@thi.ng/math
Advanced tools
Comparing version 1.5.1 to 1.6.0
export declare const absDiff: (x: number, y: number) => number; | ||
export declare const sign: (x: number, eps?: number) => 1 | -1 | 0; | ||
//# sourceMappingURL=abs.d.ts.map |
/** | ||
* Returns vector of `[sin(theta)*n, cos(theta)*n]`. | ||
* | ||
* @param theta | ||
* @param n | ||
* @param theta - | ||
* @param n - | ||
*/ | ||
@@ -11,4 +11,4 @@ export declare const sincos: (theta: number, n?: number) => number[]; | ||
* | ||
* @param theta | ||
* @param n | ||
* @param theta - | ||
* @param n - | ||
*/ | ||
@@ -19,3 +19,3 @@ export declare const cossin: (theta: number, n?: number) => number[]; | ||
* | ||
* @param theta | ||
* @param theta - | ||
*/ | ||
@@ -28,4 +28,4 @@ export declare const absTheta: (theta: number) => number; | ||
* | ||
* @param a | ||
* @param b | ||
* @param a - | ||
* @param b - | ||
*/ | ||
@@ -36,4 +36,4 @@ export declare const angleDist: (a: number, b: number) => number; | ||
* | ||
* @param y | ||
* @param x | ||
* @param y - | ||
* @param x - | ||
*/ | ||
@@ -44,3 +44,3 @@ export declare const atan2Abs: (y: number, x: number) => number; | ||
* | ||
* @param theta | ||
* @param theta - | ||
*/ | ||
@@ -51,3 +51,3 @@ export declare const quadrant: (theta: number) => number; | ||
* | ||
* @param theta angle in radians | ||
* @param theta - angle in radians | ||
*/ | ||
@@ -58,3 +58,3 @@ export declare const deg: (theta: number) => number; | ||
* | ||
* @param theta angle in degrees | ||
* @param theta - angle in degrees | ||
*/ | ||
@@ -65,3 +65,3 @@ export declare const rad: (theta: number) => number; | ||
* | ||
* @param theta angle in radians | ||
* @param theta - angle in radians | ||
*/ | ||
@@ -72,3 +72,3 @@ export declare const csc: (theta: number) => number; | ||
* | ||
* @param theta angle in radians | ||
* @param theta - angle in radians | ||
*/ | ||
@@ -79,3 +79,3 @@ export declare const sec: (theta: number) => number; | ||
* | ||
* @param theta angle in radians | ||
* @param theta - angle in radians | ||
*/ | ||
@@ -87,5 +87,5 @@ export declare const cot: (theta: number) => number; | ||
* | ||
* @param a | ||
* @param b | ||
* @param gamma | ||
* @param a - | ||
* @param b - | ||
* @param gamma - | ||
*/ | ||
@@ -96,7 +96,7 @@ export declare const loc: (a: number, b: number, gamma: number) => number; | ||
* | ||
* @param x | ||
* @param x - | ||
*/ | ||
export declare const normCos: (x: number) => number; | ||
/** | ||
* Fast cosine approximation using `normCos()` (polynomial). Max. error | ||
* Fast cosine approximation using {@link normCos} (polynomial). Max. error | ||
* ~0.00059693 | ||
@@ -106,10 +106,11 @@ * | ||
* | ||
* @param theta in radians | ||
* @param theta - in radians | ||
*/ | ||
export declare const fastCos: (theta: number) => number; | ||
/** | ||
* @see fastCos | ||
* {@link fastCos} | ||
* | ||
* @param theta in radians | ||
* @param theta - in radians | ||
*/ | ||
export declare const fastSin: (theta: number) => number; | ||
//# sourceMappingURL=angle.d.ts.map |
46
angle.js
@@ -5,4 +5,4 @@ import { DEG2RAD, HALF_PI, INV_HALF_PI, PI, RAD2DEG, TAU } from "./api"; | ||
* | ||
* @param theta | ||
* @param n | ||
* @param theta - | ||
* @param n - | ||
*/ | ||
@@ -16,4 +16,4 @@ export const sincos = (theta, n = 1) => [ | ||
* | ||
* @param theta | ||
* @param n | ||
* @param theta - | ||
* @param n - | ||
*/ | ||
@@ -27,3 +27,3 @@ export const cossin = (theta, n = 1) => [ | ||
* | ||
* @param theta | ||
* @param theta - | ||
*/ | ||
@@ -36,4 +36,4 @@ export const absTheta = (theta) => ((theta %= TAU), theta < 0 ? TAU + theta : theta); | ||
* | ||
* @param a | ||
* @param b | ||
* @param a - | ||
* @param b - | ||
*/ | ||
@@ -44,4 +44,4 @@ export const angleDist = (a, b) => absInnerAngle(absTheta((b % TAU) - (a % TAU))); | ||
* | ||
* @param y | ||
* @param x | ||
* @param y - | ||
* @param x - | ||
*/ | ||
@@ -52,3 +52,3 @@ export const atan2Abs = (y, x) => absTheta(Math.atan2(y, x)); | ||
* | ||
* @param theta | ||
* @param theta - | ||
*/ | ||
@@ -59,3 +59,3 @@ export const quadrant = (theta) => (absTheta(theta) * INV_HALF_PI) | 0; | ||
* | ||
* @param theta angle in radians | ||
* @param theta - angle in radians | ||
*/ | ||
@@ -66,3 +66,3 @@ export const deg = (theta) => theta * RAD2DEG; | ||
* | ||
* @param theta angle in degrees | ||
* @param theta - angle in degrees | ||
*/ | ||
@@ -73,3 +73,3 @@ export const rad = (theta) => theta * DEG2RAD; | ||
* | ||
* @param theta angle in radians | ||
* @param theta - angle in radians | ||
*/ | ||
@@ -80,3 +80,3 @@ export const csc = (theta) => 1 / Math.sin(theta); | ||
* | ||
* @param theta angle in radians | ||
* @param theta - angle in radians | ||
*/ | ||
@@ -87,3 +87,3 @@ export const sec = (theta) => 1 / Math.cos(theta); | ||
* | ||
* @param theta angle in radians | ||
* @param theta - angle in radians | ||
*/ | ||
@@ -95,5 +95,5 @@ export const cot = (theta) => 1 / Math.tan(theta); | ||
* | ||
* @param a | ||
* @param b | ||
* @param gamma | ||
* @param a - | ||
* @param b - | ||
* @param gamma - | ||
*/ | ||
@@ -104,3 +104,3 @@ export const loc = (a, b, gamma) => Math.sqrt(a * a + b * b - 2 * a * b * Math.cos(gamma)); | ||
* | ||
* @param x | ||
* @param x - | ||
*/ | ||
@@ -116,3 +116,3 @@ export const normCos = (x) => { | ||
/** | ||
* Fast cosine approximation using `normCos()` (polynomial). Max. error | ||
* Fast cosine approximation using {@link normCos} (polynomial). Max. error | ||
* ~0.00059693 | ||
@@ -122,3 +122,3 @@ * | ||
* | ||
* @param theta in radians | ||
* @param theta - in radians | ||
*/ | ||
@@ -140,6 +140,6 @@ export const fastCos = (theta) => { | ||
/** | ||
* @see fastCos | ||
* {@link fastCos} | ||
* | ||
* @param theta in radians | ||
* @param theta - in radians | ||
*/ | ||
export const fastSin = (theta) => fastCos(HALF_PI - theta); |
@@ -40,1 +40,2 @@ export declare const PI: number; | ||
} | ||
//# sourceMappingURL=api.d.ts.map |
@@ -6,2 +6,14 @@ # Change Log | ||
# [1.6.0](https://github.com/thi-ng/umbrella/compare/@thi.ng/math@1.5.1...@thi.ng/math@1.6.0) (2020-01-24) | ||
### Features | ||
* **math:** add clamp05, update wrapOnce, wrap01, wrap11 ([19af252](https://github.com/thi-ng/umbrella/commit/19af2527a3c7afee4f829e36bf06acaeaf045be7)) | ||
* **math:** add expFactor(), update wrap/wrapOnce() ([bb07348](https://github.com/thi-ng/umbrella/commit/bb07348da2e252641c1bc4de1e577451ead3607b)) | ||
## [1.5.1](https://github.com/thi-ng/umbrella/compare/@thi.ng/math@1.5.0...@thi.ng/math@1.5.1) (2019-11-30) | ||
@@ -8,0 +20,0 @@ |
@@ -5,3 +5,4 @@ import { Crossing } from "./api"; | ||
* | ||
* ``` | ||
* @example | ||
* ```ts | ||
* b1 a2 | ||
@@ -13,6 +14,6 @@ * \/ | ||
* | ||
* @param a1 | ||
* @param a2 | ||
* @param b1 | ||
* @param b2 | ||
* @param a1 - | ||
* @param a2 - | ||
* @param b1 - | ||
* @param b2 - | ||
*/ | ||
@@ -23,3 +24,4 @@ export declare const isCrossOver: (a1: number, a2: number, b1: number, b2: number) => boolean; | ||
* | ||
* ``` | ||
* @example | ||
* ```ts | ||
* a1 b2 | ||
@@ -31,23 +33,24 @@ * \/ | ||
* | ||
* @param a1 | ||
* @param a2 | ||
* @param b1 | ||
* @param b2 | ||
* @param a1 - | ||
* @param a2 - | ||
* @param b1 - | ||
* @param b2 - | ||
*/ | ||
export declare const isCrossUnder: (a1: number, a2: number, b1: number, b2: number) => boolean; | ||
/** | ||
* Returns `Crossing` classifier indicating the relationship of line A | ||
* Returns {@link Crossing} classifier indicating the relationship of line A | ||
* to line B. The optional epsilon value is used to determine if both | ||
* lines are considered equal or flat. | ||
* | ||
* @see isCrossUp | ||
* @see isCrossDown | ||
* @see Crossing | ||
* - {@link isCrossOver} | ||
* - {@link isCrossUnder} | ||
* - {@link Crossing} | ||
* | ||
* @param a1 | ||
* @param a2 | ||
* @param b1 | ||
* @param b2 | ||
* @param eps | ||
* @param a1 - | ||
* @param a2 - | ||
* @param b1 - | ||
* @param b2 - | ||
* @param eps - | ||
*/ | ||
export declare const classifyCrossing: (a1: number, a2: number, b1: number, b2: number, eps?: number) => Crossing; | ||
//# sourceMappingURL=crossing.d.ts.map |
@@ -6,3 +6,4 @@ import { EPS } from "./api"; | ||
* | ||
* ``` | ||
* @example | ||
* ```ts | ||
* b1 a2 | ||
@@ -14,6 +15,6 @@ * \/ | ||
* | ||
* @param a1 | ||
* @param a2 | ||
* @param b1 | ||
* @param b2 | ||
* @param a1 - | ||
* @param a2 - | ||
* @param b1 - | ||
* @param b2 - | ||
*/ | ||
@@ -24,3 +25,4 @@ export const isCrossOver = (a1, a2, b1, b2) => a1 < b1 && a2 > b2; | ||
