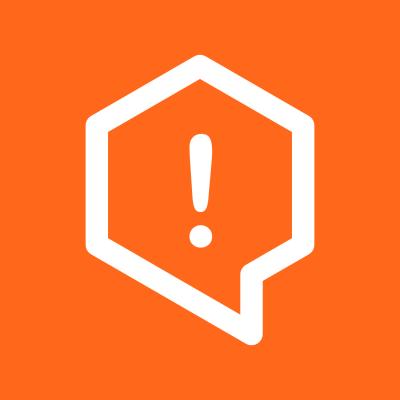
Security News
Fluent Assertions Faces Backlash After Abandoning Open Source Licensing
Fluent Assertions is facing backlash after dropping the Apache license for a commercial model, leaving users blindsided and questioning contributor rights.
@thi.ng/api
Advanced tools
@thi.ng/api is a collection of TypeScript interfaces, types, and utility functions for functional programming, event handling, and other common tasks in JavaScript/TypeScript projects.
Event Handling
This feature allows you to create and manage custom events. The code sample demonstrates creating an event, adding a listener, and dispatching the event.
const { Event } = require('@thi.ng/api');
const event = new Event('test');
event.addListener('test', (e) => console.log('Event received:', e));
event.dispatch('test', { message: 'Hello, World!' });
Functional Programming Utilities
This feature provides utility functions for functional programming. The code sample demonstrates filtering an array to get even numbers and then mapping the array to get their squares.
const { map, filter } = require('@thi.ng/api');
const nums = [1, 2, 3, 4, 5];
const evenNums = filter((x) => x % 2 === 0, nums);
const squaredNums = map((x) => x * x, evenNums);
console.log(squaredNums); // [4, 16]
Type Utilities
This feature includes type-checking utilities. The code sample demonstrates checking if a value is a string or a number.
const { isString, isNumber } = require('@thi.ng/api');
console.log(isString('hello')); // true
console.log(isNumber(123)); // true
console.log(isNumber('123')); // false
Lodash is a modern JavaScript utility library delivering modularity, performance, and extras. It provides similar functional programming utilities like map, filter, and type-checking functions. However, it does not focus on event handling.
RxJS is a library for reactive programming using Observables, to make it easier to compose asynchronous or callback-based code. It offers more advanced event handling and functional programming utilities compared to @thi.ng/api.
Ramda is a practical functional library for JavaScript programmers. It focuses on immutability and side-effect-free functions, providing utilities similar to @thi.ng/api's functional programming features.
[!NOTE] This is one of 200 standalone projects, maintained as part of the @thi.ng/umbrella monorepo and anti-framework.
🚀 Please help me to work full-time on these projects by sponsoring me on GitHub. Thank you! ❤️
Common, generic types, interfaces & mixins.
This package is implicitly used by most other projects in this repository. It defines:
STABLE - used in production
Search or submit any issues for this package
yarn add @thi.ng/api
ESM import:
import * as api from "@thi.ng/api";
Browser ESM import:
<script type="module" src="https://esm.run/@thi.ng/api"></script>
For Node.js REPL:
const api = await import("@thi.ng/api");
Package sizes (brotli'd, pre-treeshake): ESM: 2.41 KB
None
If this project contributes to an academic publication, please cite it as:
@misc{thing-api,
title = "@thi.ng/api",
author = "Karsten Schmidt and others",
note = "https://thi.ng/api",
year = 2016
}
© 2016 - 2025 Karsten Schmidt // Apache License 2.0
FAQs
Common, generic types, interfaces & mixins
The npm package @thi.ng/api receives a total of 140,754 weekly downloads. As such, @thi.ng/api popularity was classified as popular.
We found that @thi.ng/api demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Fluent Assertions is facing backlash after dropping the Apache license for a commercial model, leaving users blindsided and questioning contributor rights.
Research
Security News
Socket researchers uncover the risks of a malicious Python package targeting Discord developers.
Security News
The UK is proposing a bold ban on ransomware payments by public entities to disrupt cybercrime, protect critical services, and lead global cybersecurity efforts.