What is @supabase/supabase-js?
@supabase/supabase-js is a JavaScript client library for interacting with Supabase, an open-source Firebase alternative. It allows developers to perform a variety of database operations, authentication, and real-time subscriptions with ease.
What are @supabase/supabase-js's main functionalities?
Database Operations
This feature allows you to perform CRUD operations on your database. The code sample demonstrates how to fetch data from a table using the Supabase client.
const { createClient } = require('@supabase/supabase-js');
const supabase = createClient('https://your-project.supabase.co', 'public-anon-key');
async function fetchData() {
let { data, error } = await supabase
.from('your_table')
.select('*');
if (error) console.error(error);
else console.log(data);
}
fetchData();
Authentication
This feature allows you to manage user authentication, including sign-up, sign-in, and sign-out. The code sample demonstrates how to sign up a new user.
const { createClient } = require('@supabase/supabase-js');
const supabase = createClient('https://your-project.supabase.co', 'public-anon-key');
async function signUp() {
let { user, error } = await supabase.auth.signUp({
email: 'example@example.com',
password: 'example-password'
});
if (error) console.error(error);
else console.log(user);
}
signUp();
Real-time Subscriptions
This feature allows you to subscribe to real-time changes in your database. The code sample demonstrates how to listen for new rows being inserted into a table.
const { createClient } = require('@supabase/supabase-js');
const supabase = createClient('https://your-project.supabase.co', 'public-anon-key');
supabase
.from('your_table')
.on('INSERT', payload => {
console.log('New row added!', payload);
})
.subscribe();
Other packages similar to @supabase/supabase-js
firebase
Firebase is a comprehensive app development platform by Google that offers a variety of tools including Firestore for database operations, Firebase Auth for authentication, and Firebase Realtime Database for real-time data synchronization. Compared to @supabase/supabase-js, Firebase offers a more extensive suite of services but is not open-source.
hasura
Hasura is an open-source engine that provides instant GraphQL APIs on your data. It supports real-time subscriptions and integrates well with existing databases. Compared to @supabase/supabase-js, Hasura focuses on GraphQL APIs and offers more advanced querying capabilities.
parse
Parse is an open-source backend framework that provides a variety of features including a database, user authentication, and real-time notifications. It is similar to @supabase/supabase-js in its open-source nature and feature set but has a different architecture and community support.
supabase-js

An isomorphic JavaScript client for Supabase.
Usage
First of all, you need to install the library:
npm install @supabase/supabase-js
Then you're able to import the library and establish the connection with the database:
import { createClient } from '@supabase/supabase-js'
const supabase = createClient('https://xyzcompany.supabase.co', 'public-anon-key')
UMD
You can now use plain <script>
s to import supabase-js from CDNs, like:
<script src="https://cdn.jsdelivr.net/npm/@supabase/supabase-js"></script>
or even:
<script src="https://unpkg.com/@supabase/supabase-js"></script>
Then you can use it from a global supabase
variable:
<script>
const { createClient } = supabase
const _supabase = createClient('https://xyzcompany.supabase.co', 'public-anon-key')
console.log('Supabase Instance: ', _supabase)
</script>
ESM
You can now use type="module" <script>
s to import supabase-js from CDNs, like:
<script type="module">
import { createClient } from 'https://cdn.jsdelivr.net/npm/@supabase/supabase-js/+esm'
const supabase = createClient('https://xyzcompany.supabase.co', 'public-anon-key')
console.log('Supabase Instance: ', supabase)
</script>
Custom fetch
implementation
supabase-js
uses the cross-fetch
library to make HTTP requests, but an alternative fetch
implementation can be provided as an option. This is most useful in environments where cross-fetch
is not compatible, for instance Cloudflare Workers:
import { createClient } from '@supabase/supabase-js'
const supabase = createClient('https://xyzcompany.supabase.co', 'public-anon-key', {
global: {
fetch: (...args) => fetch(...args),
},
})
We are building the features of Firebase using enterprise-grade, open source products. We support existing communities wherever possible, and if the products don’t exist we build them and open source them ourselves. Thanks to these sponsors who are making the OSS ecosystem better for everyone.
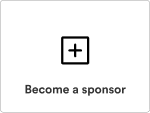