@thi.ng/binary
Advanced tools
Comparing version 1.2.0 to 1.2.1
@@ -6,4 +6,4 @@ import { Pow2 } from "./api"; | ||
* | ||
* @param addr | ||
* @param size | ||
* @param addr - value to align | ||
* @param size - alignment value | ||
*/ | ||
@@ -15,1 +15,2 @@ export declare const align: (addr: number, size: Pow2) => number; | ||
export declare const isAligned: (addr: number, size: Pow2) => boolean; | ||
//# sourceMappingURL=align.d.ts.map |
@@ -5,4 +5,4 @@ /** | ||
* | ||
* @param addr | ||
* @param size | ||
* @param addr - value to align | ||
* @param size - alignment value | ||
*/ | ||
@@ -9,0 +9,0 @@ export const align = (addr, size) => (size--, (addr + size) & ~size); |
@@ -7,1 +7,2 @@ export declare type Lane8 = 0 | 1 | 2 | 3; | ||
export declare const MASKS: number[]; | ||
//# sourceMappingURL=api.d.ts.map |
@@ -6,2 +6,10 @@ # Change Log | ||
## [1.2.1](https://github.com/thi-ng/umbrella/compare/@thi.ng/binary@1.2.0...@thi.ng/binary@1.2.1) (2020-01-24) | ||
**Note:** Version bump only for package @thi.ng/binary | ||
# [1.2.0](https://github.com/thi-ng/umbrella/compare/@thi.ng/binary@1.1.1...@thi.ng/binary@1.2.0) (2019-11-30) | ||
@@ -8,0 +16,0 @@ |
/** | ||
* Returns number of 1 bits in `x`. | ||
* | ||
* @param x | ||
* @param x - | ||
*/ | ||
export declare const popCount: (x: number) => number; | ||
/** | ||
* https://en.wikipedia.org/wiki/Hamming_distance | ||
* Returns number of bit changes between `x` and `y`. | ||
* | ||
* @param x | ||
* @param y | ||
* {@link https://en.wikipedia.org/wiki/Hamming_distance} | ||
* | ||
* @param x - | ||
* @param y - | ||
*/ | ||
@@ -17,5 +19,5 @@ export declare const hammingDist: (x: number, y: number) => number; | ||
* | ||
* https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/Math/clz32$revision/1426816 | ||
* {@link https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/Math/clz32$revision/1426816} | ||
* | ||
* @param x | ||
* @param x - | ||
*/ | ||
@@ -25,6 +27,8 @@ export declare const clz32: (x: number) => number; | ||
/** | ||
* Returns the number of bits required to encode `x` (MUST be > 0). | ||
* Returns the number of bits required to encode `x`. Returns zero if | ||
* `x` <= 1. | ||
* | ||
* @param x | ||
* @param x - | ||
*/ | ||
export declare const bitSize: (x: number) => number; | ||
//# sourceMappingURL=count.d.ts.map |
21
count.js
/** | ||
* Returns number of 1 bits in `x`. | ||
* | ||
* @param x | ||
* @param x - | ||
*/ | ||
@@ -10,6 +10,8 @@ export const popCount = (x) => ((x = x - ((x >>> 1) & 0x55555555)), | ||
/** | ||
* https://en.wikipedia.org/wiki/Hamming_distance | ||
* Returns number of bit changes between `x` and `y`. | ||
* | ||
* @param x | ||
* @param y | ||
* {@link https://en.wikipedia.org/wiki/Hamming_distance} | ||
* | ||
* @param x - | ||
* @param y - | ||
*/ | ||
@@ -20,5 +22,5 @@ export const hammingDist = (x, y) => popCount(x ^ y); | ||
* | ||
* https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/Math/clz32$revision/1426816 | ||
* {@link https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/Math/clz32$revision/1426816} | ||
* | ||
* @param x | ||
* @param x - | ||
*/ | ||
@@ -38,6 +40,7 @@ export const clz32 = (x) => x !== 0 ? 31 - ((Math.log(x >>> 0) / Math.LN2) | 0) : 32; | ||