* | ||
* ``` | ||
* @example | ||
* ```ts | ||
* a1 b2 | ||
@@ -32,22 +34,22 @@ * \/ | ||
* | ||
* @param a1 | ||
* @param a2 | ||
* @param b1 | ||
* @param b2 | ||
* @param a1 - | ||
* @param a2 - | ||
* @param b1 - | ||
* @param b2 - | ||
*/ | ||
export const isCrossUnder = (a1, a2, b1, b2) => a1 > b1 && a2 < b2; | ||
/** | ||
* Returns `Crossing` classifier indicating the relationship of line A | ||
* Returns {@link Crossing} classifier indicating the relationship of line A | ||
* to line B. The optional epsilon value is used to determine if both | ||
* lines are considered equal or flat. | ||
* | ||
* @see isCrossUp | ||
* @see isCrossDown | ||
* @see Crossing | ||
* - {@link isCrossOver} | ||
* - {@link isCrossUnder} | ||
* - {@link Crossing} | ||
* | ||
* @param a1 | ||
* @param a2 | ||
* @param b1 | ||
* @param b2 | ||
* @param eps | ||
* @param a1 - | ||
* @param a2 - | ||
* @param b1 - | ||
* @param b2 - | ||
* @param eps - | ||
*/ | ||
@@ -54,0 +56,0 @@ export const classifyCrossing = (a1, a2, b1, b2, eps = EPS) => { |
@@ -10,14 +10,15 @@ /** | ||
* | ||
* @param a left value | ||
* @param b right value | ||
* @param eps epsilon / tolerance, default `1e-6` | ||
* @param a - left value | ||
* @param b - right value | ||
* @param eps - epsilon / tolerance, default `1e-6` | ||
*/ | ||
export declare const eqDelta: (a: number, b: number, eps?: number) => boolean; | ||
/** | ||
* Similar to `eqDelta()`, but used given `eps` as is. | ||
* Similar to {@link eqDelta}, but used given `eps` as is. | ||
* | ||
* @param a left value | ||
* @param b right value | ||
* @param eps epsilon / tolerance, default `1e-6` | ||
* @param a - left value | ||
* @param b - right value | ||
* @param eps - epsilon / tolerance, default `1e-6` | ||
*/ | ||
export declare const eqDeltaFixed: (a: number, b: number, eps?: number) => boolean; | ||
//# sourceMappingURL=eqdelta.d.ts.map |
@@ -13,14 +13,14 @@ import { EPS } from "./api"; | ||
* | ||
* @param a left value | ||
* @param b right value | ||
* @param eps epsilon / tolerance, default `1e-6` | ||
* @param a - left value | ||
* @param b - right value | ||
* @param eps - epsilon / tolerance, default `1e-6` | ||
*/ | ||
export const eqDelta = (a, b, eps = EPS) => abs(a - b) <= eps * max(1, abs(a), abs(b)); | ||
/** | ||
* Similar to `eqDelta()`, but used given `eps` as is. | ||
* Similar to {@link eqDelta}, but used given `eps` as is. | ||
* | ||
* @param a left value | ||
* @param b right value | ||
* @param eps epsilon / tolerance, default `1e-6` | ||
* @param a - left value | ||
* @param b - right value | ||
* @param eps - epsilon / tolerance, default `1e-6` | ||
*/ | ||
export const eqDeltaFixed = (a, b, eps = EPS) => abs(a - b) <= eps; |
/** | ||
* Returns true if `b` is a local minima, i.e. iff a > b and b < c. | ||
* | ||
* @param a | ||
* @param b | ||
* @param c | ||
* @param a - | ||
* @param b - | ||
* @param c - | ||
*/ | ||
@@ -12,5 +12,5 @@ export declare const isMinima: (a: number, b: number, c: number) => boolean; | ||
* | ||
* @param a | ||
* @param b | ||
* @param c | ||
* @param a - | ||
* @param b - | ||
* @param c - | ||
*/ | ||
@@ -23,5 +23,5 @@ export declare const isMaxima: (a: number, b: number, c: number) => boolean; | ||
* | ||
* @param values | ||
* @param from | ||
* @param to | ||
* @param values - | ||
* @param from - | ||
* @param to - | ||
*/ | ||
@@ -34,5 +34,5 @@ export declare const minimaIndex: (values: number[], from?: number, to?: number) => number; | ||
* | ||
* @param values | ||
* @param from | ||
* @param to | ||
* @param values - | ||
* @param from - | ||
* @param to - | ||
*/ | ||
@@ -45,5 +45,5 @@ export declare const maximaIndex: (values: number[], from?: number, to?: number) => number; | ||
* | ||
* @param values | ||
* @param from | ||
* @param to | ||
* @param values - | ||
* @param from - | ||
* @param to - | ||
*/ | ||
@@ -56,6 +56,7 @@ export declare const minimaIndices: (values: number[], from?: number, to?: number) => Generator<number, void, unknown>; | ||
* | ||
* @param values | ||
* @param from | ||
* @param to | ||
* @param values - | ||
* @param from - | ||
* @param to - | ||
*/ | ||
export declare const maximaIndices: (values: number[], from?: number, to?: number) => Generator<number, void, unknown>; | ||
//# sourceMappingURL=extrema.d.ts.map |
/** | ||
* Returns true if `b` is a local minima, i.e. iff a > b and b < c. | ||
* | ||
* @param a | ||
* @param b | ||
* @param c | ||
* @param a - | ||
* @param b - | ||
* @param c - | ||
*/ | ||
@@ -12,5 +12,5 @@ export const isMinima = (a, b, c) => a > b && b < c; | ||
* | ||
* @param a | ||
* @param b | ||
* @param c | ||
* @param a - | ||
* @param b - | ||
* @param c - | ||
*/ | ||
@@ -32,5 +32,5 @@ export const isMaxima = (a, b, c) => a < b && b > c; | ||
* | ||
* @param values | ||
* @param from | ||
* @param to | ||
* @param values - | ||
* @param from - | ||
* @param to - | ||
*/ | ||
@@ -43,5 +43,5 @@ export const minimaIndex = (values, from = 0, to = values.length) => index(isMinima, values, from, to); | ||
* | ||
* @param values | ||
* @param from | ||
* @param to | ||
* @param values - | ||
* @param from - | ||
* @param to - | ||
*/ | ||
@@ -63,5 +63,5 @@ export const maximaIndex = (values, from = 0, to = values.length) => index(isMaxima, values, from, to); | ||
* | ||
* @param values | ||
* @param from | ||
* @param to | ||
* @param values - | ||
* @param from - | ||
* @param to - | ||
*/ | ||
@@ -74,6 +74,6 @@ export const minimaIndices = (values, from = 0, to = values.length) => indices(minimaIndex, values, from, to); | ||
* | ||
* @param values | ||
* @param from | ||
* @param to | ||
* @param values - | ||
* @param from - | ||
* @param to - | ||
*/ | ||
export const maximaIndices = (values, from = 0, to = values.length) => indices(minimaIndex, values, from, to); |
@@ -5,5 +5,5 @@ /** | ||
* | ||
* @param x | ||
* @param a | ||
* @param b | ||
* @param x - | ||
* @param a - | ||
* @param b - | ||
*/ | ||
@@ -16,1 +16,2 @@ export declare const norm: (x: number, a: number, b: number) => number; | ||
export declare const fit11: (x: number, a: number, b: number) => number; | ||
//# sourceMappingURL=fit.d.ts.map |
@@ -6,5 +6,5 @@ import { clamp01, clamp11 } from "./interval"; | ||
* | ||
* @param x | ||
* @param a | ||
* @param b | ||
* @param x - | ||
* @param a - | ||
* @param b - | ||
*/ | ||
@@ -11,0 +11,0 @@ export const norm = (x, a, b) => b !== a ? (x - a) / (b - a) : 0; |
@@ -16,1 +16,2 @@ export * from "./api"; | ||
export * from "./step"; | ||
//# sourceMappingURL=index.d.ts.map |
@@ -63,1 +63,2 @@ export declare const signExtend8: (a: number) => number; | ||
export declare const rshiftu32: (a: number, b: number) => number; | ||
//# sourceMappingURL=int.d.ts.map |
/** | ||
* Clamps value `x` to given closed interval. | ||
* | ||
* @param x value to clamp | ||
* @param min lower bound | ||
* @param max upper bound | ||
* @param x - value to clamp | ||
* @param min - lower bound | ||
* @param max - upper bound | ||
*/ | ||
export declare const clamp: (x: number, min: number, max: number) => number; | ||
/** | ||
* Clamps value `x` to closed [0 .. 1] interval. | ||
* | ||
* @param x | ||
*/ | ||
export declare const clamp01: (x: number) => number; | ||
/** | ||
* Clamps value `x` to closed [-1 .. 1] interval. | ||
* | ||
* @param x | ||
*/ | ||
export declare const clamp11: (x: number) => number; | ||
/** | ||
* Clamps value `x` to closed [0 .. 0.5] interval. | ||
* | ||
* @param x | ||
*/ | ||
export declare const clamp05: (x: number) => number; | ||
/** | ||
* Folds `x` back inside closed [min..max] interval. Also see | ||
* {@link wrapOnce}. | ||
* | ||
* @param x - | ||
* @param min - | ||
* @param max - | ||
*/ | ||
export declare const wrap: (x: number, min: number, max: number) => number; | ||
/** | ||
* Like {@link wrap}, but optimized for cases where `x` is guaranteed to | ||
* be in `[min - d, max + d]` interval, where `d = max - min`. Result | ||
* will be in closed `[min..max]` interval. | ||
* | ||
* @param x - | ||
* @param min - | ||
* @param max - | ||
*/ | ||
export declare const wrapOnce: (x: number, min: number, max: number) => number; | ||
/** | ||
* Similar to {@link wrapOnce} for [0..1] interval. | ||
* | ||
* @param x - | ||
*/ | ||
export declare const wrap01: (x: number) => number; | ||
/** | ||
* Similar to {@link wrapOnce} for [-1..1] interval. | ||
* | ||
* @param x - | ||
*/ | ||
