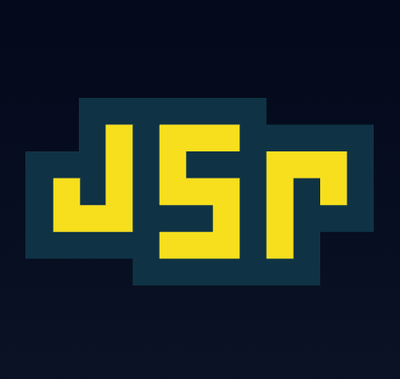
Security News
JSR Working Group Kicks Off with Ambitious Roadmap and Plans for Open Governance
At its inaugural meeting, the JSR Working Group outlined plans for an open governance model and a roadmap to enhance JavaScript package management.
@testing-library/user-event
Advanced tools
The @testing-library/user-event package is a library built on top of @testing-library/dom that simulates user interactions with the DOM. It allows developers to write tests that mimic user behavior in a way that is consistent with how a user would use a web application. This helps ensure that tests are realistic and that the application will work as expected when real users interact with it.
Typing text into an input element
Simulates typing text into an input field or other element that can receive input.
userEvent.type(screen.getByRole('textbox'), 'Hello, World!')
Clicking elements
Simulates a click event on a button, link, or any other clickable element.
userEvent.click(screen.getByText('Submit'))
Selecting options in a select element
Simulates selecting an option from a dropdown select element.
userEvent.selectOptions(screen.getByRole('combobox'), 'optionValue')
Uploading files
Simulates a file upload interaction on an input of type file.
userEvent.upload(screen.getByLabelText('Upload'), file)
Tabbing through elements
Simulates tabbing through focusable elements on the page.
userEvent.tab()
Selenium WebDriver is a browser automation library. It is more comprehensive than @testing-library/user-event as it can control the browser at a lower level, but it is also more complex and can be overkill for simple DOM interaction tests.
Cypress is an end-to-end testing framework that includes a rich set of methods for simulating user events. It is similar to @testing-library/user-event but is designed for full end-to-end testing and offers a complete testing environment with a browser-based UI.
Puppeteer is a Node library which provides a high-level API to control Chrome or Chromium over the DevTools Protocol. It is used for browser automation and can perform actions similar to @testing-library/user-event, but it operates at the browser level and can be used for tasks beyond testing, such as web scraping or generating pre-rendered content.
From testing-library/dom-testing-library#107:
[...] it is becoming apparent the need to express user actions on a web page using a higher-level abstraction than
fireEvent
user-event
tries to simulate the real events that would happen in the browser
as the user interacts with it. For example userEvent.click(checkbox)
would
change the state of the checkbox.
The library is still a work in progress and any help is appreciated.
With NPM:
npm install @testing-library/user-event --save-dev
With Yarn:
yarn add @testing-library/user-event --dev
Now simply import it in your tests:
import userEvent from "@testing-library/user-event";
// or
var userEvent = require("@testing-library/user-event");
click(element)
Clicks element
, depending on what element
is it can have different side
effects.
import React from "react";
import { render } from "@testing-library/react";
import userEvent from "@testing-library/user-event";
const { getByText, getByTestId } = test("click", () => {
render(
<div>
<label htmlFor="checkbox">Check</label>
<input id="checkbox" data-testid="checkbox" type="checkbox" />
</div>
);
});
userEvent.click(getByText("Check"));
expect(getByTestId("checkbox")).toHaveAttribute("checked", true);
dblClick(element)
Clicks element
twice, depending on what element
is it can have different
side effects.
import React from "react";
import { render } from "@testing-library/react";
import userEvent from "@testing-library/user-event";
test("double click", () => {
const onChange = jest.fn();
const { getByTestId } = render(
<input type="checkbox" id="checkbox" onChange={onChange} />
);
const checkbox = getByTestId("checkbox");
userEvent.dblClick(checkbox);
expect(onChange).toHaveBeenCalledTimes(2);
expect(checkbox).toHaveProperty("checked", false);
});
type(element, text, [options])
Writes text
inside an <input>
or a <textarea>
.
import React from "react";
import { render } from "@testing-library/react";
import userEvent from "@testing-library/user-event";
const { getByText } = test("click", () => {
render(<textarea data-testid="email" />);
});
userEvent.type(getByTestId("email"), "Hello, World!");
expect(getByTestId("email")).toHaveAttribute("value", "Hello, World!");
If options.allAtOnce
is true
, type
will write text
at once rather than
one character at the time. false
is the default value.
options.delay
is the number of milliseconds that pass between two characters
are typed. By default it's 0. You can use this option if your component has a
different behavior for fast or slow users.
selectOptions(element, values)
Selects the specified option(s) of a <select>
or a <select multiple>
element.
import React from "react";
import { render } from "@testing-library/react";
import userEvent from "@testing-library/user-event";
const { getByTestId } = render(
<select multiple data-testid="select-multiple">
<option data-testid="val1" value="1">
1
</option>
<option data-testid="val2" value="2">
2
</option>
<option data-testid="val3" value="3">
3
</option>
</select>
);
userEvent.selectOptions(getByTestId("select-multiple"), ["1", "3"]);
expect(getByTestId("val1").selected).toBe(true);
expect(getByTestId("val2").selected).toBe(false);
expect(getByTestId("val3").selected).toBe(true);
The values
parameter can be either an array of values or a singular scalar
value.
Thanks goes to these wonderful people (emoji key):
Giorgio Polvara 🐛 💻 📖 🤔 🚇 👀 ⚠️ | Weyert de Boer 💻 ⚠️ | Tim Whitbeck 🐛 💻 | Michaël De Boey 📖 | Michael Lasky 💻 📖 🤔 | Ahmad Esmaeilzadeh 📖 | Caleb Eby 💻 🐛 |
Adrià Fontcuberta 🐛 ⚠️ |
This project follows the all-contributors specification. Contributions of any kind welcome!
FAQs
Fire events the same way the user does
We found that @testing-library/user-event demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 15 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
At its inaugural meeting, the JSR Working Group outlined plans for an open governance model and a roadmap to enhance JavaScript package management.
Security News
Research
An advanced npm supply chain attack is leveraging Ethereum smart contracts for decentralized, persistent malware control, evading traditional defenses.
Security News
Research
Attackers are impersonating Sindre Sorhus on npm with a fake 'chalk-node' package containing a malicious backdoor to compromise developers' projects.