/** | ||
* Returns the number of bits required to encode `x` (MUST be > 0). | ||
* Returns the number of bits required to encode `x`. Returns zero if | ||
* `x` <= 1. | ||
* | ||
* @param x | ||
* @param x - | ||
*/ | ||
export const bitSize = (x) => Math.ceil(Math.log(x) / Math.LN2); | ||
export const bitSize = (x) => (x > 1 ? Math.ceil(Math.log2(x)) : 0); |
@@ -5,4 +5,4 @@ import { Bit } from "./api"; | ||
* | ||
* @param x value | ||
* @param bit bit number (0..31) | ||
* @param x - value | ||
* @param bit - bit number (0..31) | ||
*/ | ||
@@ -13,4 +13,4 @@ export declare const bitClear: (x: number, bit: Bit) => number; | ||
* | ||
* @param x | ||
* @param bit | ||
* @param x - value | ||
* @param bit - bit ID | ||
*/ | ||
@@ -21,4 +21,4 @@ export declare const bitFlip: (x: number, bit: Bit) => number; | ||
* | ||
* @param x value | ||
* @param bit bit number (0..31) | ||
* @param x - value | ||
* @param bit - bit number (0..31) | ||
*/ | ||
@@ -28,1 +28,2 @@ export declare const bitSet: (x: number, bit: Bit) => number; | ||
export declare const bitClearWindow: (x: number, from: number, to: number) => number; | ||
//# sourceMappingURL=edit.d.ts.map |
12
edit.js
@@ -5,4 +5,4 @@ import { defMask } from "./mask"; | ||
* | ||
* @param x value | ||
* @param bit bit number (0..31) | ||
* @param x - value | ||
* @param bit - bit number (0..31) | ||
*/ | ||
@@ -13,4 +13,4 @@ export const bitClear = (x, bit) => (x & ~(1 << bit)) >>> 0; | ||
* | ||
* @param x | ||
* @param bit | ||
* @param x - value | ||
* @param bit - bit ID | ||
*/ | ||
@@ -21,4 +21,4 @@ export const bitFlip = (x, bit) => (x ^ (1 << bit)) >>> 0; | ||
* | ||
* @param x value | ||
* @param bit bit number (0..31) | ||
* @param x - value | ||
* @param bit - bit number (0..31) | ||
*/ | ||
@@ -25,0 +25,0 @@ export const bitSet = (x, bit) => (x | (1 << bit)) >>> 0; |
@@ -7,9 +7,10 @@ export declare const floatToIntBits: (x: number) => number; | ||
* Converts given float into a sortable integer representation, using | ||
* raw bitwise conversion via `floatToIntBits()`. | ||
* raw bitwise conversion via {@link floatToIntBits}. | ||
* | ||
* https://github.com/tzaeschke/phtree/blob/master/PhTreeRevisited.pdf | ||
* {@link https://github.com/tzaeschke/phtree/blob/master/PhTreeRevisited.pdf} | ||
* (page 3) | ||
* | ||
* @param x | ||
* @param x - value to convert | ||
*/ | ||
export declare const floatToSortableInt: (x: number) => number; | ||
//# sourceMappingURL=float.d.ts.map |
@@ -10,8 +10,8 @@ const F32 = new Float32Array(1); | ||
* Converts given float into a sortable integer representation, using | ||
* raw bitwise conversion via `floatToIntBits()`. | ||
* raw bitwise conversion via {@link floatToIntBits}. | ||
* | ||
* https://github.com/tzaeschke/phtree/blob/master/PhTreeRevisited.pdf | ||
* {@link https://github.com/tzaeschke/phtree/blob/master/PhTreeRevisited.pdf} | ||
* (page 3) | ||
* | ||
* @param x | ||
* @param x - value to convert | ||
*/ | ||
@@ -18,0 +18,0 @@ export const floatToSortableInt = (x) => { |
@@ -6,5 +6,5 @@ /** | ||
* | ||
* https://en.wikipedia.org/wiki/Gray_code | ||
* {@link https://en.wikipedia.org/wiki/Gray_code} | ||
* | ||
* @param x u32 | ||
* @param x - u32 | ||
*/ | ||