export declare const wrap11: (x: number) => number; | ||
@@ -23,5 +67,5 @@ export declare const min2id: (a: number, b: number) => 1 | 0; | ||
* | ||
* @param a | ||
* @param b | ||
* @param k smooth exponent (MUST be > 0) | ||
* @param a - | ||
* @param b - | ||
* @param k - smooth exponent (MUST be > 0) | ||
*/ | ||
@@ -34,7 +78,7 @@ export declare const smin: (a: number, b: number, k: number) => number; | ||
* | ||
* https://en.wikipedia.org/wiki/Smooth_maximum | ||
* {@link https://en.wikipedia.org/wiki/Smooth_maximum} | ||
* | ||
* @param a | ||
* @param b | ||
* @param k smooth exponent (MUST be > 0) | ||
* @param a - | ||
* @param b - | ||
* @param k - smooth exponent (MUST be > 0) | ||
*/ | ||
@@ -45,6 +89,6 @@ export declare const smax: (a: number, b: number, k: number) => number; | ||
* | ||
* @param x | ||
* @param min | ||
* @param max | ||
* @param k | ||
* @param x - | ||
* @param min - | ||
* @param max - | ||
* @param k - | ||
*/ | ||
@@ -55,6 +99,12 @@ export declare const sclamp: (x: number, min: number, max: number, k: number) => number; | ||
/** | ||
* http://www.musicdsp.org/showone.php?id=203 | ||
* If `abs(x) > abs(e)`, recursively mirrors `x` back into `[-e .. +e]` | ||
* interval at respective positive/negative boundary. | ||
* | ||
* @param e | ||
* @param x | ||
* @remarks | ||
* References: | ||
* - https://www.desmos.com/calculator/lkyf2ag3ta | ||
* - https://www.musicdsp.org/en/latest/Effects/203-fold-back-distortion.html | ||
* | ||
* @param e - threshold (> 0) | ||
* @param x - input value | ||
*/ | ||
@@ -65,5 +115,5 @@ export declare const foldback: (e: number, x: number) => number; | ||
* | ||
* @param x | ||
* @param min | ||
* @param max | ||
* @param x - | ||
* @param min - | ||
* @param max - | ||
*/ | ||
@@ -74,6 +124,7 @@ export declare const inRange: (x: number, min: number, max: number) => boolean; | ||
* | ||
* @param x | ||
* @param min | ||
* @param max | ||
* @param x - | ||
* @param min - | ||
* @param max - | ||
*/ | ||
export declare const inOpenRange: (x: number, min: number, max: number) => boolean; | ||
//# sourceMappingURL=interval.d.ts.map |
118
interval.js
/** | ||
* Clamps value `x` to given closed interval. | ||
* | ||
* @param x value to clamp | ||
* @param min lower bound | ||
* @param max upper bound | ||
* @param x - value to clamp | ||
* @param min - lower bound | ||
* @param max - upper bound | ||
*/ | ||
export const clamp = (x, min, max) => x < min ? min : x > max ? max : x; | ||
/** | ||
* Clamps value `x` to closed [0 .. 1] interval. | ||
* | ||
* @param x | ||
*/ | ||
export const clamp01 = (x) => (x < 0 ? 0 : x > 1 ? 1 : x); | ||
/** | ||
* Clamps value `x` to closed [-1 .. 1] interval. | ||
* | ||
* @param x | ||
*/ | ||
export const clamp11 = (x) => (x < -1 ? -1 : x > 1 ? 1 : x); | ||
export const wrap = (x, min, max) => x < min ? x - min + max : x >= max ? x - max + min : x; | ||
export const wrap01 = (x) => (x < 0 ? x + 1 : x >= 1 ? x - 1 : x); | ||
export const wrap11 = (x) => (x < -1 ? x + 2 : x >= 1 ? x - 2 : x); | ||
/** | ||
* Clamps value `x` to closed [0 .. 0.5] interval. | ||
* | ||
* @param x | ||
*/ | ||
export const clamp05 = (x) => (x < 0 ? 0 : x > 0.5 ? 0.5 : x); | ||
/** | ||
* Folds `x` back inside closed [min..max] interval. Also see | ||
* {@link wrapOnce}. | ||
* | ||
* @param x - | ||
* @param min - | ||
* @param max - | ||
*/ | ||
export const wrap = (x, min, max) => { | ||
if (min === max) | ||
return min; | ||
if (x > max) { | ||
const d = max - min; | ||
x -= d; | ||
if (x > max) | ||
x -= d * (((x - min) / d) | 0); | ||
} | ||
else if (x < min) { | ||
const d = max - min; | ||
x += d; | ||
if (x < min) | ||
x += d * (((min - x) / d + 1) | 0); | ||
} | ||
return x; | ||
}; | ||
/** | ||
* Like {@link wrap}, but optimized for cases where `x` is guaranteed to | ||
* be in `[min - d, max + d]` interval, where `d = max - min`. Result | ||
* will be in closed `[min..max]` interval. | ||
* | ||
* @param x - | ||
* @param min - | ||
* @param max - | ||
*/ | ||
export const wrapOnce = (x, min, max) => x < min ? x - min + max : x > max ? x - max + min : x; | ||
/** | ||
* Similar to {@link wrapOnce} for [0..1] interval. | ||
* | ||
* @param x - | ||
*/ | ||
export const wrap01 = (x) => (x < 0 ? x + 1 : x > 1 ? x - 1 : x); | ||
/** | ||
* Similar to {@link wrapOnce} for [-1..1] interval. | ||
* | ||
* @param x - | ||
*/ | ||
export const wrap11 = (x) => (x < -1 ? x + 2 : x > 1 ? x - 2 : x); | ||
export const min2id = (a, b) => (a <= b ? 0 : 1); | ||
@@ -51,5 +111,5 @@ export const min3id = (a, b, c) => a <= b ? (a <= c ? 0 : 2) : b <= c ? 1 : 2; | ||
* | ||
* @param a | ||
* @param b | ||
* @param k smooth exponent (MUST be > 0) | ||
* @param a - | ||
* @param b - | ||
* @param k - smooth exponent (MUST be > 0) | ||
*/ | ||
@@ -62,7 +122,7 @@ export const smin = (a, b, k) => smax(a, b, -k); | ||
* | ||
* https://en.wikipedia.org/wiki/Smooth_maximum | ||
* {@link https://en.wikipedia.org/wiki/Smooth_maximum} | ||
* | ||
* @param a | ||
* @param b | ||
* @param k smooth exponent (MUST be > 0) | ||
* @param a - | ||
* @param b - | ||
* @param k - smooth exponent (MUST be > 0) | ||
*/ | ||
@@ -77,6 +137,6 @@ export const smax = (a, b, k) => { | ||
* | ||
* @param x | ||
* @param min | ||
* @param max | ||
* @param k | ||
* @param x - | ||
* @param min - | ||
* @param max - | ||
* @param k - | ||
*/ | ||
@@ -87,6 +147,12 @@ export const sclamp = (x, min, max, k) => smin(smax(x, min, k), max, k); | ||
/** | ||
* http://www.musicdsp.org/showone.php?id=203 | ||
* If `abs(x) > abs(e)`, recursively mirrors `x` back into `[-e .. +e]` | ||
* interval at respective positive/negative boundary. | ||
* | ||
* @param e | ||
* @param x | ||
* @remarks | ||
* References: | ||
* - https://www.desmos.com/calculator/lkyf2ag3ta | ||
* - https://www.musicdsp.org/en/latest/Effects/203-fold-back-distortion.html | ||
* | ||
* @param e - threshold (> 0) | ||
* @param x - input value | ||
*/ | ||
@@ -97,5 +163,5 @@ export const foldback = (e, x) => x < -e || x > e ? Math.abs(Math.abs((x - e) % (4 * e)) - 2 * e) - e : x; | ||
* | ||
* @param x | ||
* @param min | ||
* @param max | ||
* @param x - | ||
* @param min - | ||
* @param max - | ||
*/ | ||
@@ -106,6 +172,6 @@ export const inRange = (x, min, max) => x >= min && x <= max; | ||
* | ||
* @param x | ||
* @param min | ||
* @param max | ||
* @param x - | ||
* @param min - | ||
* @param max - | ||
*/ | ||
export const inOpenRange = (x, min, max) => x > min && x < max; |
@@ -128,5 +128,23 @@ 'use strict'; | ||
const clamp11 = (x) => (x < -1 ? -1 : x > 1 ? 1 : x); | ||
const wrap = (x, min, max) => x < min ? x - min + max : x >= max ? x - max + min : x; | ||
const wrap01 = (x) => (x < 0 ? x + 1 : x >= 1 ? x - 1 : x); | ||
const wrap11 = (x) => (x < -1 ? x + 2 : x >= 1 ? x - 2 : x); | ||
const clamp05 = (x) => (x < 0 ? 0 : x > 0.5 ? 0.5 : x); | ||
const wrap = (x, min, max) => { | ||
if (min === max) | ||
return min; | ||
if (x > max) { | ||
const d = max - min; | ||
x -= d; | ||
if (x > max) | ||
x -= d * (((x - min) / d) | 0); | ||
} | ||
else if (x < min) { | ||
const d = max - min; | ||
x += d; | ||
if (x < min) | ||
x += d * (((min - x) / d + 1) | 0); | ||
} | ||
return x; | ||
}; | ||
const wrapOnce = (x, min, max) => x < min ? x - min + max : x > max ? x - max + min : x; | ||
const wrap01 = (x) => (x < 0 ? x + 1 : x > 1 ? x - 1 : x); | ||
const wrap11 = (x) => (x < -1 ? x + 2 : x > 1 ? x - 2 : x); | ||
const min2id = (a, b) => (a <= b ? 0 : 1); | ||
@@ -327,2 +345,3 @@ const min3id = (a, b, c) => a <= b ? (a <= c ? 0 : 2) : b <= c ? 1 : 2; | ||
const sigmoid11 = (k, t) => 1 / (1 + Math.exp(-k * t)); | ||
const expFactor = (a, b, num) => Math.pow((b / a), (1 / num)); | ||
@@ -456,2 +475,3 @@ const fmod = (a, b) => a - b * Math.floor(a / b); | ||
exports.clamp01 = clamp01; | ||
exports.clamp05 = clamp05; | ||
exports.clamp11 = clamp11; | ||
@@ -476,2 +496,3 @@ exports.classifyCrossing = classifyCrossing; | ||
exports.eqDeltaFixed = eqDeltaFixed; | ||
exports.expFactor = expFactor; | ||
exports.expStep = expStep; | ||
@@ -582,2 +603,3 @@ exports.fastCos = fastCos; | ||
exports.wrap11 = wrap11; | ||
exports.wrapOnce = wrapOnce; | ||
exports.xori16 = xori16; | ||
@@ -584,0 +606,0 @@ exports.xori32 = xori32; |
@@ -1,1 +0,1 @@ | ||
