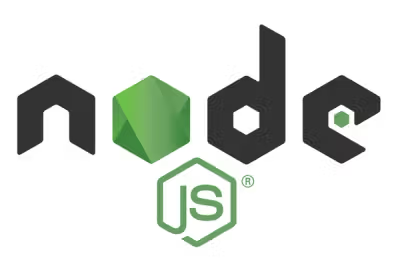
Security News
require(esm) Backported to Node.js 20, Paving the Way for ESM-Only Packages
require(esm) backported to Node.js 20, easing the transition to ESM-only packages and reducing complexity for developers as Node 18 nears end-of-life.
@talkscriber-npm/ts-client
Advanced tools
ts-client is the official TypeScript client for Talkscriber, a state-of-the-Art Speech-to-Text (STT) platform tailored for conversational AI enterprises. It provides exceptional transcription services with a strong emphasis on privacy and security to enhance customer communication while protecting sensitive information.
Follow these steps to install and use the ts-client for Talkscriber:
Install the package:
npm install @talkscriber-npm/ts-client
In your project, create a new file (e.g., transcribe.ts
) and add the following code:
import { TalkscriberTranscriptionService } from '@talkscriber-npm/ts-client';
async function main() {
const talkscriber = new TalkscriberTranscriptionService({
apiKey: '<YOUR_API_KEY>',
language: 'en', // Specify the language here (e.g., 'en' for English)
onTranscription: (text: string) => {
console.log('Transcription:', text);
},
onUtterance: (text: string) => {
console.log('Utterance:', text);
}
});
try {
await talkscriber.connect();
console.log('Connected to Talkscriber service');
// Your audio processing code here
// For example:
// const audioData = new Float32Array(/* your audio data */);
// const sampleRate = 44100; // or your actual sample rate
// talkscriber.send(audioData, sampleRate);
} catch (error) {
console.error('Error:', error instanceof Error ? error.message : String(error));
} finally {
talkscriber.close();
}
}
main().catch(console.error);
Replace <YOUR_API_KEY>
with your actual Talkscriber API key.
Install the necessary TypeScript dependencies if you haven't already:
npm install -D typescript ts-node @types/node
Compile and run your TypeScript code:
npx ts-node transcribe.ts
This will initialize the Talkscriber client and connect to the service. You'll need to add your own audio processing logic to send audio data to the talkscriber.send()
method.
For complete examples of audio file processing, refer to the examples
directory in the package source code.
To run the provided examples:
Ensure you're in the root directory of the project where package.json
is located.
Install the required dependencies if you haven't already:
npm install
Run the example:
npm run example
Please note that you need to replace <YOUR_API_KEY>
in the example script with your actual Talkscriber API key before running it.
The provided code is agnostic towards the sample rate and should be able to handle any .wav file/buffer that is pcm_s16le encoded.
The Talkscriber engine handles the following languages:
This code is released under the MIT License. See [LICENSE] for further details.
FAQs
A JS/TypeScript client module for Talkscriber
The npm package @talkscriber-npm/ts-client receives a total of 0 weekly downloads. As such, @talkscriber-npm/ts-client popularity was classified as not popular.
We found that @talkscriber-npm/ts-client demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
require(esm) backported to Node.js 20, easing the transition to ESM-only packages and reducing complexity for developers as Node 18 nears end-of-life.
Security News
PyPI now supports iOS and Android wheels, making it easier for Python developers to distribute mobile packages.
Security News
Create React App is officially deprecated due to React 19 issues and lack of maintenance—developers should switch to Vite or other modern alternatives.