@@ -15,4 +15,5 @@ export declare const encodeGray32: (x: number) => number; | ||
* | ||
* https://en.wikipedia.org/wiki/Gray_code | ||
* {@link https://en.wikipedia.org/wiki/Gray_code} | ||
*/ | ||
export declare const decodeGray32: (x: number) => number; | ||
//# sourceMappingURL=gray.d.ts.map |
@@ -6,5 +6,5 @@ /** | ||
* | ||
* https://en.wikipedia.org/wiki/Gray_code | ||
* {@link https://en.wikipedia.org/wiki/Gray_code} | ||
* | ||
* @param x u32 | ||
* @param x - u32 | ||
*/ | ||
@@ -15,3 +15,3 @@ export const encodeGray32 = (x) => (x ^ (x >>> 1)) >>> 0; | ||
* | ||
* https://en.wikipedia.org/wiki/Gray_code | ||
* {@link https://en.wikipedia.org/wiki/Gray_code} | ||
*/ | ||
@@ -18,0 +18,0 @@ export const decodeGray32 = (x) => { |
@@ -13,1 +13,2 @@ export * from "./api"; | ||
export * from "./swizzle"; | ||
//# sourceMappingURL=index.d.ts.map |
@@ -26,3 +26,3 @@ 'use strict'; | ||
}; | ||
const bitSize = (x) => Math.ceil(Math.log(x) / Math.LN2); | ||
const bitSize = (x) => (x > 1 ? Math.ceil(Math.log2(x)) : 0); | ||
@@ -29,0 +29,0 @@ const defMask = (a, b) => (~MASKS[a] & MASKS[b]) >>> 0; |
@@ -1,1 +0,1 @@ | ||
!function(t,e){"object"==typeof exports&&"undefined"!=typeof module?e(exports):"function"==typeof define&&define.amd?define(["exports"],e):e(((t=t||self).thi=t.thi||{},t.thi.ng=t.thi.ng||{},t.thi.ng.binary={}))}(this,(function(t){"use strict";const e=new Array(33).fill(0).map((t,e)=>Math.pow(2,e)-1),i=t=>(t-=t>>>1&1431655765,t=(858993459&t)+(t>>>2&858993459),16843009*(t+(t>>>4)&252645135)>>>24),n=(t,i)=>(~e[t]&e[i])>>>0,o=(t,i)=>(i&e[t])>>>0,a=new Float32Array(1),r=new Int32Array(a.buffer),l=new Uint32Array(a.buffer),s=t=>(a[0]=t,r[0]),b=(t,e)=>t>>>(3-e<<3)&255,f=(t,e)=>t>>>(7-e<<2)&15;t.MASKS=e,t.align=(t,e)=>(e--,t+e&~e),t.bitAnd=(t,e,i)=>o(t,e&i),t.bitAoi21=(t,e,i,n)=>o(t,~(e|i&n)),t.bitAoi22=(t,e,i,n,a)=>o(t,~(e&i|n&a)),t.bitClear=(t,e)=>(t&~(1<<e))>>>0,t.bitClearWindow=(t,e,i)=>t&~n(e,i),t.bitDemux=(t,e,i,n)=>[o(t,e&~n),o(t,i&n)],t.bitFlip=(t,e)=>(t^1<<e)>>>0,t.bitImply=(t,e,i)=>o(t,~e|i),t.bitMux=(t,e,i,n)=>o(t,e&~n|i&n),t.bitNand=(t,e,i)=>o(t,~(e&i)),t.bitNor=(t,e,i)=>o(t,~(e&i)),t.bitNot=(t,e)=>o(t,~e),t.bitOai21=(t,e,i,n)=>o(t,~(e&(i|n))),t.bitOai22=(t,e,i,n,a)=>o(t,~((e|i)&(n|a))),t.bitOr=(t,e,i)=>o(t,e|i),t.bitSet=(t,e)=>(t|1<<e)>>>0,t.bitSetWindow=(t,e,i,o)=>{const a=n(i,o);return t&~a|e<<(1<<i)&a},t.bitSize=t=>Math.ceil(Math.log(t)/Math.LN2),t.bitXnor=(t,e,i)=>o(t,~(e^i)),t.bitXor=(t,e,i)=>o(t,e^i),t.ceilPow2=t=>(t+=0===t,--t,t|=t>>>1,t|=t>>>2,t|=t>>>4,t|=t>>>8,(t|=t>>>16)+1),t.clz32=t=>0!==t?31-(Math.log(t>>>0)/Math.LN2|0):32,t.ctz32=t=>{let e=32;return(t&=-t)&&e--,65535&t&&(e-=16),16711935&t&&(e-=8),252645135&t&&(e-=4),858993459&t&&(e-=2),1431655765&t&&(e-=1),e},t.decodeGray32=t=>(t^=t>>>16,t^=t>>>8,t^=t>>>4,t^=t>>>2,(t^=t>>>1)>>>0),t.defMask=n,t.encodeGray32=t=>(t^t>>>1)>>>0,t.flipBytes=t=>(t>>>24|t>>8&65280|(65280&t)<<8|t<<24)>>>0,t.floatToIntBits=s,t.floatToSortableInt=t=>{-0===t&&(t=0);const e=s(t);return t<0?~e|1<<31:e},t.floatToUintBits=t=>(a[0]=t,l[0]),t.floorPow2=t=>(t|=t>>>1,t|=t>>>2,t|=t>>>4,t|=t>>>8,(t|=t>>>16)-(t>>>1)),t.hammingDist=(t,e)=>i(t^e),t.intBitsToFloat=t=>(r[0]=t,a[0]),t.isAligned=(t,e)=>!(t&e-1),t.isPow2=t=>!