!function(t,a){"object"==typeof exports&&"undefined"!=typeof module?a(exports):"function"==typeof define&&define.amd?define(["exports"],a):a(((t=t||self).thi=t.thi||{},t.thi.ng=t.thi.ng||{},t.thi.ng.math={}))}(this,(function(t){"use strict";const a=Math.PI,i=2*a,n=a/2,s=a/3,e=a/4,r=a/6,o=1/a,h=1/i,u=1/n,c=a/180,M=180/a,d=(1+Math.sqrt(5))/2,f=Math.SQRT2,l=Math.sqrt(3),m=f/2,b=l/2;var x;(x=t.Crossing||(t.Crossing={}))[x.EQUAL=0]="EQUAL",x[x.FLAT=1]="FLAT",x[x.UNDER=2]="UNDER",x[x.OVER=3]="OVER",x[x.OTHER=4]="OTHER";const p=t=>(t%=i,t<0?i+t:t),g=t=>(t=Math.abs(t),t>a?i-t:t),I=t=>{const a=t*t;return.99940307+a*(.03679168*a-.49558072)},R=t=>{switch((t%=i)<0&&(t=-t),t*u|0){case 0:return I(t);case 1:return-I(a-t);case 2:return-I(t-a);default:return I(i-t)}},T=Math.abs,E=Math.max,v=(t,a,i=1e-6)=>T(t-a)<=i*E(1,T(t),T(a)),D=(t,a,i,n)=>t<i&&a>n,A=(t,a,i,n)=>t>i&&a<n,P=(t,a,i)=>t>a&&a<i,_=(t,a,i)=>t<a&&a>i,q=(t,a,i=0,n=a.length)=>{n--;for(let s=i+1;s<n;s++)if(t(a[s-1],a[s],a[s+1]))return s;return-1},C=(t,a=0,i=t.length)=>q(P,t,a,i);function*H(t,a,i=0,n=a.length){for(;i<n;){const s=t(a,i,n);if(s<0)return;yield s,i=s+1}}const S=t=>t<0?0:t>1?1:t,w=t=>t<-1?-1:t>1?1:t,Q=(t,a,i)=>O(t,a,-i),O=(t,a,i)=>{const n=Math.exp(t*i),s=Math.exp(a*i);return(t*n+a*s)/(n+s)},U=(t,a,i)=>i!==a?(t-a)/(i-a):0,y=255,L=65535,F=t=>(t&=y,128&t?t|~y:t),N=t=>(t&=L,32768&t?t|~L:t),V=(t,a,i,n=16,s=8,e=0,r=1,o=1e-6)=>{if(s<=0)return(e+r)/2;const h=(r-e)/n;let u=e,c=1/0;for(let s=0;s<=n;s++){const n=e+s*h,r=a(i,t(n));if(r<c){if(r<=o)return n;c=r,u=n}}return V(t,a,i,n,s-1,Math.max(u-h,0),Math.min(u+h,1))},j=(t,a,i)=>t+(a-t)*i,G=t=>t-Math.floor(t);t.DEG2RAD=c,t.EPS=1e-6,t.HALF_PI=n,t.INV_HALF_PI=u,t.INV_PI=o,t.INV_TAU=h,t.PHI=d,t.PI=a,t.QUARTER_PI=e,t.RAD2DEG=M,t.SIXTH=1/6,t.SIXTH_PI=r,t.SQRT2=f,t.SQRT2_2=m,t.SQRT2_3=b,t.SQRT3=l,t.TAU=i,t.THIRD=1/3,t.THIRD_PI=s,t.TWO_THIRD=2/3,t.absDiff=(t,a)=>Math.abs(t-a),t.absInnerAngle=g,t.absMax=(t,a)=>Math.abs(t)>Math.abs(a)?t:a,t.absMin=(t,a)=>Math.abs(t)<Math.abs(a)?t:a,t.absTheta=p,t.addi16=(t,a)=>N((0|t)+(0|a)),t.addi32=(t,a)=>(0|t)+(0|a)|0,t.addi8=(t,a)=>F((0|t)+(0|a)),t.addu16=(t,a)=>(t&L)+(a&L)&L,t.addu32=(t,a)=>(t>>>0)+(a>>>0)>>>0,t.addu8=(t,a)=>(t&y)+(a&y)&y,t.andi16=(t,a)=>N((0|t)&(0|a)),t.andi32=(t,a)=>(0|t)&(0|a),t.andi8=(t,a)=>F((0|t)&(0|a)),t.andu16=(t,a)=>t&L&a&L&L,t.andu32=(t,a)=>(t>>>0&a>>>0)>>>0,t.andu8=(t,a)=>t&y&a&y&y,t.angleDist=(t,a)=>g(p(a%i-t%i)),t.atan2Abs=(t,a)=>p(Math.atan2(t,a)),t.bounce=(t,a,i)=>{const s=i*t;return 1-a*Math.sin(s)/s*Math.cos(i*n)},t.circular=t=>(t=1-t,Math.sqrt(1-t*t)),t.clamp=(t,a,i)=>t<a?a:t>i?i:t,t.clamp01=S,t.clamp11=w,t.classifyCrossing=(t,a,i,n,s=1e-6)=>D(t,a,i,n)?3:A(t,a,i,n)?2:v(t,i,s)&&v(a,n,s)?v(t,n,s)?1:0:4,t.cosine=t=>1-(.5*Math.cos(t*a)+.5),t.cossin=(t,a=1)=>[Math.cos(t)*a,Math.sin(t)*a],t.cot=t=>1/Math.tan(t),t.csc=t=>1/Math.sin(t),t.cubicPulse=(t,a,i)=>(i=Math.abs(i-a))>t?0:1-(i/=t)*i*(3-2*i),t.decimated=(t,a)=>Math.floor(a*t)/t,t.deg=t=>t*M,t.derivative=(t,a=1e-6)=>i=>(t(i+a)-t(i))/a,t.divi16=(t,a)=>N((0|t)/(0|a)),t.divi32=(t,a)=>(0|t)/(0|a)|0,t.divi8=(t,a)=>F((0|t)/(0|a)),t.divu16=(t,a)=>(t&L)/(a&L)&L,t.divu32=(t,a)=>(t>>>0)/(a>>>0)>>>0,t.divu8=(t,a)=>(t&y)/(a&y)&y,t.ease=(t,a)=>Math.pow(a,t),t.eqDelta=v,t.eqDeltaFixed=(t,a,i=1e-6)=>T(t-a)<=i,t.expStep=(t,a,i)=>1-Math.exp(-t*Math.pow(i,a)),t.fastCos=R,t.fastSin=t=>R(n-t),t.fit=(t,a,i,n,s)=>n+(s-n)*U(t,a,i),t.fit01=(t,a,i)=>a+(i-a)*S(t),t.fit10=(t,a,i)=>i+(a-i)*S(t),t.fit11=(t,a,i)=>a+(i-a)*(.5+.5*w(t)),t.fitClamped=(t,a,i,n,s)=>n+(s-n)*S(U(t,a,i)),t.fmod=(t,a)=>t-a*Math.floor(t/a),t.foldback=(t,a)=>a<-t||a>t?Math.abs(Math.abs((a-t)%(4*t))-2*t)-t:a,t.fract=G,t.gain=(t,a)=>a<.5?.5*Math.pow(2*a,t):1-.5*Math.pow(2-2*a,t),t.impulse=(t,a)=>{const i=t*a;return i*Math.exp(1-i)},t.inOpenRange=(t,a,i)=>t>a&&t<i,t.inRange=(t,a,i)=>t>=a&&t<=i,t.isCrossOver=D,t.isCrossUnder=A,t.isMaxima=_,t.isMinima=P,t.loc=(t,a,i)=>Math.sqrt(t*t+a*a-2*t*a*Math.cos(i)),t.lshifti16=(t,a)=>N((0|t)<<(0|a)),t.lshifti32=(t,a)=>(0|t)<<(0|a),t.lshifti8=(t,a)=>F((0|t)<<(0|a)),t.lshiftu16=(t,a)=>(t&L)<<(a&L)&L,t.lshiftu32=(t,a)=>t>>>0<<(a>>>0)>>>0,t.lshiftu8=(t,a)=>(t&y)<<(a&y)&y,t.max2id=(t,a)=>t>=a?0:1,t.max3id=(t,a,i)=>t>=a?t>=i?0:2:a>=i?1:2,t.max4id=(t,a,i,n)=>t>=a?t>=i?t>=n?0:3:i>=n?2:3:a>=i?a>=n?1:3:i>=n?2:3,t.maximaIndex=(t,a=0,i=t.length)=>q(_,t,a,i),t.maximaIndices=(t,a=0,i=t.length)=>H(C,t,a,i),t.min2id=(t,a)=>t<=a?0:1,t.min3id=(t,a,i)=>t<=a?t<=i?0:2:a<=i?1:2,t.min4id=(t,a,i,n)=>t<=a?t<=i?t<=n?0:3:i<=n?2:3:a<=i?a<=n?1:3:i<=n?2:3,t.minError=V,t.minimaIndex=C,t.minimaIndices=(t,a=0,i=t.length)=>H(C,t,a,i),t.mix=j,t.mixBilinear=(t,a,i,n,s,e)=>j(j(t,a,s),j(i,n,s),e),t.mixCubic=(t,a,i,n,s)=>{const e=s*s,r=1-s,o=r*r;return t*o*r+3*a*o*s+3*i*e*r+n*e*s},t.mixCubicHermite=(t,a,i,n,s)=>{const e=s-1,r=s*s,o=e*e;return(1+2*s)*o*t+s*o*a+r*(3-2*s)*i+r*e*n},t.mixHermite=(t,a,i,n,s)=>{const e=.5*(i-t),r=1.5*(a-i)+.5*(n-t);return((r*s+t-a+e-r)*s+e)*s+a},t.mixQuadratic=(t,a,i,n)=>{const s=1-n;return t*s*s+2*a*s*n+i*n*n},t.muli16=(t,a)=>N((0|t)*(0|a)),t.muli32=(t,a)=>(0|t)*(0|a)|0,t.muli8=(t,a)=>F((0|t)*(0|a)),t.mulu16=(t,a)=>(t&L)*(a&L)&L,t.mulu32=(t,a)=>(t>>>0)*(a>>>0)>>>0,t.mulu8=(t,a)=>(t&y)*(a&y)&y,t.norm=U,t.normCos=t=>{const a=t*t;return 1+a*(2*a-4)},t.noti16=t=>N(~t),t.noti32=t=>~t,t.noti8=t=>F(~t),t.notu16=t=>~t&L,t.notu32=t=>~t>>>0,t.notu8=t=>~t&y,t.ori16=(t,a)=>N(0|t|a),t.ori32=(t,a)=>0|t|a,t.ori8=(t,a)=>F(0|t|a),t.oru16=(t,a)=>(t&L|a&L)&L,t.oru32=(t,a)=>(t>>>0|a>>>0)>>>0,t.oru8=(t,a)=>(t&y|a&y)&y,t.parabola=(t,a)=>Math.pow(4*a*(1-a),t),t.quadrant=t=>p(t)*u|0,t.rad=t=>t*c,t.roundEps=(t,a=1e-6)=>{const i=G(t);return i<=a||i>=1-a?Math.round(t):t},t.roundTo=(t,a=1)=>Math.round(t/a)*a,t.rshifti16=(t,a)=>N((0|t)>>(0|a)),t.rshifti32=(t,a)=>(0|t)>>(0|a),t.rshifti8=(t,a)=>F((0|t)>>(0|a)),t.rshiftu16=(t,a)=>(t&L)>>>(a&L)&L,t.rshiftu32=(t,a)=>t>>>0>>>(a>>>0)>>>0,t.rshiftu8=(t,a)=>(t&y)>>>(a&y)&y,t.sclamp=(t,a,i,n)=>Q(O(t,a,n),i,n),t.sec=t=>1/Math.cos(t),t.sigmoid=(t,a)=>1/(1+Math.exp(-t*(2*a-1))),t.sigmoid11=(t,a)=>1/(1+Math.exp(-t*a)),t.sign=(t,a=1e-6)=>t>a?1:t<-a?-1:0,t.signExtend16=N,t.signExtend8=F,t.simplifyRatio=(t,a)=>{let i=Math.abs(t),n=Math.abs(a);for(;;){if(i<n){const t=i;i=n,n=t}const s=i%n;if(!s)return[t/n,a/n];i=s}},t.sinc=(t,i)=>(i=a*(t*i-1),Math.sin(i)/i),t.sincos=(t,a=1)=>[Math.sin(t)*a,Math.cos(t)*a],t.smax=O,t.smin=Q,t.smoothStep=(t,a,i)=>(3-2*(i=S((i-t)/(a-t))))*i*i,t.smootherStep=(t,a,i)=>(i=S((i-t)/(a-t)))*i*i*(i*(6*i-15)+10),t.solveCubic=(t,a,i,n,s=1e-9)=>{const e=t*t,r=a*a,o=a/(3*t),h=(3*t*i-r)/(3*e),u=(2*r*a-9*t*a*i+27*e*n)/(27*e*t);if(Math.abs(h)<s)return[Math.cbrt(-u)-o];if(Math.abs(u)<s)return h<0?[-Math.sqrt(-h)-o,-o,Math.sqrt(-h)-o]:[-o];{const t=u*u/4+h*h*h/27;if(Math.abs(t)<s)return[-1.5*u/h-o,3*u/h-o];if(t>0){const a=Math.cbrt(-u/2-Math.sqrt(t));return[a-h/(3*a)-o]}{const t=2*Math.sqrt(-h/3),a=Math.acos(3*u/h/t)/3,i=2*Math.PI/3;return[t*Math.cos(a)-o,t*Math.cos(a-i)-o,t*Math.cos(a-2*i)-o]}}},t.solveLinear=(t,a)=>-a/t,t.solveQuadratic=(t,a,i,n=1e-9)=>{const s=2*t;let e=a*a-4*t*i;return e<0?[]:e<n?[-a/s]:[(-a-(e=Math.sqrt(e)))/s,(-a+e)/s]},t.step=(t,a)=>a<t?0:1,t.subi16=(t,a)=>N((0|t)-(0|a)),t.subi32=(t,a)=>(0|t)-(0|a)|0,t.subi8=(t,a)=>F((0|t)-(0|a)),t.subu16=(t,a)=>(t&L)-(a&L)&L,t.subu32=(t,a)=>(t>>>0)-(a>>>0)>>>0,t.subu8=(t,a)=>(t&y)-(a&y)&y,t.tangentCardinal=(t,a,i=.5,n=0,s=2)=>i*((a-t)/(s-n)),t.tangentDiff3=(t,a,i,n=0,s=1,e=2)=>.5*((i-a)/(e-s)+(a-t)/(s-n)),t.trunc=t=>t<0?Math.ceil(t):Math.floor(t),t.tween=(t,a,i)=>n=>j(a,i,t(n)),t.wrap=(t,a,i)=>t<a?t-a+i:t>=i?t-i+a:t,t.wrap01=t=>t<0?t+1:t>=1?t-1:t,t.wrap11=t=>t<-1?t+2:t>=1?t-2:t,t.xori16=(t,a)=>N((0|t)^(0|a)),t.xori32=(t,a)=>(0|t)^(0|a),t.xori8=(t,a)=>F((0|t)^(0|a)),t.xoru16=(t,a)=>(t&L^a&L)&L,t.xoru32=(t,a)=>(t>>>0^a>>>0)>>>0,t.xoru8=(t,a)=>(t&y^a&y)&y,Object.defineProperty(t,"__esModule",{value:!0})})); | ||
