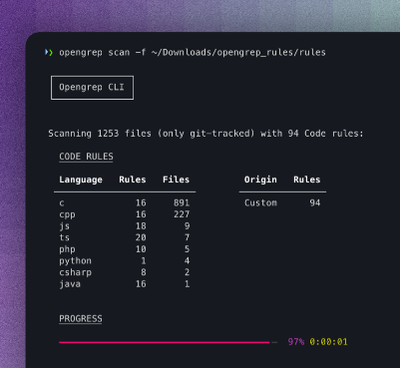
Security News
Opengrep Emerges as Open Source Alternative Amid Semgrep Licensing Controversy
Opengrep forks Semgrep to preserve open source SAST in response to controversial licensing changes.
@times-components/tracking
Advanced tools
Tracking is a collection of Higher-Order-Components (HOCs) that extract and report analytics of a component. All HOCs consume a component and an object describing how to create the analytics event.
The returned component expects an analyticsStream
to be available in React
context or to be passed directly to the component via props. Every tracking HOC
passes it's analyticsStream
to it's descendants via React context.
n.b. while there is a "tracking context" that uses "React context", they are not the same thing. The "tracking context" can be thought of as a "decoration boundary" between logical business layers which are useful for reporting.
How to implement tracking: +------------------------------------------+ | | | Tracking context HOC | | | | +----------------------------------+ | | | | | | | Tracking HOC - what events | | | | should be sent? | | | | +-------------------------+ | | | | | Component | | | | | | | | | | | +-------------------------+ | | | | | | | +----------------------------------+ | | | +------------------------------------------+ Compose for this architecture: | | | v +-------------------------------------------------------------------+ | | | Last tracking context - send to a reporter (such as Tealium) | | | | +-------------------------------------------------------------+ | | | Tracking context - edition information added | | | | | | | | +-------------------------------------------------------+ | | | | | | | | | | | Component - knows nothing about tracking which | | | | | | is passed via context | | | | | | +------------------------------------------+ | | | | | | | Tracking context - section information | | | | | | | | added | | | | | | | | +---------------------------+ | | | | | | | | | | | | | | | | | | | | | | | | | | | | | Component with | | | | | | | | | | tracking HOC sends click | | | | | | | | | | events | | | | | | | | | +---------------------------+ | | | | | | | | | | | | | | | | | | | | | | | +------------------------------------------+ | | | | | +-------------------------------------------------------+ | | | +-------------------------------------------------------------+ | +-------------------------------------------------------------------+
The analyticsStream
prop is expected to be a function that consumes an event
object.
To test whether your component propagates the correct events, pass jest.fn()
as the analyticsStream
and test if your mock received the expected events.
Mind that you need to mock the Date
and setTimeout
to make your tests
reproducible.
const events = jest.fn();
renderer.create(<ComponentWithTracking analyticsStream={events} />);
expect(events.mock.calls).toMatchObject(...);
Please read CONTRIBUTING.md before contributing to this package
Please see our main README.md to get the project running locally
The code can be formatted and linted in accordance with the agreed standards.
yarn fmt
yarn lint
This package uses yarn (latest) to run unit tests on each platform with jest.
yarn test:web
Visit the official storybook to see our available tracking templates.
FAQs
Tracking
The npm package @times-components/tracking receives a total of 1,252 weekly downloads. As such, @times-components/tracking popularity was classified as popular.
We found that @times-components/tracking demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Opengrep forks Semgrep to preserve open source SAST in response to controversial licensing changes.
Security News
Critics call the Node.js EOL CVE a misuse of the system, sparking debate over CVE standards and the growing noise in vulnerability databases.
Security News
cURL and Go security teams are publicly rejecting CVSS as flawed for assessing vulnerabilities and are calling for more accurate, context-aware approaches.