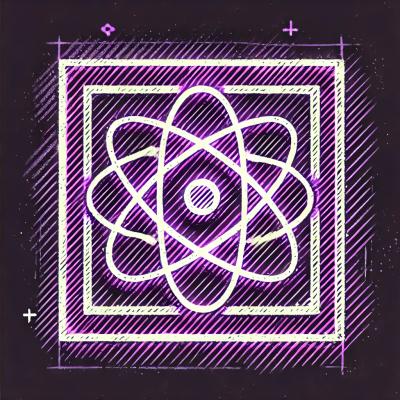
Security News
Create React App Officially Deprecated Amid React 19 Compatibility Issues
Create React App is officially deprecated due to React 19 issues and lack of maintenance—developers should switch to Vite or other modern alternatives.
@technologyadvice/ta-good-table
Advanced tools
TA Good Table is forked from [Vue Good Table](https://github.com/xaksis/vue-good-table). We've made some updates to it to fit our Traction needs, and have been trying to capture the major changes below.
TA Good Table is forked from Vue Good Table. We've made some updates to it to fit our Traction needs, and have been trying to capture the major changes below.
npm install @technologyadvice/ta-good-table
import Component:
import VueGoodTable from '@technologyadvice/ta-good-table'
import Styles:
@import '~ta-good-table/src/styles/style.scss';
rr repo pull -fib ta-good-table
rr app package link ta-good-table accounts-portal -b
Publishing of NPM packages to the Satisfaction registry happens automatically via the publish
GitHub Actions workflow.
To enable filtering on a column, pass a filterOptions
property on the column object with the enabled flag. This will take all column field values and format it into an options dropdown.
filterOptions: {
enabled: true,
},
You can set an initial filter value by passing in an object to VueGoodTable
. When setting the filter options, ta-good-table will set the field's active property to true.
:initialFilter="{
field: plan_name, // name of the column field
value: Free, // value to filter by
}"
Use this option if you have multiple columns that may share values and want only one filter dropdown to filter on both columns. Enable the filterOptions
on both columns as shown above, and pass the following as a prop. For each set, the first field in the array will have the filter icon/dropdown.
:multi-column-filter="{
enabled: true,
columnSets: [
[ 'field_one', 'field_two' ],
],
}"
Use this if you have a static list of filter options.
filterOptions: {
enabled: true,
type: 'remote',
staticFilterOptions: [
{ id: 1, value: 'testing' },
{ id: 2, value: 'testing123' },
],
promoteActiveOptions: true, //orders the selected options before the the others when the filter is opened- defaults to false for static filters
outputFilterIdentifier: 'test_options_selected', //the key of the selected options in the request payload
},
Use this option if you have multiple fields in a single column. You will need to add a subFields
property to the column object:
subFields: [ {
field: 'cool-field',
label: 'Cool Field',
filterActive: false, //necessary for reactivity, but not needed if not using filter
filterFn: method, // optional
type: 'date' // optional - will default to string
} ],
To enable the filter, just pass the field name into the enableSubFields
array (you also need the filterActive
property shown above):
filterOptions: {
enableSubFields: [ 'client' ]
},
Sorting is enabled by default. To disable, add sortable: false
to the column object. Be sure to pass to pass the correct type
for proper sorting.
:sort-options="{
enabled: true,
initialSortBy: {
field: 'field_name',
type: 'asc', // or 'desc'
}
}"
Used for sorting (and possibly filtering in the future):
Pagination is enabled by default and has the following options. Note: currently the TA pagination is only setup for position: bottom
:pagination-options="{
enabled: true,
perPage: 10,
position: 'bottom',
setCurrentPage: 1,
}"
Remote filtering is also possible - check out this confluence document for more information.
For clickable rows, you may either listen for the on-row-click
event (which will have the row in the payload), or you can use the clickable-row-options
property for routing. rowProperty
is the value the route will use in the query params.
