What is @thi.ng/compare?
@thi.ng/compare is a utility library for various comparison operations in JavaScript. It provides a collection of comparator functions for different data types and use cases, making it easier to perform sorting, equality checks, and other comparison-based operations.
What are @thi.ng/compare's main functionalities?
Basic Comparators
Provides basic comparator functions for general use, including ascending and descending numerical comparisons.
const { compare, compareNumAsc, compareNumDesc } = require('@thi.ng/compare');
console.log(compare(5, 10)); // -1
console.log(compareNumAsc(5, 10)); // -1
console.log(compareNumDesc(5, 10)); // 1
String Comparators
Includes specialized comparators for string values, with options for case-sensitive and case-insensitive comparisons.
const { compareStr, compareStrCaseInsensitive } = require('@thi.ng/compare');
console.log(compareStr('apple', 'banana')); // -1
console.log(compareStrCaseInsensitive('Apple', 'apple')); // 0
Array Comparators
Allows for comparison of arrays, element by element, to determine their order or equality.
const { compareArrays } = require('@thi.ng/compare');
console.log(compareArrays([1, 2, 3], [1, 2, 4])); // -1
console.log(compareArrays([1, 2, 3], [1, 2, 3])); // 0
Object Comparators
Provides comparators for objects based on specific keys, useful for sorting arrays of objects.
const { compareByKey } = require('@thi.ng/compare');
const obj1 = { id: 1, name: 'Alice' };
const obj2 = { id: 2, name: 'Bob' };
console.log(compareByKey('id')(obj1, obj2)); // -1
Other packages similar to @thi.ng/compare
lodash
Lodash is a popular utility library that provides a wide range of functions for common programming tasks, including comparison operations. It offers functions like _.isEqual for deep equality checks and _.sortBy for sorting collections. Compared to @thi.ng/compare, Lodash is more comprehensive but also larger in size.
fast-deep-equal
fast-deep-equal is a small and fast library for deep equality checks. It is highly optimized for performance and is often used when deep comparison of objects or arrays is needed. Unlike @thi.ng/compare, it focuses solely on deep equality and does not provide other comparison utilities.
ramda
Ramda is a functional programming library for JavaScript that includes a variety of utility functions, including comparison functions like R.equals and R.sort. It emphasizes immutability and functional programming principles. While it offers similar comparison functionalities, it is part of a broader functional programming toolkit.


This project is part of the
@thi.ng/umbrella monorepo.
About
Comparators with optional support for types implementing the
@thi.ng/api
ICompare
interface.
Since v1.2.0 additional higher-order comparators are included, e.g. to
reverse the ordering of an existing comparator and allow hierarchical
sorting by multiple keys/dimensions, each with their own optional
comparator. See examples below.
Status
STABLE - used in production
Search or submit any issues for this package
Installation
yarn add @thi.ng/compare
// ES module
<script type="module" src="https://unpkg.com/@thi.ng/compare?module" crossorigin></script>
// UMD
<script src="https://unpkg.com/@thi.ng/compare/lib/index.umd.js" crossorigin></script>
Package sizes (gzipped, pre-treeshake): ESM: 396 bytes / CJS: 471 bytes / UMD: 519 bytes
Dependencies
Usage examples
Several demos in this repo's
/examples
directory are using this package.