!function(t,a){"object"==typeof exports&&"undefined"!=typeof module?a(exports):"function"==typeof define&&define.amd?define(["exports"],a):a(((t=t||self).thi=t.thi||{},t.thi.ng=t.thi.ng||{},t.thi.ng.math={}))}(this,(function(t){"use strict";const a=Math.PI,i=2*a,n=a/2,s=a/3,e=a/4,r=a/6,o=1/a,h=1/i,u=1/n,c=a/180,M=180/a,d=(1+Math.sqrt(5))/2,f=Math.SQRT2,l=Math.sqrt(3),m=f/2,p=l/2;var b;(b=t.Crossing||(t.Crossing={}))[b.EQUAL=0]="EQUAL",b[b.FLAT=1]="FLAT",b[b.UNDER=2]="UNDER",b[b.OVER=3]="OVER",b[b.OTHER=4]="OTHER";const x=t=>(t%=i)<0?i+t:t,g=t=>(t=Math.abs(t))>a?i-t:t,I=t=>{const a=t*t;return.99940307+a*(.03679168*a-.49558072)},R=t=>{switch((t%=i)<0&&(t=-t),t*u|0){case 0:return I(t);case 1:return-I(a-t);case 2:return-I(t-a);default:return I(i-t)}},T=Math.abs,E=Math.max,v=(t,a,i=1e-6)=>T(t-a)<=i*E(1,T(t),T(a)),D=(t,a,i,n)=>t<i&&a>n,A=(t,a,i,n)=>t>i&&a<n,P=(t,a,i)=>t>a&&a<i,_=(t,a,i)=>t<a&&a>i,q=(t,a,i=0,n=a.length)=>{n--;for(let s=i+1;s<n;s++)if(t(a[s-1],a[s],a[s+1]))return s;return-1},w=(t,a=0,i=t.length)=>q(P,t,a,i);function*C(t,a,i=0,n=a.length){for(;i<n;){const s=t(a,i,n);if(s<0)return;yield s,i=s+1}}const H=t=>t<0?0:t>1?1:t,S=t=>t<-1?-1:t>1?1:t,Q=(t,a,i)=>O(t,a,-i),O=(t,a,i)=>{const n=Math.exp(t*i),s=Math.exp(a*i);return(t*n+a*s)/(n+s)},U=(t,a,i)=>i!==a?(t-a)/(i-a):0,y=255,L=65535,F=t=>128&(t&=y)?t|~y:t,N=t=>32768&(t&=L)?t|~L:t,V=(t,a,i,n=16,s=8,e=0,r=1,o=1e-6)=>{if(s<=0)return(e+r)/2;const h=(r-e)/n;let u=e,c=1/0;for(let s=0;s<=n;s++){const n=e+s*h,r=a(i,t(n));if(r<c){if(r<=o)return n;c=r,u=n}}return V(t,a,i,n,s-1,Math.max(u-h,0),Math.min(u+h,1))},j=(t,a,i)=>t+(a-t)*i,G=t=>t-Math.floor(t);t.DEG2RAD=c,t.EPS=1e-6,t.HALF_PI=n,t.INV_HALF_PI=u,t.INV_PI=o,t.INV_TAU=h,t.PHI=d,t.PI=a,t.QUARTER_PI=e,t.RAD2DEG=M,t.SIXTH=1/6,t.SIXTH_PI=r,t.SQRT2=f,t.SQRT2_2=m,t.SQRT2_3=p,t.SQRT3=l,t.TAU=i,t.THIRD=1/3,t.THIRD_PI=s,t.TWO_THIRD=2/3,t.absDiff=(t,a)=>Math.abs(t-a),t.absInnerAngle=g,t.absMax=(t,a)=>Math.abs(t)>Math.abs(a)?t:a,t.absMin=(t,a)=>Math.abs(t)<Math.abs(a)?t:a,t.absTheta=x,t.addi16=(t,a)=>N((0|t)+(0|a)),t.addi32=(t,a)=>(0|t)+(0|a)|0,t.addi8=(t,a)=>F((0|t)+(0|a)),t.addu16=(t,a)=>(t&L)+(a&L)&L,t.addu32=(t,a)=>(t>>>0)+(a>>>0)>>>0,t.addu8=(t,a)=>(t&y)+(a&y)&y,t.andi16=(t,a)=>N((0|t)&(0|a)),t.andi32=(t,a)=>(0|t)&(0|a),t.andi8=(t,a)=>F((0|t)&(0|a)),t.andu16=(t,a)=>t&L&a&L&L,t.andu32=(t,a)=>(t>>>0&a>>>0)>>>0,t.andu8=(t,a)=>t&y&a&y&y,t.angleDist=(t,a)=>g(x(a%i-t%i)),t.atan2Abs=(t,a)=>x(Math.atan2(t,a)),t.bounce=(t,a,i)=>{const s=i*t;return 1-a*Math.sin(s)/s*Math.cos(i*n)},t.circular=t=>(t=1-t,Math.sqrt(1-t*t)),t.clamp=(t,a,i)=>t<a?a:t>i?i:t,t.clamp01=H,t.clamp05=t=>t<0?0:t>.5?.5:t,t.clamp11=S,t.classifyCrossing=(t,a,i,n,s=1e-6)=>D(t,a,i,n)?3:A(t,a,i,n)?2:v(t,i,s)&&v(a,n,s)?v(t,n,s)?1:0:4,t.cosine=t=>1-(.5*Math.cos(t*a)+.5),t.cossin=(t,a=1)=>[Math.cos(t)*a,Math.sin(t)*a],t.cot=t=>1/Math.tan(t),t.csc=t=>1/Math.sin(t),t.cubicPulse=(t,a,i)=>(i=Math.abs(i-a))>t?0:1-(i/=t)*i*(3-2*i),t.decimated=(t,a)=>Math.floor(a*t)/t,t.deg=t=>t*M,t.derivative=(t,a=1e-6)=>i=>(t(i+a)-t(i))/a,t.divi16=(t,a)=>N((0|t)/(0|a)),t.divi32=(t,a)=>(0|t)/(0|a)|0,t.divi8=(t,a)=>F((0|t)/(0|a)),t.divu16=(t,a)=>(t&L)/(a&L)&L,t.divu32=(t,a)=>(t>>>0)/(a>>>0)>>>0,t.divu8=(t,a)=>(t&y)/(a&y)&y,t.ease=(t,a)=>Math.pow(a,t),t.eqDelta=v,t.eqDeltaFixed=(t,a,i=1e-6)=>T(t-a)<=i,t.expFactor=(t,a,i)=>Math.pow(a/t,1/i),t.expStep=(t,a,i)=>1-Math.exp(-t*Math.pow(i,a)),t.fastCos=R,t.fastSin=t=>R(n-t),t.fit=(t,a,i,n,s)=>n+(s-n)*U(t,a,i),t.fit01=(t,a,i)=>a+(i-a)*H(t),t.fit10=(t,a,i)=>i+(a-i)*H(t),t.fit11=(t,a,i)=>a+(i-a)*(.5+.5*S(t)),t.fitClamped=(t,a,i,n,s)=>n+(s-n)*H(U(t,a,i)),t.fmod=(t,a)=>t-a*Math.floor(t/a),t.foldback=(t,a)=>a<-t||a>t?Math.abs(Math.abs((a-t)%(4*t))-2*t)-t:a,t.fract=G,t.gain=(t,a)=>a<.5?.5*Math.pow(2*a,t):1-.5*Math.pow(2-2*a,t),t.impulse=(t,a)=>{const i=t*a;return i*Math.exp(1-i)},t.inOpenRange=(t,a,i)=>t>a&&t<i,t.inRange=(t,a,i)=>t>=a&&t<=i,t.isCrossOver=D,t.isCrossUnder=A,t.isMaxima=_,t.isMinima=P,t.loc=(t,a,i)=>Math.sqrt(t*t+a*a-2*t*a*Math.cos(i)),t.lshifti16=(t,a)=>N((0|t)<<(0|a)),t.lshifti32=(t,a)=>(0|t)<<(0|a),t.lshifti8=(t,a)=>F((0|t)<<(0|a)),t.lshiftu16=(t,a)=>(t&L)<<(a&L)&L,t.lshiftu32=(t,a)=>t>>>0<<(a>>>0)>>>0,t.lshiftu8=(t,a)=>(t&y)<<(a&y)&y,t.max2id=(t,a)=>t>=a?0:1,t.max3id=(t,a,i)=>t>=a?t>=i?0:2:a>=i?1:2,t.max4id=(t,a,i,n)=>t>=a?t>=i?t>=n?0:3:i>=n?2:3:a>=i?a>=n?1:3:i>=n?2:3,t.maximaIndex=(t,a=0,i=t.length)=>q(_,t,a,i),t.maximaIndices=(t,a=0,i=t.length)=>C(w,t,a,i),t.min2id=(t,a)=>t<=a?0:1,t.min3id=(t,a,i)=>t<=a?t<=i?0:2:a<=i?1:2,t.min4id=(t,a,i,n)=>t<=a?t<=i?t<=n?0:3:i<=n?2:3:a<=i?a<=n?1:3:i<=n?2:3,t.minError=V,t.minimaIndex=w,t.minimaIndices=(t,a=0,i=t.length)=>C(w,t,a,i),t.mix=j,t.mixBilinear=(t,a,i,n,s,e)=>j(j(t,a,s),j(i,n,s),e),t.mixCubic=(t,a,i,n,s)=>{const e=s*s,r=1-s,o=r*r;return t*o*r+3*a*o*s+3*i*e*r+n*e*s},t.mixCubicHermite=(t,a,i,n,s)=>{const e=s-1,r=s*s,o=e*e;return(1+2*s)*o*t+s*o*a+r*(3-2*s)*i+r*e*n},t.mixHermite=(t,a,i,n,s)=>{const e=.5*(i-t),r=1.5*(a-i)+.5*(n-t);return((r*s+t-a+e-r)*s+e)*s+a},t.mixQuadratic=(t,a,i,n)=>{const s=1-n;return t*s*s+2*a*s*n+i*n*n},t.muli16=(t,a)=>N((0|t)*(0|a)),t.muli32=(t,a)=>(0|t)*(0|a)|0,t.muli8=(t,a)=>F((0|t)*(0|a)),t.mulu16=(t,a)=>(t&L)*(a&L)&L,t.mulu32=(t,a)=>(t>>>0)*(a>>>0)>>>0,t.mulu8=(t,a)=>(t&y)*(a&y)&y,t.norm=U,t.normCos=t=>{const a=t*t;return 1+a*(2*a-4)},t.noti16=t=>N(~t),t.noti32=t=>~t,t.noti8=t=>F(~t),t.notu16=t=>~t&L,t.notu32=t=>~t>>>0,t.notu8=t=>~t&y,t.ori16=(t,a)=>N(0|t|a),t.ori32=(t,a)=>0|t|a,t.ori8=(t,a)=>F(0|t|a),t.oru16=(t,a)=>(t&L|a&L)&L,t.oru32=(t,a)=>(t>>>0|a>>>0)>>>0,t.oru8=(t,a)=>(t&y|a&y)&y,t.parabola=(t,a)=>Math.pow(4*a*(1-a),t),t.quadrant=t=>x(t)*u|0,t.rad=t=>t*c,t.roundEps=(t,a=1e-6)=>{const i=G(t);return i<=a||i>=1-a?Math.round(t):t},t.roundTo=(t,a=1)=>Math.round(t/a)*a,t.rshifti16=(t,a)=>N((0|t)>>(0|a)),t.rshifti32=(t,a)=>(0|t)>>(0|a),t.rshifti8=(t,a)=>F((0|t)>>(0|a)),t.rshiftu16=(t,a)=>(t&L)>>>(a&L)&L,t.rshiftu32=(t,a)=>t>>>0>>>(a>>>0)>>>0,t.rshiftu8=(t,a)=>(t&y)>>>(a&y)&y,t.sclamp=(t,a,i,n)=>Q(O(t,a,n),i,n),t.sec=t=>1/Math.cos(t),t.sigmoid=(t,a)=>1/(1+Math.exp(-t*(2*a-1))),t.sigmoid11=(t,a)=>1/(1+Math.exp(-t*a)),t.sign=(t,a=1e-6)=>t>a?1:t<-a?-1:0,t.signExtend16=N,t.signExtend8=F,t.simplifyRatio=(t,a)=>{let i=Math.abs(t),n=Math.abs(a);for(;;){if(i<n){const t=i;i=n,n=t}const s=i%n;if(!s)return[t/n,a/n];i=s}},t.sinc=(t,i)=>(i=a*(t*i-1),Math.sin(i)/i),t.sincos=(t,a=1)=>[Math.sin(t)*a,Math.cos(t)*a],t.smax=O,t.smin=Q,t.smoothStep=(t,a,i)=>(3-2*(i=H((i-t)/(a-t))))*i*i,t.smootherStep=(t,a,i)=>(i=H((i-t)/(a-t)))*i*i*(i*(6*i-15)+10),t.solveCubic=(t,a,i,n,s=1e-9)=>{const e=t*t,r=a*a,o=a/(3*t),h=(3*t*i-r)/(3*e),u=(2*r*a-9*t*a*i+27*e*n)/(27*e*t);if(Math.abs(h)<s)return[Math.cbrt(-u)-o];if(Math.abs(u)<s)return h<0?[-Math.sqrt(-h)-o,-o,Math.sqrt(-h)-o]:[-o];{const t=u*u/4+h*h*h/27;if(Math.abs(t)<s)return[-1.5*u/h-o,3*u/h-o];if(t>0){const a=Math.cbrt(-u/2-Math.sqrt(t));return[a-h/(3*a)-o]}{const t=2*Math.sqrt(-h/3),a=Math.acos(3*u/h/t)/3,i=2*Math.PI/3;return[t*Math.cos(a)-o,t*Math.cos(a-i)-o,t*Math.cos(a-2*i)-o]}}},t.solveLinear=(t,a)=>-a/t,t.solveQuadratic=(t,a,i,n=1e-9)=>{const s=2*t;let e=a*a-4*t*i;return e<0?[]:e<n?[-a/s]:(e=Math.sqrt(e),[(-a-e)/s,(-a+e)/s])},t.step=(t,a)=>a<t?0:1,t.subi16=(t,a)=>N((0|t)-(0|a)),t.subi32=(t,a)=>(0|t)-(0|a)|0,t.subi8=(t,a)=>F((0|t)-(0|a)),t.subu16=(t,a)=>(t&L)-(a&L)&L,t.subu32=(t,a)=>(t>>>0)-(a>>>0)>>>0,t.subu8=(t,a)=>(t&y)-(a&y)&y,t.tangentCardinal=(t,a,i=.5,n=0,s=2)=>i*((a-t)/(s-n)),t.tangentDiff3=(t,a,i,n=0,s=1,e=2)=>.5*((i-a)/(e-s)+(a-t)/(s-n)),t.trunc=t=>t<0?Math.ceil(t):Math.floor(t),t.tween=(t,a,i)=>n=>j(a,i,t(n)),t.wrap=(t,a,i)=>{if(a===i)return a;if(t>i){const n=i-a;(t-=n)>i&&(t-=n*((t-a)/n|0))}else if(t<a){const n=i-a;(t+=n)<a&&(t+=n*((a-t)/n+1|0))}return t},t.wrap01=t=>t<0?t+1:t>1?t-1:t,t.wrap11=t=>t<-1?t+2:t>1?t-2:t,t.wrapOnce=(t,a,i)=>t<a?t-a+i:t>i?t-i+a:t,t.xori16=(t,a)=>N((0|t)^(0|a)),t.xori32=(t,a)=>(0|t)^(0|a),t.xori8=(t,a)=>F((0|t)^(0|a)),t.xoru16=(t,a)=>(t&L^a&L)&L,t.xoru32=(t,a)=>(t>>>0^a>>>0)>>>0,t.xoru8=(t,a)=>(t&y^a&y)&y,Object.defineProperty(t,"__esModule",{value:!0})})); |
@@ -16,10 +16,11 @@ /** | ||
* | ||
* @param fn function to evaluate | ||
* @param error error function | ||
* @param q target value | ||
* @param res number of samples per interval | ||
* @param iter max number of iterations / recursion limit | ||
* @param start interval start | ||
* @param end interval end | ||
* @param fn - function to evaluate | ||
* @param error - error function | ||
* @param q - target value | ||
* @param res - number of samples per interval | ||
* @param iter - max number of iterations / recursion limit | ||
* @param start - interval start | ||
* @param end - interval end | ||
*/ | ||
export declare const minError: <T>(fn: (x: number) => T, error: (p: T, q: T) => number, q: T, res?: number, iter?: number, start?: number, end?: number, eps?: number) => number; | ||
//# sourceMappingURL=min-error.d.ts.map |
@@ -17,9 +17,9 @@ import { EPS } from "./api"; | ||
* | ||
* @param fn function to evaluate | ||
* @param error error function | ||
* @param q target value | ||
* @param res number of samples per interval | ||
* @param iter max number of iterations / recursion limit | ||
* @param start interval start | ||
* @param end interval end | ||
* @param fn - function to evaluate | ||
* @param error - error function | ||
* @param q - target value | ||
* @param res - number of samples per interval | ||
