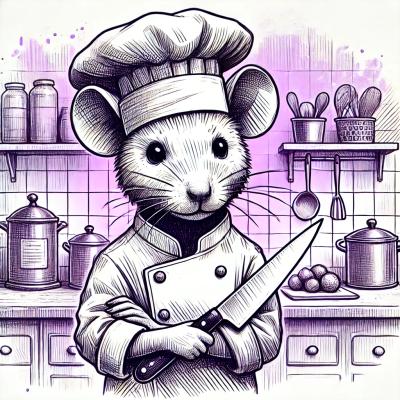
Research
Security News
Quasar RAT Disguised as an npm Package for Detecting Vulnerabilities in Ethereum Smart Contracts
Socket researchers uncover a malicious npm package posing as a tool for detecting vulnerabilities in Etherium smart contracts.
@synonymdev/blocktank-util-slack-client
Advanced tools
Client to interact with the blocktank-util-slack service.
import { SlackClient } from '@synonymdev/blocktank-util-slack-client';
const client = new SlackClient('myConfiguredChannelName') // See service config for channel names.
await client.info('myTitle', 'myMessage') // Send message.
If the channel name is empty, the message will be logged to the console instead of sent to slack.
Messages can be ratelimited to not overwhelm the channel. The rate limit is based on a key. If the key was already seen within the limitFor
duration, the message will be ignored.
interface RateLimitOptions {
// Key of the operation that will be rate limited. Example: `blocktank-instant:funds-are-low`.
key: string,
// Duration of the rate limit until the next message can be sent.
limitFor: Duration
}
// Amount of time the limit should be applied for this key.
interface Duration {
years?: number;
months?: number;
weeks?: number;
days?: number;
hours?: number;
minutes?: number;
seconds?: number;
}
Let's make an example:
await client.error('Couldnt read file', 'errorMessage', {
key: 'blocktank-ls-btc:fileReadKey',
limitFor: {
hours: 1
}
})
This will send a message with the level error
and the title Couldnt read file
.
The message will be sent only if the last message with the key blocktank-ls-btc:fileReadKey
was sent more than 1 hour ago.
If the last message was sent less than 1 hour ago, the message will be ignored.
SlackMessageLevel | Emoji |
---|---|
debug | |
success | ✅ |
info | ℹ️ |
notice | 📢 |
warning | ⚠️ |
error | ❌ |
critical | ‼️ |
alert | 🚨 |
emergency | 🆘 |
Execute npx blocktank-util-slack-cli
in the terminal to send test messages. Make sure you install this package first.
package.json
.CHANGELOG.md
.git tag v0.1.0
.git push origin v0.1.0
.npm publish
.FAQs
Blocktank Slack client
We found that @synonymdev/blocktank-util-slack-client demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 4 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
Socket researchers uncover a malicious npm package posing as a tool for detecting vulnerabilities in Etherium smart contracts.
Security News
Research
A supply chain attack on Rspack's npm packages injected cryptomining malware, potentially impacting thousands of developers.
Research
Security News
Socket researchers discovered a malware campaign on npm delivering the Skuld infostealer via typosquatted packages, exposing sensitive data.