(!t||t&t-1),t.lane2=(t,e)=>t>>>(15-e<<1)&3,t.lane4=f,t.lane8=b,t.maskH=(t,i)=>(i&~e[t])>>>0,t.maskL=o,t.popCount=i,t.rotateLeft=(t,e)=>(t<<e|t>>>32-e)>>>0,t.rotateRight=(t,e)=>(t>>>e|t<<32-e)>>>0,t.same4=t=>(t>>4&15)==(15&t),t.same8=t=>(t>>8&255)==(255&t),t.setLane2=(t,e,i)=>{const n=15-i<<1;return(~(3<<n)&t|(3&e)<<n)>>>0},t.setLane4=(t,e,i)=>{const n=7-i<<2;return(~(15<<n)&t|(15&e)<<n)>>>0},t.setLane8=(t,e,i)=>{const n=3-i<<3;return(~(255<<n)&t|(255&e)<<n)>>>0},t.splat16_32=t=>(t&=65535,(t<<16|t)>>>0),t.splat4_24=t=>1118481*(15&t),t.splat4_32=t=>286331153*(15&t)>>>0,t.splat8_24=t=>65793*(255&t),t.splat8_32=t=>16843009*(255&t)>>>0,t.swizzle4=(t,e,i,n,o,a,r,l,s)=>(f(t,e)<<28|f(t,i)<<24|f(t,n)<<20|f(t,o)<<16|f(t,a)<<12|f(t,r)<<8|f(t,l)<<4|f(t,s))>>>0,t.swizzle8=(t,e,i,n,o)=>(b(t,e)<<24|b(t,i)<<16|b(t,n)<<8|b(t,o))>>>0,t.uintBitsToFloat=t=>(l[0]=t,a[0]),Object.defineProperty(t,"__esModule",{value:!0})})); | ||
!function(t,e){"object"==typeof exports&&"undefined"!=typeof module?e(exports):"function"==typeof define&&define.amd?define(["exports"],e):e(((t=t||self).thi=t.thi||{},t.thi.ng=t.thi.ng||{},t.thi.ng.binary={}))}(this,(function(t){"use strict";const e=new Array(33).fill(0).map((t,e)=>Math.pow(2,e)-1),i=t=>16843009*((t=(858993459&(t-=t>>>1&1431655765))+(t>>>2&858993459))+(t>>>4)&252645135)>>>24,n=(t,i)=>(~e[t]&e[i])>>>0,o=(t,i)=>(i&e[t])>>>0,a=new Float32Array(1),r=new Int32Array(a.buffer),l=new Uint32Array(a.buffer),s=t=>(a[0]=t,r[0]),b=(t,e)=>t>>>(3-e<<3)&255,f=(t,e)=>t>>>(7-e<<2)&15;t.MASKS=e,t.align=(t,e)=>t+--e&~e,t.bitAnd=(t,e,i)=>o(t,e&i),t.bitAoi21=(t,e,i,n)=>o(t,~(e|i&n)),t.bitAoi22=(t,e,i,n,a)=>o(t,~(e&i|n&a)),t.bitClear=(t,e)=>(t&~(1<<e))>>>0,t.bitClearWindow=(t,e,i)=>t&~n(e,i),t.bitDemux=(t,e,i,n)=>[o(t,e&~n),o(t,i&n)],t.bitFlip=(t,e)=>(t^1<<e)>>>0,t.bitImply=(t,e,i)=>o(t,~e|i),t.bitMux=(t,e,i,n)=>o(t,e&~n|i&n),t.bitNand=(t,e,i)=>o(t,~(e&i)),t.bitNor=(t,e,i)=>o(t,~(e&i)),t.bitNot=(t,e)=>o(t,~e),t.bitOai21=(t,e,i,n)=>o(t,~(e&(i|n))),t.bitOai22=(t,e,i,n,a)=>o(t,~((e|i)&(n|a))),t.bitOr=(t,e,i)=>o(t,e|i),t.bitSet=(t,e)=>(t|1<<e)>>>0,t.bitSetWindow=(t,e,i,o)=>{const a=n(i,o);return t&~a|e<<(1<<i)&a},t.bitSize=t=>t>1?Math.ceil(Math.log2(t)):0,t.bitXnor=(t,e,i)=>o(t,~(e^i)),t.bitXor=(t,e,i)=>o(t,e^i),t.ceilPow2=t=>(t+=0===t,--t,t|=t>>>1,t|=t>>>2,t|=t>>>4,t|=t>>>8,(t|=t>>>16)+1),t.clz32=t=>0!==t?31-(Math.log(t>>>0)/Math.LN2|0):32,t.ctz32=t=>{let e=32;return(t&=-t)&&e--,65535&t&&(e-=16),16711935&t&&(e-=8),252645135&t&&(e-=4),858993459&t&&(e-=2),1431655765&t&&(e-=1),e},t.decodeGray32=t=>(t^=t>>>16,t^=t>>>8,t^=t>>>4,t^=t>>>2,(t^=t>>>1)>>>0),t.defMask=n,t.encodeGray32=t=>(t^t>>>1)>>>0,t.flipBytes=t=>(t>>>24|t>>8&65280|(65280&t)<<8|t<<24)>>>0,t.floatToIntBits=s,t.floatToSortableInt=t=>{-0===t&&(t=0);const e=s(t);return t<0?~e|1<<31:e},t.floatToUintBits=t=>(a[0]=t,l[0]),t.floorPow2=t=>(t|=t>>>1,t|=t>>>2,t|=t>>>4,t|=t>>>8,(t|=t>>>16)-(t>>>1)),t.hammingDist=(t,e)=>i(t^e),t.intBitsToFloat=t=>(r[0]=t,a[0]),t.isAligned=(t,e)=>!(t&e-1),t.isPow2=t=>!