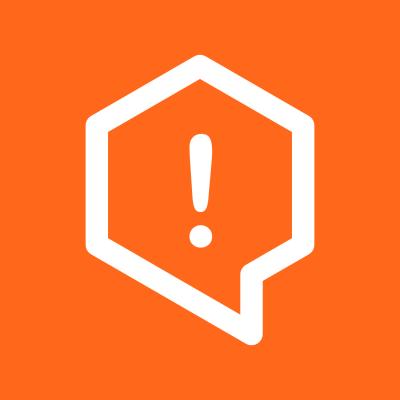
Security News
Fluent Assertions Faces Backlash After Abandoning Open Source Licensing
Fluent Assertions is facing backlash after dropping the Apache license for a commercial model, leaving users blindsided and questioning contributor rights.
@thream/socketio-jwt
Advanced tools
Authenticate socket.io incoming connections with JWTs.
⚠️ This project is not maintained anymore, you can still use the code as you wish and fork it to maintain it yourself.
Authenticate socket.io incoming connections with JWTs.
This repository was originally forked from auth0-socketio-jwt and it is not intended to take any credit but to improve the code from now on.
Note: It is a package that is recommended to use/install on both the client and server sides.
npm install --save @thream/socketio-jwt
import { Server } from "socket.io"
import { authorize } from "@thream/socketio-jwt"
const io = new Server(9000)
io.use(
authorize({
secret: "your secret or public key",
}),
)
io.on("connection", async (socket) => {
// jwt payload of the connected client
console.log(socket.decodedToken)
const clients = await io.sockets.allSockets()
if (clients != null) {
for (const clientId of clients) {
const client = io.sockets.sockets.get(clientId)
client?.emit("messages", { message: "Success!" })
// we can access the jwt payload of each connected client
console.log(client?.decodedToken)
}
}
})
jwks-rsa
(example)import jwksClient from "jwks-rsa"
import { Server } from "socket.io"
import { authorize } from "@thream/socketio-jwt"
const client = jwksClient({
jwksUri: "https://sandrino.auth0.com/.well-known/jwks.json",
})
const io = new Server(9000)
io.use(
authorize({
secret: async (decodedToken) => {
const key = await client.getSigningKeyAsync(decodedToken.header.kid)
return key.getPublicKey()
},
}),
)
io.on("connection", async (socket) => {
// jwt payload of the connected client
console.log(socket.decodedToken)
// You can do the same things of the previous example there...
})
onAuthentication
(example)import { Server } from "socket.io"
import { authorize } from "@thream/socketio-jwt"
const io = new Server(9000)
io.use(
authorize({
secret: "your secret or public key",
onAuthentication: async (decodedToken) => {
// return the object that you want to add to the user property
// or throw an error if the token is unauthorized
},
}),
)
io.on("connection", async (socket) => {
// jwt payload of the connected client
console.log(socket.decodedToken)
// You can do the same things of the previous example there...
// user object returned in onAuthentication
console.log(socket.user)
})
authorize
optionssecret
is a string containing the secret for HMAC algorithms, or a function that should fetch the secret or public key as shown in the example with jwks-rsa
.algorithms
(default: HS256
)onAuthentication
is a function that will be called with the decodedToken
as a parameter after the token is authenticated. Return a value to add to the user
property in the socket object.import { io } from "socket.io-client"
import { isUnauthorizedError } from "@thream/socketio-jwt/build/UnauthorizedError.js"
// Require Bearer Token
const socket = io("http://localhost:9000", {
auth: { token: `Bearer ${yourJWT}` },
})
// Handling token expiration
socket.on("connect_error", (error) => {
if (isUnauthorizedError(error)) {
console.log("User token has expired")
}
})
// Listening to events
socket.on("messages", (data) => {
console.log(data)
})
Anyone can help to improve the project, submit a Feature Request, a bug report or even correct a simple spelling mistake.
The steps to contribute can be found in the CONTRIBUTING.md file.
FAQs
Authenticate socket.io incoming connections with JWTs.
We found that @thream/socketio-jwt demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Fluent Assertions is facing backlash after dropping the Apache license for a commercial model, leaving users blindsided and questioning contributor rights.
Research
Security News
Socket researchers uncover the risks of a malicious Python package targeting Discord developers.
Security News
The UK is proposing a bold ban on ransomware payments by public entities to disrupt cybercrime, protect critical services, and lead global cybersecurity efforts.