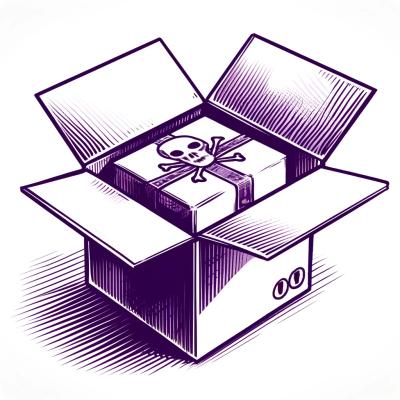
Security News
Research
Data Theft Repackaged: A Case Study in Malicious Wrapper Packages on npm
The Socket Research Team breaks down a malicious wrapper package that uses obfuscation to harvest credentials and exfiltrate sensitive data.
@tensorflow/tfjs
Advanced tools
TensorFlow.js is an open-source hardware-accelerated JavaScript library for training and deploying machine learning models.
Develop ML in the Browser
Use flexible and intuitive APIs to build models from scratch using the low-level
JavaScript linear algebra library or the high-level layers API.
Develop ML in Node.js
Execute native TensorFlow with the same TensorFlow.js API under the Node.js
runtime.
Run Existing models
Use TensorFlow.js model converters to run pre-existing TensorFlow models right
in the browser.
Retrain Existing models
Retrain pre-existing ML models using sensor data connected to the browser or
other client-side data.
This repository contains the logic and scripts that combine several packages.
APIs:
Backends/Platforms:
If you care about bundle size, you can import those packages individually.
If you are looking for Node.js support, check out the TensorFlow.js Node directory.
Check out our examples repository and our tutorials.
Be sure to check out the gallery of all projects related to TensorFlow.js.
Be sure to also check out our models repository where we host pre-trained models on NPM.
There are two main ways to get TensorFlow.js in your JavaScript project: via script tags or by installing it from NPM and using a build tool like Parcel, WebPack, or Rollup.
Add the following code to an HTML file:
<html>
<head>
<!-- Load TensorFlow.js -->
<script src="https://cdn.jsdelivr.net/npm/@tensorflow/tfjs/dist/tf.min.js"> </script>
<!-- Place your code in the script tag below. You can also use an external .js file -->
<script>
// Notice there is no 'import' statement. 'tf' is available on the index-page
// because of the script tag above.
// Define a model for linear regression.
const model = tf.sequential();
model.add(tf.layers.dense({units: 1, inputShape: [1]}));
// Prepare the model for training: Specify the loss and the optimizer.
model.compile({loss: 'meanSquaredError', optimizer: 'sgd'});
// Generate some synthetic data for training.
const xs = tf.tensor2d([1, 2, 3, 4], [4, 1]);
const ys = tf.tensor2d([1, 3, 5, 7], [4, 1]);
// Train the model using the data.
model.fit(xs, ys).then(() => {
// Use the model to do inference on a data point the model hasn't seen before:
// Open the browser devtools to see the output
model.predict(tf.tensor2d([5], [1, 1])).print();
});
</script>
</head>
<body>
</body>
</html>
Open up that HTML file in your browser, and the code should run!
Add TensorFlow.js to your project using yarn or npm. Note: Because
we use ES2017 syntax (such as import
), this workflow assumes you are using a modern browser or a bundler/transpiler
to convert your code to something older browsers understand. See our
examples
to see how we use Parcel to build
our code. However, you are free to use any build tool that you prefer.
import * as tf from '@tensorflow/tfjs';
// Define a model for linear regression.
const model = tf.sequential();
model.add(tf.layers.dense({units: 1, inputShape: [1]}));
// Prepare the model for training: Specify the loss and the optimizer.
model.compile({loss: 'meanSquaredError', optimizer: 'sgd'});
// Generate some synthetic data for training.
const xs = tf.tensor2d([1, 2, 3, 4], [4, 1]);
const ys = tf.tensor2d([1, 3, 5, 7], [4, 1]);
// Train the model using the data.
model.fit(xs, ys).then(() => {
// Use the model to do inference on a data point the model hasn't seen before:
model.predict(tf.tensor2d([5], [1, 1])).print();
});
See our tutorials, examples and documentation for more details.
We support porting pre-trained models from:
Please refer below :
TensorFlow.js is a part of the TensorFlow ecosystem. For more info:
tfjs
tag on the TensorFlow Forum.Thanks, BrowserStack, for providing testing support.
FAQs
An open-source machine learning framework.
The npm package @tensorflow/tfjs receives a total of 75,361 weekly downloads. As such, @tensorflow/tfjs popularity was classified as popular.
We found that @tensorflow/tfjs demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 10 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Research
The Socket Research Team breaks down a malicious wrapper package that uses obfuscation to harvest credentials and exfiltrate sensitive data.
Research
Security News
Attackers used a malicious npm package typosquatting a popular ESLint plugin to steal sensitive data, execute commands, and exploit developer systems.
Security News
The Ultralytics' PyPI Package was compromised four times in one weekend through GitHub Actions cache poisoning and failure to rotate previously compromised API tokens.