(!t||t&t-1),t.lane2=(t,e)=>t>>>(15-e<<1)&3,t.lane4=f,t.lane8=b,t.maskH=(t,i)=>(i&~e[t])>>>0,t.maskL=o,t.popCount=i,t.rotateLeft=(t,e)=>(t<<e|t>>>32-e)>>>0,t.rotateRight=(t,e)=>(t>>>e|t<<32-e)>>>0,t.same4=t=>(t>>4&15)==(15&t),t.same8=t=>(t>>8&255)==(255&t),t.setLane2=(t,e,i)=>{const n=15-i<<1;return(~(3<<n)&t|(3&e)<<n)>>>0},t.setLane4=(t,e,i)=>{const n=7-i<<2;return(~(15<<n)&t|(15&e)<<n)>>>0},t.setLane8=(t,e,i)=>{const n=3-i<<3;return(~(255<<n)&t|(255&e)<<n)>>>0},t.splat16_32=t=>((t&=65535)<<16|t)>>>0,t.splat4_24=t=>1118481*(15&t),t.splat4_32=t=>286331153*(15&t)>>>0,t.splat8_24=t=>65793*(255&t),t.splat8_32=t=>16843009*(255&t)>>>0,t.swizzle4=(t,e,i,n,o,a,r,l,s)=>(f(t,e)<<28|f(t,i)<<24|f(t,n)<<20|f(t,o)<<16|f(t,a)<<12|f(t,r)<<8|f(t,l)<<4|f(t,s))>>>0,t.swizzle8=(t,e,i,n,o)=>(b(t,e)<<24|b(t,i)<<16|b(t,n)<<8|b(t,o))>>>0,t.uintBitsToFloat=t=>(l[0]=t,a[0]),Object.defineProperty(t,"__esModule",{value:!0})})); |
@@ -15,1 +15,2 @@ export declare const bitNot: (n: number, x: number) => number; | ||
export declare const bitDemux: (n: number, a: number, b: number, s: number) => [number, number]; | ||
//# sourceMappingURL=logic.d.ts.map |
@@ -5,3 +5,4 @@ /** | ||
* | ||
* ``` | ||
* @example | ||
* ```ts | ||
* defMask(1,31).toString(16) // 7ffffffe | ||
@@ -11,4 +12,4 @@ * defMask(3,8).toString(16) // f8 | ||
* | ||
* @param a | ||
* @param b | ||
* @param a - first bit | ||
* @param b - last bit | ||
*/ | ||
@@ -19,4 +20,4 @@ export declare const defMask: (a: number, b: number) => number; | ||
* | ||
* @param n | ||
* @param x | ||
* @param n - number of LSB bits | ||
* @param x - value | ||
*/ | ||
@@ -27,5 +28,6 @@ export declare const maskL: (n: number, x: number) => number; | ||
* | ||
* @param n | ||
* @param x | ||
* @param n - number of MSB bits | ||
* @param x - value | ||
*/ | ||
export declare const maskH: (n: number, x: number) => number; | ||
//# sourceMappingURL=mask.d.ts.map |
15
mask.js
@@ -6,3 +6,4 @@ import { MASKS } from "./api"; | ||
* | ||
* ``` | ||
* @example | ||
* ```ts | ||
* defMask(1,31).toString(16) // 7ffffffe | ||
@@ -12,4 +13,4 @@ * defMask(3,8).toString(16) // f8 | ||
* | ||
* @param a | ||
* @param b | ||
* @param a - first bit | ||
* @param b - last bit | ||
*/ | ||
@@ -20,4 +21,4 @@ export const defMask = (a, b) => (~MASKS[a] & MASKS[b]) >>> 0; | ||
* | ||
* @param n | ||
* @param x | ||
* @param n - number of LSB bits | ||
* @param x - value | ||
*/ | ||
@@ -28,5 +29,5 @@ export const maskL = (n, x) => (x & MASKS[n]) >>> 0; | ||
* | ||
* @param n | ||
* @param x | ||
* @param n - number of MSB bits | ||
* @param x - value | ||
*/ | ||
export const maskH = (n, x) => (x & ~MASKS[n]) >>> 0; |
{ | ||
"name": "@thi.ng/binary", | ||
"version": "1.2.0", | ||
"version": "1.2.1", | ||
"description": "50+ assorted binary / bitwise operations, conversions, utilities", | ||
@@ -26,13 +26,15 @@ "module": "./index.js", | ||
"doc": "node_modules/.bin/typedoc --mode modules --out doc src", | ||
"doc:ae": "mkdir -p .ae/doc .ae/temp && node_modules/.bin/api-extractor run --local --verbose", | ||
"pub": "yarn build:release && yarn publish --access public" | ||
}, | ||
"devDependencies": { | ||
"@istanbuljs/nyc-config-typescript": "^0.1.3", | ||
"@types/mocha": "^5.2.6", | ||
"@types/node": "^12.12.11", | ||
"mocha": "^6.2.2", | ||
"nyc": "^14.0.0", | ||
"ts-node": "^8.5.2", | ||
"typedoc": "^0.15.2", | ||
"typescript": "^3.7.2" | ||
"@istanbuljs/nyc-config-typescript": "^1.0.1", | ||
"@microsoft/api-extractor": "^7.7.7", | ||
"@types/mocha": "^5.2.7", | ||
"@types/node": "^13.5.0", | ||