* @param iter - max number of iterations / recursion limit | ||
* @param start - interval start | ||
* @param end - interval end | ||
*/ | ||
@@ -26,0 +26,0 @@ export const minError = (fn, error, q, res = 16, iter = 8, start = 0, end = 1, eps = EPS) => { |
122
mix.d.ts
export declare const mix: (a: number, b: number, t: number) => number; | ||
/** | ||
* ``` | ||
* @example | ||
* ```ts | ||
* c d | ||
@@ -11,8 +12,8 @@ * +----+ | ||
* | ||
* @param a BL value | ||
* @param b BR value | ||
* @param c TL value | ||
* @param d TR value | ||
* @param u 1st interpolation factor | ||
* @param v 2nd interpolation factor | ||
* @param a - BL value | ||
* @param b - BR value | ||
* @param c - TL value | ||
* @param d - TR value | ||
* @param u - 1st interpolation factor | ||
* @param v - 2nd interpolation factor | ||
*/ | ||
@@ -29,19 +30,19 @@ export declare const mixBilinear: (a: number, b: number, c: number, d: number, u: number, v: number) => number; | ||
* Assumes all inputs are uniformly spaced. If that's not the case, use | ||
* `mixCubicHermite()` with one of the tangent generators supporting | ||
* {@link mixCubicHermite} with one of the tangent generators supporting | ||
* non-uniform spacing of points. | ||
* | ||
* See: https://www.desmos.com/calculator/j4gf8g9vkr | ||
* See: {@link https://www.desmos.com/calculator/j4gf8g9vkr} | ||
* | ||
* Source: | ||
* https://www.musicdsp.org/en/latest/Other/93-hermite-interpollation.html | ||
* {@link https://www.musicdsp.org/en/latest/Other/93-hermite-interpollation.html} | ||
* | ||
* @see mixCubicHermite | ||
* @see tangentCardinal | ||
* @see tangentDiff3 | ||
* - {@link mixCubicHermite} | ||
* - {@link tangentCardinal} | ||
* - {@link tangentDiff3} | ||
* | ||
* @param a | ||
* @param b | ||
* @param c | ||
* @param d | ||
* @param t | ||
* @param a - | ||
* @param b - | ||
* @param c - | ||
* @param d - | ||
* @param t - | ||
*/ | ||
@@ -53,17 +54,17 @@ export declare const mixHermite: (a: number, b: number, c: number, d: number, t: number) => number; | ||
* | ||
* https://en.wikipedia.org/wiki/Cubic_Hermite_spline | ||
* {@link https://en.wikipedia.org/wiki/Cubic_Hermite_spline} | ||
* | ||
* @see mixHermite | ||
* @see tangentCardinal | ||
* @see tangentDiff3 | ||
* - {@link mixHermite} | ||
* - {@link tangentCardinal} | ||
* - {@link tangentDiff3} | ||
* | ||
* @param a | ||
* @param ta | ||
* @param b | ||
* @param tb | ||
* @param t | ||
* @param a - | ||
* @param ta - | ||
* @param b - | ||
* @param tb - | ||
* @param t - | ||
*/ | ||
export declare const mixCubicHermite: (a: number, ta: number, b: number, tb: number, t: number) => number; | ||
/** | ||
* Helper function for `mixCubicHermite()`. Computes cardinal tangents | ||
* Helper function for {@link mixCubicHermite}. Computes cardinal tangents | ||
* based on point neighbors of a point B (not given), i.e. `a` | ||
@@ -75,13 +76,13 @@ * (predecessor) and `c` (successor) and their times (defaults to | ||
* | ||
* https://en.wikipedia.org/wiki/Cubic_Hermite_spline#Cardinal_spline | ||
* {@link https://en.wikipedia.org/wiki/Cubic_Hermite_spline#Cardinal_spline} | ||
* | ||
* @param prev | ||
* @param next | ||
* @param scale | ||
* @param ta | ||
* @param tc | ||
* @param prev - | ||
* @param next - | ||
* @param scale - | ||
* @param ta - | ||
* @param tc - | ||
*/ | ||
export declare const tangentCardinal: (prev: number, next: number, scale?: number, ta?: number, tc?: number) => number; | ||
/** | ||
* Helper function for `mixCubicHermite()`. Computes tangent for `curr`, | ||
* Helper function for {@link mixCubicHermite}. Computes tangent for `curr`, | ||
* based on 3-point finite difference, where `prev` & `next` are | ||
@@ -92,13 +93,13 @@ * `curr`'s neighbors and the `tX` the three points' respective time | ||
* Using this function with equal spacing of 1.0 and together with | ||
* `mixCubicHermite()` will produce same results as the somewhat | ||
* optimized variant `mixHermite()`. | ||
* {@link mixCubicHermite} will produce same results as the somewhat | ||
* optimized variant {@link mixHermite}. | ||
* | ||
* https://en.wikipedia.org/wiki/Cubic_Hermite_spline#Finite_difference | ||
* {@link https://en.wikipedia.org/wiki/Cubic_Hermite_spline#Finite_difference} | ||
* | ||
* @param prev | ||
* @param curr | ||
* @param next | ||
* @param ta | ||
* @param tb | ||
* @param tc | ||
* @param prev - | ||
* @param curr - | ||
* @param next - | ||
* @param ta - | ||
* @param tb - | ||
* @param tc - | ||
*/ | ||
@@ -111,5 +112,5 @@ export declare const tangentDiff3: (prev: number, curr: number, next: number, ta?: number, tb?: number, tc?: number) => number; | ||
* | ||
* @param f | ||
* @param from | ||
* @param to | ||
* @param f - | ||
* @param from - | ||
* @param to - | ||
*/ | ||
@@ -120,3 +121,3 @@ export declare const tween: (f: (t: number) => number, from: number, to: number) => (t: number) => number; | ||
* | ||
* @param t interpolation factor (0.0 .. 1.0) | ||
* @param t - interpolation factor (0.0 .. 1.0) | ||
*/ | ||
@@ -134,4 +135,4 @@ export declare const circular: (t: number) => number; | ||
* | ||
* @param ease easing behavior [0.0 .. ∞] | ||
* @param t | ||
* @param ease - easing behavior [0.0 .. ∞] | ||
* @param t - | ||
*/ | ||
@@ -142,3 +143,3 @@ export declare const ease: (ease: number, t: number) => number; | ||
* | ||
* @param k impulse width (higher values => shorter impulse) | ||
* @param k - impulse width (higher values => shorter impulse) | ||
*/ | ||
@@ -153,4 +154,4 @@ export declare const impulse: (k: number, t: number) => number; | ||
* | ||
* @param k | ||
* @param t | ||
* @param k - | ||
* @param t - | ||
*/ | ||
@@ -161,5 +162,16 @@ export declare const sigmoid: (k: number, t: number) => number; | ||
* | ||
* @param k | ||
* @param t | ||
* @param k - | ||
* @param t - | ||
*/ | ||
export declare const sigmoid11: (k: number, t: number) => number; | ||
/** | ||
* Computes exponential factor to interpolate from `a` to `b` over | ||
* `num` steps. I.e. multiplying `a` with the returned factor will yield | ||
* `b` after `num` steps. All args must be > 0. | ||
* | ||
* @param a | ||
* @param b | ||
* @param num | ||
*/ | ||
export declare const expFactor: (a: number, b: number, num: number) => number; | ||
//# sourceMappingURL=mix.d.ts.map |
121
mix.js
import { HALF_PI, PI } from "./api"; | ||
export const mix = (a, b, t) => a + (b - a) * t; | ||
/** | ||
* ``` | ||
* @example | ||
* ```ts | ||
* c d | ||
@@ -12,8 +13,8 @@ * +----+ | ||
* | ||
* @param a BL value | ||
* @param b BR value | ||
* @param c TL value | ||
* @param d TR value | ||
* @param u 1st interpolation factor | ||
* @param v 2nd interpolation factor | ||
* @param a - BL value | ||
* @param b - BR value | ||
* @param c - TL value | ||
* @param d - TR value | ||
* @param u - 1st interpolation factor | ||
* @param v - 2nd interpolation factor | ||
*/ | ||
@@ -38,19 +39,19 @@ export const mixBilinear = (a, b, c, d, u, v) => mix(mix(a, b, u), mix(c, d, u), v); | ||
* Assumes all inputs are uniformly spaced. If that's not the case, use | ||
* `mixCubicHermite()` with one of the tangent generators supporting | ||
* {@link mixCubicHermite} with one of the tangent generators supporting | ||
* non-uniform spacing of points. | ||
* | ||
* See: https://www.desmos.com/calculator/j4gf8g9vkr | ||
* See: {@link https://www.desmos.com/calculator/j4gf8g9vkr} | ||
* | ||
* Source: | ||
* https://www.musicdsp.org/en/latest/Other/93-hermite-interpollation.html | ||
* {@link https://www.musicdsp.org/en/latest/Other/93-hermite-interpollation.html} | ||
* | ||
* @see mixCubicHermite | ||
* @see tangentCardinal | ||
* @see tangentDiff3 | ||
* - {@link mixCubicHermite} | ||
* - {@link tangentCardinal} | ||
* - {@link tangentDiff3} | ||
* | ||
* @param a | ||
* @param b | ||
* @param c | ||
* @param d | ||
* @param t | ||
* @param a - | ||
* @param b - | ||
* @param c - | ||
* @param d - | ||
* @param t - | ||
*/ | ||
@@ -66,13 +67,13 @@ export const mixHermite = (a, b, c, d, t) => { | ||
* | ||
* https://en.wikipedia.org/wiki/Cubic_Hermite_spline | ||
* {@link https://en.wikipedia.org/wiki/Cubic_Hermite_spline} | ||
* | ||
* @see mixHermite | ||
* @see tangentCardinal | ||
* @see tangentDiff3 | ||
* - {@link mixHermite} | ||
* - {@link tangentCardinal} | ||
* - {@link tangentDiff3} | ||
* | ||
* @param a | ||
* @param ta | ||
* @param b | ||
* @param tb | ||
* @param t | ||
* @param a - | ||
* @param ta - | ||
