.
-
Replace an existing file at the specified path with a new one:
const fileBody = ...
const { data, error } = await storageClient
.from('bucket')
.update('path/to/file', fileBody)
Note: The upload
method also accepts a map of optional parameters. For a complete list see the Supabase API reference.
-
Move an existing file:
const { data, error } = await storageClient
.from('bucket')
.move('old/path/to/file', 'new/path/to/file')
-
Delete files within the same bucket:
const { data, error } = await storageClient.from('bucket').remove(['path/to/file'])
-
Create signed URL to download file without requiring permissions:
const expireIn = 60
const { data, error } = await storageClient
.from('bucket')
.createSignedUrl('path/to/file', expireIn)
-
Retrieve URLs for assets in public buckets:
const { data, error } = await storageClient.from('public-bucket').getPublicUrl('path/to/file')
We are building the features of Firebase using enterprise-grade, open source products. We support existing communities wherever possible, and if the products don’t exist we build them and open source them ourselves. Thanks to these sponsors who are making the OSS ecosystem better for everyone.
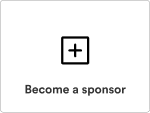