"mocha": "^7.0.0", | ||
"nyc": "^15.0.0", | ||
"ts-node": "^8.6.2", | ||
"typedoc": "^0.16.8", | ||
"typescript": "^3.7.5" | ||
}, | ||
@@ -56,3 +58,3 @@ "keywords": [ | ||
"sideEffects": false, | ||
"gitHead": "36c4d9e967bd80ccdbfa0f4a42f594080f95f105" | ||
"gitHead": "93d8af817724c1c5b06d80ffa2492fe5b4fb7bc4" | ||
} |
@@ -5,1 +5,2 @@ import { Pow2 } from "./api"; | ||
export declare const floorPow2: (x: number) => number; | ||
//# sourceMappingURL=pow.d.ts.map |
@@ -35,2 +35,4 @@ <!-- This file is generated - DO NOT EDIT! --> | ||
Package sizes (gzipped): ESM: 1.2KB / CJS: 1.5KB / UMD: 1.3KB | ||
## Dependencies | ||
@@ -43,3 +45,3 @@ | ||
Several demos in this repo's | ||
[/examples](https://github.com/thi-ng/umbrella/tree/master/examples) | ||
[/examples](https://github.com/thi-ng/umbrella/tree/develop/examples) | ||
directory are using this package. | ||
@@ -51,5 +53,5 @@ | ||
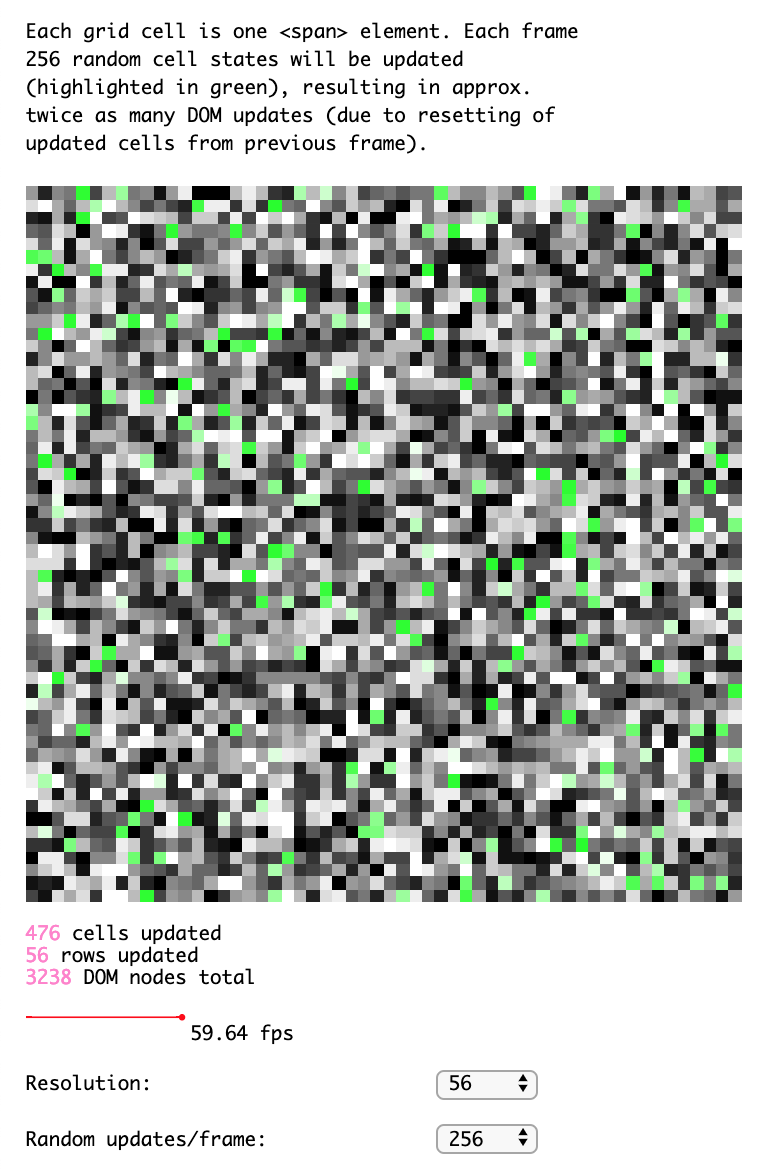 | ||
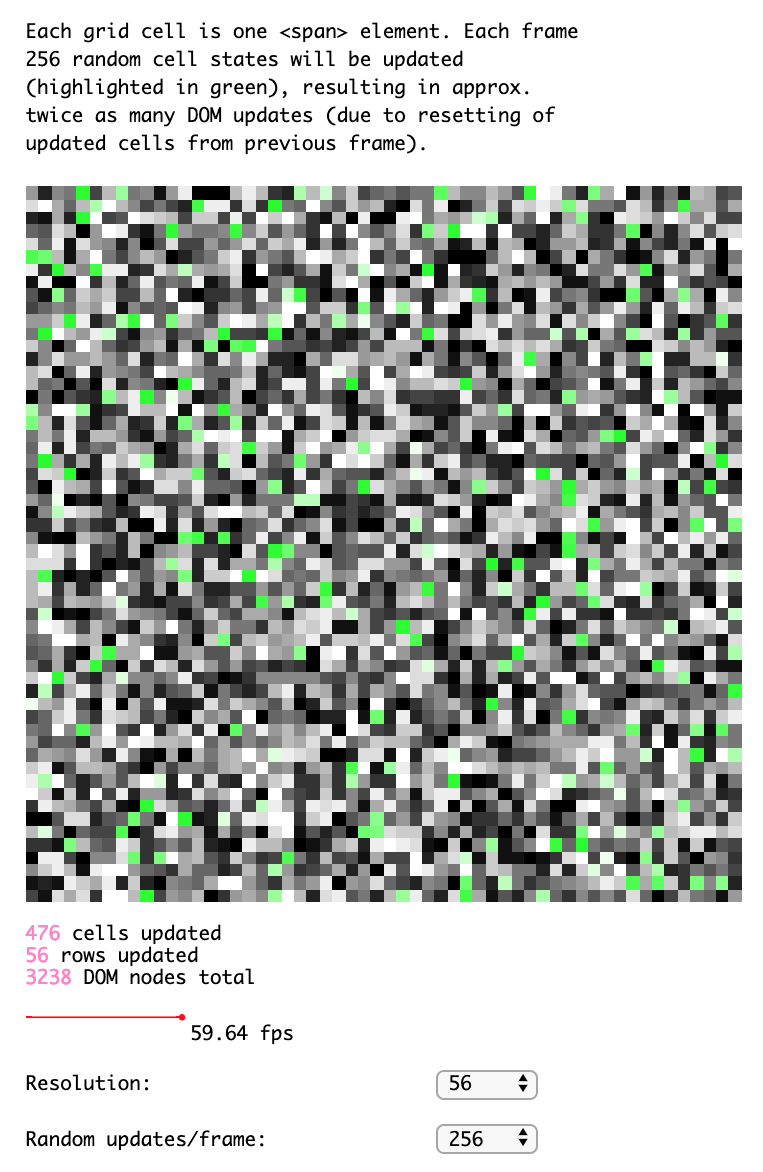 | ||
[Live demo](https://demo.thi.ng/umbrella/hdom-benchmark2/) | [Source](https://github.com/thi-ng/umbrella/tree/master/examples/hdom-benchmark2) | ||
[Live demo](https://demo.thi.ng/umbrella/hdom-benchmark2/) | [Source](https://github.com/thi-ng/umbrella/tree/develop/examples/hdom-benchmark2) | ||
@@ -68,2 +70,2 @@ ## API | ||
© 2016 - 2019 Karsten Schmidt // Apache Software License 2.0 | ||
© 2016 - 2020 Karsten Schmidt // Apache Software License 2.0 |
@@ -30,2 +30,4 @@ # ${pkg.name} | ||
${pkg.size} | ||
## Dependencies | ||
@@ -32,0 +34,0 @@ |
@@ -5,4 +5,4 @@ import { Bit } from "./api"; | ||
* | ||
* @param x | ||
* @param n | ||
* @param x - value | ||
* @param n - rotation step | ||
*/ | ||
@@ -13,5 +13,6 @@ export declare const rotateLeft: (x: number, n: Bit) => number; | ||
* | ||
* @param x | ||
* @param n | ||
* @param x - value | ||
* @param n - rotation step | ||
*/ | ||
export declare const rotateRight: (x: number, n: Bit) => number; | ||
//# sourceMappingURL=rotate.d.ts.map |
/** | ||
* Rotates `x` `n` bits to the left. | ||
* | ||
* @param x | ||
* @param n | ||
* @param x - value | ||
* @param n - rotation step | ||
*/ | ||
@@ -11,5 +11,5 @@ export const rotateLeft = (x, n) => ((x << n) | (x >>> (32 - n))) >>> 0; | ||
* | ||
* @param x | ||
* @param n | ||
* @param x - value | ||
* @param n - rotation step | ||
*/ | ||
export const rotateRight = (x, n) => ((x >>> n) | (x << (32 - n))) >>> 0; |
/** | ||
* Repeats lowest nibble of `x` as 24 bit uint. | ||
* | ||
* @param x | ||
* @param x - | ||
*/ | ||
@@ -10,3 +10,3 @@ export declare const splat4_24: (x: number) => number; | ||
* | ||
* @param x | ||
* @param x - | ||
*/ | ||
@@ -17,3 +17,3 @@ export declare const splat4_32: (x: number) => number; | ||
* | ||
* @param x | ||
* @param x - | ||
*/ | ||
@@ -24,3 +24,3 @@ export declare const splat8_24: (x: number) => number; | ||
* | ||
* @param x | ||
* @param x - | ||
*/ | ||
@@ -31,3 +31,3 @@ export declare const splat8_32: (x: number) => number; | ||
* | ||
* @param x | ||
* @param x - | ||
*/ | ||
@@ -38,3 +38,3 @@ export declare const splat16_32: (x: number) => number; | ||
* | ||
* @param x | ||
* @param x - | ||
*/ | ||
@@ -45,4 +45,5 @@ export declare const same4: (x: number) => boolean; | ||
* | ||
