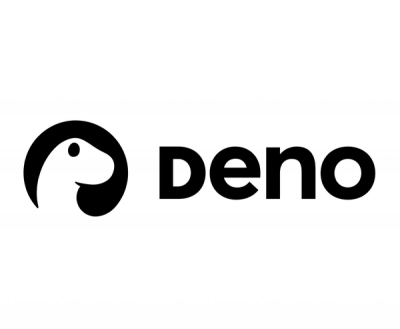
Security News
Deno 2.2 Improves Dependency Management and Expands Node.js Compatibility
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
@tokenbound/sdk
Advanced tools
An SDK for interacting with [ERC-6551 accounts](https://eips.ethereum.org/EIPS/eip-6551) using viem.
An SDK for interacting with ERC-6551 accounts using viem.
$ npm install @tokenbound/sdk
Using viem's WalletClient:
import { TokenboundClient } from "@tokenbound/sdk";
import { goerli } from 'viem/chains'
const tokenboundClient = new TokenboundClient({ walletClient, chainId: goerli.id });
or, with a legacy Wagmi / Ethers signer:
import { TokenboundClient } from "@tokenbound/sdk";
const tokenboundClient = new TokenboundClient({ signer, chainId: 1 });
import { TokenboundClient } from "@tokenbound/sdk";
import { goerli } from 'viem/chains';
const tokenboundClient = new TokenboundClient({ walletClient, chainId: goerli.id });
const tokenBoundAccount = tokenboundClient.getAccount({
tokenContract: "<token_contract_address>",
tokenId: "<token_id>",
});
import { prepareExecuteCall } from "@tokenbound/sdk";
const to = "0xe7134a029cd2fd55f678d6809e64d0b6a0caddcb"; // any address
const value = 0n; // amount of ETH to send in WEI
const data = ""; // calldata
const preparedCall = await tokenboundClient.prepareExecuteCall({
account: "<account_address>",
to: "<recipient_address>",
value: value,
data: data,
});
// Execute encoded call
const hash = await walletClient.sendTransaction(preparedCall);
The SDK supports custom 6551 implementations.
If you've deployed your own implementation, you can optionally pass custom configuration parameters when instantiating your TokenboundClient:
import { TokenboundClient } from "@tokenbound/sdk";
const tokenboundClient = new TokenboundClient({
signer: <signer>,
chainId: <chainId>,
implementationAddress: "<custom_implementation_address>",
})
// Custom implementation AND custom registry (uncommon for most implementations)
const tokenboundClientWithCustomRegistry = new TokenboundClient({
signer: <signer>,
chainId: <chainId>,
implementationAddress: "<custom_implementation_address>",
registryAddress: "<custom_registry_address>",
})
### Documentation
See the [Tokenbound docs](https://docs.tokenbound.org/sdk/installation) for complete documentation.
FAQs
An SDK for interacting with [ERC-6551 accounts](https://eips.ethereum.org/EIPS/eip-6551) using viem.
The npm package @tokenbound/sdk receives a total of 545 weekly downloads. As such, @tokenbound/sdk popularity was classified as not popular.
We found that @tokenbound/sdk demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
Security News
React's CRA deprecation announcement sparked community criticism over framework recommendations, leading to quick updates acknowledging build tools like Vite as valid alternatives.
Security News
Ransomware payment rates hit an all-time low in 2024 as law enforcement crackdowns, stronger defenses, and shifting policies make attacks riskier and less profitable.