:clickable-row-options="{
url: '/',
rowProperty: 'uuid',
useVueRouter: true,
}"
pass the class-name
prop to the VueGoodTable
to dynamically generate classes on the table, rows, and headers using the field property on the column object.
class-name: "cool-table"
will generate:
cool-table
on the table root elementcool-table__cool-field
on the header and cells (if the field is cool-field
)cool-table__cool-field--header
on the column's header elementcool-table__cool-field--cell
on the column's cell element
cool-table__customname-cell
Pass the zero-state-message
prop to the VueGoodTable
if you want a custom zero state for that implementation of the table. The default message is "No results found" but good UX practices dictate selecting a zero message that is relevant to the content of your table.
v-slot:table-full-row="props"
v-slot:table-row="props"
v-slot:table-column="props"
(see implementation below)When mode="remote"
and you have selectOptions.selectAllByPage=false
, ta-good-tables uses prop selectedRowsRemote
for its selected rows, selectOptions.selectionIdentifier
for the row identifier to use when selecting, selectOptions.getAllSelectionItems
function to get all selected rows and on-selected-rows-changed
to keep the selectedRowsRemote
updated.
selectOptions.selectionIdentifier
is the unique field for a row, most likely uuid
.
selectedRowsRemote
is a prop for ta-good-tables that should be a list of your selectOptions.selectionIdentifier
for each selected row.
selectOptions.getAllSelectionItems
should return a list of all selectable row identifiers. This should take into account the same filtering and searching that the table is using. So if your current table only shows 3 rows and your selectOptions.selectionIdentifier
is "uuid"
then this function should return a list of the 3 UUIDs.
on-selected-rows-changed
returns the current list of selected rows and should replace your selectedRowsRemote
prop value.
Table:
<VueGoodTable
:cols="columns"
:rows="rows"
class-name="cool-table"
:clickable-row-options="{
url: '/cool',
rowProperty: 'uuid',
useVueRouter: true,
}"
:sort-options="{
enabled: true,
initialSortBy: {
field: 'date',
type: 'asc'/'desc',
}
}"
:multi-column-filter="{
enabled: true,
columnSets: [
[ 'field_one', 'field_two' ],
],
}"
:pagination-options="{
enabled: true,
mode: 'records',
perPage: type === 'unresolved' ? 10 : 5,
position: 'bottom',
}"
zero-state-message="Looks like you don't have any rows">
<template v-slot:table-full-row="props">
// use a custom component for the full row instead of just a single column
<td :colspan="columns.length">
<CustomRowComponent :item="props.row"></CustomRowComponent>
</td>
</template>
<template v-slot:table-row="props">
<span v-if="props.column.field == 'field_name'">
// Custom Row
</span>
</template>
<template v-slot:table-column="props">
<span v-if="props.column.field == 'field_name'">
// Custom Header
</span>
</template>
</VueGoodTable>
Columns
const columns = [
{
label: 'Name',
field: 'name',
subFields: [ { field: 'client', label: 'Client', filterActive: false } ],
filterOptions: {
enableSubField: [ 'client' ]
},
},
{
label: 'Brand',
field: 'brand',
filterOptions: {
enabled: true,
},
},
{
label: 'Date',
field: 'date',
type: 'date',
dateInputFormat: 'yyyy-MM-dd',
dateOutputFormat: 'E, MMM dd y',
},
{
label: 'Count',
field: 'count',
type: 'number',
},
]
A common issue with blank columns happens when there are custom columns on the page
you may see something like this in the table-row
slot:
<template v-if="props.column.field === 'name'">...</template>
<template v-if="props.column.field === 'date'">...</template>
The above code could cause blank columns. The solution would be every conditional <template>
after the initial v-if
, should be a v-else-if
<span v-if="props.column.field === 'name'"></span>
<span v-else-if="props.column.field === 'date'"></span>
FAQs
TA Good Table is forked from [Vue Good Table](https://github.com/xaksis/vue-good-table). We've made some updates to it to fit our Traction needs, and have been trying to capture the major changes below.
We found that @technologyadvice/ta-good-table demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 16 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Create React App is officially deprecated due to React 19 issues and lack of maintenance—developers should switch to Vite or other modern alternatives.
Security News
Oracle seeks to dismiss fraud claims in the JavaScript trademark dispute, delaying the case and avoiding questions about its right to the name.
Security News
The Linux Foundation is warning open source developers that compliance with global sanctions is mandatory, highlighting legal risks and restrictions on contributions.