* @param x | ||
* @param x - | ||
*/ | ||
export declare const same8: (x: number) => boolean; | ||
//# sourceMappingURL=splat.d.ts.map |
14
splat.js
/** | ||
* Repeats lowest nibble of `x` as 24 bit uint. | ||
* | ||
* @param x | ||
* @param x - | ||
*/ | ||
@@ -10,3 +10,3 @@ export const splat4_24 = (x) => (x & 0xf) * 0x111111; | ||
* | ||
* @param x | ||
* @param x - | ||
*/ | ||
@@ -17,3 +17,3 @@ export const splat4_32 = (x) => ((x & 0xf) * 0x11111111) >>> 0; | ||
* | ||
* @param x | ||
* @param x - | ||
*/ | ||
@@ -24,3 +24,3 @@ export const splat8_24 = (x) => (x & 0xff) * 0x010101; | ||
* | ||
* @param x | ||
* @param x - | ||
*/ | ||
@@ -31,3 +31,3 @@ export const splat8_32 = (x) => ((x & 0xff) * 0x01010101) >>> 0; | ||
* | ||
* @param x | ||
* @param x - | ||
*/ | ||
@@ -38,3 +38,3 @@ export const splat16_32 = (x) => ((x &= 0xffff), ((x << 16) | x) >>> 0); | ||
* | ||
* @param x | ||
* @param x - | ||
*/ | ||
@@ -45,4 +45,4 @@ export const same4 = (x) => ((x >> 4) & 0xf) === (x & 0xf); | ||
* | ||
* @param x | ||
* @param x - | ||
*/ | ||
export const same8 = (x) => ((x >> 8) & 0xff) === (x & 0xff); |
@@ -10,4 +10,4 @@ import { Lane2, Lane4, Lane8 } from "./api"; | ||
* | ||
* @param x | ||
* @param lane | ||
* @param x - | ||
* @param lane - lane ID enum | ||
*/ | ||
@@ -27,4 +27,4 @@ export declare const lane8: (x: number, lane: Lane8) => number; | ||
* | ||
* @param x | ||
* @param lane | ||
* @param x - | ||
* @param lane - lane ID enum | ||
*/ | ||
@@ -36,7 +36,7 @@ export declare const lane4: (x: number, lane: Lane4) => number; | ||
* | ||
* @see lane8 | ||
* {@link lane8} | ||
* | ||
* @param x | ||
* @param y | ||
* @param lane | ||
* @param x - | ||
* @param y - | ||
* @param lane - lane ID enum | ||
*/ | ||
@@ -47,7 +47,7 @@ export declare const setLane8: (x: number, y: number, lane: Lane8) => number; | ||
* | ||
* @see lane4 | ||
* {@link lane4} | ||
* | ||
* @param x | ||
* @param y | ||
* @param lane | ||
* @param x - | ||
* @param y - | ||
* @param lane - lane ID enum | ||
*/ | ||
@@ -58,7 +58,7 @@ export declare const setLane4: (x: number, y: number, lane: Lane4) => number; | ||
* | ||
* @see lane2 | ||
* {@link lane2} | ||
* | ||
* @param x | ||
* @param y | ||
* @param lane | ||
* @param x - | ||
* @param y - | ||
* @param lane - lane ID enum | ||
*/ | ||
@@ -69,3 +69,4 @@ export declare const setLane2: (x: number, y: number, lane: Lane2) => number; | ||
* | ||
* ``` | ||
* @example | ||
* ```ts | ||
* swizzle(0x12345678, 3, 2, 1, 0) // 0x78563412 | ||
@@ -76,7 +77,7 @@ * swizzle(0x12345678, 1, 0, 3, 2) // 0x34127856 | ||
* | ||
* @param x | ||
* @param a | ||
* @param b | ||
* @param c | ||
* @param d | ||
* @param x - value | ||
* @param a - lane ID enum | ||
* @param b - lane ID enum | ||
* @param c - lane ID enum | ||
* @param d - lane ID enum | ||
*/ | ||
@@ -86,11 +87,11 @@ export declare const swizzle8: (x: number, a: Lane8, b: Lane8, c: Lane8, d: Lane8) => number; | ||
* | ||
* @param x | ||
* @param a | ||
* @param b | ||
* @param c | ||
* @param d | ||
* @param e | ||
* @param f | ||
* @param g | ||
* @param h | ||
* @param x - value | ||
* @param a - lane ID enum | ||
* @param b - lane ID enum | ||
* @param c - lane ID enum | ||
* @param d - lane ID enum | ||
