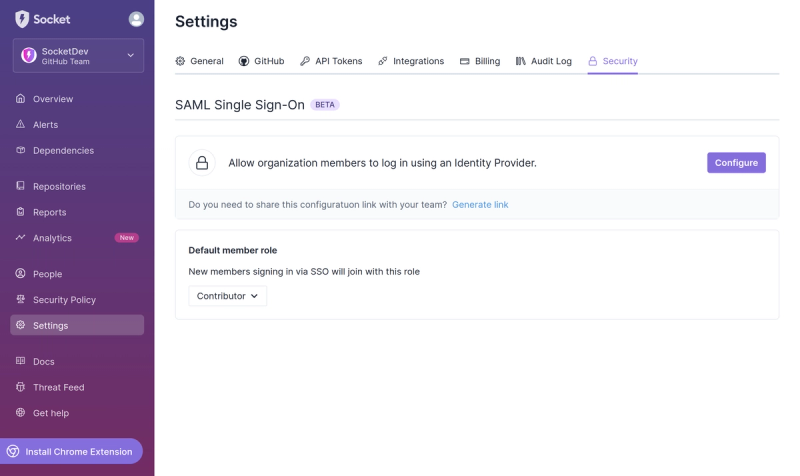
Product
Introducing SSO
Streamline your login process and enhance security by enabling Single Sign-On (SSO) on the Socket platform, now available for all customers on the Enterprise plan, supporting 20+ identity providers.
@suyongs/solid-utility
Advanced tools
Readme
Utility component, hooks or anything else for Solid JS
pnpm add @suyongs/solid-utility
npm install --save @suyongs/solid-utility
yarn add @suyongs/solid-utility
Transition
: Vue3 like Transition component
name
: [string]
, name of transitionappear
: [boolean]
, whether transition should be applied on initial render, default is false
mode
: in-out
out-in
, default is in-out
enterFromClass
: [string]
, class name of enter from, default is enter-from
enterActiveClass
: [string]
, class name of enter active, default is enter-active
enterToClass
: [string]
, class name of enter to, default is enter-to
leaveFromClass
: [string]
, class name of leave from, default is leave-from
leaveActiveClass
: [string]
, class name of leave active, default is leave-active
leaveToClass
: [string]
, class name of leave to, default is leave-to
onBeforeEnter
: [(el: HTMLElement) => void]
, callback before enteronEnter
: [(el: HTMLElement) => void]
, callback when enteronAfterEnter
: [(el: HTMLElement) => void]
, callback after enteronBeforeLeave
: [(el: HTMLElement) => void]
, callback before leaveonLeave
: [(el: HTMLElement) => void]
, callback when leaveonAfterLeave
: [(el: HTMLElement) => void]
, callback after leaveimport { Transition } from '@suyongs/solid-utility';
const Component = () => {
const [show, setShow] = createSignal(true);
return (
<Transition name={'fade'}>
<Show when={show()}>
<div>hello world</div>
</Show>
</Transition>
)
};
Marquee
: alternative for marquee tag
speed
: [number]
, move speed of marquee. pixel per seconds, default is 70
gap
: [number]
, gap between two marquee, default is 0
direction
: left
right
up
down
, default is left
mode
: [auto | scroll | truncate | hover | force-hover]
default is auto
'
auto
: contents are scrolled when overflow its parentscroll
mode always scrolledtruncate
mode will truncate the content when overflowhover
mode will scroll when hoverforce-hover
mode will scroll when hovered, even if it's not overflowautoUpdate
: [boolean]
, whether marquee should update automatically, default is true
component
: Component
, marquee can be any component, default is div
slots
: { first: Component; second: Component }
set internal component, default is same as component
slotProps
: { first: Props; second: Props }
internal component's propertiesref
: MarqueeRef
, ref of marquee. It has updateOverflow
method to update overflow statusimport { Marquee } from '@suyongs/solid-utility';
const Component = () => {
return (
<Marquee component={'a'} href={'https://github.com'}>
if you want to make this marquee 'a' tag, you should set component as 'a'
<span>
Also, you can set any components as marquee's children
</span>
</Marquee>
);
};
FAQs
Utilities for SolidJS
The npm package @suyongs/solid-utility receives a total of 104 weekly downloads. As such, @suyongs/solid-utility popularity was classified as not popular.
We found that @suyongs/solid-utility demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Product
Streamline your login process and enhance security by enabling Single Sign-On (SSO) on the Socket platform, now available for all customers on the Enterprise plan, supporting 20+ identity providers.
Security News
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
Security News
As cyber threats become more autonomous, AI-powered defenses are crucial for businesses to stay ahead of attackers who can exploit software vulnerabilities at scale.