* @param b - | ||
* @param tb - | ||
* @param t - | ||
*/ | ||
@@ -90,3 +91,3 @@ export const mixCubicHermite = (a, ta, b, tb, t) => { | ||
/** | ||
* Helper function for `mixCubicHermite()`. Computes cardinal tangents | ||
* Helper function for {@link mixCubicHermite}. Computes cardinal tangents | ||
* based on point neighbors of a point B (not given), i.e. `a` | ||
@@ -98,13 +99,13 @@ * (predecessor) and `c` (successor) and their times (defaults to | ||
* | ||
* https://en.wikipedia.org/wiki/Cubic_Hermite_spline#Cardinal_spline | ||
* {@link https://en.wikipedia.org/wiki/Cubic_Hermite_spline#Cardinal_spline} | ||
* | ||
* @param prev | ||
* @param next | ||
* @param scale | ||
* @param ta | ||
* @param tc | ||
* @param prev - | ||
* @param next - | ||
* @param scale - | ||
* @param ta - | ||
* @param tc - | ||
*/ | ||
export const tangentCardinal = (prev, next, scale = 0.5, ta = 0, tc = 2) => scale * ((next - prev) / (tc - ta)); | ||
/** | ||
* Helper function for `mixCubicHermite()`. Computes tangent for `curr`, | ||
* Helper function for {@link mixCubicHermite}. Computes tangent for `curr`, | ||
* based on 3-point finite difference, where `prev` & `next` are | ||
@@ -115,13 +116,13 @@ * `curr`'s neighbors and the `tX` the three points' respective time | ||
* Using this function with equal spacing of 1.0 and together with | ||
* `mixCubicHermite()` will produce same results as the somewhat | ||
* optimized variant `mixHermite()`. | ||
* {@link mixCubicHermite} will produce same results as the somewhat | ||
* optimized variant {@link mixHermite}. | ||
* | ||
* https://en.wikipedia.org/wiki/Cubic_Hermite_spline#Finite_difference | ||
* {@link https://en.wikipedia.org/wiki/Cubic_Hermite_spline#Finite_difference} | ||
* | ||
* @param prev | ||
* @param curr | ||
* @param next | ||
* @param ta | ||
* @param tb | ||
* @param tc | ||
* @param prev - | ||
* @param curr - | ||
* @param next - | ||
* @param ta - | ||
* @param tb - | ||
* @param tc - | ||
*/ | ||
@@ -134,5 +135,5 @@ export const tangentDiff3 = (prev, curr, next, ta = 0, tb = 1, tc = 2) => 0.5 * ((next - curr) / (tc - tb) + (curr - prev) / (tb - ta)); | ||
* | ||
* @param f | ||
* @param from | ||
* @param to | ||
* @param f - | ||
* @param from - | ||
* @param to - | ||
*/ | ||
@@ -143,3 +144,3 @@ export const tween = (f, from, to) => (t) => mix(from, to, f(t)); | ||
* | ||
* @param t interpolation factor (0.0 .. 1.0) | ||
* @param t - interpolation factor (0.0 .. 1.0) | ||
*/ | ||
@@ -163,4 +164,4 @@ export const circular = (t) => { | ||
* | ||
* @param ease easing behavior [0.0 .. ∞] | ||
* @param t | ||
* @param ease - easing behavior [0.0 .. ∞] | ||
* @param t - | ||
*/ | ||
@@ -171,3 +172,3 @@ export const ease = (ease, t) => Math.pow(t, ease); | ||
* | ||
* @param k impulse width (higher values => shorter impulse) | ||
* @param k - impulse width (higher values => shorter impulse) | ||
*/ | ||
@@ -191,4 +192,4 @@ export const impulse = (k, t) => { | ||
* | ||
* @param k | ||
* @param t | ||
* @param k - | ||
* @param t - | ||
*/ | ||
@@ -199,5 +200,15 @@ export const sigmoid = (k, t) => 1 / (1 + Math.exp(-k * (2 * t - 1))); | ||
* | ||
* @param k | ||
* @param t | ||
* @param k - | ||
* @param t - | ||
*/ | ||
export const sigmoid11 = (k, t) => 1 / (1 + Math.exp(-k * t)); | ||
/** | ||
* Computes exponential factor to interpolate from `a` to `b` over | ||
* `num` steps. I.e. multiplying `a` with the returned factor will yield | ||
* `b` after `num` steps. All args must be > 0. | ||
* | ||
* @param a | ||
* @param b | ||
* @param num | ||
*/ | ||
export const expFactor = (a, b, num) => Math.pow((b / a), (1 / num)); |
{ | ||
"name": "@thi.ng/math", | ||
"version": "1.5.1", | ||
"version": "1.6.0", | ||
"description": "Assorted common math functions & utilities", | ||
@@ -26,13 +26,15 @@ "module": "./index.js", | ||
"doc": "node_modules/.bin/typedoc --mode modules --out doc src", | ||
"doc:ae": "mkdir -p .ae/doc .ae/temp && node_modules/.bin/api-extractor run --local --verbose", | ||
"pub": "yarn build:release && yarn publish --access public" | ||
}, | ||
"devDependencies": { | ||
"@istanbuljs/nyc-config-typescript": "^0.1.3", | ||
"@types/mocha": "^5.2.6", | ||
"@types/node": "^12.12.11", | ||
"mocha": "^6.2.2", | ||
"nyc": "^14.0.0", | ||
"ts-node": "^8.5.2", | ||
"typedoc": "^0.15.2", | ||
"typescript": "^3.7.2" | ||
"@istanbuljs/nyc-config-typescript": "^1.0.1", | ||
"@microsoft/api-extractor": "^7.7.7", | ||
"@types/mocha": "^5.2.7", | ||
"@types/node": "^13.5.0", | ||
"mocha": "^7.0.0", | ||
"nyc": "^15.0.0", | ||
"ts-node": "^8.6.2", | ||
"typedoc": "^0.16.8", | ||
"typescript": "^3.7.5" | ||
}, | ||
@@ -52,3 +54,3 @@ "keywords": [ | ||
"sideEffects": false, | ||
"gitHead": "36c4d9e967bd80ccdbfa0f4a42f594080f95f105" | ||
"gitHead": "93d8af817724c1c5b06d80ffa2492fe5b4fb7bc4" | ||
} |
/** | ||
* Returns `a - b * floor(a/b)` | ||
* | ||
* @param a | ||
* @param b | ||
* @param a - | ||
* @param b - | ||
*/ | ||
@@ -12,7 +12,8 @@ export declare const fmod: (a: number, b: number) => number; | ||
/** | ||
* Only rounds `x` to nearest int if `fract(x)` < `eps` or > `1-eps`. | ||
* Only rounds `x` to nearest int if `fract(x)` <= `eps` or >= `1-eps`. | ||
* | ||
* @param x | ||
* @param eps | ||
* @param x - | ||
* @param eps - | ||
*/ | ||
export declare const roundEps: (x: number, eps?: number) => number; | ||
//# sourceMappingURL=prec.d.ts.map |
10
prec.js
@@ -5,4 +5,4 @@ import { EPS } from "./api"; | ||
* | ||
* @param a | ||
* @param b | ||
* @param a - | ||
* @param b - | ||
*/ | ||
@@ -14,6 +14,6 @@ export const fmod = (a, b) => a - b * Math.floor(a / b); | ||
/** | ||
* Only rounds `x` to nearest int if `fract(x)` < `eps` or > `1-eps`. | ||
* Only rounds `x` to nearest int if `fract(x)` <= `eps` or >= `1-eps`. | ||
* | ||
* @param x | ||
* @param eps | ||
* @param x - | ||
* @param eps - | ||
*/ | ||
@@ -20,0 +20,0 @@ export const roundEps = (x, eps = EPS) => { |
export declare const simplifyRatio: (num: number, denom: number) => number[]; | ||
//# sourceMappingURL=ratio.d.ts.map |
@@ -41,2 +41,4 @@ <!-- This file is generated - DO NOT EDIT! --> | ||
Package sizes (gzipped): ESM: 3.4KB / CJS: 3.9KB / UMD: 3.2KB | ||
## Dependencies | ||
@@ -49,3 +51,3 @@ | ||
Several demos in this repo's | ||
[/examples](https://github.com/thi-ng/umbrella/tree/master/examples) | ||
[/examples](https://github.com/thi-ng/umbrella/tree/develop/examples) | ||
directory are using this package. | ||
@@ -57,7 +59,7 @@ | ||
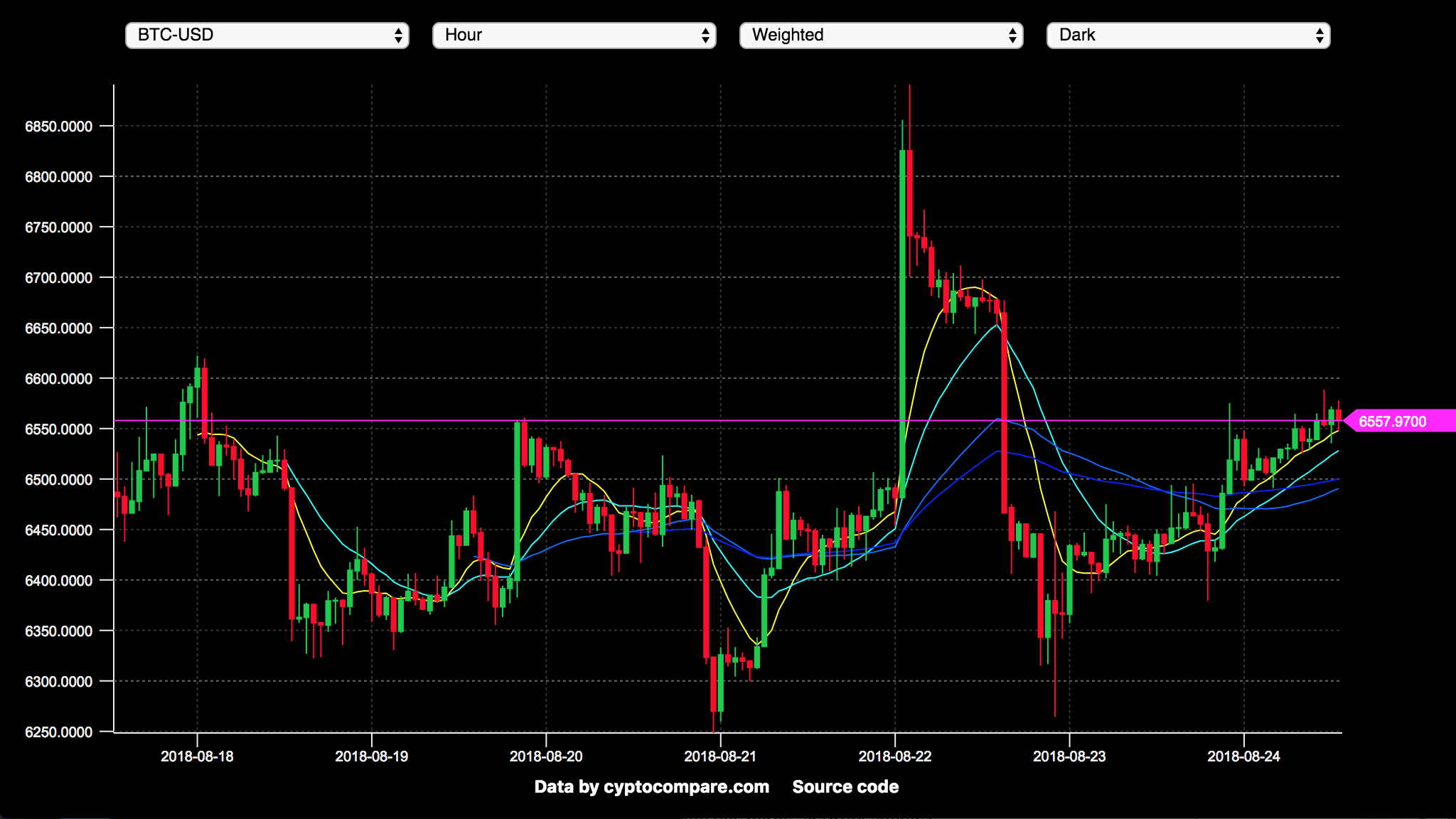 | ||
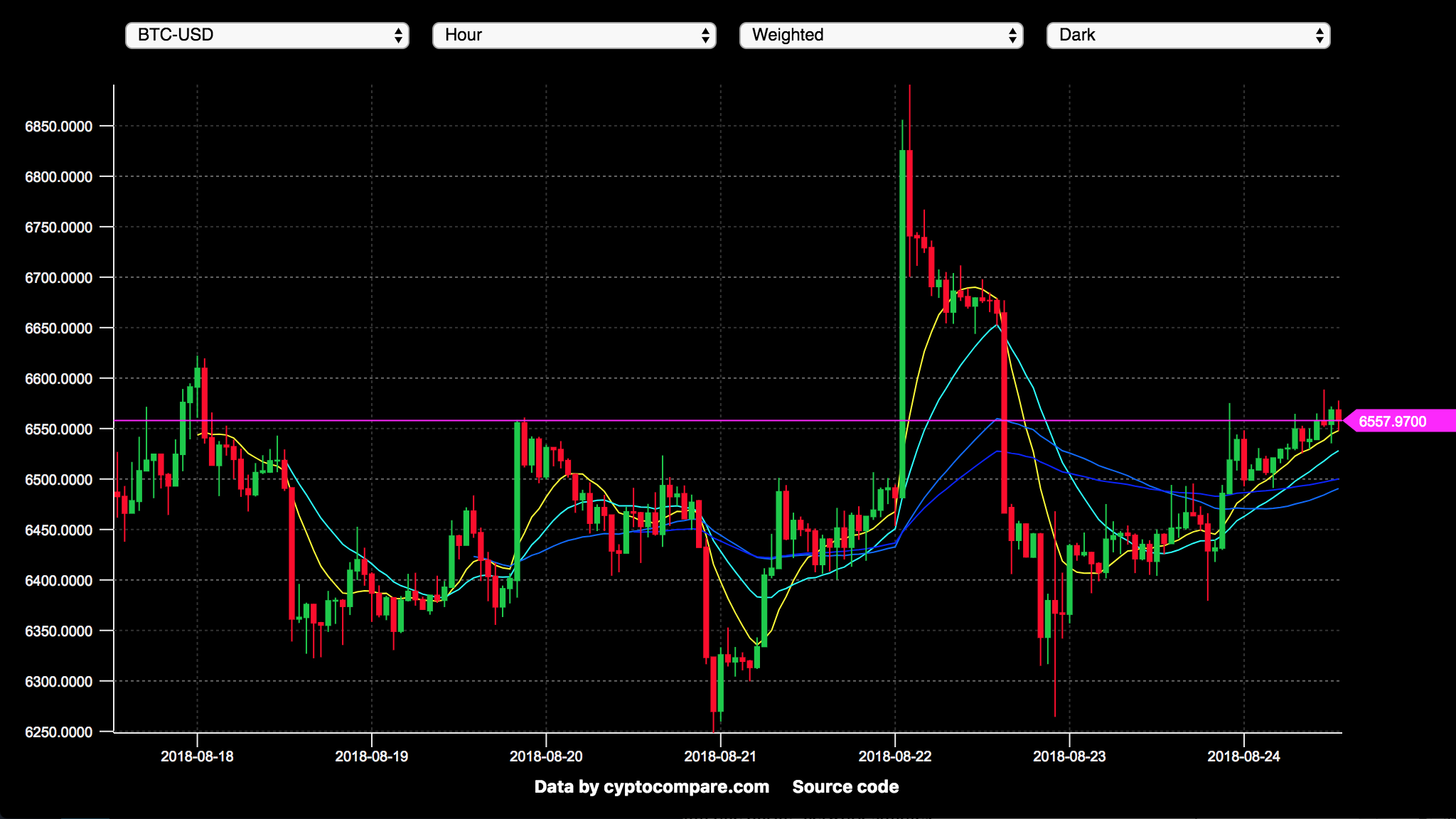 | ||
Basic crypto-currency candle chart with multiple moving averages plots | ||
[Live demo](https://demo.thi.ng/umbrella/crypto-chart/) | [Source](https://github.com/thi-ng/umbrella/tree/master/examples/crypto-chart) | ||
[Live demo](https://demo.thi.ng/umbrella/crypto-chart/) | [Source](https://github.com/thi-ng/umbrella/tree/develop/examples/crypto-chart) | ||
@@ -68,31 +70,39 @@ ### hdom-canvas-draw <!-- NOTOC --> | ||
[Live demo](https://demo.thi.ng/umbrella/hdom-canvas-draw/) | [Source](https://github.com/thi-ng/umbrella/tree/master/examples/hdom-canvas-draw) | ||
[Live demo](https://demo.thi.ng/umbrella/hdom-canvas-draw/) | [Source](https://github.com/thi-ng/umbrella/tree/develop/examples/hdom-canvas-draw) | ||
### iso-plasma <!-- NOTOC --> | ||
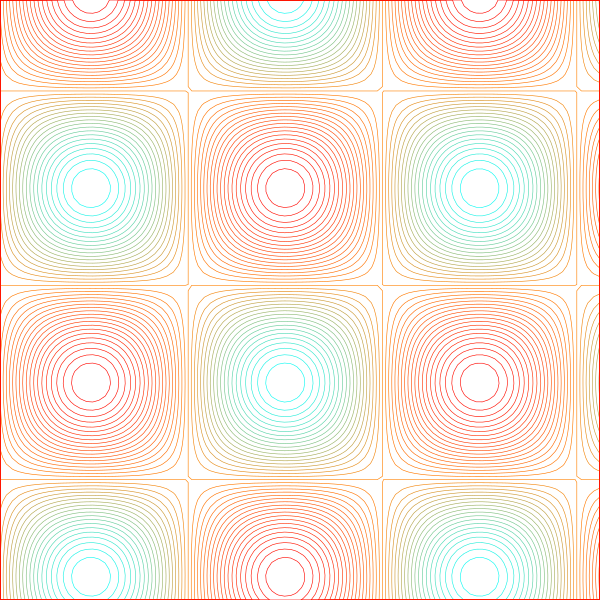 | ||
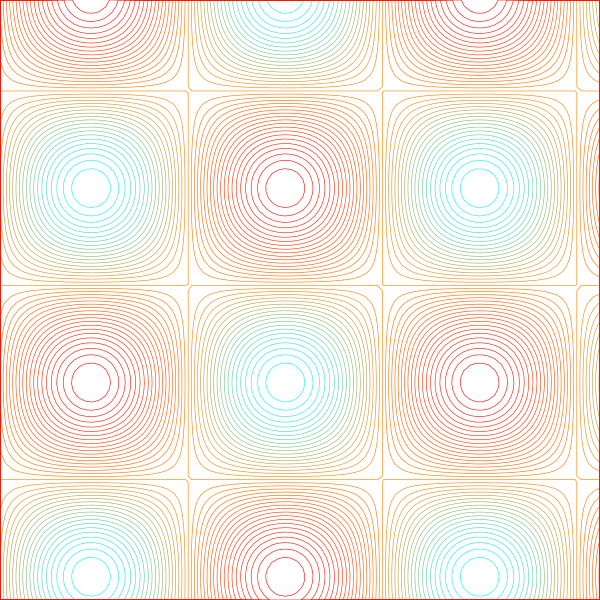 | ||
Animated sine plasma effect visualized using contour lines | ||
[Live demo](https://demo.thi.ng/umbrella/iso-plasma/) | [Source](https://github.com/thi-ng/umbrella/tree/master/examples/iso-plasma) | ||
[Live demo](https://demo.thi.ng/umbrella/iso-plasma/) | [Source](https://github.com/thi-ng/umbrella/tree/develop/examples/iso-plasma) | ||
### mandelbrot <!-- NOTOC --> | ||
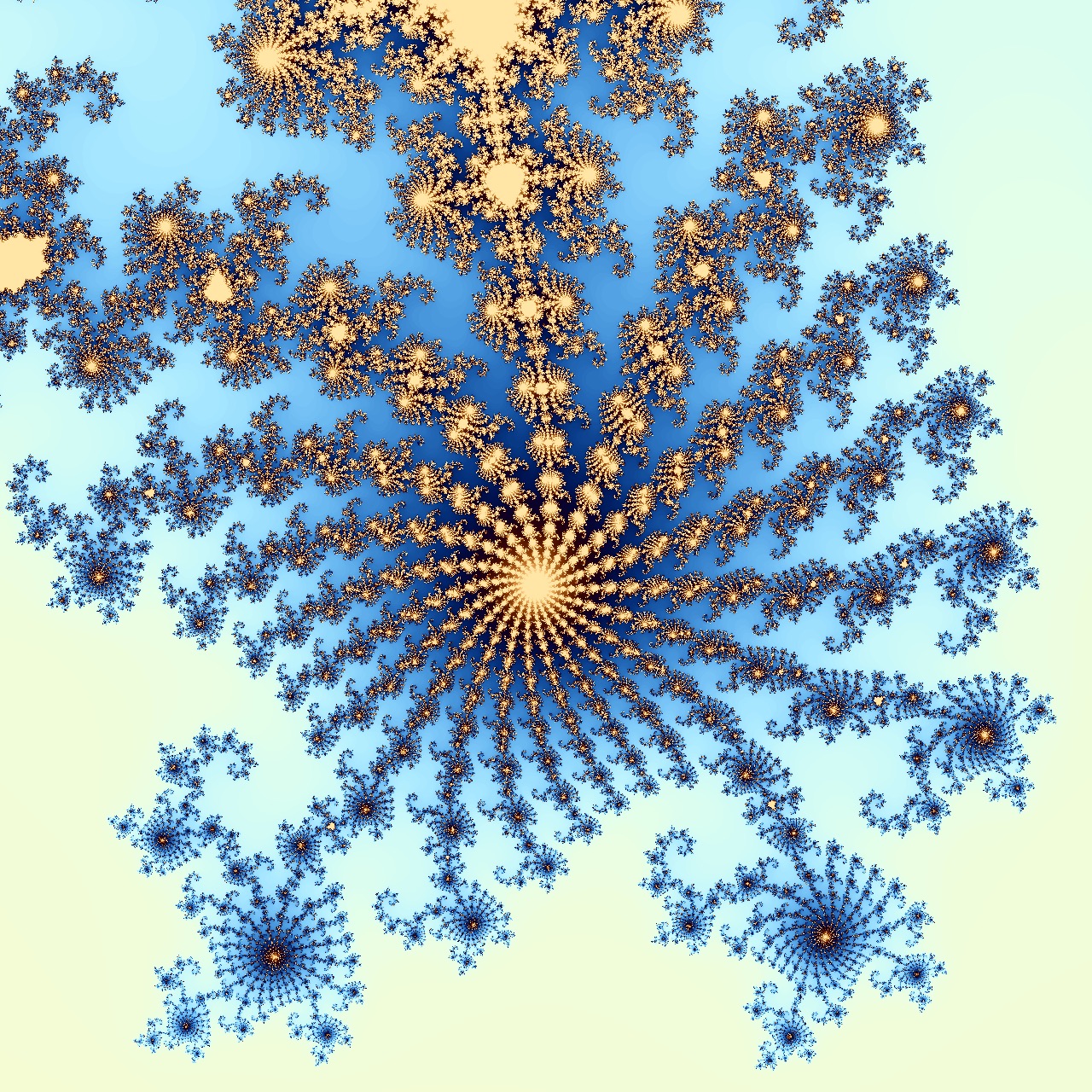 | ||
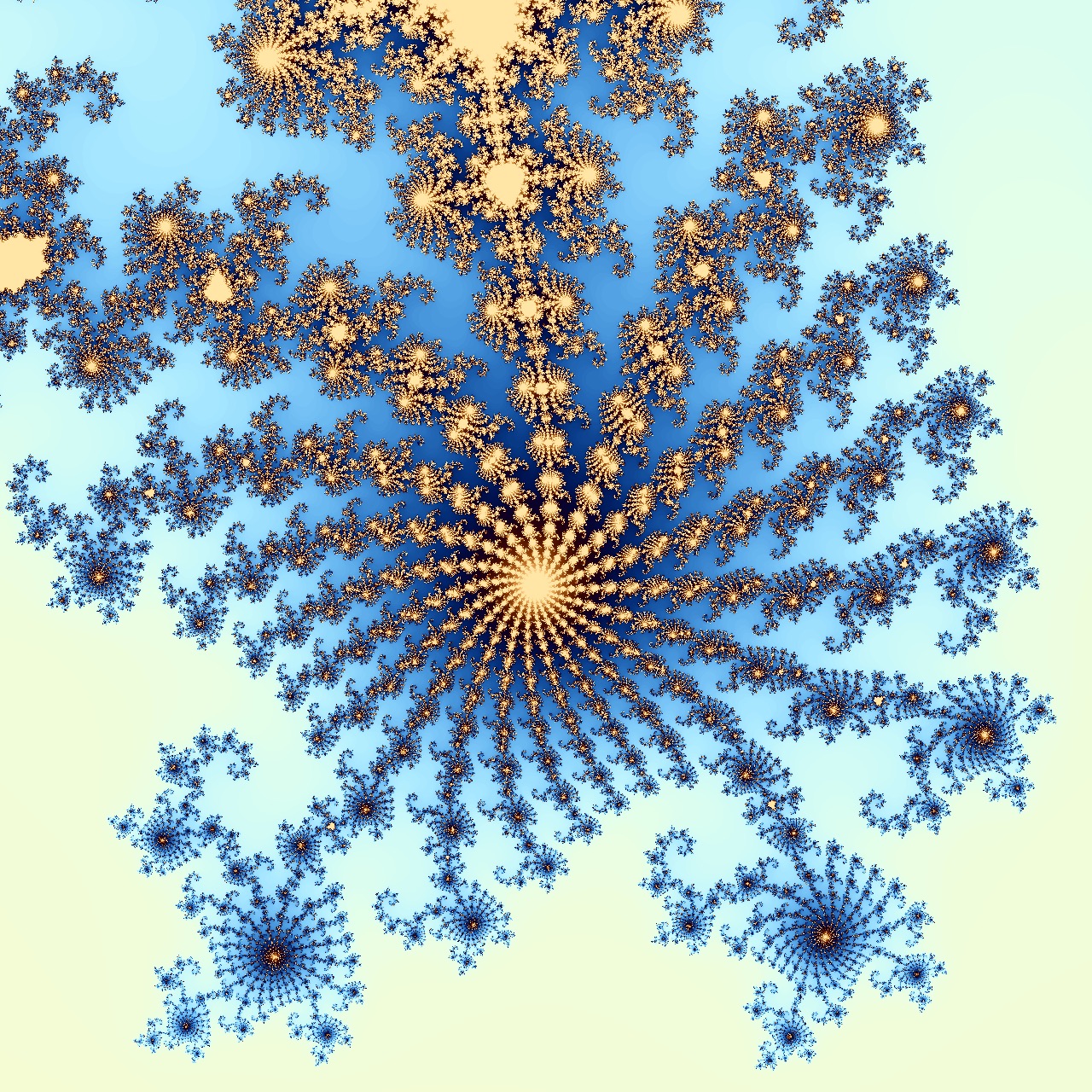 | ||
Worker based, interactive Mandelbrot visualization | ||
[Live demo](https://demo.thi.ng/umbrella/mandelbrot/) | [Source](https://github.com/thi-ng/umbrella/tree/master/examples/mandelbrot) | ||
[Live demo](https://demo.thi.ng/umbrella/mandelbrot/) | [Source](https://github.com/thi-ng/umbrella/tree/develop/examples/mandelbrot) | ||
### ramp-synth <!-- NOTOC --> | ||
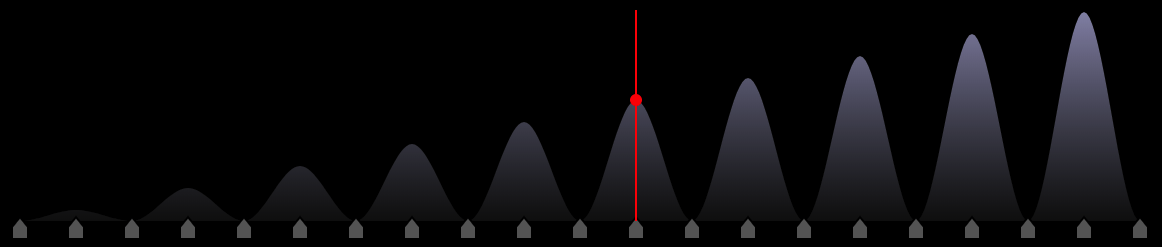 | ||
Unison wavetable synth with waveform editor | ||
[Live demo](https://demo.thi.ng/umbrella/ramp-synth/) | [Source](https://github.com/thi-ng/umbrella/tree/develop/examples/ramp-synth) | ||