* @param e - lane ID enum | ||
* @param f - lane ID enum | ||
* @param g - lane ID enum | ||
* @param h - lane ID enum | ||
*/ | ||
@@ -101,4 +102,5 @@ export declare const swizzle4: (x: number, a: Lane4, b: Lane4, c: Lane4, d: Lane4, e: Lane4, f: Lane4, g: Lane4, h: Lane4) => number; | ||
* | ||
* @param x | ||
* @param x - | ||
*/ | ||
export declare const flipBytes: (x: number) => number; | ||
//# sourceMappingURL=swizzle.d.ts.map |
@@ -9,4 +9,4 @@ /** | ||
* | ||
* @param x | ||
* @param lane | ||
* @param x - | ||
* @param lane - lane ID enum | ||
*/ | ||
@@ -26,4 +26,4 @@ export const lane8 = (x, lane) => (x >>> ((3 - lane) << 3)) & 0xff; | ||
* | ||
* @param x | ||
* @param lane | ||
* @param x - | ||
* @param lane - lane ID enum | ||
*/ | ||
@@ -35,7 +35,7 @@ export const lane4 = (x, lane) => (x >>> ((7 - lane) << 2)) & 0xf; | ||
* | ||
* @see lane8 | ||
* {@link lane8} | ||
* | ||
* @param x | ||
* @param y | ||
* @param lane | ||
* @param x - | ||
* @param y - | ||
* @param lane - lane ID enum | ||
*/ | ||
@@ -49,7 +49,7 @@ export const setLane8 = (x, y, lane) => { | ||
* | ||
* @see lane4 | ||
* {@link lane4} | ||
* | ||
* @param x | ||
* @param y | ||
* @param lane | ||
* @param x - | ||
* @param y - | ||
* @param lane - lane ID enum | ||
*/ | ||
@@ -63,7 +63,7 @@ export const setLane4 = (x, y, lane) => { | ||
* | ||
* @see lane2 | ||
* {@link lane2} | ||
* | ||
* @param x | ||
* @param y | ||
* @param lane | ||
* @param x - | ||
* @param y - | ||
* @param lane - lane ID enum | ||
*/ | ||
@@ -77,3 +77,4 @@ export const setLane2 = (x, y, lane) => { | ||
* | ||
* ``` | ||
* @example | ||
* ```ts | ||
* swizzle(0x12345678, 3, 2, 1, 0) // 0x78563412 | ||
@@ -84,7 +85,7 @@ * swizzle(0x12345678, 1, 0, 3, 2) // 0x34127856 | ||
* | ||
* @param x | ||
* @param a | ||
* @param b | ||
* @param c | ||
* @param d | ||
* @param x - value | ||
* @param a - lane ID enum | ||
* @param b - lane ID enum | ||
* @param c - lane ID enum | ||
* @param d - lane ID enum | ||
*/ | ||
@@ -98,11 +99,11 @@ export const swizzle8 = (x, a, b, c, d) => ((lane8(x, a) << 24) | | ||
* | ||
* @param x | ||
* @param a | ||
* @param b | ||
* @param c | ||
* @param d | ||
* @param e | ||
* @param f | ||
* @param g | ||
* @param h | ||
* @param x - value | ||
* @param a - lane ID enum | ||
* @param b - lane ID enum | ||
* @param c - lane ID enum | ||
* @param d - lane ID enum | ||
* @param e - lane ID enum | ||
* @param f - lane ID enum | ||
* @param g - lane ID enum | ||
* @param h - lane ID enum | ||
*/ | ||
@@ -121,4 +122,4 @@ export const swizzle4 = (x, a, b, c, d, e, f, g, h) => ((lane4(x, a) << 28) | | ||
* | ||
* @param x | ||
* @param x - | ||
*/ | ||
export const flipBytes = (x) => ((x >>> 24) | ((x >> 8) & 0xff00) | ((x & 0xff00) << 8) | (x << 24)) >>> 0; |
Sorry, the diff of this file is not supported yet
Sorry, the diff of this file is not supported yet
License Policy Violation
LicenseThis package is not allowed per your license policy. Review the package's license to ensure compliance.
Found 1 instance in 1 package
License Policy Violation
LicenseThis package is not allowed per your license policy. Review the package's license to ensure compliance.
Found 1 instance in 1 package
877
68
67378
9
35