A selection:
Screenshot | Description | Live demo | Source |
---|
| Full umbrella repo doc string search w/ paginated results | Demo | Source |
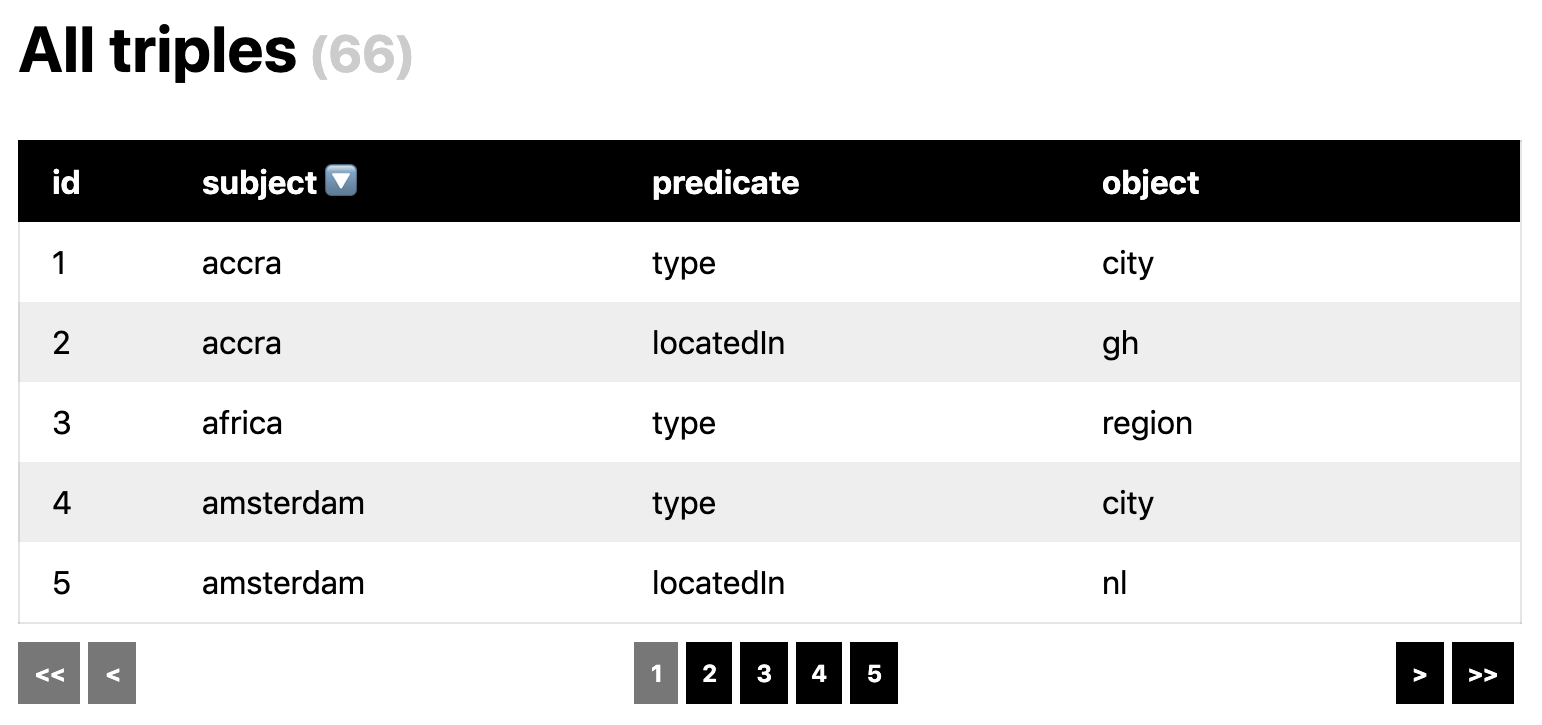 | Triple store query results & sortable table | Demo | Source |
API
Generated API docs
ICompare support
import { ICompare } from "@thi.ng/api";
import { compare } from "@thi.ng/compare";
class Foo implements ICompare<Foo> {
x: number;
constructor(x: number) {
this.x = x;
}
compare(o: Foo) {
return compare(this.x, o.x);
}
}
compare(new Foo(1), new Foo(2));
Cluster sort w/ multiple sort keys
Key-based object comparison is supported for 1 - 4 keys / dimensions.
import * as cmp from "@thi.ng/compare";
const src = [
{ id: "charlie", age: 66 },
{ id: "bart", age: 42 },
{ id: "alice", age: 23 },
{ id: "dora", age: 11 },
];
[...src].sort(cmp.compareByKeys2("id", "age"));
[...src].sort(cmp.compareByKeys2("age", "id"));
[...src].sort(cmp.compareByKeys2("age", "id", cmp.compareNumDesc));
[...src].sort(cmp.compareByKeys2("age", "id", cmp.compare, cmp.reverse(cmp.compare)));
Authors
Karsten Schmidt
If this project contributes to an academic publication, please cite it as:
@misc{thing-compare,
title = "@thi.ng/compare",
author = "Karsten Schmidt",
note = "https://thi.ng/compare",
year = 2016
}
License
© 2016 - 2021 Karsten Schmidt // Apache Software License 2.0