### scenegraph <!-- NOTOC --> | ||
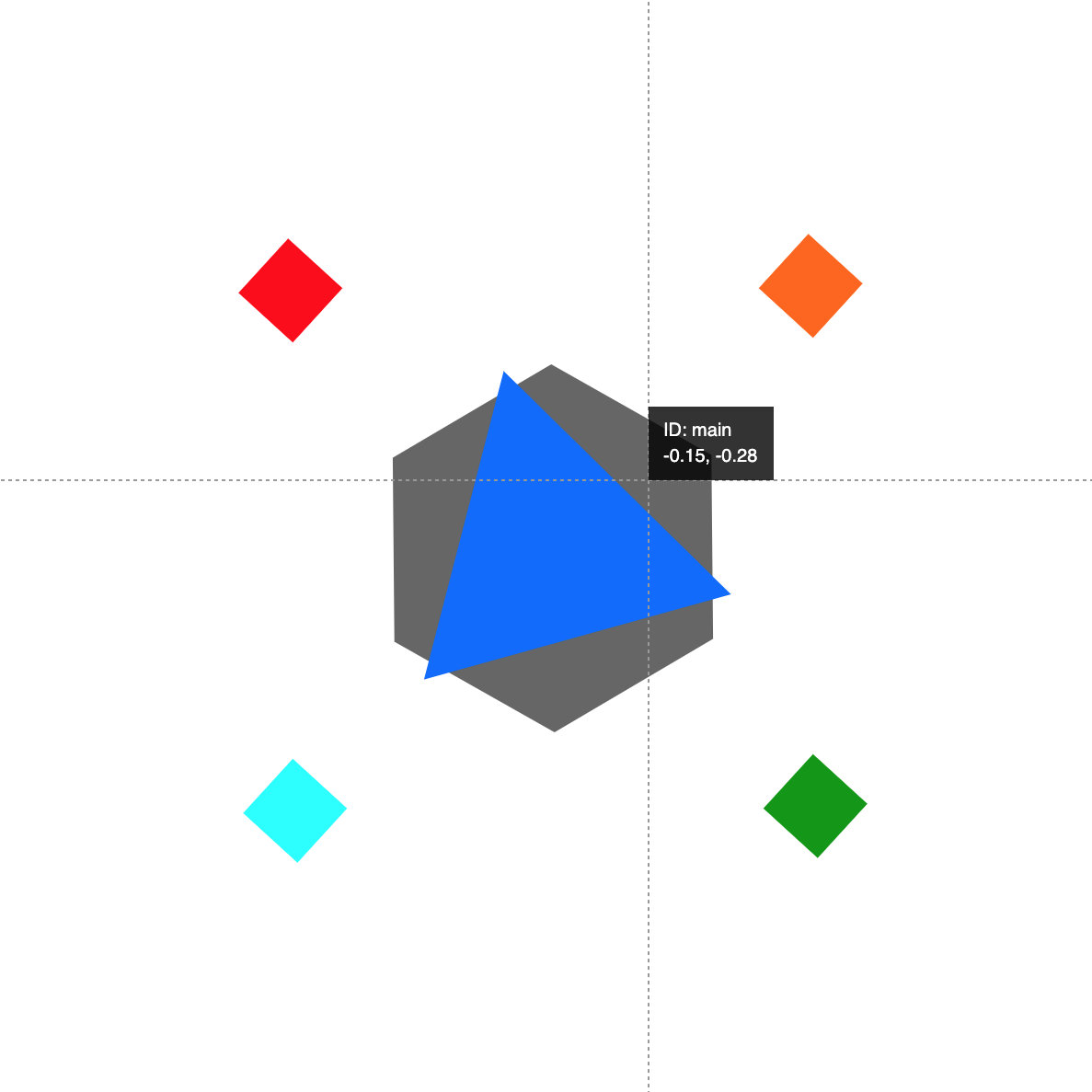 | ||
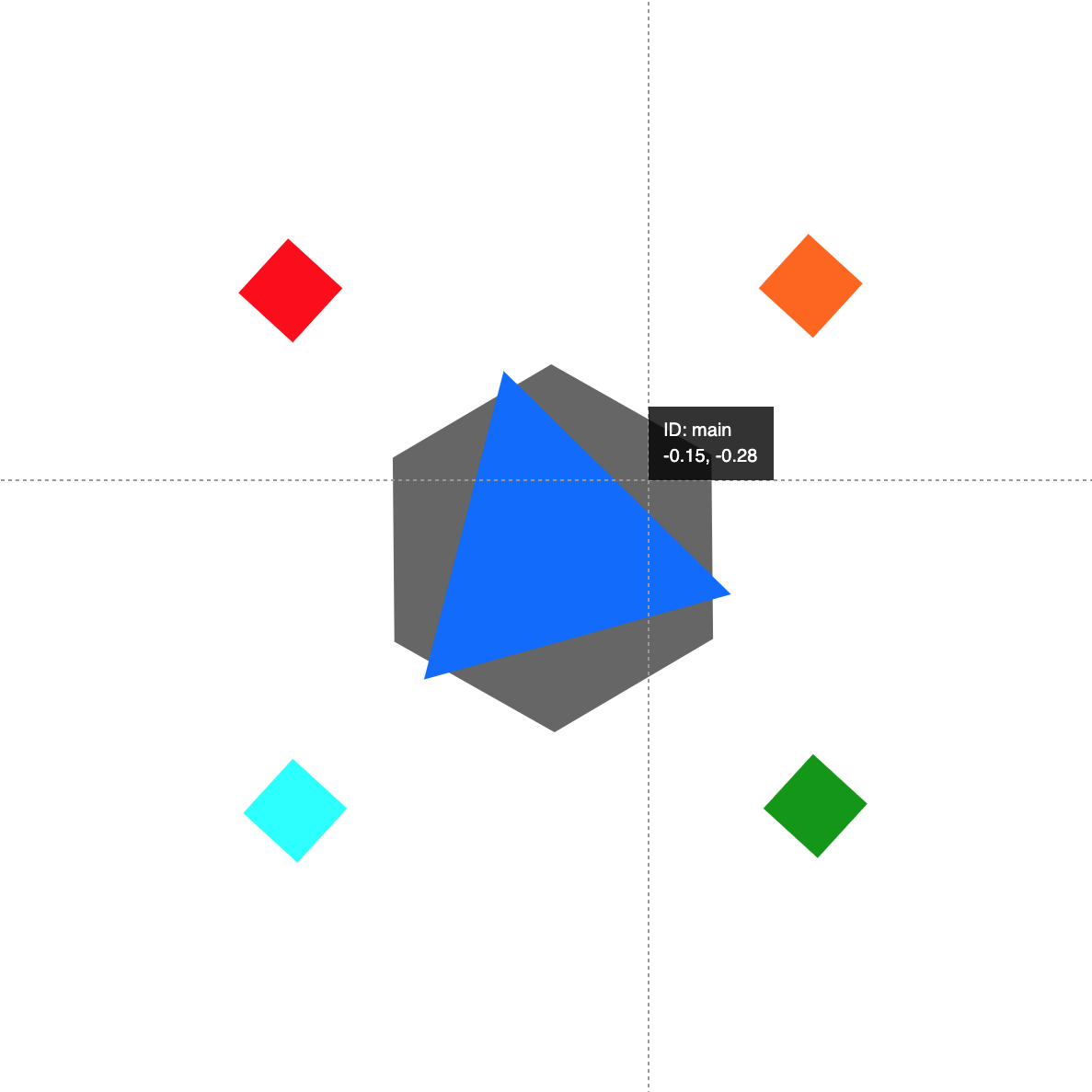 | ||
[Live demo](https://demo.thi.ng/umbrella/scenegraph/) | [Source](https://github.com/thi-ng/umbrella/tree/master/examples/scenegraph) | ||
[Live demo](https://demo.thi.ng/umbrella/scenegraph/) | [Source](https://github.com/thi-ng/umbrella/tree/develop/examples/scenegraph) | ||
### scenegraph-image <!-- NOTOC --> | ||
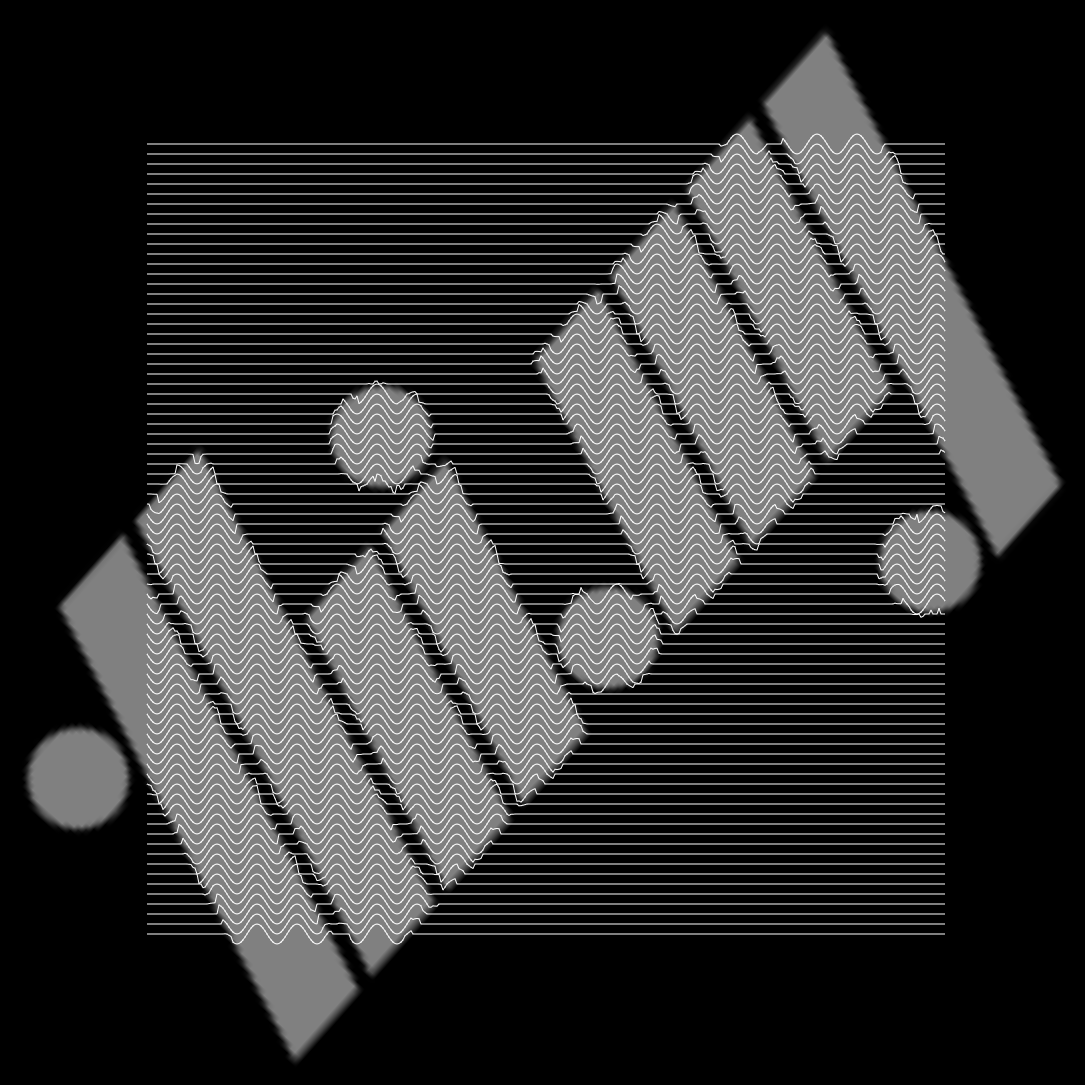 | ||
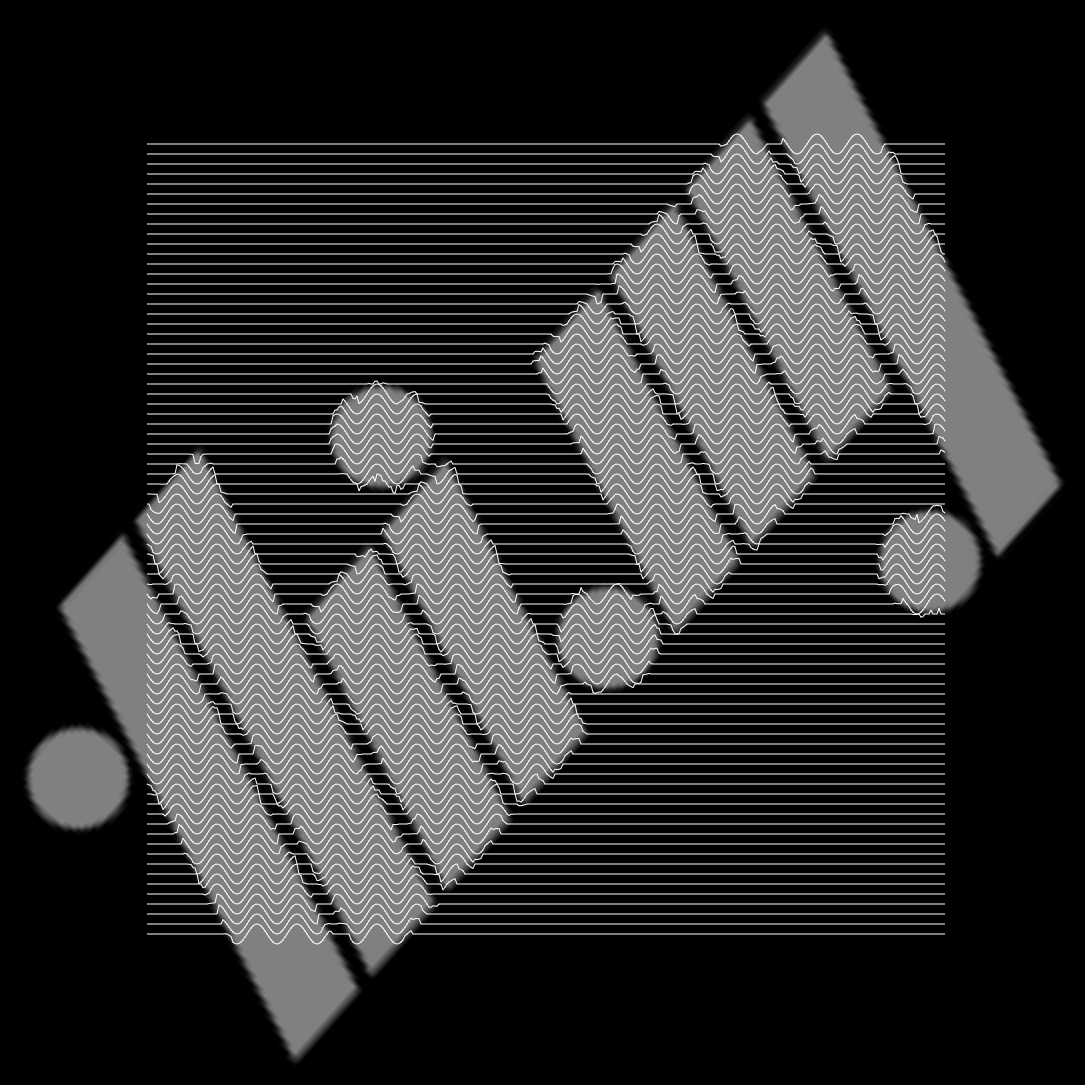 | ||
[Live demo](https://demo.thi.ng/umbrella/scenegraph-image/) | [Source](https://github.com/thi-ng/umbrella/tree/master/examples/scenegraph-image) | ||
[Live demo](https://demo.thi.ng/umbrella/scenegraph-image/) | [Source](https://github.com/thi-ng/umbrella/tree/develop/examples/scenegraph-image) | ||
@@ -117,2 +127,2 @@ ## API | ||
© 2016 - 2019 Karsten Schmidt // Apache Software License 2.0 | ||
© 2016 - 2020 Karsten Schmidt // Apache Software License 2.0 |
@@ -34,2 +34,4 @@ # ${pkg.name} | ||
${pkg.size} | ||
## Dependencies | ||
@@ -36,0 +38,0 @@ |
@@ -12,6 +12,6 @@ /** | ||
* | ||
* https://en.wikipedia.org/wiki/Derivative#Continuity_and_differentiability | ||
* {@link https://en.wikipedia.org/wiki/Derivative#Continuity_and_differentiability} | ||
* | ||
* @param fn | ||
* @param eps | ||
* @param fn - | ||
* @param eps - | ||
*/ | ||
@@ -24,4 +24,4 @@ export declare const derivative: (f: (x: number) => number, eps?: number) => (x: number) => number; | ||
* | ||
* @param a slope | ||
* @param b constant offset | ||
* @param a - slope | ||
* @param b - constant offset | ||
*/ | ||
@@ -33,11 +33,11 @@ export declare const solveLinear: (a: number, b: number) => number; | ||
* Note: `a` MUST NOT be zero. If the quadratic term is missing, | ||
* use `solveLinear` instead. | ||
* use {@link solveLinear} instead. | ||
* | ||
* https://en.wikipedia.org/wiki/Quadratic_function | ||
* https://en.wikipedia.org/wiki/Quadratic_equation | ||
* - {@link https://en.wikipedia.org/wiki/Quadratic_function} | ||
* - {@link https://en.wikipedia.org/wiki/Quadratic_equation} | ||
* | ||
* @param a quadratic coefficient | ||
* @param b linear coefficient | ||
* @param c constant offset | ||
* @param eps tolerance to determine multiple roots | ||
* @param a - quadratic coefficient | ||
* @param b - linear coefficient | ||
* @param c - constant offset | ||
* @param eps - tolerance to determine multiple roots | ||
*/ | ||
@@ -49,12 +49,13 @@ export declare const solveQuadratic: (a: number, b: number, c: number, eps?: number) => number[]; | ||
* Note: `a` MUST NOT be zero. If the cubic term is missing (i.e. zero), | ||
* use `solveQuadratic` or `solveLinear` instead. | ||
* use {@link solveQuadratic} or {@link solveLinear} instead. | ||
* | ||
* https://en.wikipedia.org/wiki/Cubic_function | ||
* {@link https://en.wikipedia.org/wiki/Cubic_function} | ||
* | ||
* @param a cubic coefficient | ||
* @param b quadratic coefficient | ||
* @param c linear coefficient | ||
* @param d constant offset | ||
* @param eps tolerance to determine multiple roots | ||
* @param a - cubic coefficient | ||
* @param b - quadratic coefficient | ||
* @param c - linear coefficient | ||
* @param d - constant offset | ||
* @param eps - tolerance to determine multiple roots | ||
*/ | ||
export declare const solveCubic: (a: number, b: number, c: number, d: number, eps?: number) => number[]; | ||
//# sourceMappingURL=solve.d.ts.map |
38
solve.js
@@ -13,6 +13,6 @@ import { EPS } from "./api"; | ||
* | ||
* https://en.wikipedia.org/wiki/Derivative#Continuity_and_differentiability | ||
* {@link https://en.wikipedia.org/wiki/Derivative#Continuity_and_differentiability} | ||
* | ||
* @param fn | ||
* @param eps | ||
* @param fn - | ||
* @param eps - | ||
*/ | ||
@@ -25,4 +25,4 @@ export const derivative = (f, eps = EPS) => (x) => (f(x + eps) - f(x)) / eps; | ||
* | ||
* @param a slope | ||
* @param b constant offset | ||
* @param a - slope | ||
* @param b - constant offset | ||
*/ | ||
@@ -34,11 +34,11 @@ export const solveLinear = (a, b) => -b / a; | ||
* Note: `a` MUST NOT be zero. If the quadratic term is missing, | ||
* use `solveLinear` instead. | ||
* use {@link solveLinear} instead. | ||
* | ||
* https://en.wikipedia.org/wiki/Quadratic_function | ||
* https://en.wikipedia.org/wiki/Quadratic_equation | ||
* - {@link https://en.wikipedia.org/wiki/Quadratic_function} | ||
* - {@link https://en.wikipedia.org/wiki/Quadratic_equation} | ||
* | ||
* @param a quadratic coefficient | ||
* @param b linear coefficient | ||
* @param c constant offset | ||
* @param eps tolerance to determine multiple roots | ||
* @param a - quadratic coefficient | ||
* @param b - linear coefficient | ||
* @param c - constant offset | ||
* @param eps - tolerance to determine multiple roots | ||
*/ | ||
@@ -58,11 +58,11 @@ export const solveQuadratic = (a, b, c, eps = 1e-9) => { | ||
* Note: `a` MUST NOT be zero. If the cubic term is missing (i.e. zero), | ||
* use `solveQuadratic` or `solveLinear` instead. | ||
* use {@link solveQuadratic} or {@link solveLinear} instead. | ||
* | ||
* https://en.wikipedia.org/wiki/Cubic_function | ||
* {@link https://en.wikipedia.org/wiki/Cubic_function} | ||
* | ||
* @param a cubic coefficient | ||
* @param b quadratic coefficient | ||
* @param c linear coefficient | ||
* @param d constant offset | ||
* @param eps tolerance to determine multiple roots | ||
* @param a - cubic coefficient | ||
* @param b - quadratic coefficient | ||
* @param c - linear coefficient | ||
* @param d - constant offset | ||
* @param eps - tolerance to determine multiple roots | ||
*/ | ||
@@ -69,0 +69,0 @@ export const solveCubic = (a, b, c, d, eps = 1e-9) => { |
/** | ||
* Step/threshold function. | ||
* | ||
* @param edge threshold | ||
* @param x test value | ||
* @param edge - threshold | ||
* @param x - test value | ||
* @returns 0, if `x < e`, else 1 | ||
@@ -12,5 +12,5 @@ */ | ||
* | ||
* @param edge lower threshold | ||
* @param edge2 upper threshold | ||
* @param x test value | ||
* @param edge - lower threshold | ||
* @param edge2 - upper threshold | ||
* @param x - test value | ||
* @returns 0, if `x < edge1`, 1 if `x > edge2`, else sigmoid interpolation | ||
@@ -20,7 +20,7 @@ */ | ||
/** | ||
* Similar to `smoothStep()` but using different polynomial. | ||
* Similar to {@link smoothStep} but using different polynomial. | ||
* | ||
* @param edge | ||
* @param edge2 | ||
* @param x | ||
* @param edge - | ||
* @param edge2 - | ||
* @param x - | ||
*/ | ||
@@ -36,6 +36,7 @@ export declare const smootherStep: (edge: number, edge2: number, x: number) => number; | ||
* | ||
* @param k | ||
* @param n | ||
* @param x | ||
* @param k - | ||
* @param n - | ||
* @param x - | ||
*/ | ||
export declare const expStep: (k: number, n: number, x: number) => number; | ||
//# sourceMappingURL=step.d.ts.map |
24
step.js
@@ -5,4 +5,4 @@ import { clamp01 } from "./interval"; | ||
* | ||
* @param edge threshold | ||
* @param x test value | ||
* @param edge - threshold | ||
* @param x - test value | ||
* @returns 0, if `x < e`, else 1 | ||
@@ -14,5 +14,5 @@ */ | ||
* | ||
* @param edge lower threshold | ||
* @param edge2 upper threshold | ||
* @param x test value | ||
* @param edge - lower threshold | ||
* @param edge2 - upper threshold | ||
* @param x - test value | ||
* @returns 0, if `x < edge1`, 1 if `x > edge2`, else sigmoid interpolation | ||
@@ -25,7 +25,7 @@ */ | ||
/** | ||
* Similar to `smoothStep()` but using different polynomial. | ||
* Similar to {@link smoothStep} but using different polynomial. | ||
* | ||
* @param edge | ||
* @param edge2 | ||
* @param x | ||
* @param edge - | ||
* @param edge2 - | ||
* @param x - | ||
*/ | ||
@@ -44,6 +44,6 @@ export const smootherStep = (edge, edge2, x) => { | ||
* | ||
* @param k | ||
* @param n | ||
* @param x | ||
* @param k - | ||
* @param n - | ||
* @param x - | ||
*/ | ||
export const expStep = (k, n, x) => 1 - Math.exp(-k * Math.pow(x, n)); |
Sorry, the diff of this file is not supported yet
Sorry, the diff of this file is not supported yet
License Policy Violation
LicenseThis package is not allowed per your license policy. Review the package's license to ensure compliance.
Found 1 instance in 1 package
License Policy Violation
LicenseThis package is not allowed per your license policy. Review the package's license to ensure compliance.
Found 1 instance in 1 package
167058
2422
124
9
42